Loading [MathJax]/extensions/ams.js
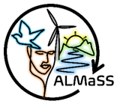 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
PLBeet class
.
More...
#include <PLBeet.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
PLBeet class
.
See PLBeet.h::PLBeetToDo for a complete list of all possible events triggered codes by the beet management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ PLBeet()
◆ Do()
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with m_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional beet.
Reimplemented from Crop.
118 g_msg->
Warn(
WARN_BUG,
"PLBeet::Do(): ",
"Harvest too late for the next crop to start!!!");
120 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
123 for (
int i = 0; i < noDates; i++) {
141 g_msg->
Warn(
WARN_BUG,
"PLBeet::Do(): ",
"Crop start attempt between 1st Jan & 1st July");
145 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
151 g_msg->
Warn(
WARN_BUG,
"PLBeet::Do(): ",
"Crop start attempt after last possible start date");
155 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
772 "Unknown event type! ",
"");
References Farm::AutumnHarrow(), cfg_pest_beet_on, cfg_pest_product_amounts, cfg_seedcoating_beet_on, cfg_seedcoating_product_amounts, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt(), Farm::FA_AmmoniumSulphate(), Farm::FA_Calcium(), Farm::FA_ManganeseSulphate(), Farm::FA_PK(), Farm::FA_Slurry(), Farm::FP_AmmoniumSulphate(), Farm::FP_Calcium(), Farm::FP_ManganeseSulphate(), Farm::FP_PK(), Farm::FP_Slurry(), Farm::FungicideTreat(), g_date, g_msg, LE::GetGreenBiomass(), LE::GetMConstants(), LE::GetMDates(), LE::GetOwner(), LE::GetPoly(), Farm::GetPreviousTov(), LE::GetRotIndex(), Farm::GetType(), Calendar::GetYearNumber(), Farm::Harvest(), Farm::HeavyCultivatorAggregate(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, FarmEvent::m_startday, FarmEvent::m_todo, Calendar::OldDays(), pl_be_autumn_harrow1, pl_be_autumn_harrow2, PL_BE_FERTI_P1, pl_be_ferti_p1, pl_be_ferti_p2, pl_be_ferti_p3, pl_be_ferti_p4, pl_be_ferti_p5, pl_be_ferti_p6, pl_be_ferti_p7, pl_be_ferti_p8, PL_BE_FERTI_S1, pl_be_ferti_s1, pl_be_ferti_s2, pl_be_ferti_s3, pl_be_ferti_s4, pl_be_ferti_s5, pl_be_ferti_s6, pl_be_ferti_s7, pl_be_ferti_s8, pl_be_fungicide1, pl_be_fungicide2, pl_be_harrow_before_emergence, pl_be_harvest, pl_be_heavy_cultivator, PL_BE_HERBI1, PL_BE_HERBI3, PL_BE_HERBI_DATE, pl_be_herbicide1, pl_be_herbicide2, pl_be_herbicide3, pl_be_herbicide4, pl_be_insecticide, pl_be_preseeding_cultivator, pl_be_preseeding_cultivator_sow, PL_BE_SPRING_FERTI, pl_be_spring_harrow, pl_be_spring_sow, pl_be_start, pl_be_stubble_harrow, PL_BE_STUBBLE_PLOUGH, pl_be_stubble_plough, pl_be_thinning, PL_BE_WATER_DATE, pl_be_watering1, pl_be_watering2, pl_be_watering3, PL_BE_WINTER_PLOUGH, pl_be_winter_plough, pl_be_winter_stubble_cultivator_heavy, ppp_1, Farm::PreseedingCultivator(), Farm::PreseedingCultivatorSow(), Farm::ProductApplication(), Farm::RowCultivation(), LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent_(), Farm::SpringHarrow(), Farm::SpringSow(), Farm::StubbleCultivatorHeavy(), Farm::StubbleHarrowing(), Farm::StubblePlough(), tof_OptimisingFarm, tov_PLBeet, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), WARN_BUG, Farm::Water(), and Farm::WinterPlough().
◆ SetUpFarmCategoryInformation()
void PLBeet::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, fmc_Watering, Crop::m_base_elements_no, Crop::m_ManagementCategories, pl_be_foobar, and PLBEET_BASE.
Referenced by PLBeet().
The documentation for this class was generated from the following files:
- /builds/ALMaSS/ALMaSS_MIDox/Landscape/cropprogs/PLBeet.h
- /builds/ALMaSS/ALMaSS_MIDox/Landscape/cropprogs/PLBeet.cpp
int GetMDates(int a, int b)
Definition: Elements.h:405
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool FP_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply Manganse Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:840
Definition: LandscapeFarmingEnums.h:242
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
int GetMConstants(int a)
Definition: Elements.h:407
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
int GetYearNumber(void)
Definition: Calendar.h:72
bool m_first_year
Definition: Farm.h:386
virtual bool StubblePlough(LE *a_field, double a_user, int a_days)
Carry out a stubble ploughing event on a_field. This is similar to normal plough but shallow (normall...
Definition: FarmFuncs.cpp:232
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
void SetUpFarmCategoryInformation()
Definition: PLBeet.h:111
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
TTypesOfFarm GetType(void)
Definition: Farm.h:956
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
#define PLBEET_BASE
Definition: PLBeet.h:24
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
#define PL_BE_WATER_DATE
Definition: PLBeet.h:33
bool value() const
Definition: Configurator.h:164
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
Definition: LandscapeFarmingEnums.h:1011
virtual bool FA_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply manganese sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1095
long OldDays(void)
Definition: Calendar.h:60
CfgBool cfg_pest_beet_on
Turn on pesticides for beet.
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
#define PL_BE_STUBBLE_PLOUGH
Definition: PLBeet.h:30
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
int GetRotIndex(void)
Definition: Elements.h:373
CfgArray_Double cfg_seedcoating_product_amounts
Amount of seed coating pesticide applied in grams of active substance per hectare for each of the 10 ...
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
#define PL_BE_FERTI_P1
A flag used to indicate autumn ploughing status.
Definition: PLBeet.h:28
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
#define PL_BE_WINTER_PLOUGH
Definition: PLBeet.h:31
virtual bool RowCultivation(LE *a_field, double a_user, int a_days)
Carry out a harrowing between crop rows on a_field.
Definition: FarmFuncs.cpp:1183
Definition: LandscapeFarmingEnums.h:696
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
#define PL_BE_SPRING_FERTI
Definition: PLBeet.h:32
#define PL_BE_HERBI_DATE
Definition: PLBeet.h:34
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
#define PL_BE_HERBI1
Definition: PLBeet.h:35
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: LandscapeFarmingEnums.h:1012
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
void SetMConstants(int a, int c)
Definition: Elements.h:408
FarmEvent * m_ev
Definition: Farm.h:500
CfgBool cfg_seedcoating_beet_on
Turn on seed coating for beet.
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
#define PL_BE_FERTI_S1
Definition: PLBeet.h:29
virtual bool HeavyCultivatorAggregate(LE *a_field, double a_user, int a_days)
Carry out a heavy cultivation event on a_field. This is non-inversion type of cultivation which can b...
Definition: FarmFuncs.cpp:259
Definition: LandscapeFarmingEnums.h:1007
#define PL_BE_HERBI3
Definition: PLBeet.h:36
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751