File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
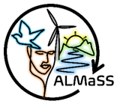 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
DE_WinterWheat class
.
More...
#include <DE_WinterWheat.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
DE_WinterWheat class
.
See DE_WinterWheat.h::DE_WinterWheatToDo for a complete list of all possible events triggered codes by the WinterWheat management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ DE_WinterWheat()
◆ Do()
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with m_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional winter wheat.
Reimplemented from Crop.
115 std::vector<std::vector<int>> flexdates(2 + 1, std::vector<int>(2, 0));
120 flexdates[1][0] = -1;
122 flexdates[2][0] = -1;
231 #ifdef ECOSTACK_BIOPESTICIDE
246 #ifdef ECOSTACK_BIOPESTICIDE
264 #ifdef ECOSTACK_BIOPESTICIDE
274 #ifdef ECOSTACK_BIOPESTICIDE
288 #ifdef ECOSTACK_BIOPESTICIDE
299 #ifdef ECOSTACK_BIOPESTICIDE
309 #ifdef ECOSTACK_BIOPESTICIDE
481 "Unknown event type! ",
"");
References Farm::AutumnPlough(), Farm::AutumnSow(), Farm::BiocideTreat(), cfg_pest_product_amounts, cfg_pest_winterwheat_on, LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), de_ww_autumn_harrow_notill, DE_WW_AUTUMN_PLOUGH, de_ww_autumn_plough, de_ww_autumn_sow, DE_WW_DECIDE_TO_GR, de_ww_ferti_p1, de_ww_ferti_p2, de_ww_ferti_p3, de_ww_ferti_p4, de_ww_ferti_s1, de_ww_ferti_s2, de_ww_ferti_s3, de_ww_ferti_s4, de_ww_fungicide1, de_ww_fungicide2, de_ww_growth_regulator1, de_ww_growth_regulator2, de_ww_harvest, de_ww_hay_bailing, de_ww_herbicide1, de_ww_herbicide2, de_ww_insecticide1, de_ww_insecticide2, de_ww_insecticide3, de_ww_start, de_ww_straw_chopping, Farm::DoIt(), Farm::DoIt_prob(), Farm::FA_AmmoniumSulphate(), Farm::FA_PK(), Farm::FP_AmmoniumSulphate(), Farm::FP_PK(), Farm::FungicideTreat(), g_date, g_landscape_ptr, g_msg, LE::GetGreenBiomass(), LE::GetMDates(), Farm::GrowthRegulator(), Farm::Harvest(), Farm::HayBailing(), Farm::HerbicideTreat(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_last_date, FarmEvent::m_lock, FarmEvent::m_todo, Calendar::OldDays(), ppp_1, Farm::ProductApplication(), Farm::ShallowHarrow(), Crop::SimpleEvent(), Crop::SimpleEvent_(), Crop::StartUpCrop(), Farm::StrawChopping(), Landscape::SupplyPestIncidenceFactor(), tov_DEWinterWheat, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void DE_WinterWheat::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
References DE_WINTERWHEAT_BASE, de_ww_foobar, fmc_Cultivation, fmc_Fertilizer, fmc_Fungicide, fmc_Harvest, fmc_Herbicide, fmc_Insecticide, fmc_Others, Crop::m_base_elements_no, and Crop::m_ManagementCategories.
Referenced by DE_WinterWheat().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: LandscapeFarmingEnums.h:1005
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
Definition: DE_WinterWheat.h:75
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
bool m_lock
Definition: Farm.h:384
Definition: DE_WinterWheat.h:66
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: DE_WinterWheat.h:74
Definition: DE_WinterWheat.h:77
Definition: DE_WinterWheat.h:92
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
#define DE_WW_DECIDE_TO_GR
Definition: DE_WinterWheat.h:58
Definition: DE_WinterWheat.h:83
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
Definition: DE_WinterWheat.h:71
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: DE_WinterWheat.h:73
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
bool value() const
Definition: Configurator.h:164
Definition: DE_WinterWheat.h:93
#define DE_WINTERWHEAT_BASE
Definition: DE_WinterWheat.h:51
Definition: DE_WinterWheat.h:68
#define DE_WW_AUTUMN_PLOUGH
A flag used to indicate autumn ploughing status.
Definition: DE_WinterWheat.h:55
Definition: DE_WinterWheat.h:94
Definition: LandscapeFarmingEnums.h:1004
Definition: DE_WinterWheat.h:87
Definition: DE_WinterWheat.h:96
Definition: DE_WinterWheat.h:78
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
Definition: DE_WinterWheat.h:80
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
long OldDays(void)
Definition: Calendar.h:60
Definition: DE_WinterWheat.h:89
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DE_WinterWheat.h:91
double SupplyPestIncidenceFactor()
Returns Landscape::m_pestincidencefactor.
Definition: Landscape.h:325
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
Definition: DE_WinterWheat.h:69
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
virtual bool ShallowHarrow(LE *a_field, double a_user, int a_days)
Carry out a shallow harrow event on a_field, e.g., after grass cutting event.
Definition: FarmFuncs.cpp:473
Definition: DE_WinterWheat.h:88
virtual bool BiocideTreat(LE *a_field, double a_user, int a_days)
Apply Biocide to a_field.
Definition: FarmFuncs.cpp:2175
void SetUpFarmCategoryInformation()
Definition: DE_WinterWheat.h:122
Definition: DE_WinterWheat.h:86
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: LandscapeFarmingEnums.h:509
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
Definition: LandscapeFarmingEnums.h:1012
Definition: DE_WinterWheat.h:84
Definition: DE_WinterWheat.h:85
virtual bool GrowthRegulator(LE *a_field, double a_user, int a_days)
Apply growth regulator to a_field.
Definition: FarmFuncs.cpp:2070
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: DE_WinterWheat.h:76
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
Landscape * g_landscape_ptr
Definition: Landscape.cpp:352
Definition: DE_WinterWheat.h:95
Definition: DE_WinterWheat.h:79
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
CfgBool cfg_pest_winterwheat_on
Turn on pesticides for winter wheat.
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751