File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
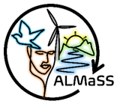 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
BEPotatoes class
.
More...
#include <BEPotatoes.h>
|
TTypesOfVegetation | m_tov |
|
void | SimpleEvent (long a_date, int a_todo, bool a_lock) |
| Adds an event to this crop management. More...
|
|
void | SimpleEvent_ (long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field) |
| Adds an event to this crop management without relying on member variables. More...
|
|
bool | StartUpCrop (int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo) |
|
Holds the translation between the farm operation enum for each cropand the farm management category associated with it More...
|
|
bool | AphidDamage (LE *a_field) |
| Compares aphid numbers per m2 with a threshold to return true if threshold is exceeded. More...
|
|
Farm * | m_farm |
|
LE * | m_field |
|
FarmEvent * | m_ev |
|
int | m_first_date |
|
int | m_count |
|
int | m_last_date |
|
int | m_ddegstoharvest |
|
int | m_base_elements_no |
|
Landscape * | m_OurLandscape |
|
bool | m_forcespringpossible = false |
| Used to signal that the crop can be forced to start in spring. More...
|
|
TTypesOfCrops | m_toc |
| The Crop type in terms of the TTypesOfCrops list (smaller list than tov, no country designation) More...
|
|
int | m_CropClassification |
| Contains information on whether this is a winter crop, spring crop, or catch crop that straddles the year boundary (0,1,2) More...
|
|
vector< FarmManagementCategory > | m_ManagementCategories |
| Holds the translation between the farm operation enum for each crop and the farm management category associated with it. More...
|
|
static int | m_date_modifier = 0 |
| Holds a value that shifts test pesticide use by this many days in crops modified to use it. More...
|
|
BEPotatoes class
.
See BEPotatoes.h::BEPotatoesToDo for a complete list of all possible events triggered codes by the potatoes management plan. When triggered these events are handled by Farm and are available as information for other objects such as animal and bird models.
◆ BEPotatoes()
◆ Do()
The one and only method for a crop management plan. All farm actions go through here.
Called every time something is done to the crop by the farmer in the first instance it is always called with a_ev->todo set to start, but susequently will be called whenever the farmer wants to carry out a new operation.
This method details all the management and relationships between operations necessary to grow and ALMaSS crop - in this case conventional potatoes.
Reimplemented from Crop.
113 g_msg->
Warn(
WARN_BUG,
"BEPotatoes::Do(): ",
"Harvest too late for the next crop to start!!!");
114 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
115 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
118 for (
int i = 0; i < noDates; i++) {
135 printf(
"Poly: %d\n", a_field->
GetPoly());
136 g_msg->
Warn(
WARN_BUG,
"BEPotatoes::Do(): ",
"Crop start attempt between 1st Jan & 1st July");
139 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
140 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
147 g_msg->
Warn(
WARN_BUG,
"BEPotatoes::Do(): ",
"Crop start attempt after last possible start date");
150 int almassnum = a_field->GetLandscape()->BackTranslateVegTypes(a_ev->
m_next_tov);
151 g_msg->
Warn(
"Next Crop ", (
double)almassnum);
209 if (a_farm->IsStockFarmer())
229 if (a_farm->IsStockFarmer())
236 if (a_ev->
m_lock || a_farm->DoIt_prob(0.50))
246 if (a_ev->
m_lock || a_farm->DoIt_prob(0.50))
256 if (a_ev->
m_lock || a_farm->DoIt_prob(0.05))
263 if (a_farm->IsStockFarmer())
299 if (a_farm->IsStockFarmer())
304 if (a_farm->IsStockFarmer())
313 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
323 if (a_farm->IsStockFarmer())
332 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
342 if (a_farm->IsStockFarmer())
365 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
375 if (a_ev->
m_lock || a_farm->DoIt_prob(0.25))
392 if (a_ev->
m_lock || a_farm->DoIt_prob(0.80))
411 if (a_ev->
m_lock || a_farm->DoIt_prob(0.75))
470 if (a_ev->
m_lock || a_farm->DoIt_prob(0.80))
555 #ifdef ECOSTACK_BIOPESTICIDE
556 if (a_ev->
m_lock || a_farm->DoIt_prob(0.80))
558 if (a_ev->
m_lock || a_farm->DoIt_prob(0.40))
565 #ifdef ECOSTACK_BIOPESTICIDE
593 "Unknown event type! ",
"");
References BE_pot_bed_forming, BE_pot_dessication1, BE_pot_dessication2, BE_pot_ferti_p1_sandy, BE_pot_ferti_p2_clay, BE_pot_ferti_p2_sandy, BE_pot_ferti_p3_clay, BE_pot_ferti_p3_sandy, BE_pot_ferti_p4, BE_pot_ferti_s1_sandy, BE_pot_ferti_s2_clay, BE_pot_ferti_s2_sandy, BE_pot_ferti_s3_clay, BE_pot_ferti_s3_sandy, BE_pot_ferti_s4, BE_POT_FUNGI1, BE_POT_FUNGI2, BE_POT_FUNGI3, BE_POT_FUNGI4, BE_POT_FUNGI5, BE_pot_fungicide1, BE_pot_fungicide10, BE_pot_fungicide11, BE_pot_fungicide12, BE_pot_fungicide13, BE_pot_fungicide14, BE_pot_fungicide15, BE_pot_fungicide2, BE_pot_fungicide3, BE_pot_fungicide4, BE_pot_fungicide5, BE_pot_fungicide6, BE_pot_fungicide7, BE_pot_fungicide8, BE_pot_fungicide9, BE_pot_harvest, BE_POT_HERBI, BE_pot_herbicide1, BE_pot_hilling1, BE_pot_insecticide, BE_pot_spring_planting, BE_pot_spring_plough_sandy, BE_pot_start, BE_pot_stubble_harrow, BE_pot_winter_plough_clay, BE_pot_winter_plough_sandy, Farm::BiocideTreat(), cfg_pest_potatoes_on, cfg_pest_product_amounts, Calendar::Date(), Calendar::DayInYear(), Farm::DoIt_prob(), g_date, g_msg, LE::GetMDates(), LE::GetOwner(), LE::GetPoly(), Farm::GetPreviousTov(), LE::GetRotIndex(), LE::GetSoilType(), Farm::GetType(), Calendar::GetYearNumber(), Farm::InsecticideTreat(), Crop::m_date_modifier, Crop::m_ev, Crop::m_farm, Crop::m_field, Crop::m_first_date, FarmEvent::m_first_year, FarmEvent::m_lock, FarmEvent::m_next_tov, FarmEvent::m_startday, FarmEvent::m_todo, Calendar::OldDays(), ppp_1, Farm::ProductApplication(), LE::SetMConstants(), LE::SetMDates(), Crop::SimpleEvent_(), Farm::StubbleHarrowing(), tof_OptimisingFarm, tov_BEPotatoes, CfgBool::value(), CfgArray_Double::value(), MapErrorMsg::Warn(), WARN_BUG, and Farm::WinterPlough().
The documentation for this class was generated from the following files:
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: BEPotatoes.h:80
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
Definition: BEPotatoes.h:68
bool m_lock
Definition: Farm.h:384
Definition: BEPotatoes.h:75
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
CfgBool cfg_pest_potatoes_on
Turn on pesticides for potatoes.
Definition: BEPotatoes.h:50
int GetYearNumber(void)
Definition: Calendar.h:72
bool m_first_year
Definition: Farm.h:386
Definition: BEPotatoes.h:73
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
Definition: BEPotatoes.h:76
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: BEPotatoes.h:56
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
TTypesOfFarm GetType(void)
Definition: Farm.h:956
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
#define BE_POT_FUNGI1
Definition: BEPotatoes.h:29
Definition: BEPotatoes.h:78
Definition: BEPotatoes.h:63
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
#define BE_POT_FUNGI3
Definition: BEPotatoes.h:31
#define BE_POT_FUNGI2
Definition: BEPotatoes.h:30
Definition: BEPotatoes.h:48
bool value() const
Definition: Configurator.h:164
Definition: LandscapeFarmingEnums.h:299
#define BE_POT_FUNGI5
Definition: BEPotatoes.h:33
Definition: BEPotatoes.h:62
Definition: BEPotatoes.h:70
Definition: BEPotatoes.h:69
Definition: BEPotatoes.h:54
long Date(void)
Definition: Calendar.h:57
int GetSoilType()
Definition: Elements.h:302
Definition: BEPotatoes.h:81
long OldDays(void)
Definition: Calendar.h:60
Definition: BEPotatoes.h:45
Definition: BEPotatoes.h:52
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
int GetRotIndex(void)
Definition: Elements.h:373
#define BE_POT_FUNGI4
Definition: BEPotatoes.h:32
#define BE_POT_HERBI
A flag used to indicate autumn ploughing status.
Definition: BEPotatoes.h:28
Definition: BEPotatoes.h:66
Definition: BEPotatoes.h:59
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
std::vector< double > value() const
Definition: Configurator.h:219
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: BEPotatoes.h:41
Definition: BEPotatoes.h:74
Definition: BEPotatoes.h:72
virtual bool BiocideTreat(LE *a_field, double a_user, int a_days)
Apply Biocide to a_field.
Definition: FarmFuncs.cpp:2175
Definition: BEPotatoes.h:64
Definition: LandscapeFarmingEnums.h:696
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: BEPotatoes.h:57
Definition: BEPotatoes.h:55
Definition: BEPotatoes.h:77
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: BEPotatoes.h:58
Definition: BEPotatoes.h:71
Definition: BEPotatoes.h:47
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: BEPotatoes.h:67
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
Definition: BEPotatoes.h:65
Definition: BEPotatoes.h:51
Definition: BEPotatoes.h:49
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Definition: BEPotatoes.h:60
Definition: BEPotatoes.h:46
Definition: BEPotatoes.h:43
Crop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: Farm.cpp:733
Definition: BEPotatoes.h:79
Definition: BEPotatoes.h:53
void SetMConstants(int a, int c)
Definition: Elements.h:408
FarmEvent * m_ev
Definition: Farm.h:500
Definition: BEPotatoes.h:44
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: BEPotatoes.h:61
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751