File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
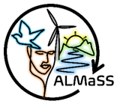 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
435 bool Run(
int a_dist,
int a_direction);
455 virtual void Running(
int a_max_dist);
460 void Walking(
int a_dist,
int a_direction);
497 double ForageSquareP(
int a_x,
int a_y,
double* a_pestexposure);
541 double GetRMR(
int a_age,
double a_size);
552 if ((a_age<0) || (a_age>5000)) {
565 if ((a_age<0) || (a_age>5000)) {
578 if ((a_age<0) || (a_age>5000)) {
591 if ((a_age<0) || (a_age>5000)) {
604 if ((a_age<0) || (a_age>5000)) {
617 if ((a_age<0) || (a_age>5000)) {
630 if ((a_size<0) || (a_size>7000)) {
631 m_TheLandscape->
Warn(
"Hare GetKJperM a_size out of bounds", std::to_string(a_size) );
643 m_DensityMap[a_type][x >> __DENSITYSHIFT][y >> __DENSITYSHIFT]++;
650 m_DensityMap[a_type][x >> __DENSITYSHIFT][y >> __DENSITYSHIFT]--;
690 __DENSITYSHIFT][y >> __DENSITYSHIFT];
697 return m_DensityMap[6][x >> __DENSITYSHIFT][y >> __DENSITYSHIFT];
709 if ((h<0) || (h>2499)) {
710 g_msg->
Warn(
"Hare Interference range out of bounds", std::to_string(h) );
731 fprintf(
BodyBurdenPrb,
"%d\t%d\t%g\t%g\n", a_year, a_day, a_bb, a_mgkg);
827 void Step(
void)
override;
882 void Step(
void)
override;
888 void Init(
double p_weight);
933 void Step(
void)
override;
939 void Init(
double p_weight);
984 void Step(
void)
override;
994 void Init(
double p_weight,
int a_age,
int a_Ref);
1084 void Step(
void)
override;
1093 void Init(
double p_weight,
int a_age,
int a_Ref);
Definition: LandscapeFarmingEnums.h:925
void Step(void) override
Step for Hare_Male.
Definition: Hare_All.cpp:2523
void ON_Dead() override
This hare has been killed.
Definition: Hare_All.cpp:1962
Definition: Hare_All.h:80
static CfgFloat cfg_littersize_SD11("HARE_LITTERSIZE_SD_K", CFG_CUSTOM, 0.6415)
~THare_Population_Manager(void) override
THare_Population_Manager destructor.
Definition: Hare_All.cpp:383
virtual void GeneralOrganoPhosphate(double)
Handles internal effects of organophosphate pesticide exposure. If any effects are needed this method...
Definition: Hare_All.h:486
CfgInt cfg_hare_max_age_var("HARE_MAX_AGE_VAR", CFG_CUSTOM, 180)
void ReInit(struct_Hare a_data)
Male object reinitiation.
Definition: Hare_All.cpp:2404
void InternalPesticideHandlingAndResponse() override
Handles internal effects of pesticide exposure. If any effects are needed this method must be re-impl...
Definition: Hare_All.cpp:3690
void SetPolyFood(int a_poly, double a_value)
Set polygon food quality.
Definition: Hare_All.h:725
int x
Definition: Hare_All.h:138
int Supply_m_Location_x() const
Returns the ALMaSS x-coordinate.
Definition: PopulationManager.h:239
Definition: Treatment.h:129
Definition: Treatment.h:64
Definition: Treatment.h:84
void CreateObjects(int ob_type, TAnimal *pvo, void *null, struct_Hare *data, int number)
Method used to create new hares.
Definition: Hare_All.cpp:873
TTypeOfHareState st_Foraging()
Female Foraging.
Definition: Hare_All.cpp:3104
Definition: Treatment.h:71
void BeginStep(void) override
BeginStep for Hare_Male.
Definition: Hare_All.cpp:2441
int GetInfantDensity(int x, int y)
Density function - returns the density of infants in the square containing by x,y....
Definition: Hare_All.h:656
static CfgFloat cfg_littersize_mean12("HARE_LITTERSIZE_MEAN_L", CFG_CUSTOM, 0.0)
static double * m_vegPalatability
Will hold and array of palatability for hare for each tov type. Most are 1, but unpalatable vegetatio...
Definition: Hare_All.h:318
int GetFemaleDensity(int x, int y)
Density function - returns the density of females in the square containing by x,y....
Definition: Hare_All.h:678
Definition: Treatment.h:88
double m_MaxDailyGrowthEnergyF[5001]
Precalculated max growth energy requirement with size for fat
Definition: Hare_All.h:789
CfgInt cfg_PmEventfrequency
bool UpdateYoung(THare *a_old, THare *a_new)
Swap a young list pointer.
Definition: Hare_All.cpp:3515
Definition: Treatment.h:68
Definition: MapErrorMsg.h:37
The class that handles all the population lists for hares.
Definition: Hare_All.h:529
static CfgFloat cfg_littersize_mean2("HARE_LITTERSIZE_MEAN_B", CFG_CUSTOM, 2.508)
Definition: Treatment.h:144
Definition: Treatment.h:52
Definition: Treatment.h:89
void GiveBirth()
Produce a litter.
Definition: Hare_All.cpp:3355
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
Definition: Treatment.h:94
void ON_Dead() override
The female is dead.
Definition: Hare_All.cpp:3647
Definition: Hare_All.h:93
CfgInt cfg_juv_starve("HARE_JUV_STARVE", CFG_CUSTOM, 2500)
CfgBool cfg_BeetleBankInvert
void Mating()
Mate.
Definition: Hare_All.cpp:3311
Definition: Treatment.h:142
CfgInt cfg_young_ddepmort("HARE_YOUNGDDEPMORT", CFG_CUSTOM, 16)
int m_DensityMap[8][200][200]
Array storing densities measured in different ways
Definition: Hare_All.h:771
double g_FarmIntensivenessH
Definition: Hare_All.cpp:301
virtual TAnimal * SupplyAnimalPtr(unsigned int a_index, unsigned int a_animal)
Returns the pointer indexed by a_index and a_animal. Note NO RANGE CHECK.
Definition: PopulationManager.h:678
double SupplyTemp(void)
Passes a request on to the associated Weather class function, the temperature for the current day.
Definition: Landscape.h:1993
double * m_PolyFood
Temporary storage
Definition: Hare_All.h:777
virtual void DoLast()
Definition: PopulationManager.cpp:656
Definition: Treatment.h:83
Definition: Treatment.h:99
int GetLitterSize(int noLitters)
Returns the litter size.
Definition: Hare_All.cpp:698
int y
Definition: Hare_All.h:139
TTypeOfHareState st_Foraging()
Young foraging.
Definition: Hare_All.cpp:1708
Definition: Treatment.h:76
void GeneralEndocrineDisruptor(double) override
Handles internal effects of endocrine distrupter pesticide exposure. If any effects are needed this m...
Definition: Hare_All.cpp:2809
Definition: Treatment.h:35
Definition: Treatment.h:81
CfgInt cfg_HareFemaleSicknessDensityDepValue("HARE_FEMALESICKNESSDENDEPVALUE", CFG_CUSTOM, 40)
int GetPegDirection()
Get direction of peg.
Definition: HareForagenPeg.cpp:467
THare_Population_Manager(Landscape *L)
This is the constructor for the hare population manager.
Definition: Hare_All.cpp:399
double m_DMWeight[5001]
Array for storing minimum acceptable size with age.
Definition: Hare_All.h:753
CfgBool cfg_hare_pesticideresponse_on("HARE_PESTICIDERESPONSE_ON", CFG_CUSTOM, false)
If set to true then hares will collect and respond to pesticide information. This will slow the simul...
static CfgInt cfg_ReproStartDay("HARE_REPROSTARTDAY", CFG_CUSTOM, 18)
Definition: Treatment.h:43
static CfgInt cfg_young_starvation_threshold("HARE_YOUNG_STARVE_THRESHOLD", CFG_CUSTOM, 4)
Definition: Treatment.h:91
~THare() override
Destructor.
Definition: Hare_THare.cpp:109
void ON_Dead() override
Definition: Hare_All.cpp:2389
Definition: Treatment.h:127
static CfgFloat cfg_littersize_SD4("HARE_LITTERSIZE_SD_D", CFG_CUSTOM, 0.6415)
Definition: Treatment.h:103
void BeginStep(void) override
BeginStep for Hare_Juvenile.
Definition: Hare_All.cpp:2007
double m_pesticidedegradationrate
State variable used to hold the daily degredation rate of the pesticide in the body.
Definition: Hare_All.h:306
double m_SpeedWalking
m/min speed of walking per kg hare
Definition: Hare_All.h:248
TTypesOfPopulation m_population_type
Definition: PopulationManager.h:858
Definition: Treatment.h:121
bool SanityCheckYoungList()
Debug function.
Definition: Hare_All.cpp:3678
void Set_all_Att_UserDefinedBool(bool(*udf_fnc)(TTypesOfLandscapeElement))
used to classify the userdefinedbool attribute and set it - this requires supplying the correct class...
Definition: Landscape.h:931
CfgBool cfg_ReallyBigOutputUsed
static CfgFloat cfg_littersize_SD2("HARE_LITTERSIZE_SD_B", CFG_CUSTOM, 0.957)
vector< unsigned > BeforeStepActions
Holds the season list of possible before step actions.
Definition: PopulationManager.h:819
Definition: Treatment.h:44
Definition: Treatment.h:56
Definition: Treatment.h:125
void st_NextStage()
'mature' to become a young
Definition: Hare_All.cpp:1505
static CfgFloat cfg_littersize_SD8("HARE_LITTERSIZE_SD_H", CFG_CUSTOM, 0.6415)
Hare_Object
Definition: Hare_All.h:58
int GetRefNum()
Get the refnum for this hare.
Definition: Hare_All.h:417
static CfgFloat cfg_littersize_mean4("HARE_LITTERSIZE_MEAN_D", CFG_CUSTOM, 1.72)
double GetKJperM(int a_size)
Get the cost of moving 1m in KJ dependent upon mass (.
Definition: Hare_All.h:628
CfgInt cfg_hare_i_cut("HARE_CUTTING_MORT_INFANT", CFG_CUSTOM, 50)
double m_EnergyMax
State variable - the amount of energy it is possible to eat as a multiplyer or RMR.
Definition: Hare_All.h:223
double g_VegHeightForageReduction
Used to scale access to crops for modern day farm intensiveness.
Definition: Hare_All.cpp:299
Definition: Hare_All.h:101
void InternalPesticideHandlingAndResponse() override
Handles internal effects of pesticide exposure. If any effects are needed this method must be re-impl...
Definition: Hare_All.cpp:2769
double m_GrowthEfficiencyF[5001]
Precalculated growth efficiency for fat with size
Definition: Hare_All.h:793
int m_guard_cell_y
The index y to the guard cell.
Definition: PopulationManager.h:374
double g_hare_peg_inertia
Definition: Hare_All.cpp:48
double m_MaxDailyGrowthEnergyP[5001]
Precalculated max growth energy requirement with size for protein
Definition: Hare_All.h:787
int age
Definition: Hare_All.h:140
bool WasPredated() override
Test for mortality.
Definition: Hare_All.cpp:2380
Definition: Treatment.h:61
Definition: Treatment.h:80
Definition: Treatment.h:60
Definition: Hare_All.h:100
Definition: Treatment.h:90
int m_Lifespan
Physiolocal lifespan, assuming nothing else kills the hare (unlikely to reach this age)
Definition: Hare_All.h:198
const char * m_ListNames[32]
A list of life-stage names.
Definition: PopulationManager.h:628
Definition: Treatment.h:123
~Hare_Female() override
Female Destructor.
Definition: Hare_All.cpp:2864
void Init(double p_weight)
Object initiation.
Definition: Hare_All.cpp:1984
double m_AdultMortRate
Input variable - Adult mortality rate.
Definition: Hare_All.h:741
void EndStep(void) override
BeginStep for Hare_Juvenile.
Definition: Hare_All.cpp:2122
Definition: Treatment.h:131
Definition: Hare_All.h:61
CfgInt cfg_hare_y_tractor("HARE_TRACTOR_MORT_YOUNG", CFG_CUSTOM, 2)
virtual bool WasPredated()
Test for predation.
Definition: Hare_THare.cpp:341
static CfgFloat cfg_MaxEnergyIntakeScaler("HARE_MAXENERGYINTAKESCALER", CFG_CUSTOM, 1.825)
static CfgFloat cfg_littersize_SD10("HARE_LITTERSIZE_SD_J", CFG_CUSTOM, 0.6415)
static CfgInt cfg_MRR1("HARE_MMRONE", CFG_CUSTOM, 999)
Definition: Treatment.h:138
void ReInit(struct_Hare a_data)
Young object reinitiation.
Definition: Hare_All.cpp:1558
Definition: Treatment.h:122
Definition: Treatment.h:39
TTypeOfHareState st_Developing() override
Female Developing.
Definition: Hare_All.cpp:3002
CfgFloat cfg_HareInterferenceConstant("HARE_INTERFERENCECONSTANT", CFG_CUSTOM, -0.03)
static CfgInt cfg_DaysToOestrous("HARE_DAYSTOOESTROUS", CFG_CUSTOM, 20)
int m_variableDD
Used to vary the density dependence each year
Definition: Hare_All.h:773
static CfgInt cfg_hare_max_dispersal("HARE_MAX_DISPERSAL", CFG_CUSTOM, 1000)
Definition: Treatment.h:110
static CfgInt cfg_hare_femalesterility("HARE_FEMALESTERILITY", CFG_CUSTOM, 1401)
CfgInt cfg_HareHuntingDate("HARE_HUNTING_DATE", CFG_CUSTOM, 312)
Definition: Treatment.h:51
double value() const
Definition: Configurator.h:142
CfgFloat cfg_HarePesticideAccumulationThreshold("HARE_PESTICIDEACCUMULATIONTHRESHOLD", CFG_CUSTOM, 0.0)
This is the value that triggers pesticide response, which may be a threshold, or if simply set to 0....
Definition: Hare_All.h:72
int GetHareRefNum()
Get the next ID number available.
Definition: Hare_All.h:546
virtual void InternalPesticideHandlingAndResponse()
Handles internal effects of pesticide exposure. If any effects are needed this method must be re-impl...
Definition: HareForagenPeg.cpp:559
Definition: Treatment.h:143
int m_ActivityTime
Minutes of potential activity time per day.
Definition: Hare_All.h:203
FarmToDo
Definition: Treatment.h:31
CfgInt cfg_FarmIntensivenessH("HARE_FARMINTENSIVENESSH", CFG_CUSTOM, 40)
int SupplyMonth(void)
Passes a request on to the associated Calendar class function, returns m_month + 1 (the calendar mont...
Definition: Landscape.h:2272
Definition: Treatment.h:133
static CfgFloat cfg_minLeveretBirthWeight("HARE_MINLEVERETBIRTHWEIGHT", CFG_CUSTOM, 95 *0.88 *0.33)
int m_LitterSize[12][7]
Storage for litter size distributions (not used in default config)
Definition: Hare_All.h:775
static CfgFloat cfg_littersize_SD5("HARE_LITTERSIZE_SD_E", CFG_CUSTOM, 0.6415)
void AddHareDensity(int x, int y, Hare_Object a_type)
Density function - adds one to the density in the square containing by x,y. Each square is 256x256m i...
Definition: Hare_All.h:642
void ON_YoungKilled(THare *a_young)
A leveret has been killed.
Definition: Hare_All.cpp:3538
CfgFloat cfg_HareHunting("HARE_HUNTING", CFG_CUSTOM, 0.1)
double GetWeight()
Definition: Hare_All.h:362
static CfgFloat cfg_littersize_SD6("HARE_LITTERSIZE_SD_F", CFG_CUSTOM, 0.6415)
static CfgInt cfg_adult_starvation_threshold("HARE_ADULT_STARVE_THRESHOLD", CFG_CUSTOM, 16)
Definition: Treatment.h:104
Hare_Female * Mum
Definition: Hare_All.h:143
void Step(void) override
Step for the Hare_Infant.
Definition: Hare_All.cpp:1261
Definition: Treatment.h:85
double ForageSquare(int a_x, int a_y)
Forage from an area.
Definition: HareForagenPeg.cpp:320
CfgFloat cfg_AgeRelatedInterferenceScaling("HARE_AGERELATEDINTERFERENCESCALING", CFG_CUSTOM, 1.0)
THare_Population_Manager * m_OurPopulationManager
Pointer to the hare population manager.
Definition: Hare_All.h:194
THare_Population_Manager * HM
Definition: Hare_All.h:142
void EndStep(void) override
Female EndStep.
Definition: Hare_All.cpp:2996
static CfgFloat cfg_maxLeveretBirthWeight("HARE_MAXLEVERETBIRTHWEIGHT", CFG_CUSTOM, 125 *0.88 *0.33)
bool OnFarmEvent(FarmToDo event) override
Do we require a response to a farm event.
Definition: Hare_THare.cpp:356
void ON_Dead() override
Definition: Hare_All.cpp:2763
Class for female hares.
Definition: Hare_All.h:1044
void BeginStep(void) override
BeginStep for the Hare_Infant.
Definition: Hare_All.cpp:1213
Definition: Hare_All.h:62
bool SupplyAttUserDefinedBool(int a_polyref)
Returns the user defined boolean attribute of a polygon using the polygon reference number a_polyref,...
Definition: Landscape.h:908
TTypeOfHareState st_Dispersal() override
Not used.
Definition: Hare_All.cpp:1741
Definition: Treatment.h:140
void Init()
Object initiation.
Definition: Hare_All.cpp:1193
Definition: Treatment.h:38
double m_GrowthEfficiencyP[5001]
Precalculated growth efficiency for protein with size
Definition: Hare_All.h:791
Definition: Treatment.h:117
Definition: Treatment.h:120
void loadVegPalatability(void)
Loads static member m_vegPalatability with data.
Definition: Hare_THare.cpp:114
Hare_Female * GetMum()
Get the mother pointer.
Definition: Hare_All.h:402
int m_ListNameLength
the number of life-stages simulated in the population manager
Definition: PopulationManager.h:626
Definition: Treatment.h:59
double GetMaxDailyGrowthEnergyF(int a_age)
Get the maximum daily energy needed for growth for this a_age for use in fat construction.
Definition: Hare_All.h:576
virtual void Dying()
A wrapped for KillThis - ideally should not be used.
Definition: PopulationManager.h:305
CfgFloat cfg_ForageRestingRatio("HARE_FORAGERESTRATIO", CFG_CUSTOM, 0.67)
double m_KJForaging
KJ/m cost of foraging per kg hare.
Definition: Hare_All.h:238
static CfgFloat cfg_HareStartWeight("HARE_STARTWEIGHT", CFG_CUSTOM, 121.0 *0.88 *0.33)
~Hare_Male() override
Destructor.
Definition: Hare_All.cpp:2426
Landscape * g_land
Definition: skylarks_all.cpp:44
Definition: Treatment.h:100
CfgFloat cfg_Hare_StdSpeedWalking("HARE_STD_SPEEDWALKING", CFG_CUSTOM, 0.65 *60)
bool ShouldMature()
Test for maturation.
Definition: Hare_All.cpp:2366
Definition: Treatment.h:150
const double g_PropSolidFood[36]
Definition: Hare_All.cpp:306
int m_HareThresholdDD
Input variable - Threshold density dependence level.
Definition: Hare_All.h:757
Definition: Treatment.h:124
Definition: Hare_All.h:94
double GetRMR()
Get todays RMR.
Definition: Hare_THare.cpp:333
Definition: Treatment.h:139
Definition: Treatment.h:126
double m_KJperM[7001]
Precalculated cost of locomotion - KJ per m per kg
Definition: Hare_All.h:795
float m_GoodYearBadYear
Variable for adding stochasticity.
Definition: Hare_All.h:749
void HuntingDifferentiatedBeetleBankArea(void)
Definition: Hare_All.cpp:3876
double Forage(int &time)
Foraging.
Definition: HareForagenPeg.cpp:52
virtual TTypeOfHareState st_Dispersal()
Base implementation only - reimplemented.
Definition: Hare_THare.cpp:122
void OutputToFile()
Definition: Hare_All.cpp:4061
void THareInit(int p_x, int p_y, THare_Population_Manager *p_PPM)
Object Initiation.
Definition: Hare_THare.cpp:70
Definition: Treatment.h:149
int GetPegDistance()
Get peg distance.
Definition: HareForagenPeg.cpp:442
Definition: Treatment.h:132
int RefNum
Definition: Hare_All.h:144
Definition: Treatment.h:148
bool value() const
Definition: Configurator.h:164
Definition: Treatment.h:67
double m_MortStochast
Stochasticity around mortality parameters
Definition: Hare_All.h:766
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
int m_trappings2
Definition: Hare_All.h:112
Definition: Hare_All.h:79
Definition: Treatment.h:42
vector< THare * > TListOfHares
Definition: Hare_All.h:46
Definition: Treatment.h:65
void Init()
Sets up data structures and calculations prior to starting simulation.
Definition: Hare_All.cpp:410
int GetYoungDensity(int x, int y)
Density function - returns the density of young in the square containing by x,y. Each square is 256x2...
Definition: Hare_All.h:661
~Hare_Infant() override
Hare infant destructor.
Definition: Hare_All.cpp:1201
Definition: Treatment.h:45
static CfgInt cfg_MRR4("HARE_MMRFOUR", CFG_CUSTOM, 999)
Definition: Treatment.h:98
int m_OestrousCounter
State variable - Days in oestrous.
Definition: Hare_All.h:1055
static CfgFloat cfg_hare_juvenile_predation("HARE_JUVENILE_PREDATION", CFG_CUSTOM, 0.0027)
AOR_Probe * m_AOR_Probe
A pointer to the AOR probe.
Definition: PopulationManager.h:875
CfgFloat cfg_Hare_StdSpeedRunning("HARE_STD_SPEEDRUNNING", CFG_CUSTOM, 13 *60)
Definition: LandscapeFarmingEnums.h:1060
std::mutex m_MyYoungMutex
mutex for the young list.
Definition: Hare_All.h:1065
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
static CfgFloat cfg_littersize_mean3("HARE_LITTERSIZE_MEAN_C", CFG_CUSTOM, 2.514)
virtual void TheRipleysOutputProbe(FILE *a_prb)
Standard spatial output.
Definition: Hare_All.cpp:1112
static CfgInt cfg_hare_firstyearsterility("HARE_FIRSTYEARSTERILITY", CFG_CUSTOM, 769)
static CfgFloat cfg_littersize_mean10("HARE_LITTERSIZE_MEAN_J", CFG_CUSTOM, 1.72)
The base class for all hare classes.
Definition: Hare_All.h:155
CfgInt cfg_HareHuntingThreshold("HARE_HUNTING_THRESHOLD", CFG_CUSTOM, 0)
static CfgFloat cfg_littersize_mean5("HARE_LITTERSIZE_MEAN_E", CFG_CUSTOM, 1.72)
Definition: Treatment.h:77
double m_KJWalking
KJ/m cost of walking per kg hare.
Definition: Hare_All.h:233
Definition: Treatment.h:96
bool OnFarmEvent(FarmToDo event) override
Do we require a response to a farm event.
Definition: Hare_All.cpp:1312
double g_FarmIntensiveness
Definition: Hare_All.cpp:300
int SimH
stores the simulation height
Definition: PopulationManager.h:614
Hare_Young(int p_x, int p_y, Landscape *p_L, THare_Population_Manager *p_PPM, double p_weight)
Constructor for Hare_Young.
Definition: Hare_All.cpp:1556
bool OnFarmEvent(FarmToDo event) override
Response to farm actions.
Definition: Hare_All.cpp:1817
static CfgFloat cfg_hare_young_predation("HARE_YOUNG_PREDATION", CFG_CUSTOM, 0.003)
static CfgFloat cfg_hare_juvenile_dispersal_threshold("HARE_JUVENILE_DISP_THESHOLD", CFG_CUSTOM, 99999)
double m_fatReserve
State variable - the energy reserve of the hare.
Definition: Hare_All.h:213
Definition: Treatment.h:54
void st_NextStage()
Maturation to Hare_Male or Hare_Female.
Definition: Hare_All.cpp:2345
virtual void DoProbe(int a_lifestage)
Definition: AOR_Probe.cpp:104
Definition: Treatment.h:130
int m_lastYearsDensity
State variable used in alternative density-dependent configurations.
Definition: Hare_All.h:276
Definition: Hare_All.h:75
Definition: Treatment.h:97
Definition: Treatment.h:141
void ExtraPopMort(void)
An extra global mortality
Definition: Hare_All.cpp:3784
Definition: Hare_All.h:81
double GetMaxDailyGrowthEnergy(int a_age)
Definition: Hare_All.h:550
int m_experiencedDensity
State variable used in alternative density-dependent configurations.
Definition: Hare_All.h:271
double m_KJRunning
KJ/m cost of running per kg hare.
Definition: Hare_All.h:228
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
static CfgFloat cfg_littersize_SD12("HARE_LITTERSIZE_SD_L", CFG_CUSTOM, 0.0)
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
CfgFloat cfg_HareHuntingBeetleBankArea("HARE_HUNTING_BEETLEBANKAREA", CFG_CUSTOM, 0.95)
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
static CfgInt cfg_juvenile_starvation_threshold("HARE_JUVENILE_STARVE_THRESHOLD", CFG_CUSTOM, 8)
Definition: Treatment.h:70
A simple class defining an x,y coordinate set.
Definition: ALMaSS_Setup.h:52
Definition: Treatment.h:46
int m_RefNum
Unique hare reference number, also functions as sex flag.
Definition: Hare_All.h:265
void ON_BeingFed(double a_someMilk)
Get energy from milk given.
Definition: Hare_All.cpp:1539
static CfgFloat cfg_littersize_mean7("HARE_LITTERSIZE_MEAN_G", CFG_CUSTOM, 2.17)
APoint CorrectCoordsPt(int x, int y)
Function to prevent wrap around errors with co-ordinates using x/y pair.
Definition: Landscape.h:2215
bool m_pesticideInfluenced1
Flag to indicate pesticide effects (e.g. can be used for endocrine distruptors with delayed effects u...
Definition: Hare_All.h:311
Definition: PopulationManager.h:63
CfgInt cfg_hare_minimum_breeding_weight("HARE_MIN_BREEDING_WT", CFG_CUSTOM, 780)
CfgInt cfg_hare_i_tillage("HARE_TILLAGE_MORT_INFANT", CFG_CUSTOM, 100)
TTypeOfHareState st_Resting()
Resting.
Definition: Hare_All.cpp:1750
APoint PlaceYoung()
Find somewhere nice for the babies to hide.
Definition: Hare_All.cpp:3448
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
void BeginStep(void) override
BeginStep for Hare_Young.
Definition: Hare_All.cpp:1587
const double g_MaxLeveretGrowthEnergy[36]
Definition: Hare_All.cpp:315
Bool configurator entry class.
Definition: Configurator.h:155
static TTypeOfHareState st_ReproBehaviour()
Currently Unused.
Definition: Hare_All.cpp:2754
void CheckManagement()
Used to start a check for any management related effects at the objects current location.
Definition: PopulationManager.cpp:1591
~Hare_Juvenile() override
Destructor for the juvenile hare object.
Definition: Hare_All.cpp:1994
void HuntingGrid(void)
Definition: Hare_All.cpp:3924
TTypeOfActivity
Enumerator for hare activities.
Definition: Hare_All.h:89
void Walking(int a_dist, int a_direction)
Walking.
Definition: Hare_THare.cpp:176
bool hare_tole_init_friendly(Landscape *m_TheLandscape, int x, int y)
Definition: Hare_toletov.cpp:4
Landscape * L
Definition: Hare_All.h:141
int m_NoYoung
State variable - current litter size.
Definition: Hare_All.h:1048
void SetWeight(double w)
Set the weight.
Definition: Hare_All.h:857
void Step(void) override
Step for Hare_Juvenile.
Definition: Hare_All.cpp:2060
static CfgFloat cfg_littersize_SD1("HARE_LITTERSIZE_SD_A", CFG_CUSTOM, 0.685)
int Supply_m_Location_y() const
Returns the ALMaSS y-coordinate.
Definition: PopulationManager.h:243
static CfgInt cfg_MRR5("HARE_MMRFIVE", CFG_CUSTOM, 270)
void EndStep(void) override
Base implementation only - reimplemented.
Definition: Hare_All.h:356
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
TTypeOfHareState st_ReproBehaviour()
Reproductive behaviour control.
Definition: Hare_All.cpp:3222
CfgFloat cfg_hare_adult_predation("HARE_ADULT_PREDATION", CFG_CUSTOM, 0.0015)
static CfgInt cfg_infant_starvation_threshold("HARE_INFANT_STARVE_THRESHOLD", CFG_CUSTOM, 4)
int m_shot
Hunting Bag.
Definition: Hare_All.h:764
Definition: Treatment.h:111
Definition: Treatment.h:135
Definition: Treatment.h:82
void SetSterile()
Female is sterile.
Definition: Hare_All.h:1100
void Init(double p_weight, int a_age, int a_Ref)
Object initiation.
Definition: Hare_All.cpp:2414
Definition: Hare_All.h:92
Definition: Treatment.h:63
void AllYoungMatured()
No more young to look after.
Definition: Hare_All.cpp:3622
double GetMaxDailyGrowthEnergyP(int a_age)
Get the maximum daily energy needed for growth for this a_age for use in protein construction.
Definition: Hare_All.h:563
void MovePeg()
Move the peg according to attraction forces.
Definition: HareForagenPeg.cpp:535
Definition: Hare_All.h:60
static CfgFloat cfg_littersize_SD7("HARE_LITTERSIZE_SD_G", CFG_CUSTOM, 0.955)
vector< MRR_Entry > m_Entries
Definition: Hare_All.h:129
Definition: Treatment.h:62
static CfgFloat cfg_littersize_mean1("HARE_LITTERSIZE_MEAN_A", CFG_CUSTOM, 1.535)
Definition: Treatment.h:119
Definition: Treatment.h:72
double GetRMR(int a_age, double a_size)
Returns the RMR given a specific age and mass.
Definition: Hare_All.cpp:932
Class for infant hares (stationary, only milk inputs)
Definition: Hare_All.h:824
TTypeOfHareState st_Resting()
Juvenile Resting.
Definition: Hare_All.cpp:2252
double m_SpeedRunning
m/min speed of running per kg hare
Definition: Hare_All.h:243
Definition: Treatment.h:112
Definition: Treatment.h:137
Definition: Treatment.h:40
void DoFirst() override
The first method called of the new time-step.
Definition: Hare_All.cpp:775
bool m_sterile
State variable - is/not sterile.
Definition: Hare_All.h:1052
double GetGrowthEfficiencyF(int a_age)
Get the growth efficiency for this a_age for creating fat.
Definition: Hare_All.h:615
Definition: Treatment.h:53
int m_HareRefNum
Definition: Hare_All.h:110
CfgInt cfg_BeetleBankMaxX
void POMOutputs()
This method is called to dump the information needed for POM approaches.
Definition: Hare_All.cpp:970
CfgInt cfg_hare_escape_dist("HARE_ESCAPE_DIST", CFG_CUSTOM, 100)
virtual void ON_Dead(void)
Mortality - overridden in descendent classes.
Definition: Hare_All.h:422
void IncTrapping()
Definition: Hare_All.h:124
double m_old_weight
State variale - last hare weight.
Definition: Hare_All.h:185
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Treatment.h:145
static CfgFloat cfg_hare_female_predation("HARE_FEMALE_PREDATION", CFG_CUSTOM, 0.0023)
int GetAge()
Provide the age of the hare.
Definition: Hare_All.h:375
int m_ddindex
State variable used in alternative density-dependent configurations.
Definition: Hare_All.h:281
Base class for all population managers for agent based models.
Definition: PopulationManager.h:645
Definition: Treatment.h:86
double m_TodaysEnergy
State variable - the amount of energy available today, can be in deficit.
Definition: Hare_All.h:218
Definition: Treatment.h:49
CfgFloat cfg_RMRrainFactor("HARE_RMRRAINFACTOR", CFG_CUSTOM, 0.47)
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
void EndStep(void) override
EndStep code for Hare_Young.
Definition: Hare_All.cpp:1684
Definition: Treatment.h:95
void st_NextStage()
Maturation to Hare_Juvenile.
Definition: Hare_All.cpp:1789
Definition: Treatment.h:147
static CfgInt cfg_ReproEndDay("HARE_REPROENDDAY", CFG_CUSTOM, 240)
CfgInt cfg_hare_y_cut("HARE_CUTTING_MORT_YOUNG", CFG_CUSTOM, 10)
~Hare_Young() override
Destructor for Hare_Young.
Definition: Hare_All.cpp:1578
CfgFloat cfg_VegHeightForageReduction("HARE_VEGHEIGHTFORAGEREDUCTION", CFG_CUSTOM, 0.01275)
Definition: Treatment.h:36
Definition: Hare_All.h:59
bool m_StepDone
Indicates whether the iterative step code is done for this timestep.
Definition: PopulationManager.h:133
bool m_is_paralleled
This is used to indicate whether the species is paralleled.
Definition: PopulationManager.h:796
TTypesOfPesticide SupplyPesticideType(void)
Gets type of pesticide effect from a reference.
Definition: Landscape.h:788
TTypeOfHareState st_Dispersal() override
Juvenile Dispersal.
Definition: Hare_All.cpp:2185
int m_x
Definition: ALMaSS_Setup.h:55
TListOfHares m_MyYoung
Pointer to litter.
Definition: Hare_All.h:1063
Definition: Treatment.h:116
Definition: Treatment.h:93
void ON_Dead() override
This hare has been killed.
Definition: Hare_All.cpp:1532
void EnergyBalance(TTypeOfActivity a_activity, int dist)
Adjust energy balance for an activity.
Definition: Hare_THare.cpp:261
TTypeOfHareState st_Developing() override
Male Development.
Definition: Hare_All.cpp:2661
Definition: Hare_All.h:97
Definition: Hare_All.h:73
int SupplyYearNumber(void)
Passes a request on to the associated Calendar class function, returns m_simulationyear
Definition: Landscape.h:2287
double m_LeveretMaterial
State variable - Mass of foetal material.
Definition: Hare_All.h:1059
Definition: Treatment.h:114
double m_foragingenergy
Energy obtained from foraging/feeding.
Definition: Hare_All.h:252
CfgInt cfg_hare_i_tractor("HARE_TRACTOR_MORT_INFANT", CFG_CUSTOM, 5)
static TTypeOfHareState st_Resting()
Male Resting.
Definition: Hare_All.cpp:2653
void ON_RemoveYoung(THare *a_young)
A leveret has matured.
Definition: Hare_All.cpp:3590
Class for running mark-release-recapture experiments.
Definition: Hare_All.h:119
int value() const
Definition: Configurator.h:116
static CfgInt cfg_MRR2("HARE_MMRTWO", CFG_CUSTOM, 999)
void ReInit(struct_Hare a_data)
Juvenile object reinitiation.
Definition: Hare_All.cpp:1974
CfgInt cfg_BeetleBankMinX
void ReInit(struct_Hare a_data)
Female object reinitiation.
Definition: Hare_All.cpp:2834
CfgFloat cfg_hare_foetusenergyproportion("HARE_FOETUSENERGYPROPORTION", CFG_CUSTOM, 0.024)
CfgFloat cfg_HareFemaleDensityDepValue("HARE_FEMALEDENDEPVALUE", CFG_CUSTOM, 0.1)
static CfgFloat cfg_hare_adult_breed_threshold("HARE_ADULT_BREED_THESHOLD", CFG_CUSTOM, 1600 *0.03)
static CfgFloat cfg_littersize_mean6("HARE_LITTERSIZE_MEAN_F", CFG_CUSTOM, 1.72)
double m_YoungMortRate
Input variable - Young mortality rate.
Definition: Hare_All.h:745
Definition: Treatment.h:75
double m_RMR[2][366]
Precalculated RMR with age
Definition: Hare_All.h:781
Definition: Treatment.h:47
CfgInt cfg_HareHuntingType("HARE_HUNTING_TYPE", CFG_CUSTOM, 0)
void dumpEnergy()
Used to record energetic status.
Definition: Hare_All.cpp:3174
void AllYoungKilled()
Last leveret predated.
Definition: Hare_All.cpp:3569
Definition: Treatment.h:106
int GetMaleDensity(int x, int y)
Density function - returns the density of males in the square containing by x,y. Each square is 256x2...
Definition: Hare_All.h:673
Definition: Treatment.h:109
int SimW
stores the simulation width
Definition: PopulationManager.h:616
int m_litter_no
State variable - current litter number.
Definition: Hare_All.h:1050
Definition: Treatment.h:48
CfgFloat cfg_HareMortStochasticity("HARE_MORTSTOCHASTICITY", CFG_CUSTOM, 1.0)
void st_Dying()
Tidy up before removing the object on death.
Definition: Hare_THare.cpp:165
double m_JuvMortRate
Input variable - Juvenile mortality rate.
Definition: Hare_All.h:737
int GetAdultDensity(int x, int y)
Density function - returns the density of adults in the square containing by x,y. Each square is 256x...
Definition: Hare_All.h:688
bool OpenTheRipleysOutputProbe()
Definition: PopulationManager.cpp:897
void ON_MumDead(Hare_Female *a_Mum)
Inform Mum that we are dead.
Definition: Hare_All.h:383
Class for young hares (low mobility, milk and solid food inputs)
Definition: Hare_All.h:879
CfgInt cfg_Hare_Recovery_Time("HARE_RECOVERY_TIME", CFG_CUSTOM, 10)
std::string EventtypeToString(int a_event)
Returns the text representation of a treatment type.
Definition: Landscape.cpp:6024
static CfgFloat cfg_littersize_mean11("HARE_LITTERSIZE_MEAN_K", CFG_CUSTOM, 1.72)
TTypesOfPesticide
Values that represent types of pesticide actions.
Definition: LandscapeFarmingEnums.h:1057
void UpdateGuardMap(int a_x, int a_y, int &a_index_x, int &a_index_y)
Get the index of the guard map for the given location.
Definition: PopulationManager.h:768
Definition: Hare_All.h:64
void Step(void) override
Female Step.
Definition: Hare_All.cpp:2942
CfgFloat l_pest_daily_mort
Daily mortality of pesticide with type 1.
Class for male hares.
Definition: Hare_All.h:981
int m_expDensity[365]
State variable used in alternative density-dependent configurations.
Definition: Hare_All.h:286
int m_DensitySum
State variable used in alternative density-dependent configurations.
Definition: Hare_All.h:291
const char * StateNames[100]
Definition: PopulationManager.h:801
const double g_hare_maxFoetalKJ[41]
Definition: Hare_All.cpp:366
Definition: Treatment.h:146
static CfgFloat cfg_hare_adult_dispersal_threshold("HARE_ADULT_DISP_THESHOLD", CFG_CUSTOM, 1600 *0.02)
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
FILE * BodyBurdenPrb
Definition: Hare_All.h:799
Definition: Treatment.h:134
double ForageSquareP(int a_x, int a_y, double *a_pestexposure)
Forage from an area and resturn pesticide exposure as well as food.
Definition: HareForagenPeg.cpp:375
double m_pesticide_burden
State variable used to hold the current body-burden of pesticide.
Definition: Hare_All.h:301
void MRROutputs()
Special probe for data to be used in mark-release-recapture simulations.
Definition: Hare_All.cpp:1142
void ReInit(struct_Hare a_data)
Infant object reinitiation.
Definition: Hare_All.cpp:1185
int m_Age
State variale - hare age.
Definition: Hare_All.h:170
double SupplyRain(void)
Passes a request on to the associated Weather class function, the amount of rain for the current day.
Definition: Landscape.h:1971
void BeginStep(void) override
Female BeginStep.
Definition: Hare_All.cpp:2869
double ForageP(int &time)
Foraging but also incorporating pesticide exposure.
Definition: HareForagenPeg.cpp:179
Definition: Treatment.h:118
MRR_Data()
Definition: Hare_All.cpp:3999
void Step(void) override
Step code for Hare_Young.
Definition: Hare_All.cpp:1635
int m_currenttrapping
Definition: Hare_All.h:128
Definition: Treatment.h:113
static CfgInt cfg_hare_DaysToGestation("HARE_GESTATIONDAYS", CFG_CUSTOM, 41)
bool OpenTheReallyBigProbe()
Definition: PopulationManager.cpp:1005
double SupplyVegHeight(int a_polyref)
Returns the height of the vegetation using the polygon reference number a_polyref or based on the x,...
Definition: Landscape.h:1527
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
unsigned GetLiveArraySize(int a_listindex) override
Gets the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:657
Definition: Hare_All.h:74
TTypeOfHareState m_CurrentHState
Defines the current activity.
Definition: Hare_All.h:165
static CfgFloat cfg_littersize_mean8("HARE_LITTERSIZE_MEAN_H", CFG_CUSTOM, 1.72)
CfgBool cfg_CfgRipleysOutputUsed
void Step(void) override
Base implementation only - reimplemented.
Definition: Hare_All.h:350
static CfgInt cfg_MRR_FirstYear("HARE_MRRFIRSTYEAR", CFG_CUSTOM, 10000)
bool m_IamSick
flag for sickness - used in conjunction with disease configurations
Definition: Hare_All.h:295
static CfgFloat cfg_AdultMaxFat("HARE_ADULT_MAXFAT", CFG_CUSTOM, 0.04/(0.33 *0.88))
Class used to pass hare information to CreateObjects.
Definition: Hare_All.h:136
double GetInterference(int h)
Return the proportion of time used in communicating with con-specifics.
Definition: Hare_All.h:707
bool ON_AreYouMyMum(THare *a_young)
Debug function.
Definition: Hare_All.cpp:3664
Definition: LandscapeFarmingEnums.h:1061
void GeneralEndocrineDisruptor(double a_pesticide_dose) override
Handles internal effects of endocrine distrupter pesticide exposure for female.
Definition: Hare_All.cpp:4101
void GeneralOrganoPhosphate(double a_pesticide_dose) override
Handles internal effects of organophosphate pesticide exposure for female.
Definition: Hare_All.cpp:4110
Definition: Treatment.h:41
Hare_Male(int p_x, int p_y, Landscape *p_L, THare_Population_Manager *p_PPM, double p_weight, int a_age, int a_Ref)
Constructor.
Definition: Hare_All.cpp:2402
Integer configurator entry class.
Definition: Configurator.h:102
static CfgFloat cfg_hare_male_predation("HARE_MALE_PREDATION", CFG_CUSTOM, 0.0023)
void CalcLitterSize(double mean, double SD, int litter)
Definition: Hare_All.cpp:665
THare(int p_x, int p_y, Landscape *p_L, THare_Population_Manager *p_PPM)
Constructor.
Definition: Hare_THare.cpp:64
Definition: Treatment.h:37
double GetPolyFood(int a_poly)
Get stored polygon food quality.
Definition: Hare_All.h:720
CfgInt cfg_BeetleBankMinY
int m_guard_cell_x
The index x to the guard cell.
Definition: PopulationManager.h:370
Definition: Treatment.h:79
virtual void GeneralEndocrineDisruptor(double)
Handles internal effects of endocrine distrupter pesticide exposure. If any effects are needed this m...
Definition: Hare_All.h:480
int m_StarvationDays
State variable - the number of consecutive days in negative energy balance.
Definition: Hare_All.h:208
void DoLactation()
Lactation.
Definition: Hare_All.cpp:3479
static CfgFloat cfg_littersize_SD9("HARE_LITTERSIZE_SD_I", CFG_CUSTOM, 0.6415)
void Hunting(void)
Definition: Hare_All.cpp:3827
CfgInt cfg_HareThresholdDD("HARE_THRESHOLDDD", CFG_CUSTOM, 5)
void EndStep(void) override
EndStep code for Hare_Male.
Definition: Hare_All.cpp:2583
Definition: Treatment.h:74
Definition: Hare_All.h:95
Double configurator entry class.
Definition: Configurator.h:126
MRR_Data * m_OurMRRData
Data structure for MarkReleaseRecapture expts
Definition: Hare_All.h:797
string m_SimulationName
stores the simulation name
Definition: PopulationManager.h:622
double m_MaxDailyGrowthEnergy[5001]
Precalculated max growth energy requirement with size
Definition: Hare_All.h:783
void TheAOROutputProbe() override
Output method.
Definition: Hare_All.cpp:1129
Definition: Treatment.h:136
Definition: Treatment.h:108
virtual unsigned SupplyListIndexSize()
Definition: PopulationManager.h:725
Definition: Treatment.h:69
Definition: Treatment.h:105
Definition: Hare_All.h:91
TTypeOfHareState
Enumerator for hare behavioural states.
Definition: Hare_All.h:71
TTypeOfHareState st_Developing()
Developmental behaviour for the infant hare.
Definition: Hare_All.cpp:1461
Definition: Hare_All.h:63
CfgInt cfg_CatastropheEventStartYear
double GetTotalWeight()
Provide the wet weight of the hare.
Definition: Hare_All.h:370
static CfgFloat cfg_hare_peg_inertia("HARE_PEG_INERTIA", CFG_CUSTOM, 1.00)
CfgInt cfg_adult_starve("HARE_ADULT_STARVE", CFG_CUSTOM, 5000)
Hare_Infant(int p_x, int p_y, Landscape *p_L, THare_Population_Manager *p_PPM)
Hare infant constructor.
Definition: Hare_All.cpp:1183
Data entry for mark release recapture data MRR_Data.
Definition: Hare_All.h:109
Definition: Treatment.h:78
static CfgFloat cfg_littersize_SD3("HARE_LITTERSIZE_SD_C", CFG_CUSTOM, 0.955)
virtual void Running(int a_max_dist)
Run.
Definition: Hare_THare.cpp:136
int GetPegPull()
Get attractive force of peg.
Definition: HareForagenPeg.cpp:458
Hare_Female * m_MyMum
Pointer to the hare's mum.
Definition: Hare_All.h:190
static void SetMum(Hare_Female *)
Set the mother pointer. Reimplemented in Hare_Infant.
Definition: Hare_All.h:396
Definition: Configurator.h:70
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
void AddEntry(int a_RefNum)
Definition: Hare_All.cpp:4007
Definition: Treatment.h:66
Definition: Treatment.h:92
void UpdateGestation()
Update gestation counter.
Definition: Hare_All.cpp:3326
Definition: Treatment.h:102
TTypeOfActivity m_reproActivity
State variable - current reproductive state.
Definition: Hare_All.h:1061
int m_peg_y
peg y-coordinate
Definition: Hare_All.h:260
double m_weight
State variale - hare weight g.
Definition: Hare_All.h:180
void SetMum(Hare_Female *a_af)
Set the mother pointer.
Definition: Hare_All.h:862
TTypeOfHareState st_Foraging()
Juvenile foraging.
Definition: Hare_All.cpp:2127
int GetJuvenileDensity(int x, int y)
Density function - returns the density of juveniles in the square containing by x,...
Definition: Hare_All.h:666
double GetGrowthEfficiencyP(int a_age)
Get the growth efficiency for this a_age for creating protein.
Definition: Hare_All.h:602
Hare_Object m_Type
State variale - the type of hare.
Definition: Hare_All.h:175
CfgFloat cfg_hare_ExtEff("HARE_EXTEFF", CFG_CUSTOM, 5.8)
bool Run(int a_dist, int a_direction)
Run a distance in a direction.
Definition: Hare_THare.cpp:186
Definition: Hare_All.h:77
CfgInt cfg_fixadult_starve("HARE_FIXADULT_STARVE", CFG_CUSTOM, 370)
Definition: Treatment.h:57
Definition: Treatment.h:87
int m_CurrentStateNo
The basic state number for all objects - '-1' indicates death.
Definition: PopulationManager.h:131
void PushIndividual(const unsigned a_listindex, TAnimal *a_individual_ptr)
Definition: PopulationManager.cpp:1682
void SubtractHareDensity(int x, int y, Hare_Object a_type)
Density function - subtracts one from the density in the square containing by x,y....
Definition: Hare_All.h:649
TTypeOfHareState st_Foraging()
Male Foraging.
Definition: Hare_All.cpp:2595
double GetGrowthEfficiency(int a_age)
Get the growth efficiency for this a_age.
Definition: Hare_All.h:589
Definition: Treatment.h:101
void Init(double p_weight, int a_age, int a_Ref)
Object initiation.
Definition: Hare_All.cpp:2844
TTypeOfHareState st_Developing()
Developmental code for the young hare.
Definition: Hare_All.cpp:1761
Definition: Treatment.h:73
double weight
Definition: Hare_All.h:145
CfgInt cfg_hare_max_age("HARE_MAX_AGE", CFG_CUSTOM, static_cast< int >(365 *12.5))
ofstream * ReallyBigOutputPrb
Definition: PopulationManager.h:873
void BeginStep(void) override
Base implementation only - reimplemented.
Definition: Hare_All.h:344
void DoLast() override
The last method called before the new time-step starts.
Definition: Hare_All.cpp:714
Hare_Juvenile(int p_x, int p_y, Landscape *p_L, THare_Population_Manager *p_PPM, double p_weight)
Constructor for the juvenile hare object.
Definition: Hare_All.cpp:1972
int SupplyLargestPolyNumUsed()
Returns m_LargestPolyNumUsed.
Definition: Landscape.h:578
double m_GrowthEfficiency[5001]
Precalculated growth efficiency with size
Definition: Hare_All.h:785
double g_RMRrainFactor
Definition: Hare_All.cpp:49
Definition: Treatment.h:55
CfgFloat cfg_JuvDDScale("HARE_JUVSCALEDD", CFG_CUSTOM, 0.5)
Definition: Hare_All.h:78
Definition: Hare_All.h:96
virtual TTypeOfHareState st_Developing()
The development code for Hare_Juvenile.
Definition: Hare_All.cpp:2266
void TimeBudget(TTypeOfActivity a_activity, int dist)
Adjust time budger for an activity.
Definition: Hare_THare.cpp:298
void Warn(std::string a_msg1, std::string a_msg2)
Wrapper for the g_msg Warn function.
Definition: Landscape.h:2250
CfgFloat cfg_min_growth_attain("HARE_MINGROWTHATTAIN", CFG_CUSTOM, 0.475)
int GetTotalDensity(int x, int y)
Density function - returns the density of all hares in the square containing by x,...
Definition: Hare_All.h:683
static TTypeOfHareState st_Resting()
Resting.
Definition: Hare_All.cpp:3210
static CfgInt cfg_MRR_LastYear("HARE_MRRLASTYEAR", CFG_CUSTOM, 0)
int m_trappings1
Definition: Hare_All.h:111
Hare_Female(int p_x, int p_y, Landscape *p_L, THare_Population_Manager *p_PPM, double p_weight, int a_age, int a_Ref)
Female Constructor.
Definition: Hare_All.cpp:2830
CfgInt cfg_BeetleBankMaxY
CfgInt cfg_HareFemaleReproMortValue("HARE_FEMALEREPROMORT", CFG_CUSTOM, 0)
Definition: Treatment.h:34
TTypesOfCrops SupplyCropType(int a_x, int a_y)
Returns the crop type of the polygon using the polygon reference number a_polyref or coordinates a_x,...
Definition: Landscape.h:1931
static CfgFloat cfg_hare_proximity_alert("HARE_PROXIMITY_ALERT", CFG_CUSTOM, 0.05)
void AddYoung(THare *a_new)
Add a leveret to the list of kids.
Definition: Hare_All.cpp:3535
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
Definition: Hare_All.h:98
static CfgInt cfg_MRR3("HARE_MMRTHREE", CFG_CUSTOM, 999)
double m_Interference[2500]
Array storing density-dependence effect with density
Definition: Hare_All.h:768
static CfgInt cfg_hare_sex_ratio("HARE_SEX_RATIO", CFG_CUSTOM, 50)
int SupplyPolyRef(int a_x, int a_y)
Get the in map polygon reference number from the x, y location.
Definition: Landscape.h:2157
void BodyBurdenOut(int a_year, int a_day, double a_bb, double a_mgkg)
BodyBurden output.
Definition: Hare_All.h:730
Definition: Treatment.h:58
CfgBool cfg_AorOutput_used
void EndStep(void) override
EndStep for the Hare_Infant.
Definition: Hare_All.cpp:1295
Definition: Treatment.h:128
static CfgInt cfg_hare_StartingNo("HARE_START_NO", CFG_CUSTOM, 1000)
CfgFloat cfg_FarmIntensiveness("HARE_FARMINTENSIVENESS", CFG_CUSTOM, 0.01)
Class for juvenile hares (after 5 weeks old, fully mobile)
Definition: Hare_All.h:930
int GetDelayedAdultDensity(int x, int y)
Density function - returns the density of adults in the square containing by x,y, but for last year a...
Definition: Hare_All.h:696
CfgFloat cfg_HareMaleDensityDepValue("HARE_MALEDENDEPVALUE", CFG_CUSTOM, 0.1)
void Catastrophe() override
Annual global change in population numbers.
Definition: Hare_All.cpp:3730
static CfgFloat cfg_littersize_mean9("HARE_LITTERSIZE_MEAN_I", CFG_CUSTOM, 1.72)
int m_peg_x
peg x-coordinate
Definition: Hare_All.h:256
Definition: LandscapeFarmingEnums.h:1059
int m_y
Definition: ALMaSS_Setup.h:56
int m_RefNums
The last hare ID used
Definition: Hare_All.h:762
void UpdateOestrous()
Update oestrous counter.
Definition: Hare_All.cpp:3280
Definition: Treatment.h:50
double GetHareFoodQuality(int a_polygon)
Returns the hare food quality of polygons LKM.
Definition: Landscape.cpp:5472
TTypeOfHareState st_Dispersal() override
Female Dispersal.
Definition: Hare_All.cpp:3187
Definition: Treatment.h:115
int m_GestationCounter
State variable - Days in gestation.
Definition: Hare_All.h:1057
Definition: Hare_All.h:90
Definition: Hare_All.h:76
const int October
Julian start dates of the month of October.
Definition: Landscape.h:56
void GeneralOrganoPhosphate(double) override
Handles internal effects of organophosphate pesticide exposure. If any effects are needed this method...
Definition: Hare_All.cpp:2816
Definition: Hare_All.h:99
Definition: Treatment.h:33
CfgFloat cfg_HareWalkingPowerConst("HARE_WALKINGPOWERCONST", CFG_CUSTOM, -0.316)
Definition: Treatment.h:107
void IncLiveArraySize(int a_listindex)
Increments the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:665