File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
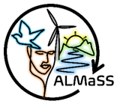 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
IRSpringBarley class
.
More...
#include <IRSpringBarley.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
IRSpringBarley class
.
See IRSpringBarley.h::IRSpringBarleyToDo for a complete list of all possible events triggered codes by the management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ IRSpringBarley()
◆ Do()
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with a_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop.
Reimplemented from Crop.
147 g_msg->
Warn(
WARN_BUG,
"IRSpringBarley::Do(): ",
"Harvest too late for the next crop to start!!!");
148 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
149 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
152 for (
int i = 0; i < noDates; i++) {
169 printf(
"Poly: %d\n", a_field->
GetPoly());
170 g_msg->
Warn(
WARN_BUG,
"IRSpringBarley::Do(): ",
"Crop start attempt between 1st Jan & 1st July");
173 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
174 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
180 g_msg->
Warn(
WARN_BUG,
"IRSpringBarley::Do(): ",
"Crop start attempt after last possible start date");
183 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
184 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
492 "Unknown event type! ",
"");
References Farm::AutumnSow(), cfg_IR_SpringBarley_SkScrapes, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt_prob(), Farm::FA_AmmoniumSulphate(), Farm::FA_NPK(), Farm::FA_Slurry(), Farm::FP_AmmoniumSulphate(), Farm::FP_NPK(), Farm::FP_Slurry(), Farm::FungicideTreat(), g_date, g_msg, LE::GetMDates(), LE::GetOwner(), LE::GetPoly(), Farm::GetPreviousTov(), LE::GetRotIndex(), Farm::GetType(), Calendar::GetYearNumber(), Farm::Harvest(), Farm::HayBailing(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), IR_SB_CP, ir_sb_cultivation_sow_mt, ir_sb_ferti_p1, ir_sb_ferti_p2, ir_sb_ferti_p3, ir_sb_ferti_s1, ir_sb_ferti_s2, ir_sb_ferti_s3, ir_sb_fungicide1, ir_sb_fungicide2, ir_sb_harvest, ir_sb_hay_bailing, ir_sb_herbicide1_cp, ir_sb_herbicide1_mt, ir_sb_herbicide2, ir_sb_insecticide1, ir_sb_insecticide2, ir_sb_molluscicide, IR_SB_MT, ir_sb_sow_cover_crop, ir_sb_spring_harrow_cp, ir_sb_spring_plough_cp, ir_sb_spring_roll, ir_sb_spring_sow_cp, ir_sb_start, ir_sb_stubble_cultivator, ir_sb_stubble_cultivator_mt, ir_sb_stubble_harrow_cp, ir_sb_stubble_harrow_mt, Farm::IsStockFarmer(), Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, LE::m_skylarkscrapes, FarmEvent::m_startday, FarmEvent::m_todo, Farm::Molluscicide(), Calendar::OldDays(), Farm::PreseedingCultivatorSow(), LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringRoll(), Farm::SpringSow(), Farm::StubbleHarrowing(), Farm::StubblePlough(), tof_OptimisingFarm, CfgBool::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void IRSpringBarley::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, IR_SB_BASE, ir_sb_foobar, Crop::m_base_elements_no, and Crop::m_ManagementCategories.
Referenced by IRSpringBarley().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
virtual bool SpringRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the spring on a_field.
Definition: FarmFuncs.cpp:487
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: IRSpringBarley.h:82
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
int GetYearNumber(void)
Definition: Calendar.h:72
Definition: IRSpringBarley.h:75
bool m_first_year
Definition: Farm.h:386
virtual bool Molluscicide(LE *a_field, double a_user, int a_days)
Apply molluscicide to a_field.
Definition: FarmFuncs.cpp:2310
virtual bool StubblePlough(LE *a_field, double a_user, int a_days)
Carry out a stubble ploughing event on a_field. This is similar to normal plough but shallow (normall...
Definition: FarmFuncs.cpp:232
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: IRSpringBarley.h:72
Definition: LandscapeFarmingEnums.h:1006
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
Definition: IRSpringBarley.h:83
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
Definition: IRSpringBarley.h:87
TTypesOfFarm GetType(void)
Definition: Farm.h:956
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
Definition: IRSpringBarley.h:95
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
#define IR_SB_CP
Definition: IRSpringBarley.h:58
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: IRSpringBarley.h:81
Definition: IRSpringBarley.h:77
Definition: IRSpringBarley.h:94
bool value() const
Definition: Configurator.h:164
Definition: IRSpringBarley.h:76
Definition: IRSpringBarley.h:88
Definition: LandscapeFarmingEnums.h:1004
bool m_skylarkscrapes
For management testing of skylark scrapes.
Definition: Elements.h:95
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
Definition: IRSpringBarley.h:71
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: IRSpringBarley.h:93
Definition: LandscapeFarmingEnums.h:1003
void SetUpFarmCategoryInformation()
Definition: IRSpringBarley.h:119
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
int GetRotIndex(void)
Definition: Elements.h:373
Definition: IRSpringBarley.h:74
Definition: IRSpringBarley.h:84
Definition: IRSpringBarley.h:85
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Definition: IRSpringBarley.h:90
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
CfgBool cfg_IR_SpringBarley_SkScrapes("IR_CROP_SB_SK_SCRAPES", CFG_CUSTOM, false)
Definition: IRSpringBarley.h:92
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
Definition: IRSpringBarley.h:80
Definition: IRSpringBarley.h:91
Definition: LandscapeFarmingEnums.h:696
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
Definition: IRSpringBarley.h:89
Definition: IRSpringBarley.h:67
#define IR_SB_MT
A flag used to indicate autumn ploughing status.
Definition: IRSpringBarley.h:57
Definition: IRSpringBarley.h:70
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
Definition: LandscapeFarmingEnums.h:1012
Definition: IRSpringBarley.h:79
Definition: IRSpringBarley.h:73
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: IRSpringBarley.h:86
void SetMConstants(int a, int c)
Definition: Elements.h:408
Definition: IRSpringBarley.h:69
#define IR_SB_BASE
Definition: IRSpringBarley.h:53
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: IRSpringBarley.h:78
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459