Loading [MathJax]/extensions/ams.js
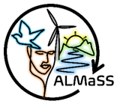 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
#include <DK_OCerealLegume_Whole.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
◆ DK_OCerealLegume_Whole()
◆ Do()
bool DK_OCerealLegume_Whole::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
Reimplemented from Crop.
53 std::vector<std::vector<int>> flexdates(1 + 1, std::vector<int>(2, 0));
257 "Unknown event type! ",
"" );
References Farm::AutumnPlough(), LE::ClearManagementActionSum(), Farm::CutToHay(), Calendar::Date(), Calendar::DayInYear(), dk_oclw_autumn_plough, dk_oclw_cutting1, dk_oclw_cutting2, dk_oclw_harvest, dk_oclw_harvest_lo, dk_oclw_manure1_p, dk_oclw_manure1_s, dk_oclw_manure2_p, dk_oclw_manure2_s, dk_oclw_spring_harrow, dk_oclw_spring_plough, dk_oclw_spring_sow, dk_oclw_spring_sow_lo, dk_oclw_start, dk_oclw_strigling1, dk_oclw_strigling2, dk_oclw_water, dk_oclw_water_lo, Farm::DoIt_prob(), Farm::FA_Manure(), Farm::FP_Manure(), g_date, g_msg, LE::GetMDates(), Farm::Harvest(), Farm::IsStockFarmer(), Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_last_date, FarmEvent::m_lock, FarmEvent::m_todo, Calendar::OldDays(), Crop::SimpleEvent(), Farm::SpringHarrow(), Farm::SpringPlough(), Farm::SpringSow(), Crop::StartUpCrop(), Farm::Strigling(), tov_DKOCerealLegume_Whole, MapErrorMsg::Warn(), WARN_BUG, and Farm::Water().
◆ SetUpFarmCategoryInformation()
void DK_OCerealLegume_Whole::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: DK_OCerealLegume_Whole.h:53
void SetUpFarmCategoryInformation()
Definition: DK_OCerealLegume_Whole.h:68
Definition: DK_OCerealLegume_Whole.h:48
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Strigling(LE *a_field, double a_user, int a_days)
Carry out a mechanical weeding on a_field.
Definition: FarmFuncs.cpp:1206
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
Definition: DK_OCerealLegume_Whole.h:54
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: DK_OCerealLegume_Whole.h:52
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: DK_OCerealLegume_Whole.h:39
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
Definition: DK_OCerealLegume_Whole.h:49
Definition: DK_OCerealLegume_Whole.h:50
int m_base_elements_no
Definition: Farm.h:505
Definition: DK_OCerealLegume_Whole.h:47
virtual bool CutToHay(LE *a_field, double a_user, int a_days)
Carry out hay cutting on a_field.
Definition: FarmFuncs.cpp:1607
int m_first_date
Definition: Farm.h:501
Definition: DK_OCerealLegume_Whole.h:43
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: LandscapeFarmingEnums.h:1009
Definition: LandscapeFarmingEnums.h:1004
Definition: DK_OCerealLegume_Whole.h:51
long Date(void)
Definition: Calendar.h:57
Definition: DK_OCerealLegume_Whole.h:40
Definition: DK_OCerealLegume_Whole.h:41
Definition: LandscapeFarmingEnums.h:1008
Definition: LandscapeFarmingEnums.h:1003
Definition: LandscapeFarmingEnums.h:1011
long OldDays(void)
Definition: Calendar.h:60
Definition: DK_OCerealLegume_Whole.h:37
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
Definition: DK_OCerealLegume_Whole.h:34
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: DK_OCerealLegume_Whole.h:42
Definition: DK_OCerealLegume_Whole.h:36
Definition: LandscapeFarmingEnums.h:1012
Definition: DK_OCerealLegume_Whole.h:35
Definition: DK_OCerealLegume_Whole.h:38
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
#define DK_OCLW_BASE
Definition: DK_OCerealLegume_Whole.h:31
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: DK_OCerealLegume_Whole.h:44
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
Definition: LandscapeFarmingEnums.h:368