File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
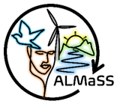 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
26 #ifndef POECILUS_CUPREUS_ALL_H
27 #define POECILUS_CUPREUS_ALL_H
29 #include <unordered_map>
static CfgFloat cfg_PoecilusPreOviMinTemp("POECILUS_PREOVIMINTEMP", CFG_CUSTOM, 12.0)
static CfgFloat cfg_PoecilusOvipositionLength_B("POECILUS_OVIPOSITIONLENGTH_B", CFG_CUSTOM, -9.7004)
void DoBeetleActiveProbe(int a_lifestage)
Counts all active beetles and saves the results to a file.
Definition: Beetle_BaseClasses.cpp:1918
Definition: Treatment.h:129
Definition: Treatment.h:64
Definition: Treatment.h:84
bool IsStartHabitat(int a_x, int a_y) const
Used to specify legal starting habitats for simulation start-up.
Definition: Beetle_BaseClasses.cpp:1557
Definition: Treatment.h:71
Definition: Treatment.h:88
static void SetMeanDistance(double a_temp)
Determines the base distance moved today.
Definition: PoecilusCupreus_All.cpp:478
Definition: Treatment.h:68
double m_Dormancy_exit_multiplier
The multiplier to apply if above the threshold dormancy_exit_threshold.
Definition: PoecilusCupreus_All.h:232
static CfgFloat cfg_PoecilusPreOviMaxTemp("POECILUS_PREOVIMAXTEMP", CFG_CUSTOM, 27.0)
Definition: Treatment.h:52
static CfgInt cfg_PoecilusADDepMort0("POECILUS_ADDEPMORTZERO", CFG_CUSTOM, 2)
Storage for density-dependent mortality parameter.
Definition: Treatment.h:89
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
void DayDegreeCalculations(int a_dayinyear, bool a_usehourly) override
Does day degree development calculations here.
Definition: PoecilusCupreus_All.cpp:491
Class for beetle larval stage 2, most functionality is in Beetle_Larvae.
Definition: PoecilusCupreus_All.h:99
Definition: Treatment.h:94
double GetDormancyChance() const
Get the daily chance to enter dormancy.
Definition: PoecilusCupreus_All.h:300
Definition: Treatment.h:142
virtual bool OnFarmEventPupae(FarmToDo event, Beetle_Base *a_caller)
Used to determine mortality based on an event for any beetle class - not a pure vitual but must be ov...
Definition: Beetle_BaseClasses.h:1038
int m_InFieldNo
In-field counter.
Definition: Beetle_BaseClasses.h:1117
static CfgFloat cfg_PoecilusDailyVarianceEggFactorA("POECILUS_DAILY_EGGFACTORVARIANCE_A", CFG_CUSTOM, -0.006)
TTypesOfVegetation TranslateVegTypes(int VegReference)
Returns vegetation type translated from the ALMaSS reference number.
Definition: Landscape.h:2326
double SupplyTemp(void)
Passes a request on to the associated Weather class function, the temperature for the current day.
Definition: Landscape.h:1993
PoecilusCupreus_Population_Manager * m_OurPopulation
Pointer to the population manager.
Definition: PoecilusCupreus_All.h:151
Definition: Treatment.h:83
Definition: Treatment.h:99
void AddStageProductionRecord(int a_value, int a_stage)
Adds to the stage production record attribute.
Definition: Beetle_BaseClasses.h:1146
static double m_PoecilusCoverThreshold
The minimum LAI considered cover.
Definition: PoecilusCupreus_All.h:168
Definition: Treatment.h:76
static CfgFloat cfg_PoecilusTempShadowThreshold("POECILUS_TEMP_SHADOW_THRESHOLD", CFG_CUSTOM, 0.0)
The Temperature above which Poecilus will prefer open habitats.
Definition: Beetle_BaseClasses.h:86
Definition: Treatment.h:35
Definition: Treatment.h:81
static CfgFloat cfg_PoecilusCoverThreshold("POECILUS_COVER_THRESHOLD", CFG_CUSTOM, 0.3)
The minimum LAI for cover.
Definition: PoecilusCupreus_All.h:126
vector< double > m_DevelConst1
Day degree threshold constant for eggs to pupae.
Definition: Beetle_BaseClasses.h:1068
Definition: Treatment.h:43
Definition: Treatment.h:91
double m_Dormancy_multiplier
The multiplier to apply if below the threshold dormancy_threshold.
Definition: PoecilusCupreus_All.h:228
static CfgFloat cfg_dormancy_threshold("POECILUS_DORMANCY_THRESHOLD", CFG_CUSTOM, 5.0)
Threshold temeperature. eac h day below that temperature increases hibernation chances.
Definition: Treatment.h:127
Definition: Treatment.h:103
TTypesOfPopulation m_population_type
Definition: PopulationManager.h:858
Definition: Treatment.h:121
PointDirection m_plPoint
A helper attribute when simulating movement.
Definition: Beetle_BaseClasses.h:911
static CfgInt cfg_PoecilusDormancyStartDateEarly("POECILUS_DORMANCY_STARTEARLY_DAY", CFG_CUSTOM, 153)
The Earliest date dormancy can occur.
static CfgFloat cfg_PoecilusMoveDistanceParamB("POECILUS_MOVE_DIST_PARAM_B", CFG_CUSTOM, 12.0)
Parameter B for caluculation mean daily movement distance.
int m_PoecilusDormancyStartDateEarly
Earliest day in the year for entering dormancy.
Definition: PoecilusCupreus_All.h:246
Definition: Treatment.h:44
double GetEggFormationProgress(int a_day) const
Returns the current progress towards egg formation based on a given date to become reproductively act...
Definition: PoecilusCupreus_All.h:282
Definition: Treatment.h:56
Definition: Treatment.h:125
PoecilusCupreus_Pupae(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
constructor for pupae
Definition: PoecilusCupreus_All.cpp:244
bool WinterMort() const override
The method for calculating overwintering mortality.
Definition: PoecilusCupreus_All.h:193
void CalculateDailyEggProduction(double a_temp) override
Figure out the maximum number of eggs that can be laid today - must be overridden in descendent class...
Definition: PoecilusCupreus_All.cpp:739
static CfgFloat cfg_PoecilusADDepMort1("POECILUS_ADDEPMORTONE", CFG_CUSTOM, 0.10)
Storage for density-dependent mortality parameter.
static void SetAdultDenDepMort0(const int a_value)
Storage for density-dependent mortality parameter.
Definition: Beetle_BaseClasses.h:842
virtual void DayDegreeCalculations(int a_dayinyear, bool a_usehourly)
Does the day degree calculations needed for the species here.
Definition: Beetle_BaseClasses.cpp:1601
Definition: Treatment.h:61
Definition: Treatment.h:80
Definition: Treatment.h:60
Definition: Treatment.h:90
double SupplyMaxTemp(void)
Passes a request on to the associated Weather class function, the maximum temperature for the current...
Definition: Landscape.h:2003
Definition: Treatment.h:123
const char * g_simulationName
Definition: Treatment.h:131
virtual double GetInsecticideApplication() const
the method overrides the method that returns the mortality due to insecticide application
Definition: Beetle_BaseClasses.h:367
static CfgFloat cfg_PoecilusPreOviFactorA("POECILUS_PREOVI_FACTOR_A", CFG_CUSTOM, 0.229)
Used to map locations of individuals for density estimates - space inefficient but good for testing.
Definition: PositionMap.h:45
Definition: PopulationManager.h:78
std::array< double, 365 > m_OvipostitionlengthProgress
Storage for daily oviposition period progress.
Definition: PoecilusCupreus_All.h:223
static CfgInt cfg_PoecilusDormancyStartDate("POECILUS_DORMANCY_START_DAY", CFG_CUSTOM, 244)
The typical date dormancy can occur.
Definition: Treatment.h:138
double m_PreOviRate
Rate of change with temperature for preovipostion development (calculated from other configs)
Definition: PoecilusCupreus_All.h:258
Definition: Treatment.h:122
Definition: Treatment.h:39
Landscape * m_l
Definition: Beetle_BaseClasses.h:129
static void SetEggFormationThreshold(double a_value)
Definition: PoecilusCupreus_All.h:196
Definition: Treatment.h:110
Definition: Treatment.h:51
double value() const
Definition: Configurator.h:142
double m_PoecilusDailyEggFactorB
Parameter b used to calculate the number of eggs laid per day.
Definition: PoecilusCupreus_All.h:236
Definition: Treatment.h:143
FarmToDo
Definition: Treatment.h:31
Definition: Treatment.h:133
double m_EggFormationProgress
A parameter that holds the value that determines when the first eggs are formed.
Definition: PoecilusCupreus_All.h:153
static int m_DayInYear
A holder for the day in year shared with all TAnimal objects.
Definition: PopulationManager.h:358
double GetDailyMortalityRate() const override
the override of the function that returns the background mortality rate
Definition: Beetle_BaseClasses.h:933
Definition: Treatment.h:104
Class for beetle larval stage 1, most functionality is in Beetle_Larvae.
Definition: PoecilusCupreus_All.h:85
Definition: Treatment.h:85
void CreateObjects(int ob_type, TAnimal *a_pvo_ptr, void *null, Struct_Beetle *a_data, int a_number) override
Method to add beetles to the population.
Definition: PoecilusCupreus_All.cpp:438
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: PoecilusCupreus_All.cpp:212
static double m_EggFormationThreshold
Definition: PoecilusCupreus_All.h:162
Definition: ALMaSS_Random.h:70
~PoecilusCupreus_Population_Manager() override=default
Destructor: the same as in base class.
Definition: Treatment.h:140
TTypesOfBeetleState
The enumeration lists all beetle behavioural states used by all the beetle species.
Definition: Beetle_BaseClasses.h:58
Definition: Treatment.h:38
Definition: Treatment.h:117
unsigned m_Lifestage
This is a useful parameter holding the beetle type (this includes larval stages)
Definition: Beetle_BaseClasses.h:166
void TryToReproduce() override
Does reproduction if possible.
Definition: PoecilusCupreus_All.h:182
Definition: Treatment.h:120
int m_y
Definition: Beetle_BaseClasses.h:128
static CfgFloat cfg_dormancy_exit_threshold("POECILUS_DORMANCY_EXIT_THRESHOLD", CFG_CUSTOM, 8.0)
Threshold for dormancy exit temperature. each day above that temperature increases hibernation exit c...
Definition: Treatment.h:59
The class describing the PoecilusCupreus Egg_List objects. In general case this class should have onl...
Definition: PoecilusCupreus_All.h:68
TTypesOfBeetleState CheckDormancy() override
Determines whether its time to hibernate.
Definition: PoecilusCupreus_All.cpp:307
Definition: Treatment.h:100
static double m_PoecilusTempShadowThreshold
The Temperature above which Poecilus will prefer open habitats.
Definition: PoecilusCupreus_All.h:164
Definition: Treatment.h:150
Definition: Treatment.h:124
int m_PoecilusDormancyExitDate
Latest day in the year for leaving dormancy.
Definition: PoecilusCupreus_All.h:252
Definition: Treatment.h:139
Definition: Treatment.h:126
static void SetTodaysEggProduction(const int a_value)
Daily temperature determined egg production.
Definition: Beetle_BaseClasses.h:848
static CfgFloat cfg_dormancy_multiplier("POECILUS_DORMANCY_MULTIPLIER", CFG_CUSTOM, 1.2)
The multiplier to apply if below the threshold dormancy_threshold.
virtual void TurningForced()
Turning returns a direction to move in next step.
Definition: Beetle_BaseClasses.h:973
Definition: Treatment.h:132
static void SetDevelopmentConstants(const std::vector< double > &a_value)
Set the day degree constant.
Definition: Beetle_BaseClasses.h:310
Definition: Treatment.h:148
static void SetLDDepMort1(const double a_value)
Storage for density-dependent mortality parameter.
Definition: Beetle_BaseClasses.h:578
Definition: Treatment.h:67
void CalcDailyRandomEggFactor(double a_temp)
Updates DailyRandomEggFactor. Should be called daily
Definition: PoecilusCupreus_All.cpp:755
double m_DormancyExitChance
Used to store the daily chance to exit dormancy.
Definition: PoecilusCupreus_All.h:219
static CfgFloat cfg_PoecilusDailyEggFactorA("POECILUS_DAILY_EGGFACTOR_A", CFG_CUSTOM, -0.02753)
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
double m_DormancyChance
Used to store the daily chance to enter dormancy.
Definition: PoecilusCupreus_All.h:217
static CfgFloat cfg_PoecilusStandardDormancyExitChance("POECILUS_STANDARD_DORMANCY_EXITCHANCE", CFG_CUSTOM, 0.03)
base probability to exit dormancy
Definition: Treatment.h:42
double m_Dormancy_threshold
Threshold temeperature. eac h day below that temperature increases hibernation chances.
Definition: PoecilusCupreus_All.h:226
PoecilusCupreus_Larvae3(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
constructor for Larvae3
Definition: PoecilusCupreus_All.cpp:223
Definition: Treatment.h:65
Definition: Treatment.h:45
Definition: Treatment.h:98
int m_x
Definition: Beetle_BaseClasses.h:98
static CfgFloat cfg_PoecilusDailyEggFactorC("POECILUS_DAILY_EGGFACTOR_C", CFG_CUSTOM, -16.70355)
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: Treatment.h:77
double m_PoecilusDailyEggFactorA
Parameter a used to calculate the number of eggs laid per day.
Definition: PoecilusCupreus_All.h:234
Definition: Treatment.h:96
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Beetle_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: Beetle_BaseClasses.cpp:655
double m_PoecilusStandardDormancyExitChance
Base chance per day of leaving dormancy.
Definition: PoecilusCupreus_All.h:256
void DoLast() override
Adds output adult locations to DoLast.
Definition: PoecilusCupreus_All.h:303
Definition: Treatment.h:54
static double CalcOvipositionLength(double a_temp)
Uses day degrees to determine when the oviposition period is over.
Definition: PoecilusCupreus_All.cpp:744
double m_PoecilusDailyVarianceEggFactorB
Parameter b used to calculate variance in the number of eggs laid per day.
Definition: PoecilusCupreus_All.h:242
Beetle_Population_Manager * m_OurPopulation
Pointer to the population manager.
Definition: Beetle_BaseClasses.h:160
Definition: Treatment.h:97
Definition: Treatment.h:141
static void SetLarvalDailyMort(const vector< double > &a_value, const int a_numLarvalStages, const int a_catagories)
Set the daily fixed mortality probability based on the larval stages and temperature.
Definition: Beetle_BaseClasses.h:582
The class describing the beetle Egg_List objects.
Definition: Beetle_BaseClasses.h:382
double m_PoecilusStandardDormancyChance
Base chance per day of entering dormancy.
Definition: PoecilusCupreus_All.h:254
static CfgFloat cfg_PoecilusDailyEggMort("POECILUS_DAILYEGGMORT", CFG_CUSTOM, 0.021)
The daily fixed mortality egg probability.
static CfgFloat cfg_EggFormationThreshold("POECILUS_EGGFORMATIONTHRESHOLD", CFG_CUSTOM, 1.0)
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
int m_LarvalStage
Current larval growth stage (1-3), used as a small speed hack.
Definition: Beetle_BaseClasses.h:619
int MoveTo_Quality_Assess() override
Determines whether a move is OK, and the turning rate.
Definition: PoecilusCupreus_All.cpp:336
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
bool CheckManagementBeetle() override
Definition: PoecilusCupreus_All.h:133
CfgInt cfg_PmEventfrequency
Definition: Treatment.h:70
bool OnFarmEventPupae(FarmToDo event, Beetle_Base *a_caller) override
Method dealing with the farming mortalities this differs for pupae.
Definition: PoecilusCupreus_All.cpp:734
Definition: Treatment.h:46
The population manager class for beetles.
Definition: Beetle_BaseClasses.h:1006
static CfgArray_Double cfg_PoecilusDevelopmentThresholds("POECILUS_DEVELOPMENTTHRESHOLDS", CFG_CUSTOM, 10, vector< double >{8.0, 7.0, 7.0, 7.0, 12.5, 99999, 99999, 99999, 99999, 99999})
Standard deviation of the mean daily movement distance.
void CheckReproduction() override
Starts reproduction when ready.
Definition: PoecilusCupreus_All.cpp:325
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
double GetOvipositionPeriodProgress(int a_day) const
Returns the current progress towards ending the oviposition period based on the first active day.
Definition: PoecilusCupreus_All.h:284
Bool configurator entry class.
Definition: Configurator.h:155
void Catastrophe2()
Method to arbitrarily alter populations size restricted spatially.
Definition: Beetle_BaseClasses.cpp:2030
double m_CurrentEggFormationProgress
Definition: PoecilusCupreus_All.h:213
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
Definition: Treatment.h:111
TTreatmentvsMortalityList m_Pc_Treatment_Mortalities
The list that summarises farming events that involve fertilizer applications and the mortalities they...
Definition: PoecilusCupreus_All.h:294
double m_DailyEggVariance
Used to store the daily variance in egg production.
Definition: PoecilusCupreus_All.h:215
double CalcDailyEggFormationProgress(double a_temp)
Calculates the additional development for egg formation for today.
Definition: PoecilusCupreus_All.cpp:748
static CfgFloat cfg_PoecilusPreOviMin("POECILUS_PREOVIMIN", CFG_CUSTOM, 1.0/41.2)
Definition: Treatment.h:135
Definition: Treatment.h:82
CfgInt cfg_Beetlestartnos
The number of beetles to start in the simulation.
CfgFloat cfg_PoecilusAdultMovementTempThreshold("POECILUS_ADULTMOVEMENTTEMPTHRESHOLD", CFG_CUSTOM, 6.9)
The temperature threshold for movement.
Definition: Treatment.h:63
Movement maps are used for rapid computing of animal movement. This version uses values of 0-3 only.
Definition: MovementMap.h:88
bool m_postOvi_bool
flag to shut down reproduction
Definition: PoecilusCupreus_All.h:157
static CfgInt cfg_PoecilusLarvalMortCategories("BEMBIDION_LARVALMORTCATEGORIES", CFG_CUSTOM, 6)
Definition: Treatment.h:62
Definition: Treatment.h:119
bool FertilizerMortality(FarmToDo a_event)
The method that deals with the fertilizer mortality for the larvae.
Definition: PoecilusCupreus_All.cpp:816
Definition: Treatment.h:72
static void SetAdultDenDepMort1(const double a_value)
Set density-dependent mortality parameter.
Definition: Beetle_BaseClasses.h:844
Definition: Treatment.h:112
Definition: Treatment.h:137
Definition: Treatment.h:40
Definition: Treatment.h:53
virtual void ReInit(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
ReInit for object pool.
Definition: PoecilusCupreus_All.cpp:192
static CfgFloat cfg_PoecilusOvipositionLength_C("POECILUS_OVIPOSITIONLENGTH_C", CFG_CUSTOM, 0.2377)
virtual double GetHarvestMortality() const
the method overrides the method that returns the mortality due to harvest
Definition: Beetle_BaseClasses.h:369
static CfgFloat cfg_PoecilusPreOviMax("POECILUS_PREOVIMAX", CFG_CUSTOM, 1.0/5.0)
static void SetDailyLarvaMort(const double a_value)
Set the daily fixed mortality probability.
Definition: Beetle_BaseClasses.h:580
static CfgFloat cfg_PoecilusDailyVarianceEggFactorB("POECILUS_DAILY_EGGFACTORVARIANCE_B", CFG_CUSTOM, 0.27)
Definition: Treatment.h:145
Definition: Configurator.h:208
static CfgFloat cfg_PoecilusDailyLarvalMort("POECILUS_DAILYLARVALMORT", CFG_CUSTOM, 0.005)
The daily fixed mortality larval probability.
TTypesOfVegetation m_InCropRef
In crop tole reference.
Definition: Beetle_BaseClasses.h:1123
Definition: Treatment.h:86
int m_BeetleLarvalStagesNum
Definition: Beetle_BaseClasses.h:1058
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: PoecilusCupreus_All.cpp:231
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
bool m_CanReproduce_bool
Signal reproductive readiness.
Definition: Beetle_BaseClasses.h:969
double g_speedy_Divides[2001]
A generally useful array of fast divide calculators by multiplication.
Definition: Landscape.cpp:344
Definition: Treatment.h:95
Definition: Treatment.h:147
int m_AgeDays
To hold the age in days.
Definition: PopulationManager.h:378
CfgFloat cfg_BeetleAdultMovementTempThreshold
The temperature threshold for movement.
Definition: Treatment.h:36
static void SetDailyEggMort(const double a_value)
Set the daily fixed mortality probability.
Definition: Beetle_BaseClasses.h:446
static vector< double > m_DailyAdultMortalityWithSeason
Definition: PoecilusCupreus_All.h:160
void CalcDormancyExitChance(double a_temp, int a_day)
Updates probability of leaving dormancy. Should be called daily
Definition: PoecilusCupreus_All.cpp:790
CfgInt cfg_BeetleInCropRef
A reference to a crop type if we are looking for mortality locations within a special crop.
Definition: Treatment.h:116
CfgInt cfg_BeetleLarvalStagesNum
The number of larval stages for this species.
Definition: Treatment.h:93
static CfgInt cfg_PoecilusLDDepMort0("POECILUS_LDDEPMORTZERO", CFG_CUSTOM, 3)
Storage for density-dependent mortality parameter.
virtual void TryToReproduce()
Does reproduction if possible.
Definition: Beetle_BaseClasses.cpp:1250
Definition: Treatment.h:114
std::array< double, 365 > m_EggFormationProgress
Storage for daily eggformation progress.
Definition: PoecilusCupreus_All.h:221
static CfgArray_Double cfg_PoecilusLarvalDailyTemperatureMort("POECILUS_LARVALDAILYTEMPERATUREMORT", CFG_CUSTOM, 3 *6, vector< double >{ 0.0855, 0.0855, 0.1036, 0.0563, 0.0515, 0.0657, 0.0626, 0.0626, 0.0940, 0.0529, 0.0492, 0.0633, 0.0631, 0.0631, 0.0545, 0.0268, 0.0236, 0.0295 })
The daily fixed temperature related mmortality larval probability.
static CfgFloat cfg_PoecilusOvipositionLength_A("POECILUS_OVIPOSITIONLENGTH_A", CFG_CUSTOM, 129.9)
double SupplyVegCover(int a_polyref)
Returns the vegetation cover proportion of the vegetation using the polygon reference number a_polyre...
Definition: Landscape.h:1667
int value() const
Definition: Configurator.h:116
static CfgFloat cfg_PoecilusDailyEggFactorB("POECILUS_DAILY_EGGFACTOR_B", CFG_CUSTOM, 1.72330)
Definition: Treatment.h:75
std::vector< double > value() const
Definition: Configurator.h:219
Definition: Beetle_BaseClasses.h:85
Definition: Treatment.h:47
void Catastrophe() override
Method to arbitrarily alter populations size.
Definition: Beetle_BaseClasses.cpp:1967
Definition: Treatment.h:106
Definition: Treatment.h:109
static void SetAdultMovementTempThreshold(const double a_value)
Set the temperature threshold for movement.
Definition: Beetle_BaseClasses.h:830
TTypesOfBeetleState m_CurrentBState
Current behavioural state.
Definition: Beetle_BaseClasses.h:158
static void SetMoveDistribution(const string &a_type_str, const string &a_args_str)
Set the movement distribution.
Definition: Beetle_BaseClasses.cpp:320
The class describing the beetle larvae objects.
Definition: Beetle_BaseClasses.h:535
bool OnFarmEvent(FarmToDo a_event, Beetle_Base *a_caller) override
Method dealing with the farming mortalities.
Definition: PoecilusCupreus_All.cpp:514
A data class for Beetle data.
Definition: Beetle_BaseClasses.h:125
double m_PoecilusDailyVarianceEggFactorA
Parameter a used to calculate variance in the number of eggs laid per day.
Definition: PoecilusCupreus_All.h:240
The class describing the adult (female) beetle objects.
Definition: Beetle_BaseClasses.h:793
PoecilusCupreus_Larvae2(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
constructor for Larvae2
Definition: PoecilusCupreus_All.cpp:203
std::unordered_map< FarmToDo, double > TTreatmentvsMortalityList
Definition: PoecilusCupreus_All.h:42
Definition: Treatment.h:146
static CfgFloat cfg_PoecilusStandardDormancyChance("POECILUS_STANDARD_DORMANCY_CHANCE", CFG_CUSTOM, 0.03)
base probability of dormancy
Definition: Treatment.h:134
bool CheckDormancyExit() override
Determines whether its time to disperse.
Definition: PoecilusCupreus_All.cpp:316
static CfgFloat cfg_PoecilusMoveDistanceParamA("POECILUS_MOVE_DIST_PARAM_A", CFG_CUSTOM, 1.81)
Parameter A for caluculation mean daily movement distance.
static CfgInt cfg_PoecilusDormancyExitDateEarly("POECILUS_DORMANCY_EXITEARLY_DAY", CFG_CUSTOM, 60)
The Earliest date dormancy exit can occur.
Class for beetle larval stage 3, most functionality is in Beetle_Larvae.
Definition: PoecilusCupreus_All.h:113
Definition: Treatment.h:118
Definition: Beetle_BaseClasses.h:88
Definition: Treatment.h:113
PoecilusCupreus_Larvae1(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
constructor for Larvae1
Definition: PoecilusCupreus_All.cpp:177
static void SetPoecilusLaiCoverThreshold(double a_value)
Set the minimum LAI considered cover.
Definition: PoecilusCupreus_All.h:200
static CfgFloat cfg_dormancy_exit_multiplier("POECILUS_DORMANCY_EXIT_MULTIPLIER", CFG_CUSTOM, 1.2)
The multiplier to apply if above the threshold dormancy_exit_threshold.
Definition: Treatment.h:41
int m_InCropNo
In-crop counter.
Definition: Beetle_BaseClasses.h:1119
Integer configurator entry class.
Definition: Configurator.h:102
Definition: PoecilusCupreus_All.h:209
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
ReInit for object pool.
Definition: PoecilusCupreus_All.cpp:270
static double m_TemperatureToday
A holder for the temperature today shared with all TAnimal objects.
Definition: PopulationManager.h:354
Definition: Treatment.h:37
static CfgFloat cfg_PoecilusPreOviFactorC("POECILUS_PREOVI_FACTOR_C", CFG_CUSTOM, 140.28)
PoecilusCupreus_Population_Manager(Landscape *a_l_ptr)
Constructor.
Definition: PoecilusCupreus_All.cpp:359
Definition: Treatment.h:79
double SupplyMinTemp(void)
Passes a request on to the associated Weather class function, the minimum temperature for the current...
Definition: Landscape.h:1998
static CfgFloat cfg_PoecilusPreOviFactorB("POECILUS_PREOVI_FACTOR_B", CFG_CUSTOM, -11.14)
The class describing the beetle pupae objects.
Definition: Beetle_BaseClasses.h:688
TTypesOfBeetleState Ageing() override
The daily ageing for a beetle.
Definition: PoecilusCupreus_All.cpp:287
Definition: Treatment.h:74
Definition: Beetle_BaseClasses.h:83
Double configurator entry class.
Definition: Configurator.h:126
CfgFloat cfg_biocide_reduction_val
Definition: PoecilusCupreus_All.h:147
string m_SimulationName
stores the simulation name
Definition: PopulationManager.h:622
static CfgFloat cfg_PoecilusPostOviMort("POECILUS_POSTOVIMORT", CFG_CUSTOM, 0.1)
CfgBool cfg_CfgRipleysOutputUsed
Definition: Treatment.h:136
Definition: Treatment.h:108
Definition: Treatment.h:69
Definition: Treatment.h:105
PoecilusCupreus_Adult(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
constructor for PoecilusCupreus adult
Definition: PoecilusCupreus_All.cpp:259
static CfgFloat cfg_PoecilusLDDepMort1("POECILUS_LDDEPMORTONE", CFG_CUSTOM, 0.10)
Storage for density-dependent mortality parameter.
Definition: Treatment.h:78
Definition: Configurator.h:70
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
Definition: Treatment.h:66
Definition: Treatment.h:92
Definition: Treatment.h:102
static CfgInt cfg_PoecilusDormancyExitDate("POECILUS_DORMANCY_EXIT_DAY", CFG_CUSTOM, 152)
The typical date dormancy exit is finished.
static CfgArray_Double cfg_PoecilusDevelopmentConstants("POECILUS_DEVELOPMENTCONSTANTS", CFG_CUSTOM, 10, vector< double >{88.7, 85.0, 85.0, 90.0, 110.0, 0, 0, 0, 0, 0})
The egg, larval and pupal development target in day degrees above a threshold (10 possible stages)
The base class for all beetles.
Definition: Beetle_BaseClasses.h:150
void CalcDormancyChance(double a_temp, int a_day)
Updates probability of enterning dormancy. Should be called daily
Definition: PoecilusCupreus_All.cpp:760
int m_OffFieldNo
Off-field counter.
Definition: Beetle_BaseClasses.h:1121
static double m_PoecilusTempMoveThreshold
The minimum temperature for movement.
Definition: PoecilusCupreus_All.h:166
Definition: Treatment.h:57
Definition: Treatment.h:87
static void SetDDepRange(const int a_value)
Set density-dependent range parameter.
Definition: Beetle_BaseClasses.h:328
void PushIndividual(const unsigned a_listindex, TAnimal *a_individual_ptr)
Definition: PopulationManager.cpp:1682
Definition: Treatment.h:101
Definition: Beetle_BaseClasses.h:73
static void SetLDDepMort0(const int a_value)
Storage for density-dependent mortality parameter.
Definition: Beetle_BaseClasses.h:576
int m_x
Definition: Beetle_BaseClasses.h:127
Definition: Treatment.h:73
int SupplyLastTreatment(int a_polyref, int *a_index)
Returns the last treatment recorded for the polygon.
Definition: Landscape.h:1941
std::unique_ptr< MovementMap > m_MoveMap
Map of suitability for movement.
Definition: Beetle_BaseClasses.h:1107
Definition: Treatment.h:32
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Beetle_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: Beetle_BaseClasses.cpp:898
vector< vector< forward_list< TAnimal * > * > > TheSubArrays
Hold all the animal pointers.
Definition: PopulationManager.h:804
Definition: Treatment.h:55
int m_PoecilusDormancyExitDateEarly
Earliest day in the year for leaving dormancy.
Definition: PoecilusCupreus_All.h:250
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Beetle_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: Beetle_BaseClasses.cpp:781
static void SetPoecilusTempShadowThreshold(double a_value)
Set the Temperature above which Poecilus will prefer open habitats.
Definition: PoecilusCupreus_All.h:198
Definition: Beetle_BaseClasses.h:82
int m_y
Definition: Beetle_BaseClasses.h:99
void SetPc_Treatment_Mortalities()
Hard-coded for now method to set fertilizer mortality chances.
Definition: PoecilusCupreus_All.cpp:825
int m_FirstActiveDay
Remembers the first day of activity for egg formation calculation.
Definition: PoecilusCupreus_All.h:155
virtual double GetStriglingMortality() const
Mortality by Strigling: similar for all forms– should use base class method.
Definition: Beetle_BaseClasses.h:363
Definition: Treatment.h:34
PoecilusCupreus_Egg_List(int a_today, PoecilusCupreus_Population_Manager *a_bpm_ptr, Landscape *a_l_ptr)
Egg_List class constructor.
Definition: PoecilusCupreus_All.cpp:162
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
int m_PoecilusDormancyStartDate
Typical day in the year for entering dormancy.
Definition: PoecilusCupreus_All.h:248
Definition: Beetle_BaseClasses.h:87
virtual double GetSoilCultivationMortality() const
the method overrides the method that returns the mortality due to soil cultivation
Definition: Beetle_BaseClasses.h:365
CfgBool cfg_ReallyBigOutputUsed
Definition: Treatment.h:58
double m_Dormancy_exit_threshold
Threshold for dormancy exit temperature. each day above that temperature increases hibernation exit c...
Definition: PoecilusCupreus_All.h:230
Definition: Treatment.h:128
double GetDormancyExitChance() const
Get the daily chance to exit dormancy.
Definition: PoecilusCupreus_All.h:302
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, PoecilusCupreus_Population_Manager *a_bpm_ptr)
ReInit for object pool.
Definition: PoecilusCupreus_All.cpp:249
static CfgFloat cfg_PoecilusDailyVarianceEggFactorC("POECILUS_DAILY_EGGFACTORVARIANCE_C", CFG_CUSTOM, -2.32)
Definition: Beetle_BaseClasses.h:84
double m_PoecilusDailyEggFactorC
Parameter c used to calculate the number of eggs laid per day.
Definition: PoecilusCupreus_All.h:238
Definition: Beetle_BaseClasses.h:70
double m_PoecilusDailyVarianceEggFactorC
Parameter c used to calculate variance in the number of eggs laid per day.
Definition: PoecilusCupreus_All.h:244
Definition: Treatment.h:115
Definition: Treatment.h:33
static CfgInt cfg_PoecilusDDepRange("POECILUS_DDEPRANGE", CFG_CUSTOM, 3)
The density-dependent range parameter.
Definition: Treatment.h:107
vector< std::array< double, 365 > > m_DayDegs
Storage for daily day degrees for non-adult stages.
Definition: Beetle_BaseClasses.h:1052
void IncLiveArraySize(int a_listindex)
Increments the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:665