Loading [MathJax]/extensions/ams.js
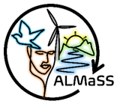 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
FI_OSpringBarley_Fodder class
.
More...
#include <FI_OSpringBarley_Fodder.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
FI_OSpringBarley_Fodder class
.
See FI_OSpringBarley_Fodder.h::FI_OSpringBarley_FodderToDo for a complete list of all possible events triggered codes by the spring barley fodder management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ FI_OSpringBarley_Fodder()
◆ Do()
bool FI_OSpringBarley_Fodder::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with a_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case organic spring barley fodder.
Reimplemented from Crop.
144 g_msg->
Warn(
WARN_BUG,
"FI_OSpringBarley_Fodder::Do(): ",
"Harvest too late for the next crop to start!!!");
145 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
146 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
149 for (
int i = 0; i < noDates; i++) {
166 printf(
"Poly: %d\n", a_field->
GetPoly());
167 g_msg->
Warn(
WARN_BUG,
"FI_OSpringBarley_Fodder::Do(): ",
"Crop start attempt between 1st Jan & 1st July");
170 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
171 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
177 g_msg->
Warn(
WARN_BUG,
"FI_OSpringBarley_Fodder::Do(): ",
"Crop start attempt after last possible start date");
180 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
181 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
276 if (!a_farm->
FP_NPKS(a_field, 0.0,
297 if (!a_farm->
Harvest(a_field, 0.0,
315 else if (a_ev->
m_lock || a_farm->
DoIt(25/25)) {
341 "Unknown event type! ",
"");
References Farm::AutumnPlough(), cfg_FI_OSpringBarley_Fodder_SkScrapes, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), Farm::DoIt(), Farm::FA_Slurry(), FI_OSBF_AUTUMN_PLOUGH, fi_osbf_autumn_plough, FI_OSBF_DECIDE_TO_FI, FI_OSBF_DECIDE_TO_HERB, fi_osbf_fertilizer, fi_osbf_harrow, fi_osbf_harvest, fi_osbf_hay_bailing, fi_osbf_preseeding_cultivation, fi_osbf_preseeding_sow, fi_osbf_slurry, fi_osbf_sow, fi_osbf_spring_plough, fi_osbf_start, fi_osbf_straw_chopping, fi_osbf_stubble_cultivator1, fi_osbf_stubble_cultivator2, Farm::FP_NPKS(), g_date, g_msg, LE::GetMDates(), LE::GetOwner(), LE::GetPoly(), Farm::GetPreviousTov(), LE::GetRotIndex(), Farm::GetType(), Calendar::GetYearNumber(), Farm::Harvest(), Farm::HayBailing(), Farm::IsStockFarmer(), Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, LE::m_skylarkscrapes, FarmEvent::m_startday, FarmEvent::m_todo, Calendar::OldDays(), Farm::PreseedingCultivator(), Farm::PreseedingCultivatorSow(), LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringSowWithFerti(), Farm::StrawChopping(), Farm::StubbleCultivatorHeavy(), tof_OptimisingFarm, CfgBool::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void FI_OSpringBarley_Fodder::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
Definition: FI_OSpringBarley_Fodder.h:71
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
int GetYearNumber(void)
Definition: Calendar.h:72
bool m_first_year
Definition: Farm.h:386
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
class Calendar * g_date
Definition: Calendar.cpp:37
#define FI_OSBF_DECIDE_TO_FI
Definition: FI_OSpringBarley_Fodder.h:55
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
Definition: FI_OSpringBarley_Fodder.h:72
TTypesOfFarm GetType(void)
Definition: Farm.h:956
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
bool value() const
Definition: Configurator.h:164
#define FI_OSBF_AUTUMN_PLOUGH
A flag used to indicate autumn ploughing status.
Definition: FI_OSpringBarley_Fodder.h:53
Definition: LandscapeFarmingEnums.h:1004
#define FI_OSBF_DECIDE_TO_HERB
Definition: FI_OSpringBarley_Fodder.h:54
Definition: FI_OSpringBarley_Fodder.h:66
#define FI_OSBF_BASE
Definition: FI_OSpringBarley_Fodder.h:49
bool m_skylarkscrapes
For management testing of skylark scrapes.
Definition: Elements.h:95
long Date(void)
Definition: Calendar.h:57
Definition: FI_OSpringBarley_Fodder.h:69
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
Definition: FI_OSpringBarley_Fodder.h:68
Definition: FI_OSpringBarley_Fodder.h:70
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
int GetRotIndex(void)
Definition: Elements.h:373
CfgBool cfg_FI_OSpringBarley_Fodder_SkScrapes("DK_CROP_OSBF_SK_SCRAPES", CFG_CUSTOM, false)
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Definition: FI_OSpringBarley_Fodder.h:78
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: FI_OSpringBarley_Fodder.h:73
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
Definition: FI_OSpringBarley_Fodder.h:76
Definition: FI_OSpringBarley_Fodder.h:79
Definition: FI_OSpringBarley_Fodder.h:74
Definition: LandscapeFarmingEnums.h:696
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool SpringSowWithFerti(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event with start fertilizer in the spring on a_field.
Definition: FarmFuncs.cpp:537
Definition: LandscapeFarmingEnums.h:1012
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: FI_OSpringBarley_Fodder.h:64
void SetMConstants(int a, int c)
Definition: Elements.h:408
Definition: FI_OSpringBarley_Fodder.h:77
Definition: FI_OSpringBarley_Fodder.h:75
void SetUpFarmCategoryInformation()
Definition: FI_OSpringBarley_Fodder.h:103
Definition: MapErrorMsg.h:34
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: FI_OSpringBarley_Fodder.h:67
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
virtual bool FP_NPKS(LE *a_field, double a_user, int a_days)
Apply NPKS fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:630