Loading [MathJax]/extensions/ams.js
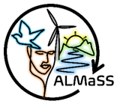 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
NLMaize class
.
More...
#include <NLMaize.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
NLMaize class
.
See NLMaize.h::NLMaizeToDo for a complete list of all possible events triggered codes by the mazie management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ NLMaize()
◆ Do()
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with m_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional maize.
Reimplemented from Crop.
76 int l_nextcropstartdate;
89 std::vector<std::vector<int>> flexdates(1 + 1, std::vector<int>(2, 0));
106 int day_num_shift = 365;
342 m_farm->
AddNewEvent(
tov_NLCatchCropPea,
g_date->
Date(),
m_ev->
m_field,
PROG_START,
m_ev->
m_field->GetRunNum(),
false, l_nextcropstartdate,
false, l_tov,
fmc_Others,
false,
false);
357 "Unknown event type! ",
"");
References Farm::AddNewEvent(), cfg_NLCatchCropPct, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt_prob(), Farm::FA_AmmoniumSulphate(), Farm::FA_Slurry(), fmc_Others, Farm::FP_AmmoniumSulphate(), Farm::FP_Slurry(), g_date, g_msg, LE::GetGreenBiomass(), LE::GetMDates(), Farm::GetNextCropStartDate(), LE::GetSoilType(), LE::GetUnsprayedMarginPolyRef(), Farm::Harvest(), Farm::HerbicideTreat(), Farm::IsStockFarmer(), Crop::m_ev, Crop::m_farm, FarmEvent::m_field, Crop::m_field, FarmEvent::m_forcespring, Crop::m_last_date, FarmEvent::m_lock, Crop::m_OurLandscape, FarmEvent::m_todo, nl_m_ferti_p1, nl_m_ferti_p2, nl_m_ferti_s1, nl_m_ferti_s2, nl_m_harrow, nl_m_harvest, nl_m_herbicide1, nl_m_preseeding_cultivator, nl_m_spring_plough1, nl_m_spring_plough2, nl_m_spring_sow, nl_m_spring_sow_with_ferti, nl_m_start, NL_M_START_FERTI, nl_m_straw_chopping, nl_m_stubble_harrow1, nl_m_stubble_harrow2, nl_m_winter_plough1, nl_m_winter_plough2, Calendar::OldDays(), Farm::PreseedingCultivator(), PROG_START, LE::SetVegPatchy(), LE::SetVegType(), Farm::ShallowHarrow(), Crop::SimpleEvent_(), Farm::SpringPlough(), Farm::SpringSow(), Farm::SpringSowWithFerti(), Crop::StartUpCrop(), Farm::StrawChopping(), Farm::StubbleHarrowing(), Landscape::SupplyLEPointer(), tov_NLCatchCropPea, tov_NLMaize, tov_Undefined, CfgFloat::value(), MapErrorMsg::Warn(), WARN_BUG, and Farm::WinterPlough().
◆ SetUpFarmCategoryInformation()
void NLMaize::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
The documentation for this class was generated from the following files:
- /builds/ALMaSS/ALMaSS_MIDox/Landscape/cropprogs/NLMaize.h
- /builds/ALMaSS/ALMaSS_MIDox/Landscape/cropprogs/NLMaize.cpp
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
LE * SupplyLEPointer(int a_polyref)
Returns a pointer to the object referred to by the polygon number.
Definition: Landscape.h:1722
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
#define NLMAIZE_BASE
Definition: NLMaize.h:24
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
int GetNextCropStartDate(LE *a_field, TTypesOfVegetation &a_curr_veg)
Returns the start date of the next crop in the rotation.
Definition: Farm.cpp:920
void AddNewEvent(TTypesOfVegetation a_event, long a_date, LE *a_field, int a_todo, long a_num, bool a_lock, int a_start, bool a_first_year, TTypesOfVegetation a_crop, FarmManagementCategory a_fmc, bool a_forcespring, bool a_forcespringOK)
Adds an event to the event queue for a farm.
Definition: Farm.cpp:845
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
double value() const
Definition: Configurator.h:142
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
#define NL_M_START_FERTI
A flag used to indicate autumn ploughing status.
Definition: NLMaize.h:28
CfgFloat cfg_NLCatchCropPct
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
int GetSoilType()
Definition: Elements.h:302
Definition: LandscapeFarmingEnums.h:253
Definition: LandscapeFarmingEnums.h:1003
void SetVegPatchy(bool p)
Definition: Elements.h:229
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
LE * m_field
Definition: Farm.h:391
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
void SetUpFarmCategoryInformation()
Definition: NLMaize.h:80
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
Definition: LandscapeFarmingEnums.h:610
virtual void SetVegType(TTypesOfVegetation)
Definition: Elements.h:175
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
virtual bool ShallowHarrow(LE *a_field, double a_user, int a_days)
Carry out a shallow harrow event on a_field, e.g., after grass cutting event.
Definition: FarmFuncs.cpp:473
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool SpringSowWithFerti(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event with start fertilizer in the spring on a_field.
Definition: FarmFuncs.cpp:537
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: LandscapeFarmingEnums.h:1012
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
int GetUnsprayedMarginPolyRef(void)
Definition: Elements.h:383
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
bool m_forcespringpossible
Used to signal that the crop can be forced to start in spring.
Definition: Farm.h:508
#define PROG_START
Definition: Farm.h:69
FarmEvent * m_ev
Definition: Farm.h:500
Definition: LandscapeFarmingEnums.h:268
Landscape * m_OurLandscape
Definition: Farm.h:506
Definition: MapErrorMsg.h:34
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751