File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
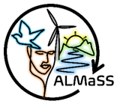 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
#include <DK_OSpringBarley_CC.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
◆ DK_OSpringBarley_CC()
◆ Do()
bool DK_OSpringBarley_CC::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
Reimplemented from Crop.
44 int l_nextcropstartdate;
54 std::vector<std::vector<int>> flexdates(3 + 1, std::vector<int>(2, 0));
74 int day_num_shift = 365;
247 m_farm->
AddNewEvent(
tov_DKOCatchCrop,
g_date->
Date(),
m_ev->
m_field,
PROG_START,
m_ev->
m_field->GetRunNum(),
false, l_nextcropstartdate,
false, l_tov,
fmc_Others,
false,
false);
260 "Unknown event type! ",
"" );
References Farm::AddNewEvent(), cfg_DKCatchCropPct, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), dk_osbmcc_harvest, dk_osbmcc_hay_baling, dk_osbmcc_row_cultivation1, dk_osbmcc_row_cultivation2, dk_osbmcc_shallow_harrow1, dk_osbmcc_shallow_harrow2, dk_osbmcc_slurry_p, dk_osbmcc_slurry_s, dk_osbmcc_spring_harrow1, dk_osbmcc_spring_harrow2, dk_osbmcc_spring_plough, dk_osbmcc_spring_sow, dk_osbmcc_start, dk_osbmcc_straw_chopping, dk_osbmcc_swathing, dk_osbmcc_wait, dk_osbmcc_water, Farm::DoIt(), Farm::DoIt_prob(), Farm::FA_Slurry(), fmc_Others, Farm::FP_Slurry(), g_date, g_msg, LE::GetMDates(), Farm::GetNextCropStartDate(), LE::GetUnsprayedMarginPolyRef(), Farm::Harvest(), Farm::HayBailing(), Farm::IsStockFarmer(), Crop::m_ev, Crop::m_farm, FarmEvent::m_field, Crop::m_field, FarmEvent::m_forcespring, Crop::m_last_date, FarmEvent::m_lock, Crop::m_OurLandscape, FarmEvent::m_todo, Calendar::OldDays(), PROG_START, Farm::RowCultivation(), LE::SetVegPatchy(), LE::SetVegType(), Farm::ShallowHarrow(), Crop::SimpleEvent(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringSow(), Crop::StartUpCrop(), Farm::StrawChopping(), Landscape::SupplyLEPointer(), Farm::Swathing(), tov_DKOCatchCrop, tov_DKOSpringBarley_CC, tov_Undefined, CfgFloat::value(), MapErrorMsg::Warn(), WARN_BUG, and Farm::Water().
◆ SetUpFarmCategoryInformation()
void DK_OSpringBarley_CC::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
LE * SupplyLEPointer(int a_polyref)
Returns a pointer to the object referred to by the polygon number.
Definition: Landscape.h:1722
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: DK_OSpringBarley_CC.h:48
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
void SetUpFarmCategoryInformation()
Definition: DK_OSpringBarley_CC.h:68
int GetNextCropStartDate(LE *a_field, TTypesOfVegetation &a_curr_veg)
Returns the start date of the next crop in the rotation.
Definition: Farm.cpp:920
void AddNewEvent(TTypesOfVegetation a_event, long a_date, LE *a_field, int a_todo, long a_num, bool a_lock, int a_start, bool a_first_year, TTypesOfVegetation a_crop, FarmManagementCategory a_fmc, bool a_forcespring, bool a_forcespringOK)
Adds an event to the event queue for a farm.
Definition: Farm.cpp:845
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
double value() const
Definition: Configurator.h:142
int m_base_elements_no
Definition: Farm.h:505
Definition: DK_OSpringBarley_CC.h:38
int m_first_date
Definition: Farm.h:501
CfgFloat cfg_DKCatchCropPct
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: DK_OSpringBarley_CC.h:34
Definition: DK_OSpringBarley_CC.h:42
Definition: LandscapeFarmingEnums.h:432
Definition: LandscapeFarmingEnums.h:1009
Definition: LandscapeFarmingEnums.h:421
Definition: LandscapeFarmingEnums.h:1004
Definition: DK_OSpringBarley_CC.h:47
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
void SetVegPatchy(bool p)
Definition: Elements.h:229
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
Definition: LandscapeFarmingEnums.h:1011
Definition: DK_OSpringBarley_CC.h:44
Definition: DK_OSpringBarley_CC.h:39
Definition: DK_OSpringBarley_CC.h:35
long OldDays(void)
Definition: Calendar.h:60
Definition: DK_OSpringBarley_CC.h:54
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
#define DK_OSBMCC_BASE
Definition: DK_OSpringBarley_CC.h:31
Definition: DK_OSpringBarley_CC.h:53
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
LE * m_field
Definition: Farm.h:391
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: DK_OSpringBarley_CC.h:49
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: DK_OSpringBarley_CC.h:41
int m_todo
Definition: Farm.h:388
Definition: LandscapeFarmingEnums.h:610
virtual void SetVegType(TTypesOfVegetation)
Definition: Elements.h:175
virtual bool ShallowHarrow(LE *a_field, double a_user, int a_days)
Carry out a shallow harrow event on a_field, e.g., after grass cutting event.
Definition: FarmFuncs.cpp:473
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
virtual bool RowCultivation(LE *a_field, double a_user, int a_days)
Carry out a harrowing between crop rows on a_field.
Definition: FarmFuncs.cpp:1183
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DK_OSpringBarley_CC.h:45
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: DK_OSpringBarley_CC.h:46
Definition: LandscapeFarmingEnums.h:1012
Definition: DK_OSpringBarley_CC.h:40
int GetUnsprayedMarginPolyRef(void)
Definition: Elements.h:383
Definition: DK_OSpringBarley_CC.h:36
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: DK_OSpringBarley_CC.h:37
virtual bool Swathing(LE *a_field, double a_user, int a_days)
Cut the crop on a_field and leave it lying (probably rape)
Definition: FarmFuncs.cpp:1350
#define PROG_START
Definition: Farm.h:69
FarmEvent * m_ev
Definition: Farm.h:500
Landscape * m_OurLandscape
Definition: Farm.h:506
Definition: MapErrorMsg.h:34
Definition: DK_OSpringBarley_CC.h:43
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459