File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
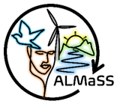 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
#include <DK_WinterRye_CC.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
◆ DK_WinterRye_CC()
◆ Do()
Reimplemented from Crop.
51 int l_nextcropstartdate;
68 std::vector<std::vector<int>> flexdates(1 + 1, std::vector<int>(2, 0));
462 m_farm->
AddNewEvent(
tov_DKCatchCrop,
g_date->
Date(),
m_ev->
m_field,
PROG_START,
m_ev->
m_field->GetRunNum(),
false, l_nextcropstartdate,
false, l_tov,
fmc_Others,
false,
false);
478 "Unknown event type! ",
"");
References Farm::AddNewEvent(), Crop::AphidDamage(), Farm::AutumnPlough(), Farm::AutumnRoll(), cfg_DKCatchCropPct, cfg_pest_product_amounts, cfg_pest_winterrye_on, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), dk_wrcc_autumn_plough, dk_wrcc_autumn_roll, dk_wrcc_autumn_sow, dk_wrcc_ferti_p1, dk_wrcc_ferti_p2, dk_wrcc_ferti_p3, dk_wrcc_ferti_p4, dk_wrcc_ferti_p5, dk_wrcc_ferti_p6, dk_wrcc_ferti_p7, dk_wrcc_ferti_s1, dk_wrcc_ferti_s2, dk_wrcc_ferti_s3, dk_wrcc_ferti_s4, dk_wrcc_ferti_s5, dk_wrcc_ferti_s6, dk_wrcc_ferti_s7, dk_wrcc_fungicide, dk_wrcc_gr1, dk_wrcc_gr2, dk_wrcc_harvest, dk_wrcc_herbicide1, dk_wrcc_herbicide2, dk_wrcc_herbicide3, dk_wrcc_herbicide4, dk_wrcc_herbicide5, dk_wrcc_insecticide, DK_WRCC_MN_P, DK_WRCC_MN_S, dk_wrcc_remove_straw, dk_wrcc_start, dk_wrcc_stubble_harrow, DK_WRCC_TILL_1, DK_WRCC_TILL_2, dk_wrcc_wait, Farm::DoIt_prob(), Farm::FA_Calcium(), Farm::FA_ManganeseSulphate(), Farm::FA_NPK(), Farm::FA_PK(), Farm::FA_Slurry(), fmc_Others, Farm::FP_Calcium(), Farm::FP_ManganeseSulphate(), Farm::FP_NPK(), Farm::FP_PK(), Farm::FP_Slurry(), Farm::FungicideTreat(), g_date, g_msg, LE::GetMDates(), Farm::GetNextCropStartDate(), LE::GetSoilType(), LE::GetUnsprayedMarginPolyRef(), Farm::GrowthRegulator(), Farm::Harvest(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, FarmEvent::m_field, Crop::m_field, Crop::m_last_date, FarmEvent::m_lock, Crop::m_OurLandscape, FarmEvent::m_todo, Calendar::OldDays(), ppp_1, Farm::PreseedingCultivatorSow(), Farm::ProductApplication(), PROG_START, LE::SetVegPatchy(), LE::SetVegType(), Crop::SimpleEvent(), Farm::SleepAllDay(), Crop::StartUpCrop(), Farm::StrawRemoval(), Farm::StubbleHarrowing(), Landscape::SupplyLEPointer(), tos_LoamySand, tos_Sand, tos_SandyClayLoam, tos_SandyLoam, tov_DKCatchCrop, tov_DKOCatchCrop, tov_DKWinterRye_CC, tov_Undefined, CfgFloat::value(), CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void DK_WinterRye_CC::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References DK_WRCC_BASE, dk_wrcc_foobar, fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, Crop::m_base_elements_no, and Crop::m_ManagementCategories.
Referenced by DK_WinterRye_CC().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
#define DK_WRCC_MN_P
Definition: DK_WinterRye_CC.h:34
Definition: DK_WinterRye_CC.h:46
LE * SupplyLEPointer(int a_polyref)
Returns a pointer to the object referred to by the polygon number.
Definition: Landscape.h:1722
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: DK_WinterRye_CC.h:50
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool FP_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply Manganse Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:840
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
virtual bool StrawRemoval(LE *a_field, double a_user, int a_days)
Straw covering applied on a_field.
Definition: FarmFuncs.cpp:1752
Definition: LandscapeFarmingEnums.h:722
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
CfgBool cfg_pest_winterrye_on
Turn on pesticides for winter rye.
Definition: LandscapeFarmingEnums.h:1006
Definition: DK_WinterRye_CC.h:45
Definition: LandscapeFarmingEnums.h:721
int GetNextCropStartDate(LE *a_field, TTypesOfVegetation &a_curr_veg)
Returns the start date of the next crop in the rotation.
Definition: Farm.cpp:920
Definition: DK_WinterRye_CC.h:66
#define DK_WRCC_TILL_1
Definition: DK_WinterRye_CC.h:35
void AddNewEvent(TTypesOfVegetation a_event, long a_date, LE *a_field, int a_todo, long a_num, bool a_lock, int a_start, bool a_first_year, TTypesOfVegetation a_crop, FarmManagementCategory a_fmc, bool a_forcespring, bool a_forcespringOK)
Adds an event to the event queue for a farm.
Definition: Farm.cpp:845
double value() const
Definition: Configurator.h:142
CfgFloat cfg_DKCatchCropPct
Definition: DK_WinterRye_CC.h:47
int m_base_elements_no
Definition: Farm.h:505
Definition: DK_WinterRye_CC.h:44
Definition: DK_WinterRye_CC.h:49
Definition: DK_WinterRye_CC.h:60
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: DK_WinterRye_CC.h:70
Definition: DK_WinterRye_CC.h:67
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: DK_WinterRye_CC.h:69
bool value() const
Definition: Configurator.h:164
Definition: DK_WinterRye_CC.h:40
Definition: DK_WinterRye_CC.h:57
Definition: DK_WinterRye_CC.h:55
Definition: DK_WinterRye_CC.h:68
Definition: LandscapeFarmingEnums.h:421
Definition: LandscapeFarmingEnums.h:1004
Definition: LandscapeFarmingEnums.h:424
Definition: DK_WinterRye_CC.h:61
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
int GetSoilType()
Definition: Elements.h:302
Definition: DK_WinterRye_CC.h:52
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
void SetVegPatchy(bool p)
Definition: Elements.h:229
virtual bool FA_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply manganese sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1095
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
Definition: DK_WinterRye_CC.h:43
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DK_WinterRye_CC.h:41
Definition: DK_WinterRye_CC.h:56
LE * m_field
Definition: Farm.h:391
#define DK_WRCC_MN_S
Definition: DK_WinterRye_CC.h:33
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: DK_WinterRye_CC.h:62
std::vector< double > value() const
Definition: Configurator.h:219
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: LandscapeFarmingEnums.h:719
Definition: LandscapeFarmingEnums.h:610
virtual bool AutumnRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the autumn on a_field.
Definition: FarmFuncs.cpp:299
Definition: DK_WinterRye_CC.h:53
virtual void SetVegType(TTypesOfVegetation)
Definition: Elements.h:175
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
virtual bool SleepAllDay(LE *a_field, double a_user, int a_days)
Nothing to to today on a_field.
Definition: FarmFuncs.cpp:272
Definition: DK_WinterRye_CC.h:58
Definition: DK_WinterRye_CC.h:54
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DK_WinterRye_CC.h:59
Definition: DK_WinterRye_CC.h:51
void SetUpFarmCategoryInformation()
Definition: DK_WinterRye_CC.h:84
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: DK_WinterRye_CC.h:65
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: LandscapeFarmingEnums.h:1012
virtual bool GrowthRegulator(LE *a_field, double a_user, int a_days)
Apply growth regulator to a_field.
Definition: FarmFuncs.cpp:2070
int GetUnsprayedMarginPolyRef(void)
Definition: Elements.h:383
Definition: LandscapeFarmingEnums.h:420
#define DK_WRCC_TILL_2
Definition: DK_WinterRye_CC.h:36
int DayInYear(void)
Definition: Calendar.h:58
Definition: DK_WinterRye_CC.h:42
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
#define PROG_START
Definition: Farm.h:69
bool AphidDamage(LE *a_field)
Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded.
Definition: Farm.cpp:726
Definition: DK_WinterRye_CC.h:64
Definition: DK_WinterRye_CC.h:63
Definition: DK_WinterRye_CC.h:48
FarmEvent * m_ev
Definition: Farm.h:500
Definition: LandscapeFarmingEnums.h:720
Landscape * m_OurLandscape
Definition: Farm.h:506
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
#define DK_WRCC_BASE
Definition: DK_WinterRye_CC.h:31
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
Definition: DK_WinterRye_CC.h:39