File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
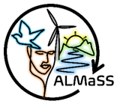 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
26 #ifndef Skylarks_All_H
27 #define Skylarks_All_H
30 #define UNREFERENCED_PARAMETER(P) (P)
31 #define SKOPTIMALHABITATSCORE 50
63 toss_MTempLeavingArea,
64 toss_MFindingTerritory,
67 toss_ScaringOffChicks,
77 toss_FTempLeavingArea,
78 toss_FFindingTerritory,
79 toss_BuildingUpResources,
81 toss_PreparingForBreeding,
83 toss_StartingNewBrood,
86 toss_StoppingBreeding,
89 toss_GivingUpTerritory,
127 for (
unsigned j = 0; j < this->GetItemsInContainer(); j++)
129 Sp = this->Get(j)->SupplyPosition();
130 const unsigned Farm = this->Get(j)->SupplyFarmOwnerRef();
131 for (
unsigned i = 0; i < p_TheProbe->
m_NoAreas; i++)
135 for (
unsigned k = 0; k < p_TheProbe->
m_NoFarms; k++)
144 for (
unsigned j = 0; j < this->GetItemsInContainer(); j++)
146 Sp = this->Get(j)->SupplyPosition();
147 for (
unsigned i = 0; i < p_TheProbe->
m_NoAreas; i++)
162 for (
unsigned j = 0; j < this->GetItemsInContainer(); j++)
164 Sp = this->Get(j)->SupplyPosition();
165 for (
unsigned i = 0; i < p_TheProbe->
m_NoAreas; i++)
180 for (
unsigned j = 0; j < this->GetItemsInContainer(); j++)
182 Sp = this->Get(j)->SupplyPosition();
183 for (
unsigned i = 0; i < p_TheProbe->
m_NoAreas; i++)
281 skTTerritory(
int x,
int y,
int TheSize,
int TheQuality,
int a_x_div10,
int a_y_div10,
int a_range_div10);
283 void TestNestPossibility();
285 m_nest_valid = valid;
286 m_nest_pos_validx = nx;
287 m_nest_pos_validy = ny;
316 const int l_size = static_cast<int>(m_sizes.size());
317 m_sizes.resize(l_size + 1);
318 m_polys.resize(l_size + 1);
319 m_polys[l_size] = a_poly;
335 int EvaluateHabitatSquare(
int xmin,
int xmax,
int ymin,
int ymax,
int NoPolygons);
350 int m_for_iter_x[1000];
351 int m_for_iter_y[1000];
352 static int PreMakeForIterator(
int a_min_incl,
int a_max_excl,
int* a_iter,
int a_norm_max_excl);
354 void PreFillQualGrid(
void);
355 void PreEvaluateQualGrid(
SkQualGrid* a_grid,
int a_x,
int a_y,
int a_width,
int a_height);
356 void PreFillQualCache(
void);
363 int PreEvaluateHabitat(
int a_x,
int a_y,
int a_range_x,
int a_range_y)
const;
364 int PreEvaluateHabitatStripX(
int a_x,
int a_y,
int a_range_x)
const;
365 int PreEvaluateHabitatStripY(
int a_x,
int a_y,
int a_range_x)
const;
367 void DumpMapGraphics(
const char* a_filename,
Landscape* a_map)
const;
372 void Tick(
void) { m_qual_cache_filled =
false; }
373 void EvaluateAllTerritories(
void);
374 void PreCachePoly(
int a_poly);
375 double PrePoly2Qual(
int a_poly);
376 double PrePolyNQual(
int a_poly,
int* a_good_polys);
377 int PolyRefData[2500];
378 int PolySizeData[2500];
379 double PolyHeightData[2500];
383 void ClaimGrid(
int x,
int y,
int range)
const;
384 void UpdateQuality();
385 bool IsGridPositionValid(
const int& a_x,
const int& a_y,
int a_range)
const;
386 bool IsExtGridPositionValid(
const int& x,
const int& y,
int range)
const;
387 int IsValid(
int nx,
int ny)
const;
388 void GetTerritoriesByDistance(
int nx,
int ny, vector<APoint>* alist)
const;
389 int Supply_quality(
int ref)
const;
390 int Supply_x(
int ref)
const;
391 int Supply_y(
int ref)
const;
392 int Supply_size(
int ref)
const;
394 void RemoveFemale(
int ref)
const;
395 void RemoveMale(
int ref)
const;
402 int SupplyNoTerritories()
const;
403 int SupplyNoMaleOccupied()
const;
404 int SupplyNoFemaleOccupied()
const;
407 const APoint p(Territories[ref]->m_nest_pos_validx, Territories[ref]->m_nest_pos_validy);
428 void DoFirst()
override;
429 void ProbeReportPOM(
int a_time);
430 float ProbePOM(
int ListIndex,
const Probe_Data* p_TheProbe);
434 void LoadParameters();
435 int m_StriglingMort[4];
449 virtual void Init(
void);
451 void WriteSKPOM1(
const int n,
const int n2)
const { fprintf(SKPOM1,
"%i\t%i\n", n, n2); }
452 void WriteSKPOM2(
const int n,
const int n2)
const { fprintf(SKPOM2,
"%i\t%i\n", n, n2); }
458 int SupplyNoTerritories()
const;
459 int TheSkylarkTerrsSupply_x(
int)
const;
460 int TheSkylarkTerrsSupply_y(
int)
const;
461 int TheSkylarkTerrsSupply_size(
int)
const;
462 int TheSkylarkTerrsSupply_quality(
int)
const;
471 int TheFledgelingProbe()
override;
472 void BreedingPairsOutput(
int Time)
override;
473 void FledgelingProbeOutput(
int Total,
int Time)
override;
474 int TheBreedingFemalesProbe(
int ProbeNo)
override;
475 bool OpenTheBreedingPairsProbe()
override;
476 bool OpenTheFledgelingProbe()
override;
498 bool OpenTheBreedingSuccessProbe()
override;
499 void BreedingSuccessProbeOutput(
double,
int,
int,
int,
int,
int,
int,
int)
override;
500 int TheBreedingSuccessProbe(
int& BreedingFemales,
int& YoungOfTheYear,
int& TotalPop,
int& TotalFemales,
int& TotalMales,
501 int& BreedingAttempts)
override;
502 void TheAOROutputProbe()
override;
503 virtual void TheRipleysOutputProbe(FILE* a_prb);
506 void Catastrophe()
override;
507 virtual void ReHouse();
539 static bool DailyMortality(
int mort);
540 #ifdef __PESTICIDE_RA
543 double m_pesticide_accumulation;
545 virtual void PesticideResponse() {
561 bool OnFarmEvent(
FarmToDo event)
override;
565 void BeginStep(
void)
override;
566 void Step(
void)
override;
567 void EndStep(
void)
override;
576 m_CurrentSkState = toss_Developing;
578 m_OurPopulationManager->incTotalEggs(Clutch_Size);
599 virtual int st_Developing();
600 virtual void st_Maturing();
601 virtual void st_Dying();
602 bool OnFarmEvent(
FarmToDo event)
override;
603 #ifdef __PESTICIDE_RA
604 void PesticideResponse(
void)
override;
612 void BeginStep(
void)
override;
613 void Step(
void)
override;
614 void EndStep(
void)
override;
616 double On_FoodSupply(
double food)
override;
618 void OnYouHaveBeenEaten();
626 int st_Developing()
override;
627 void st_Maturing()
override;
628 void st_Dying()
override;
629 double GetFood()
const;
630 double GetFledgelingEM(
int Age)
const;
631 bool OnFarmEvent(
FarmToDo event)
override;
632 #ifdef __PESTICIDE_RA
633 void PesticideResponse()
override;
641 void BeginStep(
void)
override;
642 void Step(
void)
override;
643 void EndStep(
void)
override;
651 virtual double RemoveEM(
double food);
652 double GetVegHindrance(
int PolyRef)
const;
653 double GetWeatherHindrance()
const;
654 static bool GetBadWeather();
671 virtual void CopyMyself(
int a_sktype);
681 bool st_Immigrating();
683 int st_TempLeavingArea();
684 int st_Finding_Territory();
686 int st_CaringForYoung();
687 int st_BuildingUpResources();
689 int st_PreparingForBreeding();
690 int st_GivingUpTerritory();
692 int st_StartingNewBrood();
693 int st_EggHatching();
695 int st_StoppingBreeding();
696 double GetFood(
int time);
697 int CalculateEggNumber()
const;
698 int CalcFoodTime(
double target)
const;
699 int GetMigrationMortality();
700 double CheckForFields()
const;
702 bool OnFarmEvent(
FarmToDo event)
override;
703 #ifdef __PESTICIDE_RA
704 void PesticideResponse()
override;
730 void BeginStep(
void)
override;
731 void Step(
void)
override;
732 void EndStep(
void)
override;
733 void EstablishTerritory();
740 int Supply_BreedingAttempts()
const;
741 int Supply_BreedingSuccess()
const;
742 void ResetBreedingSuccess();
747 void OnClutchDeath();
749 void OnBreedingSuccess();
750 void OnStopFeedingChicks();
752 void OnMateHomeless();
766 bool st_Arriving()
const;
767 bool st_Immigrating();
769 int st_TempLeavingArea();
770 int st_FindingTerritory();
771 int st_AttractingAMate();
772 int st_FollowingMate();
773 void ConstructAHabitatTable();
774 int EstablishingATerritory();
775 int st_ScaringOffChicks();
776 int st_CaringForYoung();
779 void ReEvaluateTerritory();
780 double GetFood(
int time);
781 void OptimiseHabitatSearchingOrder();
782 int GetMigrationMortality();
783 bool OnFarmEvent(
FarmToDo event)
override;
784 #ifdef __PESTICIDE_RA
785 void PesticideResponse()
override;
809 #ifdef __PESTICIDE_RA
810 vector<double> m_PConcTable;
820 void BeginStep(
void)
override;
821 void Step(
void)
override;
822 void EndStep(
void)
override;
841 #ifndef __PESTICIDE_RA_CHICK
847 double OnFoodMessage(
int n,
double f ,
double p )
850 m_Brood[n]->m_pesticide_accumulation+= (((f-extra)/f)*p);
858 int DefendTerritory()
const;
861 void OnMateLeaving();
864 void OnNestLocation(
int x,
int y);
869 void OnNestPredatation();
870 void OnBroodDesertion();
873 bool SupplyNestValid()
const {
return m_OurPopulationManager->TheSkylarkTerrs->SupplyIsNestValid(MyTerritory.ref); }
874 APoint SupplyNestLoc()
const {
return m_OurPopulationManager->TheSkylarkTerrs->SupplyNestPosition(MyTerritory.ref); }
CfgFloat cfg_PEmax("SK_PEMAX", CFG_CUSTOM, 4.54)
CfgFloat cfg_tramline_foraging("SK_TRAMLINE_FORAGING_PROP", CFG_CUSTOM, 0.45)
void Step(void) override
Step behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:3306
int DefendTerritory() const
Definition: skylarks_all.cpp:4520
vector< int > m_polys
Definition: skylarks_all.h:312
bool NestLoc
Definition: skylarks_all.h:713
void SetNestPossibility(const bool valid, const int nx, const int ny)
Definition: skylarks_all.h:284
Definition: PopulationManager.h:59
int Supply_m_Location_x() const
Returns the ALMaSS x-coordinate.
Definition: PopulationManager.h:239
Definition: Treatment.h:129
int No
Definition: skylarks_all.h:225
Definition: Treatment.h:64
CfgFloat cfg_skylark_pesticide_globaleggshellreduction
The proportion of eggs assumed to crack from pesticide effects as a global effect.
CfgInt cfg_SkStartNos("SK_STARTNOS", CFG_CUSTOM, 6000)
The number of skylarks that start in the simulation.
Definition: Treatment.h:84
int y
Definition: skylarks_all.h:201
CfgFloat cfg_hindconstantH_b("SK_HINDCONSTH_B", CFG_CUSTOM,-0.025)
Definition: Treatment.h:71
CfgFloat cfg_MinDaysToHatch
The time taken to egg hatch under optimal conditions.
static int NestlingMortProb
Definition: skylarks_all.cpp:159
TTypesOfSkState st_Floating()
Definition: skylarks_all.cpp:4561
double m_EM
Definition: skylarks_all.h:596
Definition: Treatment.h:88
int SupplyNoFemaleOccupied() const
Definition: skylarks_all.cpp:1373
Definition: Treatment.h:68
Definition: MapErrorMsg.h:37
bool SupplyNestValid() const
Definition: skylarks_all.h:873
int PreEvaluateHabitat(int a_x, int a_y, int a_range_x, int a_range_y) const
Definition: skylarks_all.cpp:1600
int st_FollowingMate()
Definition: skylarks_all.cpp:4577
void PreFillQualGrid(void)
Definition: skylarks_all.cpp:1495
int m_NoPestEffects
Definition: skylarks_all.h:441
void incTotalEggs(const int eggs)
Definition: skylarks_all.h:484
Definition: Treatment.h:144
Definition: skylarks_all.h:552
Definition: Treatment.h:52
Definition: Treatment.h:89
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
Skylark_Female * F_Owner
Definition: skylarks_all.h:280
virtual double RemoveEM(double food)
Definition: skylarks_all.cpp:3527
Definition: Treatment.h:94
static int Breed_Res_Thresh1
Definition: skylarks_all.cpp:167
CfgFloat cfg_densityconstant_b("SK_DENSITYCONST_B", CFG_CUSTOM,-0.26)
void BeginStep(void) override
BeingStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:3302
CfgInt cfg_densityconstant_c("SK_DENSITYCONST_C", CFG_CUSTOM, 10)
Definition: Treatment.h:142
vector< int > m_HabitatTable_Size
Definition: skylarks_all.h:807
bool SupplyIsNestValid(const int ref) const
Definition: skylarks_all.h:405
int SupplyF_Mig_Mort() const
Definition: skylarks_all.h:456
void AddStriglingMort(const int lifestage) const
Definition: skylarks_all.h:531
void OnReHouse()
Definition: skylarks_all.cpp:4085
static int PreMakeForIterator(int a_min_incl, int a_max_excl, int *a_iter, int a_norm_max_excl)
Definition: skylarks_all.cpp:1875
virtual TAnimal * SupplyAnimalPtr(unsigned int a_index, unsigned int a_animal)
Returns the pointer indexed by a_index and a_animal. Note NO RANGE CHECK.
Definition: PopulationManager.h:678
double SupplyTemp(void)
Passes a request on to the associated Weather class function, the temperature for the current day.
Definition: Landscape.h:1993
Definition: skylarks_all.h:648
Definition: skylarks_all.h:198
CfgFloat cfg_skylark_pesticide_eggshellreduction("SK_PESTICIDEEGGSHELLREDUC", CFG_CUSTOM, 0.0)
The proportion of eggs assumed to crack from pesticide effects.
CfgFloat cfg_NestLeavingWeight("SK_NEST_LEAVING_WEIGHT", CFG_CUSTOM, 20.5)
void OptimiseHabitatSearchingOrder()
Definition: skylarks_all.cpp:4971
Definition: Treatment.h:83
int m_nest_pos_validy
Definition: skylarks_all.h:269
Definition: Treatment.h:99
void Tick(void)
Definition: skylarks_all.h:372
void AddStriglingMort(const int lifestage)
Definition: skylarks_all.h:490
int WhatState() override
Returns the objects current state number.
Definition: skylarks_all.h:534
CfgInt cfg_PmEventfrequency
TTypesOfVegetation SupplyVegType(int a_x, int a_y)
Returns the vegetation type of the polygon using the polygon reference number a_polyref or coordinate...
Definition: Landscape.h:1925
CfgInt cfg_HQualityTrack("SK_HQTRACK", CFG_CUSTOM, 10)
int m_NestTime
Definition: skylarks_all.h:712
Definition: Treatment.h:76
skTTerritory * Supply_terr(int ref) const
Definition: skylarks_all.cpp:1380
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
CfgInt cfg_NestLeavingChance("SK_NESTLEAVECHANCE", CFG_CUSTOM, 23)
double EMi
Definition: skylarks_all.h:433
Definition: Treatment.h:35
void Step(void) override
Step behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:5258
void PreFillQualCache(void)
Definition: skylarks_all.cpp:1573
Definition: Treatment.h:81
Definition: skylarks_all.h:297
~SkTerritories()
Definition: skylarks_all.cpp:1345
Definition: LandscapeFarmingEnums.h:94
int m_sim_h_div_10
Definition: skylarks_all.h:337
CfgFloat cfg_EM_Nestling_b
int st_AttractingAMate()
Definition: skylarks_all.cpp:4543
bool validnest
Definition: skylarks_all.h:304
CfgInt cfg_ClutchMortProb("SK_CLUTCH_MORT_PROB", CFG_CUSTOM, 350)
static int ClutchMortProb
Definition: skylarks_all.cpp:158
bool OnEvicted()
Definition: skylarks_all.cpp:4063
int SupplySimH() const
Returns landscape height in m.
Definition: PopulationManager.h:569
CfgFloat cfg_skylark_pesticide_eggshellreduction
The proportion of eggs assumed to crack from pesticide effects.
CfgInt cfg_strigling_clutch("SK_STRIGLING_C", CFG_CUSTOM, 72)
std::string VegtypeToString(TTypesOfVegetation a_veg)
Returns the text representation of a TTypesOfVegetation type.
Definition: Landscape.cpp:6518
double m_heterogeneity
Definition: skylarks_all.h:292
Definition: Treatment.h:43
Definition: Treatment.h:91
void Step(void) override
Step behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:2859
CfgFloat cfg_Skylark_female_NOEL
Can be used to trigger a response to pesticides for the females.
Definition: Treatment.h:127
virtual void Init(void)
Definition: skylarks_all.cpp:297
Definition: Treatment.h:103
double HeightScore[111]
Definition: skylarks_all.cpp:193
CfgFloat cfg_ReturnProbability
Used to change the timing of start of arrival in the landscape.
TTypesOfPopulation m_population_type
Definition: PopulationManager.h:858
Definition: Treatment.h:121
CfgInt cfg_HQualityWater("SK_HQWATER", CFG_CUSTOM, 0)
int SupplyNoHabitatRefs() const
Definition: skylarks_all.h:830
int SupplyBroodSize() const
Definition: skylarks_all.h:832
virtual void st_Maturing()
Definition: skylarks_all.cpp:2999
void SetQuality(const int a_qual)
Definition: skylarks_all.h:278
double GetVegHindrance(int PolyRef) const
Definition: skylarks_all.cpp:3567
int GoodWeather
Definition: skylarks_all.h:790
bool sex
Definition: skylarks_all.h:251
bool SupplySkScrapes(int a_polyref)
Returns the presence of skylark scrapes in the vegetation using the polygon reference number a_polyre...
Definition: Landscape.h:1567
static int FoodTripsPerDay
Definition: skylarks_all.cpp:166
void PreCachePoly(int a_poly)
Definition: skylarks_all.cpp:1493
int st_ScaringOffChicks()
Definition: skylarks_all.cpp:4779
virtual void ReInit(int x, int y, Landscape *L, SkTerritories *Terrs, Skylark_Male *Daddy, bool sex, double size, int age, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3125
vector< unsigned > BeforeStepActions
Holds the season list of possible before step actions.
Definition: PopulationManager.h:819
Landscape * g_land
Definition: skylarks_all.cpp:44
Definition: Treatment.h:44
CfgInt cfg_CatastropheEventStartYear
void SetVirtualDiameter(const double dia)
Definition: skylarks_all.h:272
Definition: Treatment.h:56
Definition: Treatment.h:125
int HQualityOpenTallVeg
Definition: skylarks_all.cpp:228
Skylark_Clutch * SupplyMyClutch() const
Definition: skylarks_all.h:738
int GetMigrationMortality()
Definition: skylarks_all.cpp:4532
CfgInt cfg_HQualityHedge("SK_HQHEDGE", CFG_CUSTOM, -250)
void CorrectCoords(int &x, int &y)
Function to prevent wrap around errors with co-ordinates using x/y pair.
Definition: Landscape.h:2206
vector< int > m_polys
Definition: skylarks_all.h:259
const double KcalPerGInsect_inv
Definition: skylarks_all.cpp:176
virtual void ReHouse()
Definition: skylarks_all.cpp:1145
void OnStopFeedingChicks()
Definition: skylarks_all.cpp:5458
TTypesOfSkState m_CurrentSkState
Definition: skylarks_all.h:517
Skylark_Male * Dad
Definition: skylarks_all.h:241
int SupplyGrazingPressure(int a_polyref)
Returns the grazing pressure of the polygon using the polygon reference number a_polyref or coordinat...
Definition: Landscape.h:1848
double VegHindranceD[111]
Definition: skylarks_all.cpp:191
void Split(int ref)
Definition: skylarks_all.cpp:2034
double m_competitionscaler
Definition: skylarks_all.h:270
void incNoFledgeDeaths()
Definition: skylarks_all.h:478
Definition: Treatment.h:61
Definition: Treatment.h:80
Definition: Treatment.h:60
int HQualityMetalRoad
Definition: skylarks_all.cpp:232
class Calendar * g_date
Definition: Calendar.cpp:37
CfgInt cfg_PreFledgeMortProb
Definition: Treatment.h:90
Landscape * L
Definition: skylarks_all.h:214
void OnYouHaveBeenEaten()
Definition: skylarks_all.cpp:3037
const char * m_ListNames[32]
A list of life-stage names.
Definition: PopulationManager.h:628
Definition: Treatment.h:123
int TramlinePremium
Definition: skylarks_all.cpp:243
CfgFloat cfg_Skylark_nestling_NOEL
Can be used to trigger a response to pesticides for the nestlings.
int Supply_size(int ref) const
Definition: skylarks_all.cpp:1394
CfgFloat cfg_EM_Nestling_a("SK_EM_NESTLING_A", CFG_CUSTOM, 0.8542)
CfgBool cfg_AorOutput_used
Definition: Treatment.h:131
void WriteSKPOM1(const int n, const int n2) const
Definition: skylarks_all.h:451
static double EggTemp
Definition: skylarks_all.cpp:163
int x
Definition: skylarks_all.h:200
double SupplyEMi() const
Definition: skylarks_all.h:492
static bool GetBadWeather()
Extreme weather conditions check.
Definition: skylarks_all.cpp:3521
double PrePoly2Qual(int a_poly)
Definition: skylarks_toletov.cpp:103
bool m_IsBadWeather
Definition: skylarks_all.h:442
const int July
Julian start dates of the month of July.
Definition: Landscape.h:50
Definition: Treatment.h:138
Definition: Treatment.h:122
CfgInt cfg_juvreturnmort
Immigration mortality for juveniles.
Definition: Treatment.h:39
void RemoveFemale(int ref) const
Definition: skylarks_all.cpp:2026
int age
Definition: skylarks_all.h:252
Definition: Treatment.h:110
void RemoveMale(int ref) const
Definition: skylarks_all.cpp:2030
bool SupplySnowcover(void)
Passes a request on to the associated Weather class function, the snow cover for the current day.
Definition: Landscape.h:2091
int NoTerritories
Definition: skylarks_all.h:329
CfgInt cfg_insecticide_direct_mortM("SK_INSECTICDEDIRECTMORTM", CFG_CUSTOM, 0)
double OnFoodMessage(const int n, const double f) const
Definition: skylarks_all.h:842
void ReEvaluateTerritory()
Daily re-evaluation of territory.
Definition: skylarks_all.cpp:4608
CfgInt cfg_HQualityTallVeg
Definition: Treatment.h:51
int st_Incubating()
Definition: skylarks_all.cpp:6237
void SkylarkEvaluation(SkTerritories *a_skt)
Evaluation to find Skylark territory.
Definition: Landscape.cpp:5095
int m_Quality
Definition: skylarks_all.h:293
double value() const
Definition: Configurator.h:142
int by
Definition: skylarks_all.h:212
int st_Developing()
Definition: skylarks_all.cpp:2451
void ProbeReportPOM(int a_time)
Definition: skylarks_all.cpp:1073
int SupplyBroodAge(const int n) const
Definition: skylarks_all.h:834
CfgInt cfg_strigling_preflg("SK_STRIGLING_PF", CFG_CUSTOM, 72)
SkTerritories(Landscape *L)
Definition: skylarks_all.cpp:1331
Definition: Treatment.h:143
const double EM_nest_T[14]
Definition: skylarks_all.cpp:144
FarmToDo
Definition: Treatment.h:31
Skylark_Population_Manager(Landscape *L)
Definition: skylarks_all.cpp:268
int PatchyPremium
Definition: skylarks_all.cpp:239
CfgInt cfg_adultreturnmort
Immigration mortality for juveniles.
Definition: Treatment.h:133
CfgFloat cfg_Cooling_Rate_Eggs("SK_COOLING_RATE_EGGS", CFG_CUSTOM, 3.0)
Maximum immigration mortality for females.
void incTotalPrefledgelings()
Definition: skylarks_all.h:488
CfgInt cfg_FoodTripsPerDay
int m_Born_y
Definition: skylarks_all.h:521
void OnBroodDeath()
Definition: skylarks_all.cpp:4214
int m_sim_w_div_10
Definition: skylarks_all.h:337
void st_Dying()
Definition: skylarks_all.cpp:2602
void LoadParameters()
Definition: skylarks_all.cpp:423
const int February
Julian start dates of the month of February.
Definition: Landscape.h:40
CfgFloat cfg_SkScrapesPremiumII
unsigned m_x
Definition: PopulationManager.h:173
Definition: Treatment.h:104
Definition: Treatment.h:85
int x
Definition: skylarks_all.h:209
int Supply_BreedingAttempts() const
Definition: skylarks_all.cpp:5894
int ref
Definition: skylarks_all.h:302
unsigned m_y1
Definition: PopulationManager.h:117
CfgBool cfg_ReallyBigOutputMonthly_used
CfgFloat cfg_sk_triplength
void OnMaleNeverComesBack(const Skylark_Male *AMale)
Definition: skylarks_all.cpp:5879
int m_qual_grid_signal
Definition: skylarks_all.h:338
void OnPairing(Skylark_Female *female)
Definition: skylarks_all.cpp:4729
double TempHindrance[31]
Definition: skylarks_all.cpp:189
int g_stopdate
Definition: skylarks_all.cpp:40
static double MeanHatchingWeight
Definition: skylarks_all.cpp:161
int st_Emigrating()
Definition: skylarks_all.cpp:5556
SkTerritories * TheSkylarkTerrs
Definition: skylarks_all.h:496
void OnAddNestling(Skylark_Nestling *N)
Definition: skylarks_all.cpp:4649
Definition: skylarks_all.h:588
int SimW
Definition: skylarks_all.h:330
SkTerritories * m_OurTerritories
Definition: skylarks_all.h:525
CfgFloat cfg_heightconstant_b
Definition: Treatment.h:140
int HQualityNeutral
Definition: skylarks_all.cpp:234
Definition: Treatment.h:38
void BeginStep(void) override
BeingStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:5209
Definition: Treatment.h:117
virtual int st_Developing()
Definition: skylarks_all.cpp:2937
void SetF_Mig_Mort(const int m)
Definition: skylarks_all.h:466
int JuvenileReturnMort
Definition: skylarks_all.cpp:199
Definition: Treatment.h:120
virtual void ReInit(int x, int y, Skylark_Male *Daddy, Landscape *L, SkTerritories *Terrs, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:2635
Definition: skylarks_all.h:112
CfgFloat cfg_hindconstantD_b
CfgBool cfg_ReallyBigOutputUsed
int m_ListNameLength
the number of life-stages simulated in the population manager
Definition: PopulationManager.h:626
Definition: Treatment.h:59
int Insert(const int a_poly)
Definition: skylarks_all.h:315
CfgFloat cfg_NestPlacementMinQual("SK_NESTPLACEMENTMINQUAL", CFG_CUSTOM, 15)
bool OnFarmEvent(FarmToDo event) override
Must be reimplemented if used in descendent classes. Sets the action on a management event.
Definition: skylarks_all.cpp:2653
int Supply_BreedingSuccess() const
Definition: skylarks_all.cpp:5904
int GetQuality(void) const
Definition: skylarks_all.h:277
int st_TempLeavingArea()
Definition: skylarks_all.cpp:5595
double m_XFNestAcceptScore
Definition: skylarks_all.h:788
const double EM_warmblood2
Definition: skylarks_all.cpp:182
Definition: Treatment.h:100
CfgInt cfg_HQualityMetalRoad
int m_NestLeavingChance
Definition: skylarks_all.h:593
int st_FindingTerritory()
Definition: skylarks_all.cpp:4400
Definition: skylarks_all.h:223
int GetMigrationMortality()
Definition: skylarks_all.cpp:6566
void AddEgg()
Definition: skylarks_all.h:573
const double VegQuality[31]
Definition: skylarks_all.cpp:171
unsigned SupplyFarmOwnerRef() const
Get the current location farm ref if any.
Definition: PopulationManager.cpp:1547
void EndStep(void) override
EndStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:2398
Definition: Treatment.h:150
double VegHindranceH[111]
Definition: skylarks_all.cpp:190
Definition: Treatment.h:124
CfgFloat cfg_heightconstant_a("SK_HEIGHTCONST_A", CFG_CUSTOM, 1)
int SupplyVegDensity(int a_polyref)
Returns the density of the vegetation using the polygon reference number a_polyref or based on the x,...
Definition: Landscape.h:1562
FILE * SKPOM1
Definition: skylarks_all.h:444
double GetFood() const
Definition: skylarks_all.cpp:3426
CfgInt cfg_HQualityTall2("SK_HQTALLVEGTWO", CFG_CUSTOM, -1000)
bool not_nest_friendly(int x, int y)
Definition: skylarks_toletov.cpp:23
void SensibleCopy()
Definition: skylarks_all.cpp:6620
Definition: Treatment.h:139
int st_BuildingUpResources()
Definition: skylarks_all.cpp:5924
CfgInt cfg_fecundity_reduc_chance("SK_FECUNDITY_REDUC_CHANCE", CFG_CUSTOM, 0)
static double MD_Hatch
Definition: skylarks_all.cpp:168
Definition: Treatment.h:126
int m_pesticide_affected
Definition: skylarks_all.h:659
CfgInt cfg_HQualityMetalRoad("SK_HQMETALROAD", CFG_CUSTOM, -10)
int * VegTypeFledgelings
Definition: skylarks_all.h:497
vector< int > m_hr_sizes
Definition: skylarks_all.h:262
CfgInt cfg_NestlingMortProb
unsigned m_x2
Definition: PopulationManager.h:118
int st_PreparingForBreeding()
Definition: skylarks_all.cpp:5955
Definition: Treatment.h:149
int size
Definition: skylarks_all.h:301
TTypesOfSkState st_MakingNest()
Definition: skylarks_all.cpp:5992
Definition: Treatment.h:132
void TestNestPossibility()
Definition: skylarks_all.cpp:1261
int st_GivingUpTerritory()
Definition: skylarks_all.cpp:6166
Definition: Treatment.h:148
void OnMateDying()
Definition: skylarks_all.cpp:4140
bool value() const
Definition: Configurator.h:164
int IsValid(int nx, int ny) const
Definition: skylarks_all.cpp:1983
Definition: Treatment.h:67
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
double GetWeatherHindrance() const
Definition: skylarks_all.cpp:3549
bool IsGridPositionValid(const int &a_x, const int &a_y, int a_range) const
Definition: skylarks_all.cpp:1922
double size
Definition: skylarks_all.h:250
Definition: Treatment.h:42
Landscape * TheLandscape
Definition: skylarks_all.h:381
Definition: Treatment.h:65
Data structure to hold & output probe data probe data is designed to be used to return the number of ...
Definition: PopulationManager.h:421
CfgFloat cfg_MD_Threshold("SK_MD_THRESHOLD", CFG_CUSTOM, 25.8)
Skylark_Male * m_Dad
Definition: skylarks_all.h:595
Definition: Treatment.h:45
const double Breed_Res_Thresh2
Definition: skylarks_all.cpp:210
int m_Location_x
Definition: skylarks_all.h:275
Definition: Treatment.h:98
bool m_firstPF
Definition: skylarks_all.h:789
double XFNestAcceptScore
Definition: skylarks_all.cpp:224
void incNoChickDeaths()
Definition: skylarks_all.h:480
TTypesOfVegetation m_RefVeg[25]
Definition: PopulationManager.h:437
bool OpenTheBreedingPairsProbe() override
Definition: skylarks_all.cpp:817
double GetFood(int time)
Definition: skylarks_all.cpp:4898
double m_qual
Definition: skylarks_all.h:313
AOR_Probe * m_AOR_Probe
A pointer to the AOR probe.
Definition: PopulationManager.h:875
int CalculateEggNumber() const
Definition: skylarks_all.cpp:6493
void OnMateNeverComesBack(const Skylark_Female *AFemale)
Definition: skylarks_all.cpp:4357
const int June
Julian start dates of the month of June.
Definition: Landscape.h:48
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
bool Sex
Definition: skylarks_all.h:591
void incTotalNestlings()
Definition: skylarks_all.h:486
int m_MyHome
The vegetation type where the skylark was born.
Definition: skylarks_all.h:523
double Probe(const Probe_Data *p_TheProbe)
Definition: skylarks_all.h:118
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
CfgFloat cfg_MD_Threshold
int m_NoFledgeDeaths
Definition: skylarks_all.h:439
void DoFirst() override
Definition: skylarks_all.cpp:890
int Supply_x(int ref) const
Definition: skylarks_all.cpp:1358
CfgInt cfg_insecticide_direct_mortF("SK_INSECTICDEDIRECTMORTF", CFG_CUSTOM, 0)
virtual ~Skylark_struct()
Definition: skylarks_all.h:217
Definition: Treatment.h:77
int MinDegrees
Definition: skylarks_all.h:559
Definition: Treatment.h:96
int m_Location_y
Definition: skylarks_all.h:276
void OnBroodDesertion()
Definition: skylarks_all.cpp:4709
CfgFloat cfg_Skylark_prefledegling_NOEL
Used for determining the pesticide response for prefledglings.
Definition: skylarks_all.h:309
int HQualityTrack
Definition: skylarks_all.cpp:231
CfgInt cfg_PatchyPremium("SK_PATCHYPREMIUM", CFG_CUSTOM, 47)
Definition: Treatment.h:54
virtual void DoProbe(int a_lifestage)
Definition: AOR_Probe.cpp:104
Skylark_Female * Supply_F_Owner(int ref) const
Definition: skylarks_all.cpp:1386
Definition: Treatment.h:130
double RainHindrance[21]
Definition: skylarks_all.cpp:188
Definition: Treatment.h:97
Definition: Treatment.h:141
void EvaluateAllTerritories(void)
Definition: skylarks_all.cpp:1893
Skylark_Female(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:5017
double SupplyPesticide(int a_x, int a_y, PlantProtectionProducts a_ppp)
Gets total pesticide for a location.
Definition: Landscape.cpp:1386
void Occupy(int ref, Skylark_Male *Male) const
Definition: skylarks_all.cpp:2018
const double KcalPerGInsect_kg_inv
Definition: skylarks_all.cpp:177
int PolyRefData[2500]
Definition: skylarks_all.h:377
int F_Mig_Mort
Definition: skylarks_all.h:432
double m_GrNeed
Definition: skylarks_all.h:597
A struct defining two x,y coordinate sets of positive co-ords only.
Definition: PopulationManager.h:114
CfgInt cfg_fecundity_reduc_chance
int HQualityTallVeg
Definition: skylarks_all.cpp:241
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
int CalcFoodTime(double target) const
Definition: skylarks_all.cpp:6521
bool OpenTheFledgelingProbe() override
Definition: skylarks_all.cpp:860
void BreedingPairsOutput(int Time) override
Definition: skylarks_all.cpp:718
int SupplyFarmOwner(int a_x, int a_y)
Returns the farm owner pointer for the polygon referenced by a_polyref or a_x, a_y.
Definition: Landscape.h:1774
long Date(void)
Definition: Calendar.h:57
bool m_pesticide_sprayed_die
Definition: skylarks_all.h:719
bool OnFarmEvent(FarmToDo event) override
Must be reimplemented if used in descendent classes. Sets the action on a management event.
Definition: skylarks_all.cpp:5038
int HQualityVeg30cm
Definition: skylarks_all.cpp:237
CfgFloat cfg_ConversionEffReduc("SK_CONVEFFREDEC", CFG_CUSTOM, 0.023077)
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
CfgInt cfg_insecticide_direct_mortN("SK_INSECTICDEDIRECTMORTN", CFG_CUSTOM, 0)
int HQualityHedge
Definition: skylarks_all.cpp:235
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
CfgInt cfg_HQualityBareEarth("SK_HQBAREEARTH", CFG_CUSTOM, 3)
double DensityScore[111]
Definition: skylarks_all.cpp:192
static double MD_Threshold
Definition: skylarks_all.cpp:164
bool st_Immigrating()
Definition: skylarks_all.cpp:3995
Definition: Treatment.h:70
void FemaleOccupy(int ref, Skylark_Female *Female) const
Definition: skylarks_all.cpp:2022
A simple class defining an x,y coordinate set.
Definition: ALMaSS_Setup.h:52
int m_TotalEggs
Definition: skylarks_all.h:436
Definition: Treatment.h:46
CfgFloat cfg_Skylark_prefledegling_NOEL("SK_FLEDGE_NOEL", CFG_CUSTOM, 0.001)
Used for determining the pesticide response for prefledglings.
double FemaleNestAcceptScore
Definition: skylarks_all.cpp:223
void ReInit(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) override
Definition: skylarks_all.cpp:3617
CfgFloat cfg_Skylark_female_NOEL("SK_FEMALE_NOEL", CFG_CUSTOM, 0.001)
Can be used to trigger a response to pesticides for the females.
CfgFloat cfg_MaleSplitScale
CfgFloat cfg_MaleSplitScale("SK_MALESPLITSCALE", CFG_CUSTOM, 0.5)
int * m_poly_seen
Definition: skylarks_all.h:343
Skylark_Adult(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3488
CfgFloat cfg_Skylark_prefledegling_Biodegredation("SK_FLEDGE_BIODEG", CFG_CUSTOM, 0.0)
The proportion of pesticide remaining from one day to the next for prefledglings.
static double Cooling_Rate_Eggs
Definition: skylarks_all.cpp:162
CfgInt cfg_juvreturnmort("SK_JUVRETURNMORT", CFG_CUSTOM, 35)
Immigration mortality for juveniles.
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
CfgInt cfg_strigling_clutch
Bool configurator entry class.
Definition: Configurator.h:155
void CheckManagement()
Used to start a check for any management related effects at the objects current location.
Definition: PopulationManager.cpp:1591
const int terrsize[6]
Definition: skylarks_all.cpp:170
Skylark_Nestling(int x, int y, Skylark_Male *Daddy, Landscape *L, SkTerritories *Terrs, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:2617
Definition: skylarks_all.h:512
virtual void ReInit(Skylark_Female *Mum, SkTerritories *Terrs, Landscape *L, int NoEggs, int x, int y, int mh, Skylark_Population_Manager *SPM)
Definition: skylarks_all.cpp:2130
CfgInt cfg_heightconstant_c
int m_for_iter_x[1000]
Definition: skylarks_all.h:350
static bool DailyMortality(int mort)
Definition: skylarks_all.cpp:2092
int Age
Definition: skylarks_all.h:518
int Supply_m_Location_y() const
Returns the ALMaSS y-coordinate.
Definition: PopulationManager.h:243
int st_Hatching()
Definition: skylarks_all.cpp:2565
CfgFloat cfg_NestPlacementMinQual
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
CfgInt cfg_densityconstant_c
int st_Emigrating()
Definition: skylarks_all.cpp:4031
int m_y_div10
Definition: skylarks_all.h:265
int Supply_quality(int ref) const
Definition: skylarks_all.cpp:2014
void GetTerritoriesByDistance(int nx, int ny, vector< APoint > *alist) const
Definition: skylarks_all.cpp:1999
Definition: Treatment.h:111
int st_StoppingBreeding()
Definition: skylarks_all.cpp:6286
CfgInt cfg_strigling_nestling
double TerrHeterogeneity[1000]
Definition: skylarks_all.cpp:194
void DumpMapGraphics(const char *a_filename, Landscape *a_map) const
Definition: skylarks_all.cpp:1397
enum { sob_Clutch, sob_Nestling, sob_PreFledgeling, sob_Male, sob_Female } SkylarkObject
Definition: skylarks_all.h:41
void OnDadDead()
Definition: skylarks_all.cpp:3025
void SetM_Mig_Mort(const int m)
Definition: skylarks_all.h:464
int st_Finding_Territory()
Definition: skylarks_all.cpp:5631
Definition: Treatment.h:135
unsigned m_NoEleTypes
Definition: PopulationManager.h:434
CfgInt cfg_temphindpow("SK_TEMPHINDPOW", CFG_CUSTOM, 3)
Definition: Treatment.h:82
static double MeanExtractionRatePerMinute
Definition: skylarks_all.cpp:165
void StartDeveloping()
Definition: skylarks_all.h:575
bool SupplyVegPatchy(int a_polyref)
Returns whether the polygon referenced by a_polyref or a_x, a_y has a vegetation type designated as p...
Definition: Landscape.h:1004
skTTerritory(int x, int y, int TheSize, int TheQuality, int a_x_div10, int a_y_div10, int a_range_div10)
Definition: skylarks_all.cpp:1241
A class defining an animals position.
Definition: PopulationManager.h:169
Definition: Treatment.h:63
const string SimulationName
Definition: skylarks_all.cpp:48
void BreedingSuccessProbeOutput(double, int, int, int, int, int, int, int) override
Definition: skylarks_all.cpp:788
Definition: Treatment.h:62
void OnSetMyClutch(Skylark_Clutch *p_C)
Definition: skylarks_all.h:744
int EggCounter
Definition: skylarks_all.h:715
Skylark_Base(int x, int y, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:2058
Definition: Treatment.h:119
int SupplyNoTerritories() const
Definition: skylarks_all.cpp:1362
Definition: Treatment.h:72
double SupplyVegArea(int v)
Records all area vegetation types and transform it into a table.
Definition: Landscape.h:305
CfgFloat cfg_sk_triplength("SK_TRIPLENGTH", CFG_CUSTOM, 10.5)
Definition: Treatment.h:112
Definition: Treatment.h:137
Definition: Treatment.h:40
Definition: Treatment.h:53
TSkylarkList()
Definition: skylarks_all.h:114
int m_EM_fail
Definition: skylarks_all.h:592
bool * Grid
Definition: skylarks_all.h:331
void FledgelingProbeOutput(int Total, int Time) override
Definition: skylarks_all.cpp:843
double MaxFeedRain
Definition: skylarks_all.cpp:196
CfgInt cfg_insecticide_direct_mortN
const char * SupplyStateNames(int i) const
Definition: PopulationManager.h:738
int TheBreedingFemalesProbe(int ProbeNo) override
Definition: skylarks_all.cpp:668
bool st_Immigrating()
Definition: skylarks_all.cpp:5517
CfgFloat cfg_SK_hindrance_scale
Skylark_Clutch(Skylark_Female *Mum, SkTerritories *Terrs, Landscape *L, int NoEggs, int x, int y, int mh, Skylark_Population_Manager *SPM)
Definition: skylarks_all.cpp:2120
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
APoint SupplyNestPosition(const int ref) const
Definition: skylarks_all.h:406
Definition: Treatment.h:145
void ResetBreedingSuccess()
Definition: skylarks_all.cpp:5914
void EstablishTerritory()
Definition: skylarks_all.cpp:5718
int m_TotalNestlings
Definition: skylarks_all.h:437
Base class for all population managers for agent based models.
Definition: PopulationManager.h:645
CfgFloat cfg_ConversionEffReduc
rectangle m_Rect[16]
Definition: PopulationManager.h:433
Definition: Treatment.h:86
Definition: Treatment.h:49
SkTerritories * Terrs
Definition: skylarks_all.h:215
vector< double > m_InsectTable
Definition: skylarks_all.h:808
double GetWindPeriod(long a_date, unsigned int a_period)
Definition: Weather.cpp:697
~Skylark_Population_Manager(void) override
Definition: skylarks_all.cpp:527
CfgFloat cfg_NestLeavingWeight
CfgInt cfg_insecticide_direct_mortE
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
CfgFloat cfg_Skylark_male_NOEL("SK_MALE_NOEL", CFG_CUSTOM, 0.001)
Can be used to trigger a response to pesticides for the males.
int HQualityGood
Definition: skylarks_all.cpp:245
Definition: Treatment.h:95
Definition: Treatment.h:147
CfgFloat cfg_MeanHatchingWeight
const int terrnos[6]
Definition: skylarks_all.cpp:169
const int April
Julian start dates of the month of April.
Definition: Landscape.h:44
bool OnFarmEvent(FarmToDo event) override
Must be reimplemented if used in descendent classes. Sets the action on a management event.
Definition: skylarks_all.cpp:3640
int HQualityBareEarth
Definition: skylarks_all.cpp:242
Definition: Treatment.h:36
CfgBool cfg_CfgRipleysOutputUsed
bool m_nest_valid
Definition: skylarks_all.h:267
CfgInt cfg_HQualityOpenTallVeg
int BroodAge
Definition: skylarks_all.h:800
bool SupplyHasTramlines(int a_x, int a_y)
Returns whether the polygon referenced by a_polyref or a_x, a_y has a vegetation type with tramlines.
Definition: Landscape.h:1870
int y
Definition: skylarks_all.h:300
CfgInt cfg_rainhindpow("SK_RAINHINDPOW", CFG_CUSTOM, 4)
bool m_StepDone
Indicates whether the iterative step code is done for this timestep.
Definition: PopulationManager.h:133
Definition: skylarks_all.h:760
TTypesOfPesticide SupplyPesticideType(void)
Gets type of pesticide effect from a reference.
Definition: Landscape.h:788
int m_x
Definition: ALMaSS_Setup.h:55
CfgInt cfg_HQualityVeg30cm("SK_HQVEGTHIRTYCM", CFG_CUSTOM, 11)
Definition: Treatment.h:116
Skylark_Male(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3599
CfgFloat cfg_densityconstant_a
unsigned m_y2
Definition: PopulationManager.h:119
Definition: Treatment.h:93
bool Paired
Definition: skylarks_all.h:670
void Step(void) override
Step behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:3813
int SupplyYearNumber(void)
Passes a request on to the associated Calendar class function, returns m_simulationyear
Definition: Landscape.h:2287
Definition: Treatment.h:114
CfgInt cfg_Breed_Res_Thresh1("SK_BREED_RES_THRESH", CFG_CUSTOM, 600)
int m_BreedingAttempts
Definition: skylarks_all.h:716
CfgInt cfg_HQualityOpenTallVeg("SK_HQOOPENTALLVEG", CFG_CUSTOM, 16)
CfgFloat cfg_skylark_pesticide_globaleggshellreduction("SK_GLOBALPESTICIDEEGGSHELLREDUC", CFG_CUSTOM, 0.0)
The proportion of eggs assumed to crack from pesticide effects as a global effect.
double * m_qual_cache
Definition: skylarks_all.h:347
virtual double On_FoodSupply(double)
Definition: skylarks_all.h:529
void PreFillTerrPolyLists(skTTerritory *a_terr)
Definition: skylarks_all.cpp:1791
int Clutch_Size
Definition: skylarks_all.h:582
int value() const
Definition: Configurator.h:116
APoint SupplyNestLoc() const
Definition: skylarks_all.h:874
CfgFloat cfg_FemaleMinTerritoryAcceptScore
int SupplyM_Mig_Mort() const
Definition: skylarks_all.h:454
void EndStep(void) override
EndStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:3337
unsigned m_NoVegTypes
Definition: PopulationManager.h:435
double GetFledgelingEM(int Age) const
Definition: skylarks_all.cpp:3446
TTypesOfLandscapeElement m_EleType
Definition: PopulationManager.h:175
Probe_Data * TheProbe[100]
Holds a list of pointers to standard output probes.
Definition: PopulationManager.h:588
void ReInit(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) override
Definition: skylarks_all.cpp:5027
CfgFloat cfg_EM_Nestling_b("SK_EM_NESTLING_B", CFG_CUSTOM, 1.353)
int st_Flocking()
Definition: skylarks_all.cpp:3947
int m_TotalPrefledgelings
Definition: skylarks_all.h:438
CfgFloat cfg_tramline_foraging
int m_EggNumber
Definition: skylarks_all.h:718
Definition: Treatment.h:75
bool CompareDist(const APoint i, const APoint j)
Definition: skylarks_all.cpp:142
bool m_qual_cache_filled
Definition: skylarks_all.h:348
bool st_Arriving()
Definition: skylarks_all.cpp:5500
double EvaluateHabitatN(skTTerritory *a_terr)
Definition: skylarks_all.cpp:1895
Definition: Treatment.h:47
unsigned m_y
Definition: PopulationManager.h:174
bool OpenTheBreedingSuccessProbe() override
Definition: skylarks_all.cpp:830
const double EM_warmblood1
Definition: skylarks_all.cpp:181
int TheSkylarkTerrsSupply_x(int) const
Definition: skylarks_all.cpp:880
CfgFloat cfg_MeanExtractionRatePerMinute
Definition: LandscapeFarmingEnums.h:1067
Definition: Treatment.h:106
void OnEggHatch()
Definition: skylarks_all.cpp:4630
Definition: Treatment.h:109
CfgFloat cfg_densityconstant_b
int TheSkylarkTerrsSupply_y(int) const
Definition: skylarks_all.cpp:882
int HQualityHedgeScrub
Definition: skylarks_all.cpp:233
CfgFloat cfg_Skylark_nestling_Biodegredation("SK_NESTLING_BIODEG", CFG_CUSTOM, 0.0)
The proportion of pesticide accumulated from one day to the next.
int Supply_NestTime() const
Definition: skylarks_all.h:736
Definition: LandscapeFarmingEnums.h:610
Definition: Treatment.h:48
const double EM_coldblood2
Definition: skylarks_all.cpp:180
unsigned m_NoAreas
Definition: PopulationManager.h:432
CfgInt cfg_insecticide_direct_mortP("SK_INSECTICDEDIRECTMORTP", CFG_CUSTOM, 0)
bool OpenTheRipleysOutputProbe()
Definition: PopulationManager.cpp:897
CfgFloat cfg_Skylark_female_Biodegredation("SK_FEMALE_BIODEG", CFG_CUSTOM, 0.0)
The proportion of pesticide remaining from one day to the next for females.
int m_Born_x
Definition: skylarks_all.h:520
int PreEvaluateHabitatStripX(int a_x, int a_y, int a_range_x) const
Definition: skylarks_all.cpp:1619
void ClaimGrid(int x, int y, int range) const
Definition: skylarks_all.cpp:1971
std::string EventtypeToString(int a_event)
Returns the text representation of a treatment type.
Definition: Landscape.cpp:6024
static int PreFledgeMortProb
Definition: skylarks_all.cpp:160
virtual void CopyMyself()
Used to copy the object details to another in descendent classes.
Definition: PopulationManager.h:273
const double CE_nest[15]
Definition: skylarks_all.cpp:149
skTerritory_struct Supply_Territory() const
Definition: skylarks_all.cpp:4373
double GetFood(int time)
Definition: skylarks_all.cpp:6441
CfgFloat cfg_Skylark_male_Biodegredation("SK_MALE_BIODEG", CFG_CUSTOM, 0.0)
The proportion of pesticide remaining from one day to the next for males.
CfgFloat cfg_FemaleMinTerritoryAcceptScore("SK_MINFEMACCEPTSCORE", CFG_CUSTOM, 300000)
const char * StateNames[100]
Definition: PopulationManager.h:801
vector< APoint > * m_aTerrlist
Definition: skylarks_all.h:661
Definition: Treatment.h:146
virtual void CopyMyself(int a_sktype)
Definition: skylarks_all.cpp:6601
void PreProcessLandscape2(Landscape *L)
Definition: skylarks_all.cpp:1660
Skylark_Nestling * m_Brood[26]
Definition: skylarks_all.h:792
Definition: Treatment.h:134
unsigned m_RefFarms[25]
Definition: PopulationManager.h:439
void st_Dying()
Definition: skylarks_all.cpp:3922
CfgFloat cfg_heightconstant_a
int TheSkylarkTerrsSupply_size(int) const
Definition: skylarks_all.cpp:884
double SupplyInsects(int a_polyref)
Returns the insect biomass on a polygon using the polygon reference number a_polyref or based on the ...
Definition: Landscape.h:1712
double SupplyRain(void)
Passes a request on to the associated Weather class function, the amount of rain for the current day.
Definition: Landscape.h:1971
int m_Counter1
Definition: skylarks_all.h:711
int st_TempLeavingArea()
Definition: skylarks_all.cpp:4383
bool found
Definition: skylarks_all.h:202
void OnBroodDeath()
Definition: skylarks_all.cpp:5768
CfgFloat cfg_SkScrapesPremiumII("SK_SKSCRAPESPREMIUMNEST", CFG_CUSTOM, 0.24)
int GoodWeather
Definition: skylarks_all.h:656
Definition: Treatment.h:118
Definition: skylarks_all.h:326
const int May
Julian start dates of the month of May.
Definition: Landscape.h:46
vector< int > m_HabitatTable_PNum
Definition: skylarks_all.h:806
void BeginStep(void) override
BeingStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:2358
vector< int > m_hr_polys
Definition: skylarks_all.h:261
const int January
Julian start dates of the month of January.
Definition: Landscape.h:38
virtual void ReInit(int x, int y, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:2074
Definition: Treatment.h:113
CfgInt cfg_HQualityTall("SK_HQTALL", CFG_CUSTOM, -2)
class Weather * g_weather
Definition: Weather.cpp:49
void OnNestLocation(int x, int y)
Definition: skylarks_all.cpp:4717
bool OnFarmEvent(FarmToDo event) override
Must be reimplemented if used in descendent classes. Sets the action on a management event.
Definition: skylarks_all.cpp:2141
bool sex
Definition: skylarks_all.h:242
bool OpenTheReallyBigProbe()
Definition: PopulationManager.cpp:1005
CfgInt cfg_insecticide_direct_mortE("SK_INSECTICDEDIRECTMORTE", CFG_CUSTOM, 0)
double SupplyVegHeight(int a_polyref)
Returns the height of the vegetation using the polygon reference number a_polyref or based on the x,...
Definition: Landscape.h:1527
void OnBreedSuccess()
Definition: skylarks_all.h:754
void OnPreFledgelingDeath(const Skylark_PreFledgeling *P)
Definition: skylarks_all.cpp:4281
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
unsigned GetLiveArraySize(int a_listindex) override
Gets the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:657
void Catastrophe() override
Definition: skylarks_all.cpp:1156
double m_Size
Definition: skylarks_all.h:519
CfgFloat cfg_Cooling_Rate_Eggs
Maximum immigration mortality for females.
Definition: skylarks_all.h:248
double IsTramline
Definition: skylarks_all.cpp:221
virtual void ReinitialiseObject(int a_x, int a_y, Landscape *a_l_ptr)
Definition: PopulationManager.h:282
int TheFledgelingProbe() override
Definition: skylarks_all.cpp:644
const int HomeRangeDiameterDiv20
Definition: skylarks_all.cpp:186
double MyExtractEff
Definition: skylarks_all.h:658
double NotTramline
Definition: skylarks_all.cpp:222
static void OnArrayBoundsError()
Used for debugging only, tests basic object properties.
Definition: PopulationManager.cpp:1614
Definition: LandscapeFarmingEnums.h:1061
CfgInt cfg_NestLeavingChance
double GetTempPeriod(long a_date, unsigned int a_period)
Definition: Weather.cpp:707
int Size
Definition: skylarks_all.h:274
void Step(void) override
Step behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:2362
Definition: Treatment.h:41
int st_Floating()
Definition: skylarks_all.cpp:5614
int m_StriglingMort[4]
Definition: skylarks_all.h:435
unsigned m_x1
Definition: PopulationManager.h:116
Definition: skylarks_all.h:257
Integer configurator entry class.
Definition: Configurator.h:102
void PreEvaluateQualGrid(SkQualGrid *a_grid, int a_x, int a_y, int a_width, int a_height)
Definition: skylarks_all.cpp:1539
double Supply_TerritoryQual() const
Definition: skylarks_all.h:837
double m_VirtualDiameter
Definition: skylarks_all.h:291
int m_baddays
Definition: skylarks_all.h:560
FILE * SKPOM2
Definition: skylarks_all.h:445
void EndStep(void) override
EndStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:2887
Skylark_Population_Manager * m_OurPopulationManager
Definition: skylarks_all.h:526
double Resources
Definition: skylarks_all.h:714
Definition: Treatment.h:37
vector< int > m_sizes
Definition: skylarks_all.h:311
int st_CaringForYoung()
Definition: skylarks_all.cpp:4801
int HQualityTall
Definition: skylarks_all.cpp:229
CfgFloat cfg_ReturnProbability("SK_RETURNPROBABILITY", CFG_CUSTOM, 10000)
Used to change the timing of start of arrival in the landscape.
Skylark_Male * Supply_Owner(int ref) const
Definition: skylarks_all.cpp:1382
int y
Definition: skylarks_all.h:210
The base class for all farm types.
Definition: Farm.h:755
void WriteSKPOM2(const int n, const int n2) const
Definition: skylarks_all.h:452
int st_StartingNewBrood()
Definition: skylarks_all.cpp:6202
Definition: Treatment.h:79
void OnMateLeaving()
Definition: skylarks_all.cpp:4163
int st_EggHatching()
Definition: skylarks_all.cpp:6273
void FileOutput(int No, int time, int ProbeNo) const
Definition: PopulationManager.cpp:1626
CfgInt cfg_adultreturnmort("SK_ADULTRETURNMORT", CFG_CUSTOM, 35)
Immigration mortality for juveniles.
const int September
Julian start dates of the month of September.
Definition: Landscape.h:54
int m_MyMinTerritoryQual
Definition: skylarks_all.h:794
Definition: Treatment.h:74
SkQualGrid ** m_qual_grid
Definition: skylarks_all.h:339
void CreateObjects(int ob_type, TAnimal *pTAo, void *null, Skylark_struct *data, int number)
Definition: skylarks_all.cpp:539
Double configurator entry class.
Definition: Configurator.h:126
CfgInt cfg_fecundity_reduc
void OnClutchDeath()
Definition: skylarks_all.cpp:5786
CfgFloat cfg_Skylark_female_Biodegredation
The proportion of pesticide remaining from one day to the next for females.
void OnBreedingSuccess()
Definition: skylarks_all.cpp:5465
string m_SimulationName
stores the simulation name
Definition: PopulationManager.h:622
CfgBool cfg_skylark_pesticide_eggshellreduction_perclutch("SK_PESTICIDEEGGSHELLREDUCPERCLUTCH", CFG_CUSTOM, false)
If true egg shell reduction works at clutch level, if false it is considered per egg.
Definition: LandscapeFarmingEnums.h:65
vector< int > m_sizes
Definition: skylarks_all.h:260
void EndStep(void) override
EndStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:3910
int SupplySimW() const
Returns landscape width in m.
Definition: PopulationManager.h:567
const int March
Julian start dates of the month of March.
Definition: Landscape.h:42
int m_x_div10
Definition: skylarks_all.h:264
bool HaveTerritory
Definition: skylarks_all.h:813
Definition: Treatment.h:136
FILE * StriglingMort
Definition: skylarks_all.cpp:46
int m_BroodSize
Definition: skylarks_all.h:791
Definition: Treatment.h:108
virtual void TheRipleysOutputProbe(FILE *a_prb)
Definition: skylarks_all.cpp:762
virtual unsigned SupplyListIndexSize()
Definition: PopulationManager.h:725
int m_NoProbes
Definition: PopulationManager.h:787
Definition: Treatment.h:69
Definition: Treatment.h:105
CfgInt cfg_strigling_nestling("SK_STRIGLING_N", CFG_CUSTOM, 72)
int * m_poly_size
Definition: skylarks_all.h:344
CfgInt cfg_PreFledgeMortProb("SK_PREFLEDGE_MORT_PROB", CFG_CUSTOM, 50)
double GetVirtualDiameter() const
Definition: skylarks_all.h:271
CfgFloat cfg_Skylark_nestling_Biodegredation
The proportion of pesticide accumulated from one day to the next.
CfgInt cfg_SkTramlinesPremium
Skylark_Male * Dad
Definition: skylarks_all.h:233
CfgInt cfg_insecticide_direct_mortM
bool IsBadWeather() const
Definition: skylarks_all.h:494
unsigned m_NoFarms
Definition: PopulationManager.h:436
void OnNestlingDeath(const Skylark_Nestling *N)
Definition: skylarks_all.cpp:4665
int m_toowet
Definition: skylarks_all.h:720
Skylark_PreFledgeling(int x, int y, Landscape *L, SkTerritories *Terrs, Skylark_Male *Daddy, bool sex, double size, int age, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3113
CfgInt cfg_ClutchMortProb
void incNoPestEffects()
Definition: skylarks_all.h:482
CfgInt cfg_SkTramlinesPremium("SK_TRAMLINEPREMIUM", CFG_CUSTOM, 6)
CfgInt cfg_HQualityHedgeScrub
void OnDeserted()
Definition: skylarks_all.cpp:3048
Definition: Treatment.h:78
int st_Flocking()
Definition: skylarks_all.cpp:5473
Definition: skylarks_all.h:676
int SupplyBroodWeight(const int n) const
Definition: skylarks_all.h:839
CfgFloat cfg_MinDaysToHatch("SK_MINDAYSTOHATCH", CFG_CUSTOM, 10.2)
The time taken to egg hatch under optimal conditions.
Definition: skylarks_all.h:624
Definition: Configurator.h:70
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
static double NestLeavingWeight
Definition: skylarks_all.cpp:155
double NestPlacementMinQual
The minimum quality for nest placement.
Definition: skylarks_all.cpp:227
Definition: skylarks_all.h:207
Definition: Treatment.h:66
CfgFloat cfg_hindconstantH_b
Definition: Treatment.h:92
void TheAOROutputProbe() override
Definition: skylarks_all.cpp:759
~Skylark_Male() override
Definition: skylarks_all.cpp:3636
int TheBreedingSuccessProbe(int &BreedingFemales, int &YoungOfTheYear, int &TotalPop, int &TotalFemales, int &TotalMales, int &BreedingAttempts) override
Definition: skylarks_all.cpp:683
Definition: Treatment.h:102
int PreEvaluateHabitatStripY(int a_x, int a_y, int a_range_x) const
Definition: skylarks_all.cpp:1640
CfgFloat cfg_hindconstantD_b("SK_HINDCONSTD_B", CFG_CUSTOM,-0.022)
const double VeryHighDensityVeg
Definition: skylarks_all.cpp:195
double size
Definition: skylarks_all.h:240
Skylark_Male * MyMate
Definition: skylarks_all.h:724
void st_Dying() override
Definition: skylarks_all.cpp:3391
Definition: skylarks_all.h:238
int Supply_y(int ref) const
Definition: skylarks_all.cpp:1390
CfgFloat cfg_Skylark_nestling_NOEL("SK_NESTLING_NOEL", CFG_CUSTOM, 0.001)
Can be used to trigger a response to pesticides for the nestlings.
enum { toss_Initiation=0, toss_Developing, toss_Hatching, toss_CDying, toss_NDeveloping, toss_NMaturing, toss_NDying, toss_PDeveloping, toss_PMaturing, toss_PDying, toss_MFlocking, toss_MFloating, toss_MArriving, toss_MImmigrating, toss_MEmigrating, toss_MTempLeavingArea, toss_MFindingTerritory, toss_AttractingAMate, toss_FollowingMate, toss_ScaringOffChicks, toss_MCaringForYoung, toss_MDying, toss_MRehousing, toss_FFlocking, toss_FFloating, toss_FArriving, toss_FImmigrating, toss_FEmigrating, toss_FTempLeavingArea, toss_FFindingTerritory, toss_BuildingUpResources, toss_MakingNest, toss_PreparingForBreeding, toss_Laying, toss_StartingNewBrood, toss_EggHatching, toss_Incubating, toss_StoppingBreeding, toss_FCaringForYoung, toss_FDying, toss_GivingUpTerritory, toss_Destroy, } TTypesOfSkState
Definition: skylarks_all.h:92
CfgInt cfg_SkylarkFirstBreedingDate("SK_FIRSTBREEDINGDATE", CFG_CUSTOM, April)
The date at which breeding start can happen.
int mh
Definition: skylarks_all.h:213
int age
Definition: skylarks_all.h:243
bool InSquare(rectangle R) const
Definition: skylarks_all.cpp:2107
CfgInt cfg_HQualityNeutral("SK_HQNEUTRAL", CFG_CUSTOM, 0)
Definition: Treatment.h:57
CfgInt cfg_SkylarkFirstBreedingDate
The date at which breeding start can happen.
Definition: Treatment.h:87
const double EM_coldblood1
Definition: skylarks_all.cpp:179
CfgInt cfg_insecticide_direct_mortP
CfgInt cfg_HQualityBareEarth
void OnPreFledgelingMature(const Skylark_PreFledgeling *P)
Definition: skylarks_all.cpp:4319
CfgFloat cfg_EggTemp("SK_EGGTEMP", CFG_CUSTOM, 36.1)
int m_CurrentStateNo
The basic state number for all objects - '-1' indicates death.
Definition: PopulationManager.h:131
void PushIndividual(const unsigned a_listindex, TAnimal *a_individual_ptr)
Definition: PopulationManager.cpp:1682
void OnMateDying()
Definition: skylarks_all.cpp:5830
Definition: Treatment.h:101
skTTerritory * Territories[400000]
Definition: skylarks_all.h:328
int st_Developing() override
Definition: skylarks_all.cpp:3402
const double KcalPerGInsect
Definition: skylarks_all.cpp:175
const double Breed_Temp_Thresh
Definition: skylarks_all.cpp:211
Skylark_Female * Mum
Definition: skylarks_all.h:226
void OnEggsHatch()
Definition: skylarks_all.cpp:5808
CfgFloat cfg_MeanHatchingWeight("SK_MEAN_HATCHING_WEIGHT", CFG_CUSTOM, 3.23)
CfgFloat cfg_Skylark_male_NOEL
Can be used to trigger a response to pesticides for the males.
bool IsExtGridPositionValid(const int &x, const int &y, int range) const
Definition: skylarks_all.cpp:1942
~Skylark_Adult() override
Definition: skylarks_all.cpp:3517
double GetRain(long a_date)
Definition: Weather.h:506
Definition: Treatment.h:73
double nqual
Definition: skylarks_all.h:303
void SensibleCopy()
Definition: skylarks_all.cpp:6626
ofstream * ReallyBigOutputPrb
Definition: PopulationManager.h:873
void BeginStep(void) override
BeingStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:3782
void EndStep(void) override
EndStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:5446
CfgFloat cfg_densityconstant_a("SK_DENSITYCONST_A", CFG_CUSTOM, 0)
CfgFloat cfg_heightconstant_b("SK_HEIGHTCONST_B", CFG_CUSTOM,-0.22)
int SupplyLargestPolyNumUsed()
Returns m_LargestPolyNumUsed.
Definition: Landscape.h:578
Skylark_Female * Mother
Definition: skylarks_all.h:583
int HQualityTall2
Definition: skylarks_all.cpp:230
virtual void ReInit(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3502
Definition: Treatment.h:55
CfgFloat cfg_Skylark_male_Biodegredation
The proportion of pesticide remaining from one day to the next for males.
int HQualityWater
Definition: skylarks_all.cpp:236
double On_FoodSupply(double food) override
Definition: skylarks_all.cpp:3059
TTypesOfLandscapeElement m_RefEle[25]
Definition: PopulationManager.h:438
CfgFloat cfg_EM_Nestling_a
float ProbePOM(int ListIndex, const Probe_Data *p_TheProbe)
Modified probe for POM Output.
Definition: skylarks_all.cpp:1089
CfgInt cfg_HQualityTallVeg("SK_HQTALLVEG", CFG_CUSTOM, -10)
CfgInt cfg_HQualityNeutral
void OnMumGone()
Definition: skylarks_all.cpp:2342
double CheckForFields() const
Definition: skylarks_all.cpp:6577
CfgFloat cfg_MeanExtractionRatePerMinute("SK_EXTRACTION_RATE", CFG_CUSTOM, 0.0020948)
skTerritory_struct MyTerritory
Definition: skylarks_all.h:660
void FeedYoung()
Definition: skylarks_all.cpp:6344
void Warn(std::string a_msg1, std::string a_msg2)
Wrapper for the g_msg Warn function.
Definition: Landscape.h:2250
int m_nest_pos_validx
Definition: skylarks_all.h:268
int m_hash_size
Definition: skylarks_all.h:342
CfgBool cfg_skylark_pesticide_eggshellreduction_perclutch
If true egg shell reduction works at clutch level, if false it is considered per egg.
CfgFloat cfg_maxfeedrain("SK_MAXFEEDRAIN", CFG_CUSTOM, 10.0)
CfgInt cfg_SkStartNos
The number of skylarks that start in the simulation.
Definition: skylarks_all.h:423
double PrePolyNQual(int a_poly, int *a_good_polys)
Definition: skylarks_toletov.cpp:284
bool OnFarmEvent(FarmToDo event) override
Must be reimplemented if used in descendent classes. Sets the action on a management event.
Definition: skylarks_all.cpp:3137
Skylark_Male * Owner
Definition: skylarks_all.h:279
CfgInt cfg_strigling_preflg
int SupplyNoTerritories() const
Definition: skylarks_all.cpp:878
int No_HabitatTable_Refs
Definition: skylarks_all.h:793
Definition: Treatment.h:34
Skylark_Female * MyMate
Definition: skylarks_all.h:798
TTypesOfSkState st_Laying()
Definition: skylarks_all.cpp:6060
int m_for_iter_y[1000]
Definition: skylarks_all.h:351
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
CfgInt cfg_fecundity_reduc("SK_FECUNDITY_REDUC", CFG_CUSTOM, 0)
double m_MinFemaleAcceptScore
Definition: skylarks_all.h:721
int SimH
Definition: skylarks_all.h:330
const int HomeRangeDiameter
Definition: skylarks_all.cpp:185
int x
Definition: skylarks_all.h:299
CfgInt cfg_Breed_Res_Thresh1
CfgInt cfg_heightconstant_c("SK_HEIGHTCONST_C", CFG_CUSTOM, 38)
Skylark_Clutch * MyClutch
Definition: skylarks_all.h:710
Definition: skylarks_all.h:231
void ConstructAHabitatTable()
Definition: skylarks_all.cpp:4744
int st_CaringForYoung()
Definition: skylarks_all.cpp:6316
int EstablishingATerritory()
Definition: skylarks_all.cpp:4498
int SupplyDaylength(void)
Passes a request on to the associated Weather class function, the day length for the current day.
Definition: Landscape.h:2201
int SupplyPolyRef(int a_x, int a_y)
Get the in map polygon reference number from the x, y location.
Definition: Landscape.h:2157
CfgInt cfg_insecticide_direct_mortF
Definition: Treatment.h:58
CfgInt cfg_HQualityVeg30cm
Definition: Treatment.h:128
void OnAddPreFledgeling(Skylark_PreFledgeling *P, const Skylark_Nestling *N)
Definition: skylarks_all.cpp:4232
int bx
Definition: skylarks_all.h:211
virtual void st_Dying()
Definition: skylarks_all.cpp:3097
int m_NoChickDeaths
Definition: skylarks_all.h:440
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
int TheSkylarkTerrsSupply_quality(int) const
Definition: skylarks_all.cpp:886
int SupplyNoMaleOccupied() const
Definition: skylarks_all.cpp:1366
int m_y
Definition: ALMaSS_Setup.h:56
CfgFloat cfg_Skylark_prefledegling_Biodegredation
The proportion of pesticide remaining from one day to the next for prefledglings.
long SupplyGlobalDate(void)
Passes a request on to the associated Calendar class function, returns the simulation global date for...
Definition: Landscape.h:2292
void BeginStep(void) override
BeingStep behaviour - must be implemented in descendent classes.
Definition: skylarks_all.cpp:2852
Definition: Treatment.h:50
int m_range_div10
Definition: skylarks_all.h:266
void st_Maturing() override
Definition: skylarks_all.cpp:3457
CfgInt cfg_FoodTripsPerDay("SK_FOOD_TRIPS_PER_DAY", CFG_CUSTOM, 30)
Definition: Treatment.h:115
CfgInt cfg_NestlingMortProb("SK_NEST_MORT_PROB", CFG_CUSTOM, 23)
CfgInt cfg_HQualityHedgeScrub("SK_HQHEDGESCRUB", CFG_CUSTOM, 0)
void OnNestPredatation()
Definition: skylarks_all.cpp:4701
void st_Dying()
Definition: skylarks_all.cpp:5735
const int October
Julian start dates of the month of October.
Definition: Landscape.h:56
const double ExtraBroodHeat
Definition: skylarks_all.cpp:218
Definition: Treatment.h:33
int M_Mig_Mort
Definition: skylarks_all.h:431
int m_BreedingSuccess
Definition: skylarks_all.h:717
Definition: Treatment.h:107
TTypesOfVegetation m_VegType
Definition: PopulationManager.h:176
void OnMateHomeless()
Definition: skylarks_all.cpp:5856
bool st_Arriving() const
Definition: skylarks_all.cpp:3976
void IncLiveArraySize(int a_listindex)
Increments the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:665
bool BSuccess
Definition: skylarks_all.h:657