File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
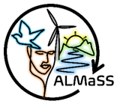 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
FI_SpringBarley_Fodder class
.
More...
#include <FI_SpringBarley_Fodder.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
FI_SpringBarley_Fodder class
.
See FI_SpringBarley_Fodder.h::FI_SpringBarley_FodderToDo for a complete list of all possible events triggered codes by the spring barley Fodder management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ FI_SpringBarley_Fodder()
◆ Do()
bool FI_SpringBarley_Fodder::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with a_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional spring barley Fodder.
Reimplemented from Crop.
108 int l_nextcropstartdate;
117 std::vector<std::vector<int>> flexdates(4 + 1, std::vector<int>(2, 0));
122 flexdates[1][0] = -1;
124 flexdates[2][0] = -1;
126 flexdates[3][0] = -1;
128 flexdates[4][0] = -1;
141 int day_num_shift = 365;
236 if (!a_farm->
FP_NPKS(a_field, 0.0,
363 "Unknown event type! ",
"");
References Farm::AutumnPlough(), cfg_pest_product_amounts, cfg_pest_springbarley_on, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt(), Farm::DoIt_prob(), Farm::FA_Slurry(), fi_sbf_autumn_plough, fi_sbf_fertilizer, fi_sbf_fungicide1, fi_sbf_fungicide2, fi_sbf_growth_reg, fi_sbf_harrow, fi_sbf_harvest, fi_sbf_hay_bailing, fi_sbf_herbicide1, fi_sbf_herbicide2, fi_sbf_insecticide, fi_sbf_preseeding_cultivation, fi_sbf_preseeding_sow, fi_sbf_slurry, fi_sbf_sow, fi_sbf_spring_plough, fi_sbf_start, fi_sbf_straw_chopping, fi_sbf_stubble_cultivator1, fi_sbf_stubble_cultivator2, Farm::FP_NPKS(), Farm::FungicideTreat(), g_date, g_msg, LE::GetMDates(), Farm::GrowthRegulator(), Farm::Harvest(), Farm::HayBailing(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, FarmEvent::m_forcespring, Crop::m_last_date, FarmEvent::m_lock, FarmEvent::m_todo, Calendar::OldDays(), ppp_1, Farm::PreseedingCultivator(), Farm::PreseedingCultivatorSow(), Farm::ProductApplication(), Crop::SimpleEvent(), Crop::SimpleEvent_(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringSowWithFerti(), Crop::StartUpCrop(), Farm::StrawChopping(), Farm::StubbleCultivatorHeavy(), tov_FISpringBarley_Fodder, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void FI_SpringBarley_Fodder::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References FI_SBF_BASE, fi_sbf_foobar, fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, Crop::m_base_elements_no, and Crop::m_ManagementCategories.
Referenced by FI_SpringBarley_Fodder().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: FI_SpringBarley_Fodder.h:72
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
void SetUpFarmCategoryInformation()
Definition: FI_SpringBarley_Fodder.h:114
Definition: LandscapeFarmingEnums.h:1006
Definition: FI_SpringBarley_Fodder.h:79
Definition: FI_SpringBarley_Fodder.h:84
Definition: LandscapeFarmingEnums.h:553
int m_base_elements_no
Definition: Farm.h:505
Definition: FI_SpringBarley_Fodder.h:70
int m_first_date
Definition: Farm.h:501
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: FI_SpringBarley_Fodder.h:86
Definition: FI_SpringBarley_Fodder.h:80
Definition: FI_SpringBarley_Fodder.h:87
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
bool value() const
Definition: Configurator.h:164
Definition: FI_SpringBarley_Fodder.h:78
Definition: FI_SpringBarley_Fodder.h:89
Definition: LandscapeFarmingEnums.h:1004
Definition: FI_SpringBarley_Fodder.h:68
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Definition: FI_SpringBarley_Fodder.h:74
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: FI_SpringBarley_Fodder.h:71
Definition: FI_SpringBarley_Fodder.h:75
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: FI_SpringBarley_Fodder.h:85
Definition: FI_SpringBarley_Fodder.h:82
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool SpringSowWithFerti(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event with start fertilizer in the spring on a_field.
Definition: FarmFuncs.cpp:537
#define FI_SBF_BASE
Definition: FI_SpringBarley_Fodder.h:53
Definition: FI_SpringBarley_Fodder.h:88
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: LandscapeFarmingEnums.h:1012
Definition: FI_SpringBarley_Fodder.h:81
CfgBool cfg_pest_springbarley_on
Turn on pesticides for spring barley.
Definition: FI_SpringBarley_Fodder.h:83
virtual bool GrowthRegulator(LE *a_field, double a_user, int a_days)
Apply growth regulator to a_field.
Definition: FarmFuncs.cpp:2070
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
bool m_forcespringpossible
Used to signal that the crop can be forced to start in spring.
Definition: Farm.h:508
FarmEvent * m_ev
Definition: Farm.h:500
Definition: FI_SpringBarley_Fodder.h:73
Definition: FI_SpringBarley_Fodder.h:76
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: LandscapeFarmingEnums.h:1007
Definition: FI_SpringBarley_Fodder.h:77
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
virtual bool FP_NPKS(LE *a_field, double a_user, int a_days)
Apply NPKS fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:630
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751