File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
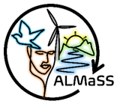 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
31 #ifndef POLLEN_NECTAR_H
32 #define POLLEN_NECTAR_H
37 #include <Eigen/Dense>
117 double getResource(
int a_given_day, vector<double>& a_resource_per_phase) {
124 if(a_resource_per_phase[i] < 0)
return 0;
125 return a_resource_per_phase[i];
vector< bool > m_tov_PollenCurveTable_sowing_flag
This is a look-up table for the the crops to indicate it starts to accumulate degree days from sowing...
Definition: PollenNectar.h:188
void SetEndingDayFlowering(int a_ending_day_flowering)
Set the ending day of flowering period.
Definition: PollenNectar.h:157
vector< double > m_pollen_per_phase
The array to store the pollen in different period, the size is the number of the flowering period pha...
Definition: PollenNectar.h:91
int m_num_species_habitat
This is used to store the number of species for habitat.
Definition: PollenNectar.h:190
int m_starting_day_flowering
The starting day number for flowering period in a year.
Definition: PollenNectar.h:79
PollenNectarDevelopmentData(string a_toleinputfile="default", string a_tovinputfile="default")
Definition: PollenNectar.cpp:98
PollenNectarData supplyPollenHabitatSpeciesGivenDay(int a_curve_num, int a_given_day)
Supply the pollen for the given species for the given day after today.
Definition: PollenNectar.cpp:345
PollenNectarDevelopmentCurveSet()
Definition: PollenNectar.cpp:57
double getNectar(int a_given_day)
Returns the nectar quantity for the given day after day 0 in a year.
Definition: PollenNectar.h:96
int GetEndingDayFlowering(void)
Returns the ending day of flowering period.
Definition: PollenNectar.h:153
double m_ending_DD_flowering
The ending degree days for ending flowering period.
Definition: PollenNectar.h:69
double m_MinFlowerLength
The minimum flowering length.
Definition: PollenNectar.h:59
double m_starting_DD_flowering
The starting degree days for starting flowering period.
Definition: PollenNectar.h:67
Definition: PopulationManager.h:73
char * value() const
Definition: Configurator.h:182
double m_MeanFlowerLength
The mean flowering length.
Definition: PollenNectar.h:63
Definition: PopulationManager.h:69
double m_MaxFlowerLength
The maximum flowering length.
Definition: PollenNectar.h:61
vector< int > m_tov_PollenCurveTable
This is a look-up table, it contains for each tov the corresponding curve number to use.
Definition: PollenNectar.h:186
int GetStartingDayFlowering(void)
Returns the starting day of flowering period.
Definition: PollenNectar.h:151
String configurator entry class.
Definition: Configurator.h:173
double m_MaxDDeg
The maximum temperature that one day can accumulate.
Definition: PollenNectar.h:57
class Calendar * g_date
Definition: Calendar.cpp:37
This class justs holds the set of resource curves related to a specific plant community or crop....
Definition: PollenNectar.h:44
PollenNectarData supplyNectarHabitatSpeciesCurrentDay(int a_curve_num)
Supply the current nectar for the given species.
Definition: PollenNectar.cpp:338
double value() const
Definition: Configurator.h:142
int m_curve_number
The reference number for the curve.
Definition: PollenNectar.h:53
double GetMaxDDeg()
Returns the maximum day degrees that one day can have.
Definition: PollenNectar.h:143
static CfgStr l_map_nectarpollen("MAP_NECTARPOLLEN_FILE", CFG_CUSTOM, "species_production_curves.csv")
CfgFloat cfg_GeneralColdWeatherThresholdFlower("GENERAL_COLD_WEATHER_THRESHOLD_FLOWER", CFG_CUSTOM, 2800)
The threshold of ending accumulated degrees for colder weather.
double m_total_sugar_whole_flowering
The total amount of sugar in the whole flowering period –mg.
Definition: PollenNectar.h:73
PollenNectarDevelopmentCurveSet * GetPollenNectarCurve(int a_ref)
Definition: PollenNectar.h:229
double getPollenQuality(int a_given_day)
Returns the pollen quality for the given day after day 0 in a year.
Definition: PollenNectar.h:111
std::map< int, double > m_flower_number_crop
The array to store the flower numbers for crop species.
Definition: PollenNectar.h:192
PollenNectarDevelopmentData * CreatePollenNectarDevelopmentData()
Definition: PollenNectar.cpp:294
CfgFloat cfg_GeneralColdWeatherAdjustFlower("GENERAL_COLD_WEATHER_ADJUST_FLOWER", CFG_CUSTOM, 3)
Adjust of the base development for colder weather in colder areas, for flower development in natural ...
class PollenNectarDevelopmentData * g_nectarpollen
Definition: Plants.cpp:41
int m_flowering_length
The flowering length in days.
Definition: PollenNectar.h:83
void RecalculatePollenNectarCurvesForYear(void)
Recalculate the pollen and nectar curves for a year for the habitat species. This function only be ca...
Definition: PollenNectar.cpp:396
double m_quantity
Definition: PollenNectar.h:167
bool value() const
Definition: Configurator.h:164
double m_total_pollen_whole_flowering
The total amount of pollen in the whole flowering period –mg.
Definition: PollenNectar.h:75
PollenNectarData()
Definition: PollenNectar.cpp:281
std::map< int, std::vector< PollenNectarData > > m_pollen_habitat_species
This is the dictionary to store the pollen for every habitat species in a year.
Definition: PollenNectar.h:182
CfgFloat cfg_GeneralMaxFloweringPeriod("GENERAL_MAX_FLOWERING_PERIOD", CFG_CUSTOM, 60.0)
The general max flowering period when there is no data in the input file.
PollenNectarDevelopmentData * CreatePollenNectarDevelopmentData()
Definition: PollenNectar.cpp:294
vector< double > m_sugar_per_phase
The array to store the sugar in different period, the size is the number of the flowering period phas...
Definition: PollenNectar.h:89
bool m_flag_not_enough_data
Flag to show not enough data is available, so this curve can't be used.
Definition: PollenNectar.h:51
double GetThreshold()
Returns the day degrees temperature threshold.
Definition: PollenNectar.h:141
Bool configurator entry class.
Definition: Configurator.h:155
Eigen::ArrayXd m_year_temp
This is used to store one year temperature data.
Definition: PollenNectar.h:178
vector< double > m_nectar_per_phase
The array to store the nectar in different period, the size is the number of the flowering period pha...
Definition: PollenNectar.h:87
static CfgStr l_map_tov_nectarpollen("MAP_TOV_NECTARPOLLEN_FILE", CFG_CUSTOM, "tovALMaSSNectarPollenInput.txt")
void SetStartingDayFlowering(int a_starting_day_flowering)
Set the starting day of flowering period.
Definition: PollenNectar.h:155
A class to manage a range of pollen and nectar development curves based on indexed rates.
Definition: PollenNectar.h:174
static CfgStr l_flower_number_crops("FLOWER_NUMBER_CROPS_FILE", CFG_CUSTOM, "crop_stacks.csv")
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
int get_array_size()
Definition: Configurator.h:222
void ClearAll()
Definition: PollenNectar.h:131
TTypesOfPopulation
An enum to hold all the possible types of population handled by a Population_Manager class.
Definition: PopulationManager.h:57
double getResource(int a_given_day, vector< double > &a_resource_per_phase)
Returns the resource for the given day after day o in a year.
Definition: PollenNectar.h:117
std::vector< double > value() const
Definition: Configurator.h:219
PollenNectarData supplyNectarHabitatSpeciesGivenDay(int a_curve_num, int a_given_day)
Supply the current nectar for the given species for the given day after today.
Definition: PollenNectar.cpp:354
PollenNectarDevelopmentCurveSet * tovGetPollenNectarCurve(TTypesOfVegetation a_tov_ref)
Definition: PollenNectar.h:204
double getSugar(int a_given_day)
Returns the sugar quantity for the given day after day 0 in a year.
Definition: PollenNectar.h:101
std::map< int, std::vector< PollenNectarData > > m_nectar_habitat_species
This is the dictionary to store the nectar for every habitat species in a year.
Definition: PollenNectar.h:184
Definition: LandscapeFarmingEnums.h:610
int GetRef()
Returns the reference number for the curve.
Definition: PollenNectar.h:145
TTypesOfVegetation TranslateVegTypes(int VegReference)
Definition: Elements.cpp:3724
CfgArray_Double cfg_FloweringPeriodPhasesProportionArray("FLOWERING_PERIOD_PHASES_PROPORTION_ARRAY", CFG_CUSTOM, 3, vector< double >{0.15, 0.7, 0.15})
Vector to store the proportions for flowering period phases, for now there are three phases; beginnin...
CfgBool cfg_pollen_nectar_on
Flag to determine whether nectar and pollen models are used - should be set to true for pollinator mo...
TTypesOfPopulation g_Species
Definition: PopulationManager.cpp:101
double m_total_nectar_whole_flowering
The total amount of nectar in the whole flowering period –mg.
Definition: PollenNectar.h:71
A data class to store nectar or pollen data.
Definition: PollenNectar.h:163
class Weather * g_weather
Definition: Weather.cpp:49
int m_ending_day_flowering
The ending day number of flowering period in a year.
Definition: PollenNectar.h:81
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
void updateHabitatFlowerResource(void)
The function to update the development of flower resource for all the habitat speccies.
Definition: PollenNectar.cpp:364
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
CfgFloat cfg_FlowerPollenNectarScaler
The scalear to reduce flower resource, default 1 which means no reduction.
Definition: PollenNectar.cpp:53
double m_StdFlowerLength
The standard value of flower length.
Definition: PollenNectar.h:65
Double configurator entry class.
Definition: Configurator.h:126
double GetEndingDDFlowering(void)
Returns the ending dd of flowering period.
Definition: PollenNectar.h:149
double m_quality
Definition: PollenNectar.h:168
int m_num_flowering_phases
The number of flowering phases.
Definition: PollenNectar.h:85
double getPollen(int a_given_day)
Returns the pollen quantity for the given day after day 0 in a year.
Definition: PollenNectar.h:106
Definition: Configurator.h:70
double GetNectarSugarConc()
Returns volume of sugar in volume of nectar (vol/vol).
Definition: PollenNectar.cpp:303
int DayInYear(void)
Definition: Calendar.h:58
bool tovGetPollenNectarCurveSowingFlag(TTypesOfVegetation a_tov_ref)
Definition: PollenNectar.h:221
CfgArray_Double cfg_FloweringPeriodPhasesLengthArray("FLOWERING_PERIOD_PHASES_LENGTH_ARRAY", CFG_CUSTOM, 3, vector< double >{0.275, 0.45, 0.275})
Vector to store the length proportion for flowering period phases.
double m_pollen_quality
The pollen quality when there is pollen, this also depends the species, by default it uses the apis v...
Definition: PollenNectar.h:77
double GetTempAfterDays(int days)
Get the temperature at the given number of days which is the number of days after today.
Definition: Weather.h:484
class LE_TypeClass * g_letype
Definition: Elements.cpp:806
int tovGetPollenNectarCurveRef(TTypesOfVegetation a_tov_ref)
Definition: PollenNectar.h:213
void ReadFlowerNumberCrop(void)
Function to read the flower number for crops.
Definition: PollenNectar.cpp:72
double m_DDthreshold
The threshold temperature to calculate day degrees.
Definition: PollenNectar.h:55
vector< int > m_day_flowering_phases
The array to store the day number for different flowering periods, the size is the number of the flow...
Definition: PollenNectar.h:93
double GetStartingDDFlowering(void)
Returns the staring dd of flowering period.
Definition: PollenNectar.h:147
~PollenNectarDevelopmentData()
Definition: PollenNectar.cpp:274
std::map< int, PollenNectarDevelopmentCurveSet > m_ResourceCurves
This is the main data set, it contains the resource curves for each species.
Definition: PollenNectar.h:180
void UpdateFlowerResource(void)
Update the flower resource for a year.
Definition: PollenNectar.cpp:454
PollenNectarData supplyPollenHabitatSpeciesCurrentDay(int a_curve_num)
Supply the current pollen for the given species.
Definition: PollenNectar.cpp:331