Loading [MathJax]/extensions/ams.js
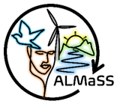 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
#include <skylarks_all.h>
|
| Skylark_Female (int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) |
|
void | ReInit (int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) override |
|
void | BeginStep (void) override |
| BeingStep behaviour - must be implemented in descendent classes. More...
|
|
void | Step (void) override |
| Step behaviour - must be implemented in descendent classes. More...
|
|
void | EndStep (void) override |
| EndStep behaviour - must be implemented in descendent classes. More...
|
|
void | EstablishTerritory () |
|
int | Supply_NestTime () const |
|
Skylark_Clutch * | SupplyMyClutch () const |
|
int | Supply_BreedingAttempts () const |
|
int | Supply_BreedingSuccess () const |
|
void | ResetBreedingSuccess () |
|
void | OnSetMyClutch (Skylark_Clutch *p_C) |
|
void | OnEggsHatch () |
|
void | OnClutchDeath () |
|
void | OnBroodDeath () |
|
void | OnBreedingSuccess () |
|
void | OnStopFeedingChicks () |
|
void | OnMateDying () |
|
void | OnMateHomeless () |
|
void | OnMaleNeverComesBack (const Skylark_Male *AMale) |
|
void | OnBreedSuccess () |
|
void | SensibleCopy () |
|
| Skylark_Adult (int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) |
|
| ~Skylark_Adult () override |
|
virtual void | CopyMyself (int a_sktype) |
|
| Skylark_Base (int x, int y, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) |
|
virtual void | ReInit (int x, int y, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh) |
|
virtual double | On_FoodSupply (double) |
|
void | AddStriglingMort (const int lifestage) const |
|
int | WhatState () override |
| Returns the objects current state number. More...
|
|
bool | InSquare (rectangle R) const |
|
| TAnimal (int x, int y, Landscape *L) |
| The TAnimal constructor saving the x,y, location and the landscape pointer. More...
|
|
| TAnimal (int x, int y) |
| The TAnimal constructor saving the x,y used if landscape is already set. More...
|
|
void | SetGuardMapIndex (int a_index_x, int a_index_y) |
| Set the guard map index, this is used to avoid two animals operating in the same location when using multithread. More...
|
|
unsigned | SupplyFarmOwnerRef () const |
| Get the current location farm ref if any. More...
|
|
AnimalPosition | SupplyPosition () const |
| Returns the objects location and habitat type and veg type. More...
|
|
APoint | SupplyPoint () const |
| Returns the objects location in ALMaSS coordinates. More...
|
|
int | SupplyPolygonRef () const |
| Returns the polygon reference where the object is located. More...
|
|
TTypesOfLandscapeElement | SupplyPolygonType () const |
| Returns the polygon type where the object is located. More...
|
|
int | Supply_m_Location_x () const |
| Returns the ALMaSS x-coordinate. More...
|
|
int | Supply_m_Location_y () const |
| Returns the ALMaSS y-coordinate. More...
|
|
int | SupplyGuardCellX () const |
| Returns the x-index to the guard cell. More...
|
|
int | SupplyGuardCellY () const |
| Returns the y-index to the guard cell. More...
|
|
int | SupplyAge () const |
| Returns the animals age in days. More...
|
|
void | SetAge (int a_age) |
| Sets the animals age in days. More...
|
|
virtual void | KillThis () |
| Sets all parameters ready for object destruction. More...
|
|
virtual void | CopyMyself () |
| Used to copy the object details to another in descendent classes. More...
|
|
void | SetX (const int a_x) |
| Sets the x-coordinate. More...
|
|
void | SetY (const int a_y) |
| Sets the y-coordinate. More...
|
|
virtual void | ReinitialiseObject (int a_x, int a_y, Landscape *a_l_ptr) |
|
virtual void | ReinitialiseObject (int a_x, int a_y) |
| Used to re-use an object - must be implemented in descendent classes. More...
|
|
virtual void | Dying () |
| A wrapped for KillThis - ideally should not be used. More...
|
|
void | CheckManagement () |
| Used to start a check for any management related effects at the objects current location. More...
|
|
void | CheckManagementXY (int a_x, int a_y) |
| Used to start a check for any management related effects at x,y. More...
|
|
int | GetCurrentStateNo () const |
| Returns the current state number. More...
|
|
void | SetCurrentStateNo (int a_num) |
| Sets the current state number. More...
|
|
bool | GetStepDone () const |
| Returns the step done indicator flag. More...
|
|
void | SetStepDone (bool a_bool) |
| Sets the step done indicator flag. More...
|
|
void | ReinitialiseObjectBase () |
| Used to initialise an object. More...
|
|
| TALMaSSObject () |
| The constructor for TALMaSSObject. More...
|
|
virtual | ~TALMaSSObject ()=default |
| The destructor for TALMaSSObject. More...
|
|
◆ Skylark_Female()
5018 :
Skylark_Adult(x, y, size, age, Terrs, L, SPM, bx, by, mh) {
References m_BreedingAttempts, m_MinFemaleAcceptScore, MyClutch, MyMate, and ResetBreedingSuccess().
◆ BeginStep()
void Skylark_Female::BeginStep |
( |
void |
| ) |
|
|
overridevirtual |
◆ CalcFoodTime()
int Skylark_Female::CalcFoodTime |
( |
double |
target | ) |
const |
|
protected |
6531 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6534 double FoodGathered = 0.0;
6539 while (FoodGathered < target && used < MyMate->SupplyNoHabitatRefs())
6544 if (available < target - FoodGathered)
6546 FoodGathered += available;
6557 FoodTime += static_cast<int>(floor(0.5 + (target - FoodGathered) / FoodPerMin));
References g_msg, MapErrorMsg::Warn(), and WARN_BUG.
Referenced by st_Incubating().
◆ CalculateEggNumber()
int Skylark_Female::CalculateEggNumber |
( |
| ) |
const |
|
protected |
6495 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6504 if (chance < 9) eggs = 2;
6505 else if (chance < 37 + 9) eggs = 3;
6506 else if (chance < 99 + 37 + 9) eggs = 4;
6511 if (chance < 29) eggs = 3;
6512 else if (chance < 29 + 80) eggs = 4;
6516 if (eggs < 1) eggs = 1;
References g_random_fnc(), and m_BreedingAttempts.
Referenced by st_Laying().
◆ CheckForFields()
double Skylark_Female::CheckForFields |
( |
| ) |
const |
|
protected |
6579 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6584 int TallFieldVeg = 0;
References Landscape::CorrectCoords(), TAnimal::m_OurLandscape, Skylark_Adult::MyTerritory, skTerritory_struct::size, Landscape::SupplyElementType(), Landscape::SupplyPolyRef(), Landscape::SupplyVegHeight(), tole_Field, tole_UnsprayedFieldMargin, skTerritory_struct::x, and skTerritory_struct::y.
◆ EndStep()
void Skylark_Female::EndStep |
( |
void |
| ) |
|
|
overridevirtual |
EndStep behaviour - must be implemented in descendent classes.
Reimplemented from TALMaSSObject.
5448 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5451 #ifdef __PESTICIDE_RA
5452 PesticideResponse();
◆ EstablishTerritory()
void Skylark_Female::EstablishTerritory |
( |
| ) |
|
5720 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
References Skylark_Adult::BSuccess, SkTerritories::FemaleOccupy(), TAnimal::m_Location_x, TAnimal::m_Location_y, Skylark_Base::m_OurPopulationManager, Skylark_Base::m_OurTerritories, MyMate, Skylark_Adult::MyTerritory, Skylark_Male::OnPairing(), Skylark_Adult::Paired, skTerritory_struct::ref, Resources, skTerritory_struct::size, Skylark_Male::Supply_Territory(), Population_Manager_Base::SupplySimH(), Population_Manager_Base::SupplySimW(), skTerritory_struct::x, and skTerritory_struct::y.
Referenced by Skylark_Male::OnReHouse(), and st_Finding_Territory().
◆ FeedYoung()
void Skylark_Female::FeedYoung |
( |
| ) |
|
|
protected |
Can only get food in daylight - dawn & dusk. Must keep them warm if the weather is bad - the quick version below catagorizes this into good or bad weather
6346 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6359 int FeedingProbs[16];
6360 double ChickFood[16];
6361 #ifdef __PESTICIDE_RA_CHICK
6362 double ChickPoison[ 16 ];
6365 const double resources_all =
GetFood(food_time );
6367 double resources =
RemoveEM(resources_all);
6368 int HungryChicks = BrSize;
6372 for (
int i = 0; i < HungryChicks; i++)
6374 FeedingProbs[i] = 0;
6376 #ifdef __PESTICIDE_RA_CHICK
6377 ChickPoison[ i ] = 0;
6383 int BroodWeight = 0;
6384 for (
int i = 0; i < HungryChicks; i++)
6388 BroodWeight += weight;
6390 for (
int j = i; j < HungryChicks; j++) FeedingProbs[j] += static_cast<int>(floor(0.5 + weight));
6392 if (
MyMate->
SupplyBroodAge(0) < 6) { resources -= pow(BroodWeight, 0.613) * 0.0719 * (35 - TempToday); }
6396 #ifdef __PESTICIDE_RA_CHICK
6397 double pcide_remain = resources * m_pcide_conc;
6399 while (resources > 0.01 && HungryChicks > 0)
6405 for (
int i = 0; i < BrSize; i++)
6407 if (choose < FeedingProbs[i])
6409 ChickFood[i] += resources;
6410 #ifdef __PESTICIDE_RA_CHICK
6411 ChickPoison[ i ] += pcide_remain;
6421 for (
int i = 0; i < BrSize; i++)
6423 #ifdef __PESTICIDE_RA_CHICK
6430 FeedingProbs[i] = -1;
References FoodTripsPerDay, g_random_fnc(), GetFood(), m_NestTime, TAnimal::m_OurLandscape, MaxFeedRain, MyMate, Skylark_Male::OnFoodMessage(), Skylark_Adult::RemoveEM(), Skylark_Male::SupplyBroodAge(), Skylark_Male::SupplyBroodSize(), Skylark_Male::SupplyBroodWeight(), Landscape::SupplyDaylength(), Landscape::SupplyRain(), and Landscape::SupplyTemp().
Referenced by st_CaringForYoung(), and st_GivingUpTerritory().
◆ GetFood()
double Skylark_Female::GetFood |
( |
int |
time | ) |
|
|
protected |
◆ GetMigrationMortality()
int Skylark_Female::GetMigrationMortality |
( |
| ) |
|
|
protected |
◆ OnBreedingSuccess()
void Skylark_Female::OnBreedingSuccess |
( |
| ) |
|
◆ OnBreedSuccess()
void Skylark_Female::OnBreedSuccess |
( |
| ) |
|
|
inline |
◆ OnBroodDeath()
void Skylark_Female::OnBroodDeath |
( |
| ) |
|
◆ OnClutchDeath()
void Skylark_Female::OnClutchDeath |
( |
| ) |
|
◆ OnEggsHatch()
void Skylark_Female::OnEggsHatch |
( |
| ) |
|
◆ OnFarmEvent()
bool Skylark_Female::OnFarmEvent |
( |
FarmToDo |
| ) |
|
|
overrideprotectedvirtual |
Must be reimplemented if used in descendent classes. Sets the action on a management event.
Reimplemented from TAnimal.
5180 #ifdef TEST_ISSUE_DEATH_WARRANT
5181 printf(
"Skylark_Female::OnFarmEvent() : %d : %d\n", event, (
int )
g_date->
Date() );
5188 #ifndef __NoPigsOutEffect
References Skylark_Base::AddStriglingMort(), autumn_harrow, autumn_or_spring_plough, autumn_plough, autumn_roll, autumn_sow, autumn_sow_with_ferti, bed_forming, biocide, bulb_harvest, burn_straw_stubble, burn_top, cattle_out, cattle_out_low, cfg_insecticide_direct_mortF, cut_to_hay, cut_to_silage, cut_weeds, Calendar::Date(), deep_ploughing, Landscape::EventtypeToString(), fa_ammoniumsulphate, fa_boron, fa_calcium, fa_cu, fa_greenmanure, fa_k, fa_manganesesulphate, fa_manure, fa_n, fa_nk, fa_npk, fa_npks, fa_p, fa_pk, fa_pks, fa_rsm, fa_sk, fa_sludge, fa_slurry, fiber_covering, fiber_removal, flammebehandling, flower_cutting, fp_ammoniumsulphate, fp_boron, fp_calcium, fp_cu, fp_greenmanure, fp_k, fp_liquidNH3, fp_manganesesulphate, fp_manure, fp_n, fp_nc, fp_nk, fp_npk, fp_npks, fp_ns, fp_p, fp_pk, fp_pks, fp_rsm, fp_sk, fp_sludge, fp_slurry, fungicide_treat, g_date, g_land, g_random_fnc(), glyphosate, green_harvest, growth_regulator, harvest, harvest_bushfruit, harvestshoots, hay_bailing, hay_turning, heavy_cultivator_aggregate, herbicide_treat, hilling_up, insecticide_treat, last_treatment, Skylark_Base::m_CurrentSkState, TAnimal::m_OurLandscape, manual_weeding, molluscicide, mow, org_fungicide, org_herbicide, org_insecticide, Skylark_Adult::Paired, pheromone, pigs_out, preseeding_cultivator, preseeding_cultivator_sow, product_treat, pruning, row_cultivation, shallow_harrow, shredding, sleep_all_day, spring_harrow, spring_plough, spring_roll, spring_sow, spring_sow_with_ferti, straw_chopping, straw_covering, straw_removal, strigling, strigling_hill, strigling_sow, stubble_cultivator_heavy, stubble_harrowing, stubble_plough, suckering, summer_harrow, summer_plough, summer_sow, swathing, syninsecticide_treat, trial_control, trial_insecticidetreat, trial_toxiccontrol, CfgInt::value(), Landscape::Warn(), water, winter_harrow, and winter_plough.
◆ OnMaleNeverComesBack()
void Skylark_Female::OnMaleNeverComesBack |
( |
const Skylark_Male * |
AMale | ) |
|
◆ OnMateDying()
void Skylark_Female::OnMateDying |
( |
| ) |
|
5832 if ( IsAlive() != 0xDEADC0DE )
References g_stopdate, Skylark_Base::m_CurrentSkState, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, Skylark_Base::m_OurTerritories, MyClutch, MyMate, Skylark_Adult::MyTerritory, Skylark_Clutch::OnMumGone(), skTerritory_struct::ref, SkTerritories::RemoveFemale(), Landscape::SupplyDayInYear(), and Skylark_Population_Manager::WriteSKPOM1().
Referenced by Skylark_Male::st_Dying().
◆ OnMateHomeless()
void Skylark_Female::OnMateHomeless |
( |
| ) |
|
5858 if ( IsAlive() != 0xDEADC0DE )
References Skylark_Adult::BSuccess, g_stopdate, Skylark_Base::m_CurrentSkState, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, MyClutch, MyMate, Skylark_Adult::MyTerritory, Skylark_Clutch::OnMumGone(), Skylark_Adult::Paired, skTerritory_struct::ref, Landscape::SupplyDayInYear(), and Skylark_Population_Manager::WriteSKPOM1().
Referenced by Skylark_Male::OnReHouse().
◆ OnSetMyClutch()
◆ OnStopFeedingChicks()
void Skylark_Female::OnStopFeedingChicks |
( |
| ) |
|
◆ ReInit()
◆ ResetBreedingSuccess()
void Skylark_Female::ResetBreedingSuccess |
( |
| ) |
|
◆ SensibleCopy()
void Skylark_Female::SensibleCopy |
( |
| ) |
|
◆ Spiral()
◆ Spiral2()
◆ st_Arriving()
bool Skylark_Female::st_Arriving |
( |
| ) |
|
|
protected |
◆ st_BuildingUpResources()
int Skylark_Female::st_BuildingUpResources |
( |
| ) |
|
|
protected |
The female forages from her home range each day. On 1st April she will make a transition to preparing for breeding which determines the time needed for egg production and nest building.
5930 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5945 if (today >=
May + 14)
return 2;
References Breed_Temp_Thresh, cfg_SkylarkFirstBreedingDate, EggCounter, g_stopdate, Skylark_Adult::GetBadWeather(), m_MinFemaleAcceptScore, TAnimal::m_OurLandscape, May, MyMate, Skylark_Male::Supply_TerritoryQual(), Landscape::SupplyDayInYear(), Landscape::SupplyTemp(), and CfgInt::value().
Referenced by Step().
◆ st_CaringForYoung()
int Skylark_Female::st_CaringForYoung |
( |
| ) |
|
|
protected |
Calls Skylark_Female::FeedYoung to get food and give it to the chicks. Once the chicks reach 18 days of age if more breeding is possible then the bird will start a new brood unless it is late in the season or there have been too many breeding attempts in which case she will stop breeding when the chicks are 30 days old. In previous versions there was a bad weather component, but this has been removed since it did not contribute to the POM fit. If future analysis finds a relationship it should be incorporated here.
6325 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6333 if (m_BreedingAttempts < 4 && m_OurLandscape->SupplyDayInYear() <
g_stopdate)
6338 if (ch_age == 30)
return 2;
References FeedYoung(), g_stopdate, MyMate, NestLoc, and Skylark_Male::SupplyBroodAge().
Referenced by BeginStep().
◆ st_Dying()
void Skylark_Female::st_Dying |
( |
void |
| ) |
|
|
protected |
5737 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5743 g_land->
Warn(
"Skylark_Female::st_Dying - Debug1 ",
nullptr);
References g_land, Skylark_Base::m_CurrentSkState, TALMaSSObject::m_CurrentStateNo, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, Skylark_Base::m_OurTerritories, MyClutch, MyMate, Skylark_Male::MyMate, Skylark_Adult::MyTerritory, Skylark_Male::OnMateDying(), Skylark_Clutch::OnMumGone(), skTerritory_struct::ref, SkTerritories::RemoveFemale(), Landscape::SupplyDayInYear(), Landscape::Warn(), and Skylark_Population_Manager::WriteSKPOM1().
Referenced by Step().
◆ st_EggHatching()
int Skylark_Female::st_EggHatching |
( |
| ) |
|
|
protected |
A transition is made to Care for Young.
6278 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
References m_toowet.
Referenced by Step().
◆ st_Emigrating()
int Skylark_Female::st_Emigrating |
( |
| ) |
|
|
protected |
Is called once a day. This state determines the return to breeding areas based on probabilities that alter with date. Once suitable conditions prevail there will be a transition to st_Immigrating.
5558 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5560 #ifdef __PESTICIDE_RA_ADULT
5578 if (chance < 300 && today >=
March + 15)
return 1;
5579 if (today >=
April + 15)
return 1;
5583 if (chance < 7 && today >=
January + 14)
return 1;
5584 if (chance < 33 && today >=
February + 14)
return 1;
5585 if (chance < 400 && today >=
March + 14)
return 1;
5586 if (chance < 1000 && today >=
April + 14)
return 1;
5587 if (today >
May)
return 1;
References Skylark_Base::Age, April, cfg_ReturnProbability, February, g_random_fnc(), g_stopdate, Skylark_Adult::GoodWeather, January, TAnimal::m_OurLandscape, Skylark_Adult::m_pesticide_affected, March, May, Landscape::SupplyDayInYear(), Landscape::SupplyTemp(), and CfgFloat::value().
Referenced by Step().
◆ st_Finding_Territory()
int Skylark_Female::st_Finding_Territory |
( |
| ) |
|
|
protected |
5633 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5647 score = testTerr.
nqual;
5667 for (
int i = 0; i < NoTerrs; i++)
5671 if (A_Male !=
nullptr)
5678 g_msg->
Warn(
WARN_BUG,
"Skylark_Female::st_Finding_Territory(): Male already paired!",
"" );
5702 #ifdef FOR_DEMONSTRATION
References Skylark_Adult::BSuccess, EstablishTerritory(), g_msg, Skylark_Adult::GetBadWeather(), Skylark_Male::HaveTerritory, TAnimal::m_Location_x, TAnimal::m_Location_y, m_MinFemaleAcceptScore, Skylark_Base::m_OurTerritories, MyMate, skTerritory_struct::nqual, Skylark_Male::OnMateNeverComesBack(), Skylark_Adult::Paired, SkTerritories::Supply_F_Owner(), SkTerritories::Supply_Owner(), Skylark_Male::Supply_Territory(), SkTerritories::SupplyNoTerritories(), MapErrorMsg::Warn(), and WARN_BUG.
Referenced by Step().
◆ st_Floating()
int Skylark_Female::st_Floating |
( |
| ) |
|
|
protected |
◆ st_Flocking()
int Skylark_Female::st_Flocking |
( |
| ) |
|
|
protected |
◆ st_GivingUpTerritory()
int Skylark_Female::st_GivingUpTerritory |
( |
| ) |
|
|
protected |
6168 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
References Skylark_Adult::BSuccess, EggCounter, FeedYoung(), TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, Skylark_Base::m_OurTerritories, MyClutch, MyMate, Skylark_Adult::MyTerritory, NestLoc, Skylark_Male::OnMateLeaving(), Skylark_Clutch::OnMumGone(), Skylark_Adult::Paired, skTerritory_struct::ref, SkTerritories::RemoveFemale(), Skylark_Male::SupplyBroodSize(), Landscape::SupplyDayInYear(), and Skylark_Population_Manager::WriteSKPOM1().
Referenced by Step().
◆ st_Immigrating()
bool Skylark_Female::st_Immigrating |
( |
| ) |
|
|
protected |
An instantaneous state which determines the chanve of migration mortality. If she does die then she has to inform any old mate that she has gone. If he is already paired with another bird then can just forget him. If not dying then breeding success for this year is reset and the bird transitions to st_Arrival.
5524 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
References Skylark_Base::Age, cfg_FemaleMinTerritoryAcceptScore, g_random_fnc(), GetMigrationMortality(), m_BreedingAttempts, m_MinFemaleAcceptScore, Skylark_Adult::m_pesticide_affected, MyClutch, MyMate, Skylark_Male::OnMateNeverComesBack(), Skylark_Adult::Paired, ResetBreedingSuccess(), and CfgFloat::value().
Referenced by Step().
◆ st_Incubating()
int Skylark_Female::st_Incubating |
( |
| ) |
|
|
protected |
Incubation occurs as described in development below. The female spends that time off the nest required to find energy to cover her basal metabolic requirements, plus that energy required to warm the eggs. Incubation continues until the eggs hatch and there is a transition to Care For Young, or the incubation period (MID) is exceeded, at which point the female will Start New Brood. This state can only be left by a call to OnEggHatch being altered on creation of a nestling or to StartNewBrood
6239 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6264 if (foodtime > Daylength) foodtime = Daylength;
References CalcFoodTime(), m_Counter1, m_NestTime, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, m_toowet, MaxFeedRain, Landscape::SupplyDaylength(), Skylark_Population_Manager::SupplyEMi(), and Landscape::SupplyRain().
Referenced by Step().
◆ st_Laying()
6067 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6077 #ifdef __PESTICIDE_RA_ADULT
6102 return toss_PreparingForBreeding;
6125 return toss_PreparingForBreeding;
6136 #ifdef FOR_DEMONSTRATION
6159 return toss_Incubating;
References Skylark_Clutch::AddEgg(), CalculateEggNumber(), cfg_skylark_pesticide_eggshellreduction, cfg_skylark_pesticide_eggshellreduction_perclutch, cfg_skylark_pesticide_globaleggshellreduction, Skylark_Population_Manager::CreateObjects(), g_msg, g_rand_uni_fnc(), Skylark_struct::L, m_BreedingAttempts, m_Counter1, m_EggNumber, TAnimal::m_Location_x, TAnimal::m_Location_y, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, Skylark_Base::m_OurTerritories, Skylark_Adult::m_pesticide_affected, MyClutch, MyMate, Skylark_Clutch::StartDeveloping(), Landscape::SupplyDayInYear(), Landscape::SupplyPesticideType(), Population_Manager_Base::SupplySimH(), Population_Manager_Base::SupplySimW(), Landscape::SupplyVegType(), Landscape::SupplyYearNumber(), ttop_eggshellthinning, ttop_ReproductiveEffects, CfgFloat::value(), CfgBool::value(), MapErrorMsg::Warn(), and WARN_BUG.
Referenced by Step().
◆ st_MakingNest()
Tests for the necessary territory quality, if OK finds a nest location and builds the nest. Nest attempts are assumed to be breeding attempts.
5997 #ifdef __PESTICIDE_RA_ADULT
6004 return toss_PreparingForBreeding;
6025 return toss_GivingUpTerritory;
6027 return toss_MakingNest;
6042 if (today >=
May + 14)
6044 return toss_GivingUpTerritory;
6046 return toss_MakingNest;
6054 if (
m_Counter1-- > 0)
return toss_MakingNest;
References g_msg, June, m_BreedingSuccess, m_Counter1, TAnimal::m_Location_x, TAnimal::m_Location_y, m_MinFemaleAcceptScore, TAnimal::m_OurLandscape, Skylark_Adult::m_pesticide_affected, APoint::m_x, APoint::m_y, May, MyMate, NestLoc, Skylark_Male::OnNestLocation(), Skylark_Male::Supply_TerritoryQual(), Landscape::SupplyDayInYear(), Skylark_Male::SupplyNestLoc(), Skylark_Male::SupplyNestValid(), MapErrorMsg::Warn(), and WARN_BUG.
Referenced by Step().
◆ st_PreparingForBreeding()
int Skylark_Female::st_PreparingForBreeding |
( |
| ) |
|
|
protected |
Builds up resources whilst waiting for good enough weather to begin nest building. Transitions to MakingNest or GiveUpTerritory (if too late in the season).
5961 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5977 if (t < 5.0)
return 0;
5979 if (t < 5.0)
return 0;
5981 if (t < 5.0)
return 0;
References EggCounter, g_stopdate, GetFood(), m_BreedingAttempts, m_Counter1, TAnimal::m_OurLandscape, Skylark_Adult::RemoveEM(), Resources, Landscape::SupplyDayInYear(), Landscape::SupplyDaylength(), Landscape::SupplyGlobalDate(), Landscape::SupplySnowcover(), and Landscape::SupplyTemp().
Referenced by Step().
◆ st_StartingNewBrood()
int Skylark_Female::st_StartingNewBrood |
( |
| ) |
|
|
protected |
Any current nests or clutches are removed. The female assesses the habitat quality of the territory. If still suitable (i.e. above MTQ) she will make a transition to Make Nest, otherwise she will go to Give Up Territory.
6208 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
6224 if (m_BreedingAttempts < 4 && m_OurLandscape->SupplyDayInYear() <
g_stopdate)
References g_stopdate, m_BreedingAttempts, m_Counter1, m_MinFemaleAcceptScore, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, m_toowet, MyClutch, MyMate, NestLoc, Skylark_Clutch::OnMumGone(), st_StoppingBreeding(), Skylark_Male::Supply_TerritoryQual(), Landscape::SupplyDayInYear(), and Skylark_Population_Manager::WriteSKPOM1().
Referenced by Step().
◆ st_StoppingBreeding()
int Skylark_Female::st_StoppingBreeding |
( |
| ) |
|
|
protected |
Called when the female stops breeding for the year. Removes the pair bond and tell the male she is leaving. She deregisters her territory ownership and if there has been no breeding success then she forgets the male.
6292 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
References Skylark_Adult::BSuccess, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, Skylark_Base::m_OurTerritories, MyClutch, MyMate, Skylark_Adult::MyTerritory, Skylark_Male::OnMateLeaving(), Skylark_Clutch::OnMumGone(), Skylark_Adult::Paired, skTerritory_struct::ref, SkTerritories::RemoveFemale(), Landscape::SupplyDayInYear(), and Skylark_Population_Manager::WriteSKPOM1().
Referenced by st_StartingNewBrood(), and Step().
◆ st_TempLeavingArea()
int Skylark_Female::st_TempLeavingArea |
( |
| ) |
|
|
protected |
◆ Step()
void Skylark_Female::Step |
( |
void |
| ) |
|
|
overridevirtual |
Step behaviour - must be implemented in descendent classes.
Reimplemented from TALMaSSObject.
5260 if ( IsAlive() != 0x0DEADC0DE ) DEADCODEError();
5266 g_land->
Warn(
"Skylark_Female::Step: DeBug5 ", NULL ); exit( 1 );
5272 case toss_Initiation:
5275 case toss_FFlocking:
5287 case toss_FFloating:
5290 case toss_FArriving:
5295 case toss_FImmigrating:
5299 #ifdef TEST_ISSUE_DEATH_WARRANT
5300 printf(
"Skylark_Female::Step() : st_Immigrating() : %d\n", (
int )
g_date->
Date() );
5305 case toss_FEmigrating:
5309 case toss_FTempLeavingArea:
5313 case toss_FFindingTerritory:
5320 g_land->
Warn(
"Skylark_Female::Step: !Paired ", NULL ); exit( 1 );
5334 case toss_BuildingUpResources:
5337 g_land->
Warn(
"Skylark_Female::Step: !Paired ", NULL ); exit( 1 );
5351 case toss_MakingNest:
5355 case toss_PreparingForBreeding:
5358 g_land->
Warn(
"Skylark_Female::Step: !Paired ", NULL ); exit( 1 );
5380 case toss_StartingNewBrood:
5395 case toss_EggHatching:
5399 case toss_Incubating:
5404 g_land->
Warn(
"Skylark_Female::Step: EggHatching ",
nullptr);
5410 g_land->
Warn(
"Skylark_Female::Step: Illegal Return Value ",
nullptr);
5423 case toss_StoppingBreeding:
5433 case toss_GivingUpTerritory:
5439 g_land->
Warn(
"Skylark_Female::Step: Unknown State", st.c_str());
References Calendar::Date(), g_date, g_land, Skylark_Base::m_CurrentSkState, TAnimal::m_Location_x, TAnimal::m_Location_y, TAnimal::m_OurLandscape, Skylark_Base::m_OurPopulationManager, TALMaSSObject::m_StepDone, m_toowet, Skylark_Clutch::Mother, MyClutch, NestLoc, Skylark_Clutch::OnMumGone(), Skylark_Adult::Paired, st_Arriving(), st_BuildingUpResources(), st_Dying(), st_EggHatching(), st_Emigrating(), st_Finding_Territory(), st_Flocking(), st_GivingUpTerritory(), st_Immigrating(), st_Incubating(), st_Laying(), st_MakingNest(), st_PreparingForBreeding(), st_StartingNewBrood(), st_StoppingBreeding(), st_TempLeavingArea(), Landscape::SupplyDayInYear(), Population_Manager::SupplyStateNames(), Landscape::Warn(), and Skylark_Population_Manager::WriteSKPOM1().
◆ Supply_BreedingAttempts()
int Skylark_Female::Supply_BreedingAttempts |
( |
| ) |
const |
◆ Supply_BreedingSuccess()
int Skylark_Female::Supply_BreedingSuccess |
( |
| ) |
const |
◆ Supply_NestTime()
int Skylark_Female::Supply_NestTime |
( |
| ) |
const |
|
inline |
◆ SupplyMyClutch()
◆ EggCounter
int Skylark_Female::EggCounter |
|
protected |
◆ m_BreedingAttempts
int Skylark_Female::m_BreedingAttempts |
|
protected |
◆ m_BreedingSuccess
int Skylark_Female::m_BreedingSuccess |
|
protected |
◆ m_Counter1
int Skylark_Female::m_Counter1 |
|
protected |
◆ m_EggNumber
int Skylark_Female::m_EggNumber |
|
protected |
◆ m_MinFemaleAcceptScore
double Skylark_Female::m_MinFemaleAcceptScore |
|
protected |
◆ m_NestTime
int Skylark_Female::m_NestTime |
|
protected |
◆ m_pesticide_sprayed_die
bool Skylark_Female::m_pesticide_sprayed_die |
|
protected |
◆ m_toowet
int Skylark_Female::m_toowet |
|
protected |
◆ MyClutch
Referenced by OnBroodDeath(), OnClutchDeath(), OnEggsHatch(), OnMateDying(), OnMateHomeless(), ReInit(), Skylark_Female(), st_Dying(), st_GivingUpTerritory(), st_Immigrating(), st_Laying(), st_StartingNewBrood(), st_StoppingBreeding(), and Step().
◆ MyMate
Referenced by EstablishTerritory(), FeedYoung(), GetFood(), OnEggsHatch(), OnMaleNeverComesBack(), OnMateDying(), OnMateHomeless(), ReInit(), Skylark_Female(), st_BuildingUpResources(), st_CaringForYoung(), st_Dying(), Skylark_Male::st_Dying(), st_Finding_Territory(), st_GivingUpTerritory(), Skylark_Clutch::st_Hatching(), st_Immigrating(), st_Laying(), st_MakingNest(), st_StartingNewBrood(), and st_StoppingBreeding().
◆ NestLoc
bool Skylark_Female::NestLoc |
|
protected |
◆ Resources
double Skylark_Female::Resources |
|
protected |
The documentation for this class was generated from the following files:
bool NestLoc
Definition: skylarks_all.h:713
Definition: Treatment.h:129
Definition: Treatment.h:64
CfgFloat cfg_skylark_pesticide_globaleggshellreduction
The proportion of eggs assumed to crack from pesticide effects as a global effect.
Definition: Treatment.h:84
Definition: Treatment.h:71
Definition: Treatment.h:88
Definition: Treatment.h:68
bool SupplyNestValid() const
Definition: skylarks_all.h:873
Definition: Treatment.h:144
Definition: Treatment.h:52
Definition: Treatment.h:89
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
Definition: Treatment.h:94
virtual double RemoveEM(double food)
Definition: skylarks_all.cpp:3527
Definition: Treatment.h:142
vector< int > m_HabitatTable_Size
Definition: skylarks_all.h:807
int SupplyF_Mig_Mort() const
Definition: skylarks_all.h:456
void AddStriglingMort(const int lifestage) const
Definition: skylarks_all.h:531
double SupplyTemp(void)
Passes a request on to the associated Weather class function, the temperature for the current day.
Definition: Landscape.h:1993
Definition: Treatment.h:83
Definition: Treatment.h:99
TTypesOfVegetation SupplyVegType(int a_x, int a_y)
Returns the vegetation type of the polygon using the polygon reference number a_polyref or coordinate...
Definition: Landscape.h:1925
int m_NestTime
Definition: skylarks_all.h:712
Definition: Treatment.h:76
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
Definition: Treatment.h:35
Definition: Treatment.h:81
Definition: skylarks_all.h:297
Definition: LandscapeFarmingEnums.h:94
CfgFloat cfg_skylark_pesticide_eggshellreduction
The proportion of eggs assumed to crack from pesticide effects.
int SupplySimH() const
Returns landscape height in m.
Definition: PopulationManager.h:569
Definition: Treatment.h:43
Definition: Treatment.h:91
Definition: Treatment.h:127
Definition: Treatment.h:103
CfgFloat cfg_ReturnProbability
Used to change the timing of start of arrival in the landscape.
Definition: Treatment.h:121
int SupplyNoHabitatRefs() const
Definition: skylarks_all.h:830
int SupplyBroodSize() const
Definition: skylarks_all.h:832
static int FoodTripsPerDay
Definition: skylarks_all.cpp:166
Landscape * g_land
Definition: skylarks_all.cpp:44
Definition: Treatment.h:44
Definition: Treatment.h:56
Definition: Treatment.h:125
void CorrectCoords(int &x, int &y)
Function to prevent wrap around errors with co-ordinates using x/y pair.
Definition: Landscape.h:2206
TTypesOfSkState m_CurrentSkState
Definition: skylarks_all.h:517
Definition: Treatment.h:61
Definition: Treatment.h:80
Definition: Treatment.h:60
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: Treatment.h:90
Landscape * L
Definition: skylarks_all.h:214
Definition: Treatment.h:123
Definition: Treatment.h:131
void WriteSKPOM1(const int n, const int n2) const
Definition: skylarks_all.h:451
double SupplyEMi() const
Definition: skylarks_all.h:492
static bool GetBadWeather()
Extreme weather conditions check.
Definition: skylarks_all.cpp:3521
Definition: Treatment.h:138
Definition: Treatment.h:122
Definition: Treatment.h:39
void RemoveFemale(int ref) const
Definition: skylarks_all.cpp:2026
Definition: Treatment.h:110
bool SupplySnowcover(void)
Passes a request on to the associated Weather class function, the snow cover for the current day.
Definition: Landscape.h:2091
double OnFoodMessage(const int n, const double f) const
Definition: skylarks_all.h:842
Definition: Treatment.h:51
int st_Incubating()
Definition: skylarks_all.cpp:6237
double value() const
Definition: Configurator.h:142
int SupplyBroodAge(const int n) const
Definition: skylarks_all.h:834
Definition: Treatment.h:143
Definition: Treatment.h:133
const int February
Julian start dates of the month of February.
Definition: Landscape.h:40
Definition: Treatment.h:104
Definition: Treatment.h:85
int ref
Definition: skylarks_all.h:302
void OnPairing(Skylark_Female *female)
Definition: skylarks_all.cpp:4729
int g_stopdate
Definition: skylarks_all.cpp:40
int st_Emigrating()
Definition: skylarks_all.cpp:5556
SkTerritories * m_OurTerritories
Definition: skylarks_all.h:525
Definition: Treatment.h:140
Definition: Treatment.h:38
Definition: Treatment.h:117
int JuvenileReturnMort
Definition: skylarks_all.cpp:199
Definition: Treatment.h:120
Definition: Treatment.h:59
int st_TempLeavingArea()
Definition: skylarks_all.cpp:5595
Definition: Treatment.h:100
Definition: skylarks_all.h:223
int GetMigrationMortality()
Definition: skylarks_all.cpp:6566
void AddEgg()
Definition: skylarks_all.h:573
Definition: Treatment.h:150
Definition: Treatment.h:124
Definition: Treatment.h:139
int st_BuildingUpResources()
Definition: skylarks_all.cpp:5924
Definition: Treatment.h:126
int m_pesticide_affected
Definition: skylarks_all.h:659
int st_PreparingForBreeding()
Definition: skylarks_all.cpp:5955
Definition: Treatment.h:149
int size
Definition: skylarks_all.h:301
TTypesOfSkState st_MakingNest()
Definition: skylarks_all.cpp:5992
Definition: Treatment.h:132
int st_GivingUpTerritory()
Definition: skylarks_all.cpp:6166
Definition: Treatment.h:148
void OnMateDying()
Definition: skylarks_all.cpp:4140
bool value() const
Definition: Configurator.h:164
Definition: Treatment.h:67
Definition: Treatment.h:42
Definition: Treatment.h:65
Definition: Treatment.h:45
Definition: Treatment.h:98
int CalculateEggNumber() const
Definition: skylarks_all.cpp:6493
void OnMateNeverComesBack(const Skylark_Female *AFemale)
Definition: skylarks_all.cpp:4357
const int June
Julian start dates of the month of June.
Definition: Landscape.h:48
Definition: Treatment.h:77
Definition: Treatment.h:96
Definition: Treatment.h:54
Skylark_Female * Supply_F_Owner(int ref) const
Definition: skylarks_all.cpp:1386
Definition: Treatment.h:130
Definition: Treatment.h:97
Definition: Treatment.h:141
const double KcalPerGInsect_kg_inv
Definition: skylarks_all.cpp:177
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
int CalcFoodTime(double target) const
Definition: skylarks_all.cpp:6521
long Date(void)
Definition: Calendar.h:57
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
Definition: Treatment.h:70
void FemaleOccupy(int ref, Skylark_Female *Female) const
Definition: skylarks_all.cpp:2022
A simple class defining an x,y coordinate set.
Definition: ALMaSS_Setup.h:52
Definition: Treatment.h:46
Skylark_Adult(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3488
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
void CheckManagement()
Used to start a check for any management related effects at the objects current location.
Definition: PopulationManager.cpp:1591
int Age
Definition: skylarks_all.h:518
Definition: Treatment.h:111
int st_StoppingBreeding()
Definition: skylarks_all.cpp:6286
int st_Finding_Territory()
Definition: skylarks_all.cpp:5631
Definition: Treatment.h:135
Definition: Treatment.h:82
void StartDeveloping()
Definition: skylarks_all.h:575
Definition: Treatment.h:63
Definition: Treatment.h:62
int EggCounter
Definition: skylarks_all.h:715
Definition: Treatment.h:119
int SupplyNoTerritories() const
Definition: skylarks_all.cpp:1362
Definition: Treatment.h:72
Definition: Treatment.h:112
Definition: Treatment.h:137
Definition: Treatment.h:40
Definition: Treatment.h:53
double MaxFeedRain
Definition: skylarks_all.cpp:196
const char * SupplyStateNames(int i) const
Definition: PopulationManager.h:738
bool st_Immigrating()
Definition: skylarks_all.cpp:5517
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Treatment.h:145
void ResetBreedingSuccess()
Definition: skylarks_all.cpp:5914
void EstablishTerritory()
Definition: skylarks_all.cpp:5718
Definition: Treatment.h:86
Definition: Treatment.h:49
vector< double > m_InsectTable
Definition: skylarks_all.h:808
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
Definition: Treatment.h:95
Definition: Treatment.h:147
const int April
Julian start dates of the month of April.
Definition: Landscape.h:44
Definition: Treatment.h:36
int y
Definition: skylarks_all.h:300
bool m_StepDone
Indicates whether the iterative step code is done for this timestep.
Definition: PopulationManager.h:133
Definition: skylarks_all.h:760
TTypesOfPesticide SupplyPesticideType(void)
Gets type of pesticide effect from a reference.
Definition: Landscape.h:788
int m_x
Definition: ALMaSS_Setup.h:55
Definition: Treatment.h:116
Definition: Treatment.h:93
bool Paired
Definition: skylarks_all.h:670
int SupplyYearNumber(void)
Passes a request on to the associated Calendar class function, returns m_simulationyear
Definition: Landscape.h:2287
Definition: Treatment.h:114
int m_BreedingAttempts
Definition: skylarks_all.h:716
int value() const
Definition: Configurator.h:116
APoint SupplyNestLoc() const
Definition: skylarks_all.h:874
CfgFloat cfg_FemaleMinTerritoryAcceptScore
int m_EggNumber
Definition: skylarks_all.h:718
Definition: Treatment.h:75
bool st_Arriving()
Definition: skylarks_all.cpp:5500
Definition: Treatment.h:47
Definition: LandscapeFarmingEnums.h:1067
Definition: Treatment.h:106
void OnEggHatch()
Definition: skylarks_all.cpp:4630
Definition: Treatment.h:109
Definition: Treatment.h:48
std::string EventtypeToString(int a_event)
Returns the text representation of a treatment type.
Definition: Landscape.cpp:6024
skTerritory_struct Supply_Territory() const
Definition: skylarks_all.cpp:4373
double GetFood(int time)
Definition: skylarks_all.cpp:6441
Definition: Treatment.h:146
Definition: Treatment.h:134
double SupplyRain(void)
Passes a request on to the associated Weather class function, the amount of rain for the current day.
Definition: Landscape.h:1971
int m_Counter1
Definition: skylarks_all.h:711
int GoodWeather
Definition: skylarks_all.h:656
Definition: Treatment.h:118
const int May
Julian start dates of the month of May.
Definition: Landscape.h:46
const int January
Julian start dates of the month of January.
Definition: Landscape.h:38
Definition: Treatment.h:113
void OnNestLocation(int x, int y)
Definition: skylarks_all.cpp:4717
double SupplyVegHeight(int a_polyref)
Returns the height of the vegetation using the polygon reference number a_polyref or based on the x,...
Definition: Landscape.h:1527
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
double MyExtractEff
Definition: skylarks_all.h:658
static void OnArrayBoundsError()
Used for debugging only, tests basic object properties.
Definition: PopulationManager.cpp:1614
Definition: LandscapeFarmingEnums.h:1061
Definition: Treatment.h:41
int st_Floating()
Definition: skylarks_all.cpp:5614
double Supply_TerritoryQual() const
Definition: skylarks_all.h:837
Skylark_Population_Manager * m_OurPopulationManager
Definition: skylarks_all.h:526
double Resources
Definition: skylarks_all.h:714
Definition: Treatment.h:37
Skylark_Male * Supply_Owner(int ref) const
Definition: skylarks_all.cpp:1382
int st_StartingNewBrood()
Definition: skylarks_all.cpp:6202
Definition: Treatment.h:79
void OnMateLeaving()
Definition: skylarks_all.cpp:4163
int st_EggHatching()
Definition: skylarks_all.cpp:6273
const int September
Julian start dates of the month of September.
Definition: Landscape.h:54
Definition: Treatment.h:74
void CreateObjects(int ob_type, TAnimal *pTAo, void *null, Skylark_struct *data, int number)
Definition: skylarks_all.cpp:539
Definition: LandscapeFarmingEnums.h:65
int SupplySimW() const
Returns landscape width in m.
Definition: PopulationManager.h:567
const int March
Julian start dates of the month of March.
Definition: Landscape.h:42
bool HaveTerritory
Definition: skylarks_all.h:813
Definition: Treatment.h:136
Definition: Treatment.h:108
Definition: Treatment.h:69
Definition: Treatment.h:105
int m_toowet
Definition: skylarks_all.h:720
Definition: Treatment.h:78
int st_Flocking()
Definition: skylarks_all.cpp:5473
int SupplyBroodWeight(const int n) const
Definition: skylarks_all.h:839
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
Definition: Treatment.h:66
Definition: Treatment.h:92
Definition: Treatment.h:102
Skylark_Male * MyMate
Definition: skylarks_all.h:724
Definition: Treatment.h:57
CfgInt cfg_SkylarkFirstBreedingDate
The date at which breeding start can happen.
Definition: Treatment.h:87
int m_CurrentStateNo
The basic state number for all objects - '-1' indicates death.
Definition: PopulationManager.h:131
Definition: Treatment.h:101
const double Breed_Temp_Thresh
Definition: skylarks_all.cpp:211
Definition: Treatment.h:73
double nqual
Definition: skylarks_all.h:303
Skylark_Female * Mother
Definition: skylarks_all.h:583
virtual void ReInit(int x, int y, double size, int age, SkTerritories *Terrs, Landscape *L, Skylark_Population_Manager *SPM, int bx, int by, int mh)
Definition: skylarks_all.cpp:3502
Definition: Treatment.h:55
void OnMumGone()
Definition: skylarks_all.cpp:2342
skTerritory_struct MyTerritory
Definition: skylarks_all.h:660
void FeedYoung()
Definition: skylarks_all.cpp:6344
void Warn(std::string a_msg1, std::string a_msg2)
Wrapper for the g_msg Warn function.
Definition: Landscape.h:2250
CfgBool cfg_skylark_pesticide_eggshellreduction_perclutch
If true egg shell reduction works at clutch level, if false it is considered per egg.
Definition: Treatment.h:34
Skylark_Female * MyMate
Definition: skylarks_all.h:798
TTypesOfSkState st_Laying()
Definition: skylarks_all.cpp:6060
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
double m_MinFemaleAcceptScore
Definition: skylarks_all.h:721
int x
Definition: skylarks_all.h:299
Skylark_Clutch * MyClutch
Definition: skylarks_all.h:710
int st_CaringForYoung()
Definition: skylarks_all.cpp:6316
int SupplyDaylength(void)
Passes a request on to the associated Weather class function, the day length for the current day.
Definition: Landscape.h:2201
int SupplyPolyRef(int a_x, int a_y)
Get the in map polygon reference number from the x, y location.
Definition: Landscape.h:2157
CfgInt cfg_insecticide_direct_mortF
Definition: Treatment.h:58
Definition: Treatment.h:128
Definition: MapErrorMsg.h:34
int m_y
Definition: ALMaSS_Setup.h:56
long SupplyGlobalDate(void)
Passes a request on to the associated Calendar class function, returns the simulation global date for...
Definition: Landscape.h:2292
Definition: Treatment.h:50
Definition: Treatment.h:115
void st_Dying()
Definition: skylarks_all.cpp:5735
const int October
Julian start dates of the month of October.
Definition: Landscape.h:56
Definition: Treatment.h:33
int m_BreedingSuccess
Definition: skylarks_all.h:717
Definition: Treatment.h:107
bool BSuccess
Definition: skylarks_all.h:657