File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
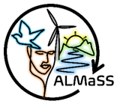 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
187 #ifdef __VoleStarvationDays
189 int m_StarvationDays;
240 #ifdef __VoleStarvationDays
243 static int m_MaxStarvationDays;
246 #ifdef __VOLEPESTICIDEON
248 double SupplyPesticideLoad() {
return m_pesticideload; }
249 double SupplyBioDegradeRate() {
return m_pesticideBioDegradeRate; }
250 void SetPesticideInfluenced1(
bool a_bool) { m_pesticideInfluenced1 = a_bool; }
251 void SetPesticideInfluenced2(
bool a_bool) { m_pesticideInfluenced2 = a_bool; }
252 bool GetPesticideInfluenced1() {
return m_pesticideInfluenced1; }
253 bool GetPesticideInfluenced2() {
return m_pesticideInfluenced2; }
255 bool m_pesticideInfluenced1;
256 bool m_pesticideInfluenced2;
257 double m_pesticideInfluenced3;
258 double m_pesticideload;
259 double m_pesticideBioDegradeRate;
260 void AddToPesticidLoad(
double a_pestamount) { m_pesticideload+=a_pestamount; }
261 void BioDegradePesticide() { m_pesticideload *= m_pesticideBioDegradeRate; }
262 void ClearPesticidLoad() { m_pesticideload = 0.0; }
263 void SetBioDegradeRate(
double a_rate) { m_pesticideBioDegradeRate = a_rate; }
264 virtual void PesticideIngestion(
void );
265 virtual void ActOnPesticideDose(
void );
266 virtual void ModelinkPesticide() { ; }
267 virtual void ModelinkPesticide21TWA(
double ) { ; }
268 virtual void Vinclozolin(
double ) { ; }
269 virtual void GeneralOrganophosphate(
double ) { ; }
270 virtual void GeneralEndocrineDisruptor(
double ) { ; }
271 virtual void GeneticDemoPesticide(
double ){ ; }
273 #ifdef __VOLERODENTICIDEON
435 void MoveTo(
int p_Vector,
int p_Distance,
int iterations);
436 void DoWalking(
int p_Distance,
int& p_Vector,
int& vx,
int& vy)
const;
437 void DoWalkingCorrect(
int p_Distance,
int& p_Vector,
int& vx,
int& vy)
const;
438 void Escape(
int p_Vector,
int p_Distance);
465 void Step()
override;
479 #ifdef __VOLEPESTICIDEON
480 virtual void ModelinkPesticide();
481 virtual void ModelinkPesticide21TWA(
double a_dose);
482 virtual void Vinclozolin(
double a_dose);
483 virtual void GeneralOrganophosphate(
double a_dose);
484 virtual void GeneralEndocrineDisruptor(
double );
485 virtual void GeneticDemoPesticide(
double );
506 void Step()
override;
517 #ifdef __VOLEPESTICIDEON
518 virtual void ModelinkPesticide();
519 virtual void ModelinkPesticide21TWA(
double a_dose);
520 virtual void Vinclozolin(
double a_dose);
521 virtual void GeneralOrganophosphate(
double a_dose);
522 virtual void GeneralEndocrineDisruptor(
double );
523 virtual void GeneticDemoPesticide(
double );
543 void Step()
override;
549 int Dispersal(
double p_OldQual,
int p_Distance);
556 #ifdef __VOLEPESTICIDEON
557 double m_maturitydelay;
558 virtual void ModelinkPesticide();
559 virtual void ModelinkPesticide21TWA(
double a_dose);
560 virtual void Vinclozolin(
double a_dose);
561 virtual void GeneralOrganophosphate(
double a_dose);
562 virtual void GeneralEndocrineDisruptor(
double );
563 virtual void GeneticDemoPesticide(
double );
565 void SetMaturityDelay(
double a_delay) { m_maturitydelay = a_delay; }
583 void Step()
override;
635 #ifdef __VOLEPESTICIDEON
636 unsigned int m_pesticideloadindex;
637 double m_pesticideloadarray[21];
638 virtual void ModelinkPesticide();
639 virtual void ModelinkPesticide21TWA(
double a_dose);
640 virtual void Vinclozolin(
double a_dose);
641 virtual void GeneralOrganophosphate(
double a_dose);
642 virtual void GeneralEndocrineDisruptor(
double );
643 static int m_EndoCrineDisruptionGestationLength;
644 virtual void GeneticDemoPesticide(
double );
const double growthperdayM
Definition: Vole_all.cpp:133
int SupplyXBorn()
Definition: vole_all.h:347
int m_LifeSpan
Definition: vole_all.h:182
void Set_ElemBorn(int a_Location_x, int a_Location_y)
Definition: vole_all.h:312
Definition: vole_all.h:96
virtual void RodenticideIngestion(void)
Definition: Vole_all.cpp:3115
Definition: Treatment.h:129
static CfgFloat cfg_PesticideAccumulationThreshold("VOLE_PESTICDEACCUMULATIONTHRESHOLD", CFG_CUSTOM, 20.0)
Definition: Treatment.h:64
Definition: VolePopulationManager.h:84
unsigned m_MotherId
Definition: vole_all.h:158
Definition: Treatment.h:84
TTypeOfVoleState
Vole behavioural states.
Definition: vole_all.h:54
Definition: Treatment.h:71
int MinReproAgeM
Definition: Vole_all.cpp:139
Definition: Treatment.h:88
Definition: Treatment.h:68
Definition: MapErrorMsg.h:37
void EndStep() override
Male vole EndStep.
Definition: Vole_all.cpp:2286
bool MortalityTest() override
Do a mortality test.
Definition: Vole_all.cpp:2556
Definition: Treatment.h:144
Definition: Treatment.h:52
Definition: Treatment.h:89
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
void UnsetGeneticFlag()
Definition: GeneticMaterial.cpp:175
Definition: Treatment.h:94
Definition: Treatment.h:142
int MinReproAgeF
Definition: Vole_all.cpp:141
int m_ElemBorn
Definition: vole_all.h:178
int SupplyTotNoYoung()
Definition: vole_all.h:345
AnimalPosition SupplyPosition() const
Returns the objects location and habitat type and veg type.
Definition: PopulationManager.cpp:1533
bool IsTrap(int p_x, int p_y) const
Definition: VolePopulationManager.h:201
Definition: VolePopulationManager.h:80
static CfgInt cfg_vole_LifeMonths("VOLE_LIFEMONTHS", CFG_CUSTOM, 3)
static double m_FHabQualThreshold2
Definition: vole_all.h:229
Definition: Treatment.h:83
static double m_MHabQualThreshold2
Definition: vole_all.h:235
TTypeOfVoleState CurrentVState
Definition: vole_all.h:406
virtual void Init(struct_Vole_Adult *a_AVoleStruct_ptr)
Definition: Vole_all.cpp:228
Definition: Treatment.h:99
int SupplyElemBorn()
Definition: vole_all.h:353
~Vole_Female() override
Definition: Vole_all.cpp:1711
TTypesOfVegetation SupplyVegType(int a_x, int a_y)
Returns the vegetation type of the polygon using the polygon reference number a_polyref or coordinate...
Definition: Landscape.h:1925
Definition: Treatment.h:76
Definition: vole_all.h:61
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
Definition: Treatment.h:35
unsigned int m_MinTerrRange
Definition: vole_all.h:156
Definition: Treatment.h:81
int SupplyMateId()
Definition: vole_all.h:586
CfgInt cfg_productapplicendyear
const int MinReproWeightF
Definition: Vole_all.cpp:145
const int FemNoMature
Definition: Vole_all.cpp:117
int SupplySimH() const
Returns landscape height in m.
Definition: PopulationManager.h:569
void AddToJuvs(const int juvs)
Definition: VolePopulationManager.h:250
Definition: Treatment.h:43
Definition: Treatment.h:91
void MoveTo(int p_Vector, int p_Distance, int iterations)
Movement.
Definition: Vole_all.cpp:883
static CfgFloat cfg_PesticideLitterSizeReduction("VOLE_PCIDE_LITTERSIZEREDUCTION", CFG_CUSTOM, 0.0038)
unsigned SupplyY()
Definition: vole_all.h:373
Definition: Treatment.h:127
Definition: Treatment.h:103
TTypeOfVoleState st_ReproBehaviour() const
Reproductive switch.
Definition: Vole_all.cpp:1794
const unsigned TheGestationPeriod
Definition: Vole_all.cpp:121
Definition: Treatment.h:121
void UnsetGeneticFlag()
Definition: vole_all.h:396
Definition: vole_all.h:66
Base class for voles - all vole objects are descended from this class.
Definition: vole_all.h:145
Definition: vole_all.h:98
int m_BirthYear
Definition: vole_all.h:149
void CheckTraps()
Definition: Vole_all.cpp:2590
int SupplyHowManyVoles(unsigned a_x, unsigned a_y, unsigned a_range) const
Definition: VolePopulationManager.cpp:890
int MoveQuality(int p_x, int p_y) const
Test a location for quality while moving.
Definition: Vole_all.cpp:1186
Definition: Treatment.h:44
~Vole_Male() override
Definition: Vole_all.cpp:2231
Definition: Treatment.h:56
static CfgInt cfg_MaxMaleTerrSize("VOLE_MAXMALETERRITORYSIZE", CFG_CUSTOM, 23)
Definition: Treatment.h:125
Vole_Female * FindClosestFemale(int p_x, int p_y, int p_steps) const
Definition: VolePopulationManager.cpp:1602
int m_VegBorn
Definition: vole_all.h:180
Definition: vole_all.h:64
static CfgFloat cfg_InfanticideRangeRelToTerRange("VOLE_IINFANTICIDERANGE_RELTO_TERRANGE", CFG_CUSTOM, 0.5)
TTypeOfVoleState st_Eval_n_Explore(void)
JuvenileMale vole main territory assessment behaviour.
Definition: Vole_all.cpp:2981
Definition: vole_all.h:63
GeneticMaterial m_MatesGenes
The DNA passed from the male on mating.
Definition: vole_all.h:624
bool m_Mature
Definition: vole_all.h:168
Definition: VolePopulationManager.h:64
double g_speedy_Divides[2001]
A generally useful array of fast divide calculators by multiplication.
Definition: Landscape.cpp:344
int m_guard_cell_y
The index y to the guard cell.
Definition: PopulationManager.h:374
TTypeOfVoleState Dispersal(double p_OldQual, int p_Distance)
JuvenileMale vole dispersal behaviour.
Definition: Vole_all.cpp:3007
int FatherStateAtBirth
Definition: vole_all.h:129
Definition: Treatment.h:61
Definition: Treatment.h:80
Definition: Treatment.h:60
InTrapPosition SupplyTrapPosition()
Definition: vole_all.h:377
bool SupplyInTrap()
Definition: vole_all.h:375
Definition: Treatment.h:90
const unsigned MinReproWeightM
Definition: Vole_all.cpp:143
Definition: VolePopulationManager.h:82
Definition: Treatment.h:123
Definition: vole_all.h:95
CfgBool l_pest_enable_pesticide_engine
Used to turn on or off the PPP functionality of ALMaSS.
void UnsetDirectFlag()
Definition: vole_all.h:398
const double VoleSoilCultivationMort
Definition: Vole_all.cpp:190
Definition: Treatment.h:131
void Setm_Mature()
Definition: vole_all.h:297
unsigned SupplyX()
Definition: vole_all.h:371
Definition: VolePopulationManager.h:76
int BackTranslateVegTypes(TTypesOfVegetation VegReference)
Returns the ALMaSS reference number translated from the TTypesOfVegetation type.
Definition: Landscape.h:2338
Definition: vole_all.h:97
void ChangeData(int a_data, int a_value)
Definition: VolePopulationManager.cpp:3302
Definition: Treatment.h:138
~Vole_JuvenileMale() override
Definition: Vole_all.cpp:3105
int HeterozygosityCount()
Definition: GeneticMaterial.cpp:325
Definition: Treatment.h:122
Definition: Treatment.h:39
static double m_MinJMVoleHabQual
Definition: vole_all.h:218
int st_Evaluate_n_Explore()
Main territory evaluation behaviour.
Definition: Vole_all.cpp:2042
Definition: Treatment.h:110
Definition: vole_all.h:69
Definition: Treatment.h:51
void Step() override
Juvenile Male vole Step.
Definition: Vole_all.cpp:2915
double value() const
Definition: Configurator.h:142
unsigned int uint32
Definition: ALMaSS_Setup.h:34
const int WeanedWeight
Definition: Vole_all.cpp:125
Definition: Treatment.h:143
void SetDirectFlag()
Definition: vole_all.h:394
static CfgFloat cfg_InfanticideProbability("INFANTI_PROBA", CFG_CUSTOM, 0.01)
FarmToDo
Definition: Treatment.h:31
const unsigned MaleMovement[4]
Definition: Vole_all.cpp:173
double g_extradispmort
Definition: Vole_all.cpp:198
bool GetFertile()
Get the male vole fertility.
Definition: vole_all.h:424
static CfgInt cfg_MaxFemaleTerrSize("VOLE_MAXFEMALETERRITORYSIZE", CFG_CUSTOM, 8)
A struct for passing data to create a new vole.
Definition: vole_all.h:115
Definition: Treatment.h:133
unsigned SupplyYoungAge()
Definition: vole_all.h:589
void SetWeight(double W)
Definition: vole_all.h:295
void Set_MateState(int MateState)
Definition: vole_all.h:592
void OnKilled() override
JuvenileMale vole death by external entity.
Definition: Vole_all.cpp:3073
unsigned m_x
Definition: PopulationManager.h:173
Definition: Treatment.h:104
const unsigned VoleMoveInterval
Definition: Vole_all.cpp:171
Definition: Treatment.h:85
GeneticMaterial m_MyGenes
Definition: vole_all.h:205
Definition: vole_all.h:60
TTypesOfLandscapeElement SupplyElemType()
Definition: vole_all.h:355
void Step() override
Male vole Step.
Definition: Vole_all.cpp:2246
unsigned m_YoungAge
The age of current litter in days.
Definition: vole_all.h:619
int SupplyIDNo()
Definition: vole_all.h:363
double g_NoFemalesMove
Definition: Vole_all.cpp:199
Definition: Treatment.h:140
static double m_FHabQualThreshold1
Definition: vole_all.h:231
Definition: Treatment.h:38
Definition: Treatment.h:117
Definition: Treatment.h:120
const double VoleHarvestMort
Definition: Vole_all.cpp:186
const int GrowStopDate
Definition: Vole_all.cpp:137
void Step() override
JuvenileFemale vole Step.
Definition: Vole_all.cpp:1279
void SendMessage(TTypeOfVoleMessage p_message, unsigned p_x, unsigned p_y, unsigned p_range, bool p_sex, double p_Weight)
Definition: VolePopulationManager.cpp:2711
bool CanFeed() const
Currently not used.
Definition: Vole_all.cpp:2583
Definition: Treatment.h:59
void UnsetDirectFlag()
Definition: GeneticMaterial.cpp:179
double weight
Definition: vole_all.h:120
Definition: VolePopulationManager.h:79
void FreeLocation() override
Map location function.
Definition: Vole_all.cpp:2861
Definition: Treatment.h:100
void Set_MotherId(unsigned MotherIdNo)
Definition: vole_all.h:302
The class for female voles.
Definition: vole_all.h:536
Definition: Treatment.h:150
CfgInt cfg_MinReproAgeF("VOLE_MINREPROAGEF", CFG_CUSTOM, 23)
Definition: vole_all.h:70
Definition: Treatment.h:124
Definition: vole_all.h:55
uint32 GetDirectFlag()
Definition: GeneticMaterial.cpp:188
void Set_XBorn(int a_Location_x)
Definition: vole_all.h:308
int m_PolyRefBorn
Definition: vole_all.h:176
virtual bool GetLocation(int, int)
Definition: vole_all.h:446
Definition: Treatment.h:139
bool m_Have_Territory
Definition: vole_all.h:196
Definition: Treatment.h:126
int WhatState() override
Definition: vole_all.h:331
double m_Weight
Definition: vole_all.h:184
void EndStep() override
All voles age at the end of the day.
Definition: Vole_all.cpp:323
int g_MaleReproductFinish
Definition: Vole_all.cpp:177
void SetFree()
Definition: vole_all.h:379
int SupplyMateSB()
Definition: vole_all.h:587
Definition: Treatment.h:149
void SetDirectFlag()
Definition: GeneticMaterial.cpp:170
Definition: Treatment.h:132
Definition: vole_all.h:57
Definition: Treatment.h:148
bool value() const
Definition: Configurator.h:164
void Set_YBorn(int a_Location_y)
Definition: vole_all.h:310
Definition: Treatment.h:67
static unsigned int m_MaxFemaleTerritorySize
Definition: vole_all.h:210
unsigned SupplyAge()
Definition: vole_all.h:369
void Set_VegBorn(int a_Location_x, int a_Location_y)
Definition: vole_all.h:319
Definition: LandscapeFarmingEnums.h:1065
int st_Evaluate_n_Explore()
Main territory evaluation behaviour.
Definition: Vole_all.cpp:1587
int m_NoOfYoung
The number of young in the current litter (if one).
Definition: vole_all.h:604
void AddToNoYoungInfanticideCount(const int m_NoOfYoung, const int m_YoungAge)
Definition: VolePopulationManager.h:251
Definition: Treatment.h:42
const double MaxWeightM
Definition: Vole_all.cpp:127
Definition: vole_all.h:99
The class for female voles.
Definition: vole_all.h:578
Definition: Treatment.h:65
int xborn
Definition: vole_all.h:121
int st_Maturation(void) const
Male vole maturation control.
Definition: Vole_all.cpp:2337
Definition: Treatment.h:45
Definition: Treatment.h:98
int Dispersal(double p_OldQual, int p_Distance)
Female dispersal.
Definition: Vole_all.cpp:1639
TTypeOfVoleState st_Lactating()
Lactation.
Definition: Vole_all.cpp:1903
const double InfanticideChanceByAge[9]
Definition: Vole_all.cpp:179
Definition: vole_all.h:68
Definition: LandscapeFarmingEnums.h:1060
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
VoleDispersalReturns
Definition: vole_all.h:74
int m_MatesIdNo
Definition: vole_all.h:599
virtual void FreeLocation()
Definition: vole_all.h:444
int m_SimH
Definition: vole_all.h:202
Definition: LandscapeFarmingEnums.h:1062
const double VoleStriglingMort
Definition: Vole_all.cpp:188
const double MaxWeightF
Definition: Vole_all.cpp:129
Definition: Treatment.h:77
Definition: Treatment.h:96
Class for the genetic material optionally carried by animals in ALMaSS.
Definition: GeneticMaterial.h:94
double CalculateCarryingCapacity(int x, int y, int a_ddep) const
Definition: Vole_all.cpp:369
static double m_MaleTerritoryRangeSlope
Definition: vole_all.h:222
void st_BecomeSubAdult(void)
Definition: Vole_all.cpp:1553
void Set_Age(int Age)
Definition: vole_all.h:328
Definition: Treatment.h:54
bool m_gflag
Definition: vole_all.h:135
void DetermineTerritorySize()
int m_XBorn
Definition: vole_all.h:172
int st_Special_Explore()
Post weaning territory expansion.
Definition: Vole_all.cpp:2083
void SetMapValue(unsigned x, unsigned y, a_type p)
Definition: MovementMap.h:143
Definition: Treatment.h:130
double misc_use
Definition: vole_all.h:137
Definition: Treatment.h:97
uint32 SupplyMyAllele(int i, int j)
Definition: vole_all.h:386
Definition: Treatment.h:141
double SupplyPesticide(int a_x, int a_y, PlantProtectionProducts a_ppp)
Gets total pesticide for a location.
Definition: Landscape.cpp:1386
a_type GetMapValue(unsigned x, unsigned y)
Definition: MovementMap.h:140
GeneticMaterial Genes
Definition: vole_all.h:133
void SetLocation() override
Location map function.
Definition: Vole_all.cpp:1612
CfgBool cfg_RecordVoleMort
int SupplyCountFemales(unsigned p_x, unsigned p_y, unsigned p_TerrRange) const
Definition: VolePopulationManager.cpp:2238
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
CfgFloat l_pest_daily_mort
Daily mortality of pesticide with type 1.
Definition: Treatment.h:70
Definition: Treatment.h:46
int SupplyYBorn()
Definition: vole_all.h:349
CfgInt cfg_MinReproAgeM("VOLE_MINREPROAGEM", CFG_CUSTOM, 30)
double g_DailyMortChance
Definition: Vole_all.cpp:183
void DoWalking(int p_Distance, int &p_Vector, int &vx, int &vy) const
Walking.
Definition: Vole_all.cpp:933
void BeginStep() override
Juvenile Male vole BeginStep.
Definition: Vole_all.cpp:2882
Definition: VolePopulationManager.h:85
Definition: vole_all.h:71
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
static CfgFloat cfg_vole_habqualscaler("VOLE_HABQUALSCALER", CFG_CUSTOM, 2.1)
const double VoleHerbicicideMort
Definition: Vole_all.cpp:194
Definition: vole_all.h:75
CfgBool cfg_AorOutput_used
Bool configurator entry class.
Definition: Configurator.h:155
void CheckManagement()
Used to start a check for any management related effects at the objects current location.
Definition: PopulationManager.cpp:1591
const unsigned WeanedAge
Definition: Vole_all.cpp:123
Class to handle statistics and constructs based on allele frequencies.
Definition: GeneticMaterial.h:61
static unsigned int m_MinMaleTerritorySize
Definition: vole_all.h:212
int FatherId
Definition: vole_all.h:127
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
GeneticMaterial SupplyGenes()
Definition: vole_all.h:400
Definition: Treatment.h:111
bool SupplyTerritorial()
Definition: vole_all.h:335
void EndStep() override
Female vole EndStep.
Definition: Vole_all.cpp:1327
CfgInt cfg_productapplicstartyear
void DoWalkingCorrect(int p_Distance, int &p_Vector, int &vx, int &vy) const
Walking where there is a danger of stepping off the world.
Definition: Vole_all.cpp:1022
const unsigned MinMaleMovement
Definition: Vole_all.cpp:175
Definition: Treatment.h:135
double SupplyWeight()
Definition: vole_all.h:361
Definition: vole_all.h:67
Definition: Treatment.h:82
CfgFloat cfg_VoleResourceRegrowth("VOLE_RESOURCEREGROWTH", CFG_CUSTOM, 0.5)
A parameter determining the density dependence effect. Zero will have no density dependence effect,...
Definition: vole_all.h:76
Vole_Population_Manager * VPM
Definition: vole_all.h:131
A class defining an animals position.
Definition: PopulationManager.h:169
Definition: Treatment.h:63
~Vole_JuvenileFemale() override
Definition: Vole_all.cpp:1231
int SupplyHeteroZyg()
Definition: vole_all.h:383
int m_DispVector
Definition: vole_all.h:194
Definition: VolePopulationManager.h:75
Definition: Treatment.h:62
Definition: Treatment.h:119
Definition: Treatment.h:72
uint32 GetGeneticFlag()
Definition: GeneticMaterial.cpp:184
void SetLocation() override
Map location function.
Definition: Vole_all.cpp:2854
Definition: Treatment.h:112
Definition: Treatment.h:137
Definition: Treatment.h:40
Definition: Treatment.h:53
int SupplyDeathCause()
Definition: vole_all.h:367
Definition: VolePopulationManager.h:78
int BirthYear
Definition: vole_all.h:126
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Treatment.h:145
int ReproTable[4][12]
Definition: VolePopulationManager.h:218
double SupplyRodenticide(int a_x, int a_y)
Gets total rodenticide for a location.
Definition: Landscape.cpp:586
Definition: VolePopulationManager.h:83
int GetGeneticFlag()
Definition: vole_all.h:388
static double m_MinFVoleHabQual
Definition: vole_all.h:216
Definition: Treatment.h:86
static double m_FHabQualThreshold3
Definition: vole_all.h:227
Definition: Treatment.h:49
int SupplyNoOfYoung()
Definition: vole_all.h:584
bool SupplyMature()
Definition: vole_all.h:365
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
double SupplyVegBiomass(int a_polyref)
Returns the biomass of the vegetation using the polygon reference number a_polyref or based on the x,...
Definition: Landscape.h:1542
Definition: Treatment.h:95
static double m_MHabQualThreshold3
Definition: vole_all.h:233
Definition: Treatment.h:147
int SupplyMotherId()
Definition: vole_all.h:339
Definition: Treatment.h:36
void ReInit(struct_Vole_Adult *p_aVoleStruct) override
Definition: Vole_all.cpp:1692
void ReInit(struct_Vole_Adult *p_aVoleStruct) override
Definition: Vole_all.cpp:3094
void st_Dying()
All voles end here on death.
Definition: Vole_all.cpp:335
bool m_StepDone
Indicates whether the iterative step code is done for this timestep.
Definition: PopulationManager.h:133
uint32 GetAllele(int pos, int Chromosome)
Definition: GeneticMaterial.cpp:230
TTypesOfPesticide SupplyPesticideType(void)
Gets type of pesticide effect from a reference.
Definition: Landscape.h:788
bool SupplyOlderFemales(unsigned p_x, unsigned p_y, unsigned p_Age, unsigned p_range) const
Definition: VolePopulationManager.cpp:744
static CfgInt cfg_VoleEndoCrineDisruptionGestationLength("VOLE_ENDOCRINEDISPRUPTORGESTATION", CFG_CUSTOM, 21)
Definition: Treatment.h:116
int m_SimW
Definition: vole_all.h:202
Definition: Treatment.h:93
unsigned IDNumber
Definition: VolePopulationManager.h:219
bool m_Sex
Definition: vole_all.h:166
void FreeLocation() override
Location map function.
Definition: Vole_all.cpp:1617
int SupplyYearNumber(void)
Passes a request on to the associated Calendar class function, returns m_simulationyear
Definition: Landscape.h:2287
Definition: Treatment.h:114
const double VoleInsecticideMort
Definition: Vole_all.cpp:196
void ClearMapValue(unsigned x, unsigned y)
Definition: MovementMap.h:146
int m_MateLive
Definition: vole_all.h:598
void OnKilled() override
Death from external entity.
Definition: Vole_all.cpp:1546
static unsigned int m_MaxMaleTerritorySize
Definition: vole_all.h:208
int value() const
Definition: Configurator.h:116
void Step() override
Female vole Step.
Definition: Vole_all.cpp:1726
bool m_Pregnant
A flag indicating whether pregnant or not.
Definition: vole_all.h:609
TTypesOfLandscapeElement m_EleType
Definition: PopulationManager.h:175
unsigned IDNo
Definition: vole_all.h:200
void st_Infanticide(void) const
Male vole infanticide behaviour.
Definition: Vole_all.cpp:2358
static CfgBool cfg_VoleMortalityDataUsed("VOLE_MORTALITY_DATA_USED", CFG_CUSTOM, false)
Definition: Treatment.h:75
int y
Definition: vole_all.h:118
GeneticMaterial.h This file contains the headers for the genetic material classes
Definition: Treatment.h:47
unsigned m_y
Definition: PopulationManager.h:174
static CfgFloat cfg_PesticideAccumulationThresholdModelink2("VOLE_PESTICDEACCUMULATIONTHRESHOLD_MODELINKTWO", CFG_CUSTOM, 20.0)
Definition: GeneticMaterial.h:192
TTypeOfVoleState st_BecomeReproductive()
Female vole maturation control.
Definition: Vole_all.cpp:1826
Definition: Treatment.h:106
Definition: Treatment.h:109
The class to handle all vole population related matters.
Definition: VolePopulationManager.h:148
static CfgInt cfg_MaxStarvationDays("VOLE_MAXSTARVATIONDAYS", CFG_CUSTOM, 29)
Definition: Treatment.h:48
Landscape * L
Definition: vole_all.h:130
std::string EventtypeToString(int a_event)
Returns the text representation of a treatment type.
Definition: Landscape.cpp:6024
TTypesOfPesticide
Values that represent types of pesticide actions.
Definition: LandscapeFarmingEnums.h:1057
void UpdateGuardMap(int a_x, int a_y, int &a_index_x, int &a_index_y)
Get the index of the guard map for the given location.
Definition: PopulationManager.h:768
void CreateObjects(VoleObject a_obType, TAnimal *a_pvo, struct_Vole_Adult *a_as, int a_number)
Definition: VolePopulationManager.cpp:2756
virtual void CopyMyself()
Used to copy the object details to another in descendent classes.
Definition: PopulationManager.h:273
CfgBool cfg_CfgRipleysOutputUsed
int SupplyTerrRange()
Definition: vole_all.h:359
Definition: Treatment.h:146
Definition: Treatment.h:134
Vole_Male(struct_Vole_Adult *p_aVoleStruct)
Vole_Male constructor.
Definition: Vole_all.cpp:2208
void DetermineTerritorySize()
Calculates the territory size needed for a vole of his weight.
Definition: Vole_all.cpp:2568
void Set_PolyRefBorn(int a_Location_x, int a_Location_y)
Definition: vole_all.h:326
const double growthperdayF
Definition: Vole_all.cpp:135
The class for juvenile male voles.
Definition: vole_all.h:458
Definition: Treatment.h:118
void st_BecomeSubAdult(void)
Definition: Vole_all.cpp:3035
Movement maps are used for rapid computing of animal movement. This version uses values of 0-15 only.
Definition: MovementMap.h:112
void Set_NoYoungTot(int a_NoOfYoung)
Definition: vole_all.h:306
Definition: vole_all.h:78
Definition: Treatment.h:113
Vole_JuvenileMale(struct_Vole_Adult *p_aVoleStruct)
Vole_JuvenileMale constructor.
Definition: Vole_all.cpp:3084
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
InTrapPosition m_intrappos
Definition: vole_all.h:206
Definition: VolePopulationManager.h:73
void Escape(int p_Vector, int p_Distance)
Dispersal - directed movement.
Definition: Vole_all.cpp:1109
void Set_BirthYear(int BirthYear)
Definition: vole_all.h:299
A class for storing the position of the trap the vole is in.
Definition: vole_all.h:106
Definition: LandscapeFarmingEnums.h:1061
Definition: Treatment.h:41
static CfgFloat cfg_volepcidebiodegredrate("VOLE_PCIDE_BIODEGREDATIONRATE", CFG_CUSTOM, 0.0)
bool GetLocation(int px, int py) override
Location map function.
Definition: Vole_all.cpp:1622
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
CfgBool cfg_ReallyBigOutputMonthly_used
Definition: Treatment.h:37
Vole_Population_Manager * m_OurPopulation
Definition: vole_all.h:407
int MotherId
Definition: vole_all.h:128
The class for male voles.
Definition: vole_all.h:499
Definition: Treatment.h:79
int m_guard_cell_x
The index x to the guard cell.
Definition: PopulationManager.h:370
VoleObject
Definition: vole_all.h:94
int m_Age
Definition: vole_all.h:170
double GetHabitatQuality(const int a_index) const
Definition: VolePopulationManager.h:230
void ReInit(struct_Vole_Adult *p_aVoleStruct) override
Definition: Vole_all.cpp:1216
Vole_JuvenileFemale(struct_Vole_Adult *p_aVoleStruct)
Vole_JuvenileFemale constructor.
Definition: Vole_all.cpp:1202
const double VolePigGrazingMort
Definition: Vole_all.cpp:192
const int September
Julian start dates of the month of September.
Definition: Landscape.h:54
virtual void ReInit(struct_Vole_Adult *a_AVoleStruct_ptr)
Definition: Vole_all.cpp:225
static double m_MinMVoleHabQual
Definition: vole_all.h:220
const double DaysAtMaxGrowth
Definition: Vole_all.cpp:131
void Set_FatherId(unsigned FatherIdNo)
Definition: vole_all.h:304
Definition: Treatment.h:74
int VegBorn
Definition: vole_all.h:125
Double configurator entry class.
Definition: Configurator.h:126
int SupplySimW() const
Returns landscape width in m.
Definition: PopulationManager.h:567
static CfgFloat cfg_PesticideWeaningReduction("VOLE_PCIDE_WEANINGREDUCTION", CFG_CUSTOM, 0.0017)
Definition: Treatment.h:136
const double FemaleResourceReq[13]
Definition: Vole_all.cpp:150
void ReInit(struct_Vole_Adult *p_aVoleStruct) override
Definition: Vole_all.cpp:2219
int SupplyInOlderTerr(unsigned p_x, unsigned p_y, unsigned p_Age, unsigned p_Range) const
Definition: VolePopulationManager.cpp:2370
Vole_Female(struct_Vole_Adult *p_aVoleStruct)
Vole_Female constructor.
Definition: Vole_all.cpp:1675
int m_YBorn
Definition: vole_all.h:174
void EndStep() override
Juvenile Male vole EndStep.
Definition: Vole_all.cpp:2955
Definition: LandscapeFarmingEnums.h:1063
Definition: Treatment.h:108
void Step() override
Step behaviour - must be implemented in descendent classes.
Definition: vole_all.h:287
int g_sigAgeDiff
Definition: Vole_all.cpp:147
Definition: Treatment.h:69
Definition: Treatment.h:105
int SupplyHomoZyg()
Definition: vole_all.h:381
void SetGeneticFlag()
Definition: GeneticMaterial.cpp:166
VoleSummaryOutput * m_VoleRecordMort
Definition: VolePopulationManager.h:193
bool GetLocation(int px, int py) override
Map location function.
Definition: Vole_all.cpp:2868
bool m_BornLastYear
A flag set if the female was born the year before.
Definition: vole_all.h:154
double MoveToLessFavourable
Definition: VolePopulationManager.cpp:155
virtual void OnKilled()
Definition: vole_all.h:402
TTypeOfVoleState st_UpdateGestation()
Gestation control.
Definition: Vole_all.cpp:1811
const int August
Julian start dates of the month of August.
Definition: Landscape.h:52
int m_DaysUntilBirth
A counter counting down gestation days.
Definition: vole_all.h:614
Definition: VolePopulationManager.h:74
bool m_inAtrap
Definition: vole_all.h:108
Definition: Treatment.h:78
TTypeOfVoleState st_Mating()
Female mating.
Definition: Vole_all.cpp:2137
static double m_FemaleTerritoryRangeSlope
Definition: vole_all.h:224
int m_Death
Definition: vole_all.h:162
Definition: Configurator.h:70
TTypeOfVoleState st_GiveBirth()
Litter production.
Definition: Vole_all.cpp:1840
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
Definition: Treatment.h:66
Definition: Treatment.h:92
bool SupplySex()
Definition: vole_all.h:341
int HomozygosityCount()
Definition: GeneticMaterial.cpp:314
Definition: Treatment.h:102
bool m_flag
Definition: vole_all.h:134
Definition: vole_all.h:65
static double m_MHabQualThreshold1
Definition: vole_all.h:237
const unsigned MinFemaleMovement
Definition: Vole_all.cpp:170
void SetGeneticFlag()
Definition: vole_all.h:392
unsigned m_FatherId
Definition: vole_all.h:160
static CfgFloat cfg_PesticideAccumulationThreshold2("VOLE_PESTICDEACCUMULATIONTHRESHOLDTWO", CFG_CUSTOM, 44.1)
int x
Definition: vole_all.h:117
static CfgFloat cfg_PesticideFemaleMaturityDelay("VOLE_PCIDE_FEMALEMATURITYDELAY", CFG_CUSTOM, 0.0192)
int yborn
Definition: vole_all.h:122
bool SupplyBornLastYear()
Were we born this year?
Definition: vole_all.h:333
Definition: Treatment.h:57
Definition: Treatment.h:87
int m_CurrentStateNo
The basic state number for all objects - '-1' indicates death.
Definition: PopulationManager.h:131
Definition: Treatment.h:101
CfgBool cfg_pest_residue_or_rate
Use residue or rate. Used in Vole code.
int SupplyGrowthStartDate() const
Definition: VolePopulationManager.h:233
Definition: Treatment.h:73
Definition: vole_all.h:58
int vole_tole_move_quality(Landscape *m_TheLandscape, int x, int y)
Definition: Vole_toletoc.cpp:5
Definition: VolePopulationManager.h:72
void OnInfanticideAttempt()
Determines whether an infanticide attempt will succeed.
Definition: Vole_all.cpp:2176
int SupplyPolyRefIndex(int a_x, int a_y)
Get the index to the m_elems array for a polygon at location x,y.
Definition: Landscape.h:2172
Vole_Base(struct_Vole_Adult *a_AVoleStruct_ptr)
Constructor for Vole_Base.
Definition: Vole_all.cpp:222
double g_DailyMortChanceMaleTerr
Definition: Vole_all.cpp:184
CfgInt cfg_MinMaleTerritorySize("VOLE_MINMALETERRITORYSIZE", CFG_CUSTOM, 9)
int GetDirectFlag()
Definition: vole_all.h:390
Definition: Treatment.h:55
Definition: vole_all.h:77
CfgBool cfg_ResistanceDominant("VOLE_RESISTANCEDOMINANT", CFG_CUSTOM, false)
Vole_Male * FindClosestMale(int p_x, int p_y, int p_steps) const
Definition: VolePopulationManager.cpp:2026
Definition: VolePopulationManager.h:81
void AddToYoung(const int young)
Definition: VolePopulationManager.h:245
void Warn(std::string a_msg1, std::string a_msg2)
Wrapper for the g_msg Warn function.
Definition: Landscape.h:2250
int ElemBorn
Definition: vole_all.h:124
int age
Definition: vole_all.h:119
static unsigned int m_MinFemaleTerritorySize
Definition: vole_all.h:214
TTypeOfVoleState st_Eval_n_Explore(void)
Male vole main territory assessment behaviour.
Definition: Vole_all.cpp:2375
int SupplyAllele(int locus, int allele)
Definition: vole_all.h:385
Definition: GeneticMaterial.h:125
Definition: Treatment.h:34
virtual bool MortalityTest()
Do a mortality test.
Definition: Vole_all.cpp:349
bool m_fertile
Flag indicating the fertility state (true means fertile)
Definition: vole_all.h:186
int m_TerrRange
Definition: vole_all.h:164
int m_Reserves
Definition: vole_all.h:198
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
CfgInt cfg_MinFemaleTerritorySize("VOLE_MINFEMALETERRITORYSIZE", CFG_CUSTOM, 8)
bool SupplyPregnant()
Definition: vole_all.h:590
void SetFertile(bool f)
Set the male vole fertility.
Definition: vole_all.h:413
int SupplyPolyRef(int a_x, int a_y)
Get the in map polygon reference number from the x, y location.
Definition: Landscape.h:2157
VoleDispersalReturns Dispersal(double p_OldQual, int p_Distance)
Male vole dispersal behaviour.
Definition: Vole_all.cpp:2482
Definition: Treatment.h:58
static bool m_BreedingSeason
Definition: vole_all.h:239
bool m_dflag
Definition: vole_all.h:136
Definition: VolePopulationManager.h:77
~Vole_Base() override
Definition: Vole_all.cpp:291
Definition: Treatment.h:128
int SupplyBirthYear()
Definition: vole_all.h:343
bool OnFarmEvent(FarmToDo event) override
External event handler.
Definition: Vole_all.cpp:1377
Definition: LandscapeFarmingEnums.h:1064
Definition: LandscapeFarmingEnums.h:1079
bool OnFarmEvent(FarmToDo event) override
JuvenileMale vole exernal event handler.
Definition: Vole_all.cpp:2687
CfgFloat cfg_extradispmort("VOLEDISPMORT", CFG_CUSTOM, 0.055)
Input parameter for daily extra mortality chance while dispersing.
Definition: LandscapeFarmingEnums.h:1059
int SupplyVegBorn()
Definition: vole_all.h:357
CfgInt cfg_VoleDDepConst("VOLE_DDEPCONST", CFG_CUSTOM, 4)
void BeginStep() override
BeingStep behaviour - must be implemented in descendent classes.
Definition: vole_all.h:284
void SetBreedingSeason(bool a_flag)
Set Breeding Season flag.
Definition: vole_all.h:293
Definition: Treatment.h:50
virtual void SetLocation()
Definition: vole_all.h:441
Definition: vole_all.h:59
Definition: GeneticMaterial.h:153
int SupplyFatherId()
Definition: vole_all.h:337
Definition: Treatment.h:115
int SupplyPolyRefBorn()
Definition: vole_all.h:351
void st_JuvenileExplore(void)
Extra movement on weaning.
Definition: Vole_all.cpp:2673
Definition: Treatment.h:33
IDMap< TAnimal * > * m_VoleMap
Definition: VolePopulationManager.h:276
int BackTranslateEleTypes(TTypesOfLandscapeElement EleReference)
Returns the ALMaSS reference number translated from the TTypesOfLandscapeElement type.
Definition: Landscape.h:2332
int m_NoOfYoungTotal
Definition: vole_all.h:192
void BeginStep() override
Female vole BeginStep.
Definition: Vole_all.cpp:1246
const unsigned FemaleMovement
Definition: Vole_all.cpp:168
Definition: Treatment.h:107
TTypesOfVegetation m_VegType
Definition: PopulationManager.h:176
int PolyRefBorn
Definition: vole_all.h:123