Loading [MathJax]/extensions/ams.js
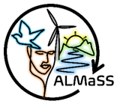 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
DE_OWinterBarley class
.
More...
#include <DE_OWinterBarley.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
DE_OWinterBarley class
.
See DE_OWinterBarley.h::DE_OWinterBarleyToDo for a complete list of all possible events triggered codes by the winter rye management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ DE_OWinterBarley()
◆ Do()
bool DE_OWinterBarley::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
Reimplemented from Crop.
102 std::vector<std::vector<int>> flexdates(6 + 1, std::vector<int>(2, 0));
107 flexdates[1][0] = -1;
113 flexdates[4][0] = -1;
115 flexdates[5][0] = -1;
117 flexdates[6][0] = -1;
188 if (newdate2 > newdate1)
285 if (d2 > d1) d1 = d2;
336 "Unknown event type! ",
"");
References Farm::AutumnPlough(), Farm::AutumnSow(), LE::ClearManagementActionSum(), Calendar::Date(), Calendar::DayInYear(), de_owb_autumn_harrow, de_owb_autumn_plough, de_owb_autumn_sow, de_owb_deep_plough, de_owb_ferti_p1, de_owb_ferti_s1, de_owb_ferti_s2, de_owb_harvest, de_owb_hay_bailing, de_owb_hay_turning, de_owb_spring_roll1, de_owb_start, de_owb_straw_chopping, de_owb_strigling1, de_owb_strigling2, de_owb_strigling3, de_owb_stubble_harrow1, de_owb_stubble_harrow2, Farm::DeepPlough(), Farm::DoIt(), Farm::DoIt_prob(), Farm::FA_Manure(), Farm::FA_Slurry(), Farm::FP_Manure(), g_date, g_msg, LE::GetMDates(), Farm::Harvest(), Farm::HayBailing(), Farm::HayTurning(), Farm::IsStockFarmer(), Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_last_date, FarmEvent::m_lock, FarmEvent::m_todo, Calendar::OldDays(), Crop::SimpleEvent_(), Farm::SpringRoll(), Crop::StartUpCrop(), Farm::StrawChopping(), Farm::Strigling(), Farm::StubbleHarrowing(), tov_DEOWinterBarley, MapErrorMsg::Warn(), and WARN_BUG.
◆ SetUpFarmCategoryInformation()
void DE_OWinterBarley::SetUpFarmCategoryInformation |
( |
| ) |
|
|
inline |
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: DE_OWinterBarley.h:81
virtual bool SpringRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the spring on a_field.
Definition: FarmFuncs.cpp:487
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
#define DE_OWINTERBARLEY_BASE
Definition: DE_OWinterBarley.h:53
Definition: DE_OWinterBarley.h:68
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: DE_OWinterBarley.h:77
virtual bool Strigling(LE *a_field, double a_user, int a_days)
Carry out a mechanical weeding on a_field.
Definition: FarmFuncs.cpp:1206
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
Definition: DE_OWinterBarley.h:70
bool m_lock
Definition: Farm.h:384
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
Definition: DE_OWinterBarley.h:84
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
Definition: DE_OWinterBarley.h:82
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: DE_OWinterBarley.h:72
Definition: LandscapeFarmingEnums.h:1009
Definition: LandscapeFarmingEnums.h:1004
Definition: DE_OWinterBarley.h:80
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: DE_OWinterBarley.h:75
Definition: LandscapeFarmingEnums.h:1003
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: DE_OWinterBarley.h:67
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DE_OWinterBarley.h:79
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: DE_OWinterBarley.h:85
int m_todo
Definition: Farm.h:388
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: LandscapeFarmingEnums.h:494
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: DE_OWinterBarley.h:74
virtual bool DeepPlough(LE *a_field, double a_user, int a_days)
Carry out a deep ploughing event on a_field.
Definition: FarmFuncs.cpp:408
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
Definition: DE_OWinterBarley.h:78
Definition: LandscapeFarmingEnums.h:1012
Definition: DE_OWinterBarley.h:73
int DayInYear(void)
Definition: Calendar.h:58
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: DE_OWinterBarley.h:65
Definition: DE_OWinterBarley.h:83
Definition: DE_OWinterBarley.h:76
void SetUpFarmCategoryInformation()
Definition: DE_OWinterBarley.h:111
virtual bool HayTurning(LE *a_field, double a_user, int a_days)
Carry out hay turning on a_field.
Definition: FarmFuncs.cpp:1491
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
Definition: DE_OWinterBarley.h:87
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751