File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
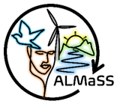 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
PLWinterTriticale class
.
More...
#include <PLWinterTriticale.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
PLWinterTriticale class
.
See PLWinterTriticale.h::PLWinterTriticaleToDo for a complete list of all possible events triggered codes by the winter triticale management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ PLWinterTriticale()
◆ Do()
bool PLWinterTriticale::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with m_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional winter triticale.
Reimplemented from Crop.
118 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): ",
"Harvest too late for the next crop to start!!!");
120 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
123 for (
int i = 0; i < noDates; i++) {
141 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): ",
"Crop start attempt between 1st Jan & 1st July");
145 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
151 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): ",
"Crop start attempt after last possible start date");
155 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
746 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'StrawChopping' execution",
"");
774 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'StrawChopping' execution",
"");
798 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'HayBailing' execution",
"");
819 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'FP_RSM' execution",
"");
841 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'FA_RSM' execution",
"");
863 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'FP_Calcium' execution",
"");
883 g_msg->
Warn(
WARN_BUG,
"PLWinterTriticale::Do(): failure in 'FA_Calcium' execution",
"");
900 "Unknown event type! ",
"");
References Farm::AutumnHarrow(), Farm::AutumnPlough(), Farm::AutumnRoll(), Farm::AutumnSow(), cfg_pest_product_amounts, cfg_pest_wintertriticale_on, cfg_seedcoating_product_amounts, cfg_seedcoating_wintertriticale_on, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt(), Farm::FA_AmmoniumSulphate(), Farm::FA_Calcium(), Farm::FA_ManganeseSulphate(), Farm::FA_NPKS(), Farm::FA_PK(), Farm::FA_RSM(), Farm::FA_Slurry(), Farm::FP_AmmoniumSulphate(), Farm::FP_Calcium(), Farm::FP_ManganeseSulphate(), Farm::FP_NPKS(), Farm::FP_PK(), Farm::FP_RSM(), Farm::FP_Slurry(), Farm::FungicideTreat(), g_date, g_msg, LE::GetGreenBiomass(), LE::GetMConstants(), LE::GetMDates(), LE::GetOwner(), LE::GetPoly(), Farm::GetPreviousTov(), LE::GetRotIndex(), Farm::GetType(), Calendar::GetYearNumber(), Farm::GrowthRegulator(), Farm::Harvest(), Farm::HayBailing(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, FarmEvent::m_startday, FarmEvent::m_todo, Calendar::OldDays(), pl_wt_autumn_harrow1, pl_wt_autumn_harrow2, pl_wt_autumn_plough, pl_wt_autumn_roll, pl_wt_autumn_sow, PL_WT_FERTI_P1, pl_wt_ferti_p1, pl_wt_ferti_p10, pl_wt_ferti_p11, pl_wt_ferti_p2, pl_wt_ferti_p3, pl_wt_ferti_p4, pl_wt_ferti_p5, pl_wt_ferti_p6, pl_wt_ferti_p7, pl_wt_ferti_p8, pl_wt_ferti_p9, PL_WT_FERTI_S1, pl_wt_ferti_s1, pl_wt_ferti_s10, pl_wt_ferti_s11, pl_wt_ferti_s2, pl_wt_ferti_s3, pl_wt_ferti_s4, pl_wt_ferti_s5, pl_wt_ferti_s6, pl_wt_ferti_s7, pl_wt_ferti_s8, pl_wt_ferti_s9, pl_wt_fungicide1, pl_wt_fungicide2, pl_wt_fungicide3, pl_wt_fungicide4, pl_wt_growth_regulator1, pl_wt_harvest, pl_wt_hay_bailing, pl_wt_herbicide1, pl_wt_herbicide2, pl_wt_insecticide1, pl_wt_insecticide2, pl_wt_insecticide3, pl_wt_preseeding_cultivator, pl_wt_preseeding_cultivator_sow, pl_wt_start, pl_wt_straw_chopping, pl_wt_stubble_cultivator_heavy, pl_wt_stubble_harrow, PL_WT_STUBBLE_PLOUGH, pl_wt_stubble_plough, ppp_1, Farm::PreseedingCultivator(), Farm::PreseedingCultivatorSow(), Farm::ProductApplication(), LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent_(), Farm::StrawChopping(), Farm::StubbleCultivatorHeavy(), Farm::StubbleHarrowing(), Farm::StubblePlough(), tof_OptimisingFarm, tov_PLWinterTriticale, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void PLWinterTriticale::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, Crop::m_base_elements_no, Crop::m_ManagementCategories, pl_wt_foobar, and PLWINTERTRITICALE_BASE.
Referenced by PLWinterTriticale().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: PLWinterTriticale.h:51
Definition: PLWinterTriticale.h:70
Definition: PLWinterTriticale.h:56
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
Definition: PLWinterTriticale.h:85
Definition: PLWinterTriticale.h:77
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
Definition: PLWinterTriticale.h:52
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: PLWinterTriticale.h:60
#define PL_WT_FERTI_P1
A flag used to indicate autumn ploughing status.
Definition: PLWinterTriticale.h:28
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
bool m_lock
Definition: Farm.h:384
virtual bool FP_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply Manganse Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:840
Definition: PLWinterTriticale.h:67
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
void SetUpFarmCategoryInformation()
Definition: PLWinterTriticale.h:109
int GetMConstants(int a)
Definition: Elements.h:407
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
int GetYearNumber(void)
Definition: Calendar.h:72
Definition: PLWinterTriticale.h:71
Definition: PLWinterTriticale.h:48
bool m_first_year
Definition: Farm.h:386
virtual bool StubblePlough(LE *a_field, double a_user, int a_days)
Carry out a stubble ploughing event on a_field. This is similar to normal plough but shallow (normall...
Definition: FarmFuncs.cpp:232
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: PLWinterTriticale.h:61
Definition: LandscapeFarmingEnums.h:1006
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
CfgArray_Double cfg_seedcoating_product_amounts
Amount of seed coating pesticide applied in grams of active substance per hectare for each of the 10 ...
virtual bool FA_NPKS(LE *a_field, double a_user, int a_days)
Apply NPKS fertilizer, on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:968
TTypesOfFarm GetType(void)
Definition: Farm.h:956
Definition: PLWinterTriticale.h:74
int m_base_elements_no
Definition: Farm.h:505
Definition: PLWinterTriticale.h:66
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: PLWinterTriticale.h:58
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
CfgBool cfg_pest_wintertriticale_on
Turn on pesticides for winter triticale.
Definition: PLWinterTriticale.h:76
bool value() const
Definition: Configurator.h:164
Definition: LandscapeFarmingEnums.h:235
Definition: PLWinterTriticale.h:63
Definition: PLWinterTriticale.h:84
virtual bool FA_RSM(LE *a_field, double a_user, int a_days)
RSM (ammonium nitrate solution) applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1154
Definition: LandscapeFarmingEnums.h:1004
Definition: PLWinterTriticale.h:83
Definition: PLWinterTriticale.h:79
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: PLWinterTriticale.h:43
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
Definition: PLWinterTriticale.h:49
Definition: PLWinterTriticale.h:53
virtual bool FA_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply manganese sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1095
long OldDays(void)
Definition: Calendar.h:60
Definition: PLWinterTriticale.h:47
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
int GetRotIndex(void)
Definition: Elements.h:373
Definition: PLWinterTriticale.h:82
Definition: PLWinterTriticale.h:59
#define PL_WT_FERTI_S1
Definition: PLWinterTriticale.h:29
Definition: PLWinterTriticale.h:46
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Definition: PLWinterTriticale.h:69
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: PLWinterTriticale.h:81
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
Definition: PLWinterTriticale.h:57
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
Definition: PLWinterTriticale.h:41
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: PLWinterTriticale.h:44
Definition: PLWinterTriticale.h:40
virtual bool AutumnRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the autumn on a_field.
Definition: FarmFuncs.cpp:299
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
Definition: LandscapeFarmingEnums.h:696
Definition: PLWinterTriticale.h:65
Definition: PLWinterTriticale.h:78
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: PLWinterTriticale.h:64
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
Definition: PLWinterTriticale.h:80
Definition: PLWinterTriticale.h:50
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: PLWinterTriticale.h:62
Definition: PLWinterTriticale.h:54
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
virtual bool FP_RSM(LE *a_field, double a_user, int a_days)
RSM (ammonium nitrate solution) applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:940
Definition: LandscapeFarmingEnums.h:1012
Definition: PLWinterTriticale.h:45
Definition: PLWinterTriticale.h:68
virtual bool GrowthRegulator(LE *a_field, double a_user, int a_days)
Apply growth regulator to a_field.
Definition: FarmFuncs.cpp:2070
CfgBool cfg_seedcoating_wintertriticale_on
Turn on seed coating for winter triticale.
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Definition: PLWinterTriticale.h:73
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
void SetMConstants(int a, int c)
Definition: Elements.h:408
#define PLWINTERTRITICALE_BASE
Definition: PLWinterTriticale.h:24
Definition: PLWinterTriticale.h:38
FarmEvent * m_ev
Definition: Farm.h:500
Definition: PLWinterTriticale.h:75
Definition: PLWinterTriticale.h:72
Definition: PLWinterTriticale.h:42
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
virtual bool FP_NPKS(LE *a_field, double a_user, int a_days)
Apply NPKS fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:630
Definition: PLWinterTriticale.h:55
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751
#define PL_WT_STUBBLE_PLOUGH
Definition: PLWinterTriticale.h:30