Loading [MathJax]/extensions/ams.js
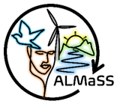 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Base class for voles - all vole objects are descended from this class.
More...
#include <vole_all.h>
|
| Vole_Base (struct_Vole_Adult *a_AVoleStruct_ptr) |
| Constructor for Vole_Base. More...
|
|
| ~Vole_Base () override |
|
virtual void | Init (struct_Vole_Adult *a_AVoleStruct_ptr) |
|
virtual void | ReInit (struct_Vole_Adult *a_AVoleStruct_ptr) |
|
void | BeginStep () override |
| BeingStep behaviour - must be implemented in descendent classes. More...
|
|
void | Step () override |
| Step behaviour - must be implemented in descendent classes. More...
|
|
void | EndStep () override |
| All voles age at the end of the day. More...
|
|
void | st_Dying () |
| All voles end here on death. More...
|
|
void | SetBreedingSeason (bool a_flag) |
| Set Breeding Season flag. More...
|
|
void | SetWeight (double W) |
|
void | Setm_Mature () |
|
void | Set_BirthYear (int BirthYear) |
|
void | Set_MotherId (unsigned MotherIdNo) |
|
void | Set_FatherId (unsigned FatherIdNo) |
|
void | Set_NoYoungTot (int a_NoOfYoung) |
|
void | Set_XBorn (int a_Location_x) |
|
void | Set_YBorn (int a_Location_y) |
|
void | Set_ElemBorn (int a_Location_x, int a_Location_y) |
|
void | Set_VegBorn (int a_Location_x, int a_Location_y) |
|
void | Set_PolyRefBorn (int a_Location_x, int a_Location_y) |
|
void | Set_Age (int Age) |
|
int | WhatState () override |
|
bool | SupplyBornLastYear () |
| Were we born this year? More...
|
|
bool | SupplyTerritorial () |
|
int | SupplyFatherId () |
|
int | SupplyMotherId () |
|
bool | SupplySex () |
|
int | SupplyBirthYear () |
|
int | SupplyTotNoYoung () |
|
int | SupplyXBorn () |
|
int | SupplyYBorn () |
|
int | SupplyPolyRefBorn () |
|
int | SupplyElemBorn () |
|
TTypesOfLandscapeElement | SupplyElemType () |
|
int | SupplyVegBorn () |
|
int | SupplyTerrRange () |
|
double | SupplyWeight () |
|
int | SupplyIDNo () |
|
bool | SupplyMature () |
|
int | SupplyDeathCause () |
|
unsigned | SupplyAge () |
|
unsigned | SupplyX () |
|
unsigned | SupplyY () |
|
bool | SupplyInTrap () |
|
InTrapPosition | SupplyTrapPosition () |
|
void | SetFree () |
|
int | SupplyHomoZyg () |
|
int | SupplyHeteroZyg () |
|
int | SupplyAllele (int locus, int allele) |
|
uint32 | SupplyMyAllele (int i, int j) |
|
int | GetGeneticFlag () |
|
int | GetDirectFlag () |
|
void | SetGeneticFlag () |
|
void | SetDirectFlag () |
|
void | UnsetGeneticFlag () |
|
void | UnsetDirectFlag () |
|
GeneticMaterial | SupplyGenes () |
|
virtual void | OnKilled () |
|
virtual bool | MortalityTest () |
| Do a mortality test. More...
|
|
void | CopyMyself (VoleObject a_vole) |
| Duplicates a vole. More...
|
|
void | SetFertile (bool f) |
| Set the male vole fertility. More...
|
|
bool | GetFertile () |
| Get the male vole fertility. More...
|
|
| TAnimal (int x, int y, Landscape *L) |
| The TAnimal constructor saving the x,y, location and the landscape pointer. More...
|
|
| TAnimal (int x, int y) |
| The TAnimal constructor saving the x,y used if landscape is already set. More...
|
|
void | SetGuardMapIndex (int a_index_x, int a_index_y) |
| Set the guard map index, this is used to avoid two animals operating in the same location when using multithread. More...
|
|
unsigned | SupplyFarmOwnerRef () const |
| Get the current location farm ref if any. More...
|
|
AnimalPosition | SupplyPosition () const |
| Returns the objects location and habitat type and veg type. More...
|
|
APoint | SupplyPoint () const |
| Returns the objects location in ALMaSS coordinates. More...
|
|
int | SupplyPolygonRef () const |
| Returns the polygon reference where the object is located. More...
|
|
TTypesOfLandscapeElement | SupplyPolygonType () const |
| Returns the polygon type where the object is located. More...
|
|
int | Supply_m_Location_x () const |
| Returns the ALMaSS x-coordinate. More...
|
|
int | Supply_m_Location_y () const |
| Returns the ALMaSS y-coordinate. More...
|
|
int | SupplyGuardCellX () const |
| Returns the x-index to the guard cell. More...
|
|
int | SupplyGuardCellY () const |
| Returns the y-index to the guard cell. More...
|
|
int | SupplyAge () const |
| Returns the animals age in days. More...
|
|
void | SetAge (int a_age) |
| Sets the animals age in days. More...
|
|
virtual void | KillThis () |
| Sets all parameters ready for object destruction. More...
|
|
virtual void | CopyMyself () |
| Used to copy the object details to another in descendent classes. More...
|
|
void | SetX (const int a_x) |
| Sets the x-coordinate. More...
|
|
void | SetY (const int a_y) |
| Sets the y-coordinate. More...
|
|
virtual void | ReinitialiseObject (int a_x, int a_y, Landscape *a_l_ptr) |
|
virtual void | ReinitialiseObject (int a_x, int a_y) |
| Used to re-use an object - must be implemented in descendent classes. More...
|
|
virtual void | Dying () |
| A wrapped for KillThis - ideally should not be used. More...
|
|
void | CheckManagement () |
| Used to start a check for any management related effects at the objects current location. More...
|
|
void | CheckManagementXY (int a_x, int a_y) |
| Used to start a check for any management related effects at x,y. More...
|
|
virtual bool | OnFarmEvent (FarmToDo) |
| Must be reimplemented if used in descendent classes. Sets the action on a management event. More...
|
|
int | GetCurrentStateNo () const |
| Returns the current state number. More...
|
|
void | SetCurrentStateNo (int a_num) |
| Sets the current state number. More...
|
|
bool | GetStepDone () const |
| Returns the step done indicator flag. More...
|
|
void | SetStepDone (bool a_bool) |
| Sets the step done indicator flag. More...
|
|
void | ReinitialiseObjectBase () |
| Used to initialise an object. More...
|
|
| TALMaSSObject () |
| The constructor for TALMaSSObject. More...
|
|
virtual | ~TALMaSSObject ()=default |
| The destructor for TALMaSSObject. More...
|
|
|
virtual void | RodenticideIngestion (void) |
|
double | CalculateCarryingCapacity (int x, int y, int a_ddep) const |
|
int | MoveQuality (int p_x, int p_y) const |
| Test a location for quality while moving. More...
|
|
void | MoveTo (int p_Vector, int p_Distance, int iterations) |
| Movement. More...
|
|
void | DoWalking (int p_Distance, int &p_Vector, int &vx, int &vy) const |
| Walking. More...
|
|
void | DoWalkingCorrect (int p_Distance, int &p_Vector, int &vx, int &vy) const |
| Walking where there is a danger of stepping off the world. More...
|
|
void | Escape (int p_Vector, int p_Distance) |
| Dispersal - directed movement. More...
|
|
void | CheckTraps () |
|
virtual void | SetLocation () |
|
virtual void | FreeLocation () |
|
virtual bool | GetLocation (int, int) |
|
void | CorrectWrapRound () |
| Corrects wrap around co-ordinate problems. More...
|
|
Base class for voles - all vole objects are descended from this class.
◆ Vole_Base()
◆ ~Vole_Base()
Vole_Base::~Vole_Base |
( |
| ) |
|
|
override |
◆ BeginStep()
void Vole_Base::BeginStep |
( |
void |
| ) |
|
|
inlineoverridevirtual |
◆ CalculateCarryingCapacity()
double Vole_Base::CalculateCarryingCapacity |
( |
int |
p_x, |
|
|
int |
p_y, |
|
|
int |
a_ddep |
|
) |
| const |
|
protected |
Returns the sum of the qualities in the area covered by the territory (even if the vole does not have one)
Uses the algorithm for fast searching of square arrays with wrap around co-ordinates.
parameters = x,y starting coordinates top left
range = extent of the space to search
bottom left coordinate is therefore x+range, y+range
22/09/2000
For each polygon it gets the quality and multiplies by area of that polygon in the territory. This is summed for all polygons in the territoryto get overall quality. The value is then divided by the number of voles present less a threshold value.
371 int PolyRefData[500][2];
382 const int xextent0 = x + range_x;
383 const int yextent0 = y + range_y;
384 const int xextent1 = x + range_x -
m_SimW;
385 const int yextent1 = y + range_y -
m_SimH;
387 int Dfinx = xextent0;
388 int Dfiny = yextent0;
394 if (x + range_x <=
m_SimW)
429 for (
int i = 0; i < Afinx; i++)
431 for (
int j = y; j < Afiny; j++)
438 for (
int k = 0; k < NoPolygons; k++)
440 if (PolyRefData[k][0] == PRef)
451 PolyRefData[NoPolygons][0] = PRef;
452 PolyRefData[NoPolygons][1] = 1;
458 for (
int i = x; i < Bfinx; i++)
460 for (
int j = 0; j < yextent1; j++)
483 for (
int k = 0; k < NoPolygons; k++)
485 if (PolyRefData[k][0] == PRef)
496 PolyRefData[NoPolygons][0] = PRef;
497 PolyRefData[NoPolygons][1] = 1;
503 for (
int i = 0; i < Cfinx; i++)
505 for (
int j = 0; j < yextent1; j++)
528 for (
int k = 0; k < NoPolygons; k++)
530 if (PolyRefData[k][0] == PRef)
541 PolyRefData[NoPolygons][0] = PRef;
542 PolyRefData[NoPolygons][1] = 1;
550 for (
int i = x; i < Dfinx; i++)
552 for (
int j = y; j < Dfiny; j++)
557 if (last == PRef) { PolyRefData[k_index][1]++; }
561 for (
int k = 0; k < NoPolygons; k++)
563 if (PolyRefData[k][0] == PRef)
575 PolyRefData[NoPolygons][0] = PRef;
576 PolyRefData[NoPolygons][1] = 1;
588 if (Voles > a_ddep) quality /= Voles - a_ddep;
References Vole_Population_Manager::GetHabitatQuality(), TAnimal::m_Location_x, TAnimal::m_Location_y, m_MinTerrRange, TAnimal::m_OurLandscape, m_OurPopulation, m_SimH, m_SimW, m_TerrRange, Vole_Population_Manager::SupplyHowManyVoles(), and Landscape::SupplyPolyRefIndex().
Referenced by Vole_Male::CanFeed(), Vole_JuvenileMale::Dispersal(), Vole_Male::Dispersal(), Vole_JuvenileFemale::Dispersal(), Vole_JuvenileMale::st_Eval_n_Explore(), Vole_Male::st_Eval_n_Explore(), Vole_JuvenileFemale::st_Evaluate_n_Explore(), Vole_Female::st_Evaluate_n_Explore(), and Vole_Female::st_Special_Explore().
◆ CheckTraps()
void Vole_Base::CheckTraps |
( |
| ) |
|
|
protected |
2595 for (
int rad = 0; rad < r; rad++)
2598 for (
int x = vx - rad; x <= vx + rad; x++)
2627 for (
int y = vy - (rad - 1); y <= vy + (rad - 1); y++)
References g_random_fnc(), Vole_Population_Manager::IsTrap(), AnimalPosition::m_EleType, InTrapPosition::m_inAtrap, m_intrappos, TAnimal::m_Location_x, TAnimal::m_Location_y, TAnimal::m_OurLandscape, m_OurPopulation, m_TerrRange, AnimalPosition::m_VegType, AnimalPosition::m_x, AnimalPosition::m_y, Landscape::SupplyElementType(), Landscape::SupplyVegType(), and Landscape::SupplyYearNumber().
Referenced by Vole_JuvenileMale::st_Eval_n_Explore(), Vole_Male::st_Eval_n_Explore(), Vole_JuvenileFemale::st_Evaluate_n_Explore(), and Vole_Female::st_Evaluate_n_Explore().
◆ CopyMyself()
Duplicates a vole.
Method used to duplicate a vole - most commonly used for experimental manipulation of populations e.g. return rate experiments
References Vole_Population_Manager::CreateObjects(), TAnimal::m_Location_x, TAnimal::m_Location_y, m_MyGenes, TAnimal::m_OurLandscape, m_OurPopulation, Landscape::SupplyElementType(), Landscape::SupplyPolyRef(), Population_Manager_Base::SupplySimH(), Population_Manager_Base::SupplySimW(), Landscape::SupplyVegType(), and struct_Vole_Adult::VPM.
Referenced by Vole_Population_Manager::Catastrophe().
◆ DoWalking()
void Vole_Base::DoWalking |
( |
int |
p_Distance, |
|
|
int & |
p_Vector, |
|
|
int & |
vx, |
|
|
int & |
vy |
|
) |
| const |
|
protected |
Walking.
This method does the actual stepping - there is no look ahead here, so steps are taken one at a time based on the habitat type and vector given.
937 t[1] = p_Vector + 1 & 0x07;
938 t[2] = p_Vector + 7 & 0x07;
939 t[3] = p_Vector + 2 & 0x07;
940 t[4] = p_Vector + 6 & 0x07;
942 for (
int i = 0; i < p_Distance; i++)
956 q[0] =
MoveQuality(vx + g_vector_x[t[0]], vy + g_vector_y[t[0]]);
957 q[1] =
MoveQuality(vx + g_vector_x[t[1]], vy + g_vector_y[t[1]]);
958 q[2] =
MoveQuality(vx + g_vector_x[t[2]], vy + g_vector_y[t[2]]);
959 q[3] =
MoveQuality(vx + g_vector_x[t[3]], vy + g_vector_y[t[3]]);
960 q[4] =
MoveQuality(vx + g_vector_x[t[4]], vy + g_vector_y[t[4]]);
964 for (
int j = 1; j < 5; j++) q[j] = -1;
969 for (
int ii = 1; ii < 5; ii++)
976 else if (q[ii] == best) score++;
986 t[1] = p_Vector + 1 & 0x07;
987 t[2] = p_Vector + 7 & 0x07;
988 t[3] = p_Vector + 2 & 0x07;
989 t[4] = p_Vector + 6 & 0x07;
997 for (
int ii = 0; ii < 5; ii++)
999 if (best == q[ii]) loop++;
1007 vx += g_vector_x[t[loop]];
1008 vy += g_vector_y[t[loop]];
References g_rand_uni_fnc(), g_random_fnc(), MoveQuality(), and MoveToLessFavourable.
Referenced by MoveTo().
◆ DoWalkingCorrect()
void Vole_Base::DoWalkingCorrect |
( |
int |
p_Distance, |
|
|
int & |
p_Vector, |
|
|
int & |
vx, |
|
|
int & |
vy |
|
) |
| const |
|
protected |
Walking where there is a danger of stepping off the world.
This method does the actual stepping - there is no look ahead here, so steps are taken one at a time based on the habitat type and vector given.
This version corrects coords for wrap around. This is slower so is only called when necessary.
1026 t[1] = p_Vector + 1 & 0x07;
1027 t[2] = p_Vector + 7 & 0x07;
1028 t[3] = p_Vector + 2 & 0x07;
1029 t[4] = p_Vector + 6 & 0x07;
1031 for (
int i = 0; i < p_Distance; i++)
1051 for (
int j = 1; j < 5; j++) q[j] = -1;
1056 for (
int ii = 1; ii < 5; ii++)
1064 if (q[ii] == best) score++;
1074 t[1] = p_Vector + 1 & 0x07;
1075 t[2] = p_Vector + 7 & 0x07;
1076 t[3] = p_Vector + 2 & 0x07;
1077 t[4] = p_Vector + 6 & 0x07;
1084 for (
int ii = 0; ii < 5; ii++)
1086 if (best == q[ii]) loop++;
1094 vx += g_vector_x[t[loop]];
1095 vy += g_vector_y[t[loop]];
References g_rand_uni_fnc(), g_random_fnc(), m_SimH, m_SimW, MoveQuality(), and MoveToLessFavourable.
Referenced by MoveTo().
◆ EndStep()
void Vole_Base::EndStep |
( |
void |
| ) |
|
|
overridevirtual |
◆ Escape()
void Vole_Base::Escape |
( |
int |
p_Vector, |
|
|
int |
p_Distance |
|
) |
| |
|
protected |
Dispersal - directed movement.
This works like MoveTo above, but with a rather more directed movement aimed at moving further from the start point.
1118 for (
int i = 0; i < p_Distance; i++)
1128 t[1] = p_Vector + 1 & 0x07;
1129 t[2] = p_Vector + 7 & 0x07;
1135 for (
int ii = 0; ii < 3; ii++) {
if (q[ii] == -1) { noscore++; } }
1154 if (q[1] != -1) loop = 1;
1159 if (q[2] != -1) loop = 2;
1164 vx += g_vector_x[t[loop]];
1165 vy += g_vector_y[t[loop]];
References FreeLocation(), g_random_fnc(), TAnimal::m_guard_cell_x, TAnimal::m_guard_cell_y, TAnimal::m_Location_x, TAnimal::m_Location_y, m_OurPopulation, m_SimH, m_SimW, MoveQuality(), SetLocation(), and Population_Manager::UpdateGuardMap().
◆ FreeLocation()
virtual void Vole_Base::FreeLocation |
( |
| ) |
|
|
inlineprotectedvirtual |
◆ GetDirectFlag()
int Vole_Base::GetDirectFlag |
( |
| ) |
|
|
inline |
◆ GetFertile()
bool Vole_Base::GetFertile |
( |
| ) |
|
|
inline |
Get the male vole fertility.
Primarily used in ecotoxicological simulations where toxic effects may render the vole sterile.
References m_fertile.
Referenced by Vole_Female::st_Mating().
◆ GetGeneticFlag()
int Vole_Base::GetGeneticFlag |
( |
| ) |
|
|
inline |
◆ GetLocation()
virtual bool Vole_Base::GetLocation |
( |
int |
, |
|
|
int |
|
|
) |
| |
|
inlineprotectedvirtual |
◆ Init()
253 const unsigned FatherIdNo = a_AVoleStruct_ptr->
FatherId;
255 const unsigned MotherIdNo = a_AVoleStruct_ptr->
MotherId;
275 #ifdef __VoleStarvationDays
276 m_StarvationDays = 0;
279 #ifdef __VOLEPESTICIDEON
282 SetPesticideInfluenced1(
false);
283 SetPesticideInfluenced2(
false);
284 m_pesticideInfluenced3 = 0.0;
References struct_Vole_Adult::BirthYear, cfg_MaxFemaleTerrSize, cfg_MaxMaleTerrSize, cfg_MaxStarvationDays, cfg_MinFemaleTerritorySize, cfg_MinMaleTerritorySize, cfg_vole_habqualscaler, cfg_vole_LifeMonths, cfg_volepcidebiodegredrate, CurrentVState, struct_Vole_Adult::FatherId, g_rand_uni_fnc(), struct_Vole_Adult::Genes, IDNo, Vole_Population_Manager::IDNumber, struct_Vole_Adult::L, m_Age, m_BornLastYear, TALMaSSObject::m_CurrentStateNo, m_DispVector, m_FemaleTerritoryRangeSlope, m_fertile, m_Have_Territory, InTrapPosition::m_inAtrap, m_intrappos, m_LifeSpan, TAnimal::m_Location_x, TAnimal::m_Location_y, m_MaleTerritoryRangeSlope, m_Mature, m_MaxFemaleTerritorySize, m_MaxMaleTerritorySize, m_MinFemaleTerritorySize, m_MinFVoleHabQual, m_MinJMVoleHabQual, m_MinMaleTerritorySize, m_MinMVoleHabQual, m_MinTerrRange, m_MyGenes, m_NoOfYoungTotal, TAnimal::m_OurLandscape, m_OurPopulation, m_Reserves, m_SimH, m_SimW, m_TerrRange, m_Weight, MaxWeightF, MaxWeightM, MinReproWeightF, MinReproWeightM, struct_Vole_Adult::MotherId, Set_BirthYear(), Set_ElemBorn(), Set_FatherId(), Set_MotherId(), Set_PolyRefBorn(), Set_VegBorn(), Set_XBorn(), Set_YBorn(), Population_Manager_Base::SupplySimH(), Population_Manager_Base::SupplySimW(), tovs_InitialState, CfgInt::value(), CfgFloat::value(), struct_Vole_Adult::VPM, struct_Vole_Adult::x, and struct_Vole_Adult::y.
Referenced by ReInit(), Vole_JuvenileMale::ReInit(), Vole_Male::ReInit(), Vole_JuvenileFemale::ReInit(), Vole_Female::ReInit(), and Vole_Base().
◆ MortalityTest()
bool Vole_Base::MortalityTest |
( |
| ) |
|
|
virtual |
◆ MoveQuality()
int Vole_Base::MoveQuality |
( |
int |
p_x, |
|
|
int |
p_y |
|
) |
| const |
|
protected |
◆ MoveTo()
void Vole_Base::MoveTo |
( |
int |
p_Vector, |
|
|
int |
p_Distance, |
|
|
int |
p_iterations |
|
) |
| |
|
protected |
Movement.
Do an assessment of the quality of the voles territory, and move. This function does the same as the other CalcuateCarryingCapacity except that it alters the two values stand_x, stand_y to set them to be in the best polygon in the territory
Returns the mean of the qualities in the area covered by the territory (even if the vole does not have one)
Uses the algorithm for fast searching of square arrays with wrap around co-ordinates.
parameters = x,y starting coordinates top left
range = extent of the space to search
bottom left coordinate is therefore x+range, y+range
22/09/2000
For each polygon it gets the quality and multiplies by area of that polygon in the territory. This is summed for all polygons in the territory to get overall quality. This will alter m_Location_x & m_Location_y.
It will give a rather directed movement towards p_Vector.
Generally the vole will stay in the best habitat, but occasional mis-steps occur.
p_Vector gives the preferred direction (0-7), p_Distance is the number of steps.
884 const int offset = p_Distance * p_iterations;
References DoWalking(), DoWalkingCorrect(), FreeLocation(), GetLocation(), TAnimal::m_guard_cell_x, TAnimal::m_guard_cell_y, TAnimal::m_Location_x, TAnimal::m_Location_y, m_OurPopulation, m_SimH, m_SimW, SetLocation(), and Population_Manager::UpdateGuardMap().
Referenced by Vole_JuvenileMale::Dispersal(), Vole_Male::Dispersal(), Vole_JuvenileFemale::Dispersal(), Vole_JuvenileMale::st_JuvenileExplore(), and Vole_Female::st_Special_Explore().
◆ OnKilled()
virtual void Vole_Base::OnKilled |
( |
| ) |
|
|
inlinevirtual |
◆ ReInit()
◆ RodenticideIngestion()
void Vole_Base::RodenticideIngestion |
( |
void |
| ) |
|
|
protectedvirtual |
◆ Set_Age()
void Vole_Base::Set_Age |
( |
int |
Age | ) |
|
|
inline |
Set our age
References m_Age.
◆ Set_BirthYear()
void Vole_Base::Set_BirthYear |
( |
int |
BirthYear | ) |
|
|
inline |
◆ Set_ElemBorn()
void Vole_Base::Set_ElemBorn |
( |
int |
a_Location_x, |
|
|
int |
a_Location_y |
|
) |
| |
|
inline |
◆ Set_FatherId()
void Vole_Base::Set_FatherId |
( |
unsigned |
FatherIdNo | ) |
|
|
inline |
◆ Set_MotherId()
void Vole_Base::Set_MotherId |
( |
unsigned |
MotherIdNo | ) |
|
|
inline |
◆ Set_NoYoungTot()
void Vole_Base::Set_NoYoungTot |
( |
int |
a_NoOfYoung | ) |
|
|
inline |
◆ Set_PolyRefBorn()
void Vole_Base::Set_PolyRefBorn |
( |
int |
a_Location_x, |
|
|
int |
a_Location_y |
|
) |
| |
|
inline |
◆ Set_VegBorn()
void Vole_Base::Set_VegBorn |
( |
int |
a_Location_x, |
|
|
int |
a_Location_y |
|
) |
| |
|
inline |
◆ Set_XBorn()
void Vole_Base::Set_XBorn |
( |
int |
a_Location_x | ) |
|
|
inline |
Set x-coordinate of birth location
References m_XBorn.
Referenced by Init().
◆ Set_YBorn()
void Vole_Base::Set_YBorn |
( |
int |
a_Location_y | ) |
|
|
inline |
Set y-coordinate of birth location
References m_YBorn.
Referenced by Init().
◆ SetBreedingSeason()
void Vole_Base::SetBreedingSeason |
( |
bool |
a_flag | ) |
|
|
inline |
◆ SetDirectFlag()
void Vole_Base::SetDirectFlag |
( |
| ) |
|
|
inline |
◆ SetFertile()
void Vole_Base::SetFertile |
( |
bool |
f | ) |
|
|
inline |
Set the male vole fertility.
Primarily used in ecotoxicological simulations where toxic effects may render the vole sterile.
References m_fertile.
◆ SetFree()
void Vole_Base::SetFree |
( |
| ) |
|
|
inline |
◆ SetGeneticFlag()
void Vole_Base::SetGeneticFlag |
( |
| ) |
|
|
inline |
◆ SetLocation()
virtual void Vole_Base::SetLocation |
( |
| ) |
|
|
inlineprotectedvirtual |
◆ Setm_Mature()
void Vole_Base::Setm_Mature |
( |
| ) |
|
|
inline |
◆ SetWeight()
void Vole_Base::SetWeight |
( |
double |
W | ) |
|
|
inline |
◆ st_Dying()
void Vole_Base::st_Dying |
( |
void |
| ) |
|
◆ Step()
void Vole_Base::Step |
( |
void |
| ) |
|
|
inlineoverridevirtual |
◆ SupplyAge()
unsigned Vole_Base::SupplyAge |
( |
| ) |
|
|
inline |
◆ SupplyAllele()
int Vole_Base::SupplyAllele |
( |
int |
locus, |
|
|
int |
allele |
|
) |
| |
|
inline |
◆ SupplyBirthYear()
int Vole_Base::SupplyBirthYear |
( |
| ) |
|
|
inline |
◆ SupplyBornLastYear()
bool Vole_Base::SupplyBornLastYear |
( |
| ) |
|
|
inline |
◆ SupplyDeathCause()
int Vole_Base::SupplyDeathCause |
( |
| ) |
|
|
inline |
Tell the cause of death
References m_Death.
◆ SupplyElemBorn()
int Vole_Base::SupplyElemBorn |
( |
| ) |
|
|
inline |
Tell our elementype at birth location
References m_ElemBorn.
◆ SupplyElemType()
◆ SupplyFatherId()
int Vole_Base::SupplyFatherId |
( |
| ) |
|
|
inline |
◆ SupplyGenes()
◆ SupplyHeteroZyg()
int Vole_Base::SupplyHeteroZyg |
( |
| ) |
|
|
inline |
◆ SupplyHomoZyg()
int Vole_Base::SupplyHomoZyg |
( |
| ) |
|
|
inline |
◆ SupplyIDNo()
int Vole_Base::SupplyIDNo |
( |
| ) |
|
|
inline |
◆ SupplyInTrap()
bool Vole_Base::SupplyInTrap |
( |
| ) |
|
|
inline |
◆ SupplyMature()
bool Vole_Base::SupplyMature |
( |
| ) |
|
|
inline |
◆ SupplyMotherId()
int Vole_Base::SupplyMotherId |
( |
| ) |
|
|
inline |
◆ SupplyMyAllele()
uint32 Vole_Base::SupplyMyAllele |
( |
int |
i, |
|
|
int |
j |
|
) |
| |
|
inline |
◆ SupplyPolyRefBorn()
int Vole_Base::SupplyPolyRefBorn |
( |
| ) |
|
|
inline |
◆ SupplySex()
bool Vole_Base::SupplySex |
( |
| ) |
|
|
inline |
◆ SupplyTerritorial()
bool Vole_Base::SupplyTerritorial |
( |
| ) |
|
|
inline |
◆ SupplyTerrRange()
int Vole_Base::SupplyTerrRange |
( |
| ) |
|
|
inline |
◆ SupplyTotNoYoung()
int Vole_Base::SupplyTotNoYoung |
( |
| ) |
|
|
inline |
◆ SupplyTrapPosition()
◆ SupplyVegBorn()
int Vole_Base::SupplyVegBorn |
( |
| ) |
|
|
inline |
Tell our vegetation type at birth location
References m_VegBorn.
◆ SupplyWeight()
double Vole_Base::SupplyWeight |
( |
| ) |
|
|
inline |
◆ SupplyX()
unsigned Vole_Base::SupplyX |
( |
| ) |
|
|
inline |
◆ SupplyXBorn()
int Vole_Base::SupplyXBorn |
( |
| ) |
|
|
inline |
Tell our x-coordinate at birth
References m_XBorn.
◆ SupplyY()
unsigned Vole_Base::SupplyY |
( |
| ) |
|
|
inline |
◆ SupplyYBorn()
int Vole_Base::SupplyYBorn |
( |
| ) |
|
|
inline |
Tell our x-coordinate at birth
References m_YBorn.
◆ UnsetDirectFlag()
void Vole_Base::UnsetDirectFlag |
( |
| ) |
|
|
inline |
◆ UnsetGeneticFlag()
void Vole_Base::UnsetGeneticFlag |
( |
| ) |
|
|
inline |
◆ WhatState()
int Vole_Base::WhatState |
( |
| ) |
|
|
inlineoverridevirtual |
◆ CurrentVState
Our current behavioural state
Referenced by Vole_JuvenileMale::BeginStep(), Vole_JuvenileFemale::BeginStep(), Vole_JuvenileMale::EndStep(), Vole_Male::EndStep(), Vole_JuvenileFemale::EndStep(), Init(), Vole_JuvenileMale::OnFarmEvent(), Vole_JuvenileFemale::OnFarmEvent(), Vole_JuvenileMale::OnKilled(), Vole_JuvenileFemale::OnKilled(), Vole_JuvenileMale::st_BecomeSubAdult(), Vole_JuvenileFemale::st_BecomeSubAdult(), Vole_Male::st_Eval_n_Explore(), Vole_JuvenileMale::Step(), Vole_Male::Step(), Vole_JuvenileFemale::Step(), Vole_Female::Step(), and WhatState().
◆ IDNo
◆ m_Age
Their age in days
Referenced by Vole_Male::Dispersal(), EndStep(), Vole_JuvenileMale::EndStep(), Vole_Male::EndStep(), Vole_JuvenileFemale::EndStep(), Init(), Vole_JuvenileMale::ReInit(), Vole_Male::ReInit(), Vole_JuvenileFemale::ReInit(), Vole_Female::ReInit(), Set_Age(), Vole_Female::st_BecomeReproductive(), Vole_JuvenileMale::st_BecomeSubAdult(), Vole_JuvenileFemale::st_BecomeSubAdult(), Vole_JuvenileMale::st_Eval_n_Explore(), Vole_Male::st_Eval_n_Explore(), Vole_Female::st_Special_Explore(), Vole_JuvenileFemale::Step(), SupplyAge(), Vole_Female::Vole_Female(), Vole_JuvenileFemale::Vole_JuvenileFemale(), Vole_JuvenileMale::Vole_JuvenileMale(), and Vole_Male::Vole_Male().
◆ m_BirthYear
int Vole_Base::m_BirthYear |
|
protected |
◆ m_BornLastYear
bool Vole_Base::m_BornLastYear |
|
protected |
◆ m_BreedingSeason
bool Vole_Base::m_BreedingSeason = false |
|
staticprotected |
◆ m_Death
◆ m_DispVector
int Vole_Base::m_DispVector |
|
protected |
◆ m_ElemBorn
int Vole_Base::m_ElemBorn |
|
protected |
◆ m_FatherId
unsigned Vole_Base::m_FatherId |
|
protected |
◆ m_FemaleTerritoryRangeSlope
double Vole_Base::m_FemaleTerritoryRangeSlope = 0 |
|
staticprotected |
◆ m_fertile
bool Vole_Base::m_fertile |
|
protected |
◆ m_FHabQualThreshold1
double Vole_Base::m_FHabQualThreshold1 |
|
staticprotected |
Habitat is v.bad below this
◆ m_FHabQualThreshold2
double Vole_Base::m_FHabQualThreshold2 |
|
staticprotected |
◆ m_FHabQualThreshold3
double Vole_Base::m_FHabQualThreshold3 |
|
staticprotected |
Habitat is v.good above this
◆ m_Have_Territory
bool Vole_Base::m_Have_Territory |
|
protected |
◆ m_intrappos
◆ m_LifeSpan
int Vole_Base::m_LifeSpan |
|
protected |
◆ m_MaleTerritoryRangeSlope
double Vole_Base::m_MaleTerritoryRangeSlope = 0 |
|
staticprotected |
◆ m_Mature
◆ m_MaxFemaleTerritorySize
unsigned int Vole_Base::m_MaxFemaleTerritorySize = 0 |
|
staticprotected |
Maximum territory size female
Referenced by Init().
◆ m_MaxMaleTerritorySize
unsigned int Vole_Base::m_MaxMaleTerritorySize = 0 |
|
staticprotected |
◆ m_MHabQualThreshold1
double Vole_Base::m_MHabQualThreshold1 |
|
staticprotected |
Habitat is v.bad below this
◆ m_MHabQualThreshold2
double Vole_Base::m_MHabQualThreshold2 |
|
staticprotected |
◆ m_MHabQualThreshold3
double Vole_Base::m_MHabQualThreshold3 |
|
staticprotected |
Habitat is v.good above this
◆ m_MinFemaleTerritorySize
unsigned int Vole_Base::m_MinFemaleTerritorySize = 0 |
|
staticprotected |
◆ m_MinFVoleHabQual
double Vole_Base::m_MinFVoleHabQual = 0 |
|
staticprotected |
◆ m_MinJMVoleHabQual
double Vole_Base::m_MinJMVoleHabQual = 0 |
|
staticprotected |
◆ m_MinMaleTerritorySize
unsigned int Vole_Base::m_MinMaleTerritorySize = 0 |
|
staticprotected |
◆ m_MinMVoleHabQual
double Vole_Base::m_MinMVoleHabQual = 0 |
|
staticprotected |
◆ m_MinTerrRange
unsigned int Vole_Base::m_MinTerrRange |
|
protected |
The minimum territory range
Referenced by CalculateCarryingCapacity(), Init(), Vole_JuvenileMale::ReInit(), Vole_Male::ReInit(), Vole_JuvenileFemale::ReInit(), Vole_Female::ReInit(), Vole_Male::st_Infanticide(), Vole_Female::Vole_Female(), Vole_JuvenileFemale::Vole_JuvenileFemale(), Vole_JuvenileMale::Vole_JuvenileMale(), and Vole_Male::Vole_Male().
◆ m_MotherId
unsigned Vole_Base::m_MotherId |
|
protected |
◆ m_MyGenes
Their genes
Referenced by CopyMyself(), GetDirectFlag(), GetGeneticFlag(), Init(), SetDirectFlag(), SetGeneticFlag(), Vole_JuvenileMale::st_BecomeSubAdult(), Vole_JuvenileFemale::st_BecomeSubAdult(), Vole_Female::st_Lactating(), SupplyAllele(), SupplyGenes(), SupplyHeteroZyg(), SupplyHomoZyg(), SupplyMyAllele(), UnsetDirectFlag(), and UnsetGeneticFlag().
◆ m_NoOfYoungTotal
int Vole_Base::m_NoOfYoungTotal |
|
protected |
◆ m_OurPopulation
Referenced by Vole_JuvenileMale::BeginStep(), Vole_JuvenileFemale::BeginStep(), CalculateCarryingCapacity(), CheckTraps(), CopyMyself(), Vole_JuvenileMale::Dispersal(), Vole_Male::Dispersal(), Vole_JuvenileFemale::Dispersal(), Vole_Male::EndStep(), Vole_JuvenileFemale::EndStep(), Escape(), Vole_JuvenileMale::FreeLocation(), Vole_JuvenileFemale::FreeLocation(), Vole_JuvenileMale::GetLocation(), Vole_JuvenileFemale::GetLocation(), Init(), MoveQuality(), MoveTo(), Vole_JuvenileMale::OnFarmEvent(), Vole_JuvenileFemale::OnFarmEvent(), Vole_Female::OnInfanticideAttempt(), Vole_JuvenileMale::OnKilled(), Vole_JuvenileFemale::OnKilled(), Vole_JuvenileMale::SetLocation(), Vole_JuvenileFemale::SetLocation(), Vole_JuvenileMale::st_BecomeSubAdult(), Vole_JuvenileFemale::st_BecomeSubAdult(), Vole_JuvenileMale::st_Eval_n_Explore(), Vole_Male::st_Eval_n_Explore(), Vole_Female::st_GiveBirth(), Vole_Male::st_Infanticide(), Vole_Female::st_Lactating(), Vole_Female::st_Mating(), Vole_Male::st_Maturation(), Vole_Female::st_Special_Explore(), Vole_JuvenileFemale::Step(), and Vole_Female::Step().
◆ m_PolyRefBorn
int Vole_Base::m_PolyRefBorn |
|
protected |
◆ m_Reserves
int Vole_Base::m_Reserves |
|
protected |
◆ m_Sex
◆ m_SimH
◆ m_SimW
◆ m_TerrRange
int Vole_Base::m_TerrRange |
|
protected |
The size of their territory (radius of a square)
Referenced by CalculateCarryingCapacity(), CheckTraps(), Vole_Male::DetermineTerritorySize(), Vole_Male::Dispersal(), Vole_JuvenileFemale::EndStep(), Init(), Vole_JuvenileMale::ReInit(), Vole_Male::ReInit(), Vole_JuvenileFemale::ReInit(), Vole_Female::ReInit(), Vole_JuvenileMale::st_Eval_n_Explore(), Vole_Male::st_Eval_n_Explore(), Vole_Female::st_Special_Explore(), SupplyTerrRange(), Vole_Female::Vole_Female(), Vole_JuvenileFemale::Vole_JuvenileFemale(), Vole_JuvenileMale::Vole_JuvenileMale(), and Vole_Male::Vole_Male().
◆ m_VegBorn
◆ m_Weight
double Vole_Base::m_Weight |
|
protected |
Their weight in grams
Referenced by Vole_Male::DetermineTerritorySize(), Vole_JuvenileMale::EndStep(), Vole_Male::EndStep(), Vole_JuvenileFemale::EndStep(), Init(), Vole_JuvenileMale::ReInit(), Vole_Male::ReInit(), Vole_JuvenileFemale::ReInit(), Vole_Female::ReInit(), SetWeight(), Vole_JuvenileMale::st_BecomeSubAdult(), Vole_JuvenileFemale::st_BecomeSubAdult(), Vole_Male::st_Infanticide(), SupplyWeight(), Vole_Female::Vole_Female(), Vole_JuvenileFemale::Vole_JuvenileFemale(), Vole_JuvenileMale::Vole_JuvenileMale(), and Vole_Male::Vole_Male().
◆ m_XBorn
◆ m_YBorn
The documentation for this class was generated from the following files:
int m_LifeSpan
Definition: vole_all.h:182
void Set_ElemBorn(int a_Location_x, int a_Location_y)
Definition: vole_all.h:312
unsigned m_MotherId
Definition: vole_all.h:158
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
void UnsetGeneticFlag()
Definition: GeneticMaterial.cpp:175
int m_ElemBorn
Definition: vole_all.h:178
bool IsTrap(int p_x, int p_y) const
Definition: VolePopulationManager.h:201
static CfgInt cfg_vole_LifeMonths("VOLE_LIFEMONTHS", CFG_CUSTOM, 3)
TTypeOfVoleState CurrentVState
Definition: vole_all.h:406
virtual void Init(struct_Vole_Adult *a_AVoleStruct_ptr)
Definition: Vole_all.cpp:228
TTypesOfVegetation SupplyVegType(int a_x, int a_y)
Returns the vegetation type of the polygon using the polygon reference number a_polyref or coordinate...
Definition: Landscape.h:1925
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
unsigned int m_MinTerrRange
Definition: vole_all.h:156
const int MinReproWeightF
Definition: Vole_all.cpp:145
int SupplySimH() const
Returns landscape height in m.
Definition: PopulationManager.h:569
int m_BirthYear
Definition: vole_all.h:149
int SupplyHowManyVoles(unsigned a_x, unsigned a_y, unsigned a_range) const
Definition: VolePopulationManager.cpp:890
int MoveQuality(int p_x, int p_y) const
Test a location for quality while moving.
Definition: Vole_all.cpp:1186
static CfgInt cfg_MaxMaleTerrSize("VOLE_MAXMALETERRITORYSIZE", CFG_CUSTOM, 23)
int m_VegBorn
Definition: vole_all.h:180
bool m_Mature
Definition: vole_all.h:168
int m_guard_cell_y
The index y to the guard cell.
Definition: PopulationManager.h:374
const unsigned MinReproWeightM
Definition: Vole_all.cpp:143
int BackTranslateVegTypes(TTypesOfVegetation VegReference)
Returns the ALMaSS reference number translated from the TTypesOfVegetation type.
Definition: Landscape.h:2338
int HeterozygosityCount()
Definition: GeneticMaterial.cpp:325
static double m_MinJMVoleHabQual
Definition: vole_all.h:218
double value() const
Definition: Configurator.h:142
static CfgInt cfg_MaxFemaleTerrSize("VOLE_MAXFEMALETERRITORYSIZE", CFG_CUSTOM, 8)
A struct for passing data to create a new vole.
Definition: vole_all.h:115
unsigned m_x
Definition: PopulationManager.h:173
GeneticMaterial m_MyGenes
Definition: vole_all.h:205
void UnsetDirectFlag()
Definition: GeneticMaterial.cpp:179
void Set_MotherId(unsigned MotherIdNo)
Definition: vole_all.h:302
Definition: vole_all.h:55
uint32 GetDirectFlag()
Definition: GeneticMaterial.cpp:188
void Set_XBorn(int a_Location_x)
Definition: vole_all.h:308
int m_PolyRefBorn
Definition: vole_all.h:176
virtual bool GetLocation(int, int)
Definition: vole_all.h:446
bool m_Have_Territory
Definition: vole_all.h:196
double m_Weight
Definition: vole_all.h:184
void SetDirectFlag()
Definition: GeneticMaterial.cpp:170
void Set_YBorn(int a_Location_y)
Definition: vole_all.h:310
static unsigned int m_MaxFemaleTerritorySize
Definition: vole_all.h:210
void Set_VegBorn(int a_Location_x, int a_Location_y)
Definition: vole_all.h:319
const double MaxWeightM
Definition: Vole_all.cpp:127
TAnimal(int x, int y, Landscape *L)
The TAnimal constructor saving the x,y, location and the landscape pointer.
Definition: PopulationManager.cpp:1553
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
virtual void FreeLocation()
Definition: vole_all.h:444
int m_SimH
Definition: vole_all.h:202
const double MaxWeightF
Definition: Vole_all.cpp:129
static double m_MaleTerritoryRangeSlope
Definition: vole_all.h:222
int m_XBorn
Definition: vole_all.h:172
a_type GetMapValue(unsigned x, unsigned y)
Definition: MovementMap.h:140
GeneticMaterial Genes
Definition: vole_all.h:133
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
double g_DailyMortChance
Definition: Vole_all.cpp:183
void DoWalking(int p_Distance, int &p_Vector, int &vx, int &vy) const
Walking.
Definition: Vole_all.cpp:933
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
static CfgFloat cfg_vole_habqualscaler("VOLE_HABQUALSCALER", CFG_CUSTOM, 2.1)
static unsigned int m_MinMaleTerritorySize
Definition: vole_all.h:212
int FatherId
Definition: vole_all.h:127
void DoWalkingCorrect(int p_Distance, int &p_Vector, int &vx, int &vy) const
Walking where there is a danger of stepping off the world.
Definition: Vole_all.cpp:1022
Vole_Population_Manager * VPM
Definition: vole_all.h:131
int m_DispVector
Definition: vole_all.h:194
uint32 GetGeneticFlag()
Definition: GeneticMaterial.cpp:184
int BirthYear
Definition: vole_all.h:126
double SupplyRodenticide(int a_x, int a_y)
Gets total rodenticide for a location.
Definition: Landscape.cpp:586
static double m_MinFVoleHabQual
Definition: vole_all.h:216
uint32 GetAllele(int pos, int Chromosome)
Definition: GeneticMaterial.cpp:230
int m_SimW
Definition: vole_all.h:202
unsigned IDNumber
Definition: VolePopulationManager.h:219
bool m_Sex
Definition: vole_all.h:166
int SupplyYearNumber(void)
Passes a request on to the associated Calendar class function, returns m_simulationyear
Definition: Landscape.h:2287
static unsigned int m_MaxMaleTerritorySize
Definition: vole_all.h:208
int value() const
Definition: Configurator.h:116
TTypesOfLandscapeElement m_EleType
Definition: PopulationManager.h:175
unsigned IDNo
Definition: vole_all.h:200
int y
Definition: vole_all.h:118
unsigned m_y
Definition: PopulationManager.h:174
static CfgInt cfg_MaxStarvationDays("VOLE_MAXSTARVATIONDAYS", CFG_CUSTOM, 29)
Landscape * L
Definition: vole_all.h:130
void UpdateGuardMap(int a_x, int a_y, int &a_index_x, int &a_index_y)
Get the index of the guard map for the given location.
Definition: PopulationManager.h:768
void CreateObjects(VoleObject a_obType, TAnimal *a_pvo, struct_Vole_Adult *a_as, int a_number)
Definition: VolePopulationManager.cpp:2756
void Set_PolyRefBorn(int a_Location_x, int a_Location_y)
Definition: vole_all.h:326
InTrapPosition m_intrappos
Definition: vole_all.h:206
void Set_BirthYear(int BirthYear)
Definition: vole_all.h:299
static CfgFloat cfg_volepcidebiodegredrate("VOLE_PCIDE_BIODEGREDATIONRATE", CFG_CUSTOM, 0.0)
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Vole_Population_Manager * m_OurPopulation
Definition: vole_all.h:407
int MotherId
Definition: vole_all.h:128
int m_guard_cell_x
The index x to the guard cell.
Definition: PopulationManager.h:370
int m_Age
Definition: vole_all.h:170
double GetHabitatQuality(const int a_index) const
Definition: VolePopulationManager.h:230
static double m_MinMVoleHabQual
Definition: vole_all.h:220
void Set_FatherId(unsigned FatherIdNo)
Definition: vole_all.h:304
int SupplySimW() const
Returns landscape width in m.
Definition: PopulationManager.h:567
int m_YBorn
Definition: vole_all.h:174
void SetGeneticFlag()
Definition: GeneticMaterial.cpp:166
bool m_BornLastYear
A flag set if the female was born the year before.
Definition: vole_all.h:154
double MoveToLessFavourable
Definition: VolePopulationManager.cpp:155
bool m_inAtrap
Definition: vole_all.h:108
static double m_FemaleTerritoryRangeSlope
Definition: vole_all.h:224
int m_Death
Definition: vole_all.h:162
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
int HomozygosityCount()
Definition: GeneticMaterial.cpp:314
unsigned m_FatherId
Definition: vole_all.h:160
int x
Definition: vole_all.h:117
int m_CurrentStateNo
The basic state number for all objects - '-1' indicates death.
Definition: PopulationManager.h:131
int vole_tole_move_quality(Landscape *m_TheLandscape, int x, int y)
Definition: Vole_toletoc.cpp:5
int SupplyPolyRefIndex(int a_x, int a_y)
Get the index to the m_elems array for a polygon at location x,y.
Definition: Landscape.h:2172
CfgInt cfg_MinMaleTerritorySize("VOLE_MINMALETERRITORYSIZE", CFG_CUSTOM, 9)
static unsigned int m_MinFemaleTerritorySize
Definition: vole_all.h:214
bool m_fertile
Flag indicating the fertility state (true means fertile)
Definition: vole_all.h:186
int m_TerrRange
Definition: vole_all.h:164
int m_Reserves
Definition: vole_all.h:198
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
CfgInt cfg_MinFemaleTerritorySize("VOLE_MINFEMALETERRITORYSIZE", CFG_CUSTOM, 8)
int SupplyPolyRef(int a_x, int a_y)
Get the in map polygon reference number from the x, y location.
Definition: Landscape.h:2157
static bool m_BreedingSeason
Definition: vole_all.h:239
virtual void SetLocation()
Definition: vole_all.h:441
IDMap< TAnimal * > * m_VoleMap
Definition: VolePopulationManager.h:276
int BackTranslateEleTypes(TTypesOfLandscapeElement EleReference)
Returns the ALMaSS reference number translated from the TTypesOfLandscapeElement type.
Definition: Landscape.h:2332
int m_NoOfYoungTotal
Definition: vole_all.h:192
TTypesOfVegetation m_VegType
Definition: PopulationManager.h:176