File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
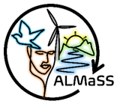 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
#include <FI_Potato_South.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
◆ FI_Potato_South()
◆ Do()
Reimplemented from Crop.
47 int l_nextcropstartdate;
57 std::vector<std::vector<int>> flexdates(1 + 1, std::vector<int>(2, 0));
72 int day_num_shift = 365;
308 #ifdef ECOSTACK_BIOPESTICIDE
317 #ifdef ECOSTACK_BIOPESTICIDE
346 "Unknown event type! ",
"");
References Farm::AutumnPlough(), Farm::BiocideTreat(), cfg_pest_potatoes_on, cfg_pest_product_amounts, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt_prob(), fi_ps_autumn_plough, fi_ps_fertilizer, fi_ps_fungicide1, fi_ps_fungicide2, fi_ps_fungicide3, fi_ps_fungicide4, fi_ps_fungicide5, fi_ps_fungicide6, fi_ps_fungicide7, fi_ps_harrow, fi_ps_harvest, fi_ps_herbicide1, fi_ps_herbicide2, fi_ps_herbicide3, fi_ps_insecticide, fi_ps_n_minerals1, fi_ps_n_minerals2, fi_ps_plant, fi_ps_preseeding_cultivation, fi_ps_preseeding_plant, fi_ps_slurry, fi_ps_spring_plough, fi_ps_start, fi_ps_stubble_cultivator, Farm::FP_NPKS(), Farm::FP_Slurry(), Farm::FungicideTreat(), g_date, g_msg, LE::GetMDates(), Farm::HarvestLong(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, FarmEvent::m_forcespring, Crop::m_last_date, FarmEvent::m_lock, FarmEvent::m_todo, Calendar::OldDays(), ppp_1, Farm::PreseedingCultivator(), Farm::PreseedingCultivatorSow(), Farm::ProductApplication(), LE::SetVegPatchy(), Crop::SimpleEvent(), Crop::SimpleEvent_(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringSow(), Crop::StartUpCrop(), Farm::StubbleCultivatorHeavy(), tov_FIPotato_South, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void FI_Potato_South::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References FI_PS_BASE, fi_ps_foobar, fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, Crop::m_base_elements_no, and Crop::m_ManagementCategories.
Referenced by FI_Potato_South().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
Definition: FI_Potato_South.h:50
Definition: LandscapeFarmingEnums.h:1005
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
void SetUpFarmCategoryInformation()
Definition: FI_Potato_South.h:76
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
bool m_lock
Definition: Farm.h:384
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
Definition: FI_Potato_South.h:45
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: FI_Potato_South.h:42
Definition: FI_Potato_South.h:52
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
Definition: FI_Potato_South.h:38
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
Definition: FI_Potato_South.h:39
int m_base_elements_no
Definition: Farm.h:505
Definition: FI_Potato_South.h:40
int m_first_date
Definition: Farm.h:501
Definition: FI_Potato_South.h:56
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: FI_Potato_South.h:61
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: FI_Potato_South.h:60
bool value() const
Definition: Configurator.h:164
Definition: FI_Potato_South.h:43
Definition: FI_Potato_South.h:37
Definition: FI_Potato_South.h:59
Definition: FI_Potato_South.h:58
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Definition: LandscapeFarmingEnums.h:540
Definition: LandscapeFarmingEnums.h:1003
void SetVegPatchy(bool p)
Definition: Elements.h:229
#define FI_PS_BASE
Definition: FI_Potato_South.h:31
Definition: FI_Potato_South.h:44
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: FI_Potato_South.h:54
Definition: FI_Potato_South.h:48
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: FI_Potato_South.h:53
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
virtual bool HarvestLong(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field (only differs in the DoIt chance cf harvest)
Definition: FarmFuncs.cpp:1421
Definition: FI_Potato_South.h:47
virtual bool BiocideTreat(LE *a_field, double a_user, int a_days)
Apply Biocide to a_field.
Definition: FarmFuncs.cpp:2175
int m_last_date
Definition: Farm.h:503
Definition: FI_Potato_South.h:57
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: FI_Potato_South.h:46
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: FI_Potato_South.h:49
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: LandscapeFarmingEnums.h:1012
Definition: FI_Potato_South.h:51
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
bool m_forcespringpossible
Used to signal that the crop can be forced to start in spring.
Definition: Farm.h:508
CfgBool cfg_pest_potatoes_on
Turn on pesticides for potatoes.
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: FI_Potato_South.h:55
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
virtual bool FP_NPKS(LE *a_field, double a_user, int a_days)
Apply NPKS fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:630
Definition: FI_Potato_South.h:41
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751