File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
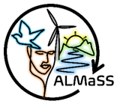 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
BEPotatoesSpring class
.
More...
#include <BEPotatoesSpring.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
BEPotatoesSpring class
.
See BEPotatoesSpring.h::BEPotatoesSpringToDo for a complete list of all possible events triggered codes by the potatoes management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ BEPotatoesSpring()
◆ Do()
bool BEPotatoesSpring::Do |
( |
Farm * |
a_farm, |
|
|
LE * |
a_field, |
|
|
FarmEvent * |
a_ev |
|
) |
| |
|
virtual |
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with a_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional potatoes.
Reimplemented from Crop.
113 g_msg->
Warn(
WARN_BUG,
"BEPotatoesSpring::Do(): ",
"Harvest too late for the next crop to start!!!");
114 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
115 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
118 for (
int i = 0; i < noDates; i++) {
134 g_msg->
Warn(
WARN_BUG,
"BEPotatoesSpring::Do(): ",
"Crop start attempt after last possible start date");
137 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
138 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
197 if (a_ev->
m_lock || a_farm->DoIt_prob(0.50))
236 if (a_farm->IsStockFarmer())
241 if (a_farm->IsStockFarmer())
250 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
260 if (a_farm->IsStockFarmer())
269 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
279 if (a_farm->IsStockFarmer())
302 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
312 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
329 if (a_ev->
m_lock || a_farm->DoIt_prob(0.80))
348 if (a_ev->
m_lock || a_farm->DoIt_prob(0.75))
407 if (a_ev->
m_lock || a_farm->DoIt_prob(0.80))
492 #ifdef ECOSTACK_BIOPESTICIDE
493 if (a_ev->
m_lock || a_farm->DoIt_prob(0.80))
495 if (a_ev->
m_lock || a_farm->DoIt_prob(0.40))
502 #ifdef ECOSTACK_BIOPESTICIDE
530 "Unknown event type! ",
"");
References BE_pots_bed_forming, BE_pots_dessication1, BE_pots_dessication2, BE_pots_ferti_p2_clay, BE_pots_ferti_p2_sandy, BE_pots_ferti_p3_clay, BE_pots_ferti_p3_sandy, BE_pots_ferti_p4, BE_pots_ferti_s2_clay, BE_pots_ferti_s2_sandy, BE_pots_ferti_s3_clay, BE_pots_ferti_s3_sandy, BE_pots_ferti_s4, BE_POTS_FUNGI1, BE_POTS_FUNGI2, BE_POTS_FUNGI3, BE_POTS_FUNGI4, BE_POTS_FUNGI5, BE_pots_fungicide1, BE_pots_fungicide10, BE_pots_fungicide11, BE_pots_fungicide12, BE_pots_fungicide13, BE_pots_fungicide14, BE_pots_fungicide15, BE_pots_fungicide2, BE_pots_fungicide3, BE_pots_fungicide4, BE_pots_fungicide5, BE_pots_fungicide6, BE_pots_fungicide7, BE_pots_fungicide8, BE_pots_fungicide9, BE_pots_harvest, BE_POTS_HERBI, BE_pots_herbicide1, BE_pots_hilling1, BE_pots_insecticide, BE_pots_spring_planting, BE_pots_spring_plough_sandy, BE_pots_start, Farm::BiocideTreat(), cfg_pest_potatoes_on, cfg_pest_product_amounts, Calendar::Date(), Calendar::DayInYear(), Farm::DoIt_prob(), Farm::FP_NPK(), g_date, g_msg, LE::GetMDates(), LE::GetOwner(), Farm::GetPreviousTov(), LE::GetRotIndex(), LE::GetSoilType(), Farm::GetType(), Calendar::GetYearNumber(), Farm::InsecticideTreat(), Farm::IsStockFarmer(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, FarmEvent::m_startday, FarmEvent::m_todo, Calendar::OldDays(), ppp_1, Farm::ProductApplication(), LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent_(), Farm::SpringPlough(), tof_OptimisingFarm, tov_BEPotatoesSpring, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), and WARN_BUG.
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: BEPotatoesSpring.h:73
CfgBool cfg_pest_potatoes_on
Turn on pesticides for potatoes.
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
Definition: BEPotatoesSpring.h:50
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: BEPotatoesSpring.h:60
bool m_lock
Definition: Farm.h:384
Definition: BEPotatoesSpring.h:52
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
int GetYearNumber(void)
Definition: Calendar.h:72
bool m_first_year
Definition: Farm.h:386
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
Definition: BEPotatoesSpring.h:61
TTypesOfFarm GetType(void)
Definition: Farm.h:956
Definition: BEPotatoesSpring.h:63
Definition: BEPotatoesSpring.h:48
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
Definition: BEPotatoesSpring.h:67
Definition: BEPotatoesSpring.h:54
#define BE_POTS_FUNGI2
Definition: BEPotatoesSpring.h:30
Definition: BEPotatoesSpring.h:51
bool value() const
Definition: Configurator.h:164
Definition: BEPotatoesSpring.h:43
Definition: BEPotatoesSpring.h:72
Definition: BEPotatoesSpring.h:44
long Date(void)
Definition: Calendar.h:57
Definition: BEPotatoesSpring.h:66
int GetSoilType()
Definition: Elements.h:302
#define BE_POTS_FUNGI3
Definition: BEPotatoesSpring.h:31
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
int GetRotIndex(void)
Definition: Elements.h:373
Definition: BEPotatoesSpring.h:68
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: BEPotatoesSpring.h:75
std::vector< double > value() const
Definition: Configurator.h:219
Definition: BEPotatoesSpring.h:65
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: BEPotatoesSpring.h:74
virtual bool BiocideTreat(LE *a_field, double a_user, int a_days)
Apply Biocide to a_field.
Definition: FarmFuncs.cpp:2175
Definition: BEPotatoesSpring.h:69
Definition: BEPotatoesSpring.h:57
Definition: LandscapeFarmingEnums.h:696
#define BE_POTS_FUNGI4
Definition: BEPotatoesSpring.h:32
Definition: BEPotatoesSpring.h:41
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: BEPotatoesSpring.h:49
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: BEPotatoesSpring.h:55
Definition: BEPotatoesSpring.h:46
Definition: BEPotatoesSpring.h:47
#define BE_POTS_FUNGI1
Definition: BEPotatoesSpring.h:29
Definition: LandscapeFarmingEnums.h:300
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: BEPotatoesSpring.h:59
#define BE_POTS_FUNGI5
Definition: BEPotatoesSpring.h:33
Definition: BEPotatoesSpring.h:76
Definition: BEPotatoesSpring.h:45
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
#define BE_POTS_HERBI
A flag used to indicate autumn ploughing status.
Definition: BEPotatoesSpring.h:28
Definition: BEPotatoesSpring.h:64
Definition: BEPotatoesSpring.h:56
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: BEPotatoesSpring.h:53
Definition: BEPotatoesSpring.h:58
void SetMConstants(int a, int c)
Definition: Elements.h:408
Definition: BEPotatoesSpring.h:71
FarmEvent * m_ev
Definition: Farm.h:500
Definition: BEPotatoesSpring.h:70
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751
Definition: BEPotatoesSpring.h:62