File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
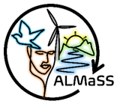 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
SE_SpringBarley class
.
More...
#include <SE_SpringBarley.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
SE_SpringBarley class
.
See SE_SpringBarley.h::SE_SpringBarleyToDo for a complete list of all possible events triggered codes by the SpringBarley management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ SE_SpringBarley()
◆ Do()
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with a_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional winter wheat.
Reimplemented from Crop.
148 g_msg->
Warn(
WARN_BUG,
"SE_SpringBarley::Do(): ",
"Harvest too late for the next crop to start!!!");
149 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
150 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
153 for (
int i = 0; i < noDates; i++) {
170 printf(
"Poly: %d\n", a_field->
GetPoly());
171 g_msg->
Warn(
WARN_BUG,
"SE_SpringBarley::Do(): ",
"Crop start attempt between 1st Jan & 1st July");
174 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
175 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
181 g_msg->
Warn(
WARN_BUG,
"SE_SpringBarley::Do(): ",
"Crop start attempt after last possible start date");
184 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
185 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
330 if (!a_farm->
FP_NPK(a_field, 0.0,
378 if (!a_farm->
FP_NS(a_field, 0.0,
416 "Unknown event type! ",
"");
References Farm::AutumnPlough(), cfg_SE_SpringBarley_SkScrapes, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt(), Farm::FA_Slurry(), Farm::FP_Manure(), Farm::FP_NPK(), Farm::FP_NS(), Farm::FP_Slurry(), Farm::FungicideTreat(), g_date, g_msg, LE::GetMDates(), LE::GetOwner(), LE::GetPoly(), Farm::GetPreviousTov(), LE::GetRotIndex(), Farm::GetType(), Calendar::GetYearNumber(), Farm::Harvest(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, LE::m_skylarkscrapes, FarmEvent::m_startday, FarmEvent::m_todo, Calendar::OldDays(), se_sb_autumn_plough, se_sb_fungicide, se_sb_harvest, se_sb_herbicide1, se_sb_herbicide2, se_sb_insecticide, se_sb_npk, se_sb_ns, se_sb_ns_manure, se_sb_slurry1, se_sb_slurry2, se_sb_spring_harrow1, se_sb_spring_harrow2, se_sb_spring_plough, se_sb_spring_sow, se_sb_spring_sow_w_ferti, se_sb_start, se_sb_straw_removal, se_sb_stubble_cultivator1, se_sb_stubble_cultivator2, LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringSow(), Farm::SpringSowWithFerti(), Farm::StrawRemoval(), Farm::StubbleCultivatorHeavy(), tof_OptimisingFarm, CfgBool::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void SE_SpringBarley::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, Crop::m_base_elements_no, Crop::m_ManagementCategories, SE_SB_BASE, and se_sb_foobar.
Referenced by SE_SpringBarley().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
#define SE_SB_BASE
Definition: SE_SpringBarley.h:53
Definition: SE_SpringBarley.h:71
Definition: SE_SpringBarley.h:85
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
int GetYearNumber(void)
Definition: Calendar.h:72
Definition: SE_SpringBarley.h:82
bool m_first_year
Definition: Farm.h:386
virtual bool StrawRemoval(LE *a_field, double a_user, int a_days)
Straw covering applied on a_field.
Definition: FarmFuncs.cpp:1752
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: SE_SpringBarley.h:73
Definition: LandscapeFarmingEnums.h:1006
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
Definition: SE_SpringBarley.h:69
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
TTypesOfFarm GetType(void)
Definition: Farm.h:956
int m_base_elements_no
Definition: Farm.h:505
Definition: SE_SpringBarley.h:84
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
Definition: SE_SpringBarley.h:80
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: SE_SpringBarley.h:70
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
bool value() const
Definition: Configurator.h:164
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: SE_SpringBarley.h:66
Definition: SE_SpringBarley.h:77
Definition: LandscapeFarmingEnums.h:1004
void SetUpFarmCategoryInformation()
Definition: SE_SpringBarley.h:109
bool m_skylarkscrapes
For management testing of skylark scrapes.
Definition: Elements.h:95
long Date(void)
Definition: Calendar.h:57
Definition: SE_SpringBarley.h:67
Definition: LandscapeFarmingEnums.h:1008
Definition: LandscapeFarmingEnums.h:1003
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
int GetRotIndex(void)
Definition: Elements.h:373
virtual bool FP_NS(LE *a_field, double a_user, int a_days)
Apply NS fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:740
Definition: SE_SpringBarley.h:74
Definition: SE_SpringBarley.h:81
Definition: SE_SpringBarley.h:65
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: SE_SpringBarley.h:78
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: SE_SpringBarley.h:83
Definition: SE_SpringBarley.h:76
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
Definition: LandscapeFarmingEnums.h:696
Definition: SE_SpringBarley.h:75
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool SpringSowWithFerti(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event with start fertilizer in the spring on a_field.
Definition: FarmFuncs.cpp:537
Definition: SE_SpringBarley.h:72
Definition: SE_SpringBarley.h:79
Definition: LandscapeFarmingEnums.h:1012
CfgBool cfg_SE_SpringBarley_SkScrapes("SE_CROP_SB_SK_SCRAPES", CFG_CUSTOM, false)
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: SE_SpringBarley.h:68
void SetMConstants(int a, int c)
Definition: Elements.h:408
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459