File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
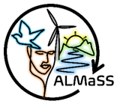 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
#include <Rastermap.h>
|
int | MapWidth (void) |
|
int | MapHeight (void) |
|
int | Get (int a_x, int a_y) |
|
char * | GetID (void) |
|
int * | GetMagicP (int a_x, int a_y) |
|
void | Put (int a_x, int a_y, int a_elem) |
|
| RasterMap (const char *a_mapfile, Landscape *m_landscape) |
|
| ~RasterMap (void) |
|
int | CellReplacementNeighbour (int a_x, int a_y, int a_polyref) |
| A method for helping remove tiny polygons. More...
|
|
bool | MissingCellReplace (int a_x, int a_y, bool a_fieldsonly) |
| A method for removing missing polygons. More...
|
|
bool | MissingCellReplaceWrap (int a_x, int a_y, bool a_fieldsonly) |
| A method for removing missing polygons - tests for edge conditions. More...
|
|
◆ RasterMap()
RasterMap::RasterMap |
( |
const char * |
a_mapfile, |
|
|
Landscape * |
m_landscape |
|
) |
| |
◆ ~RasterMap()
RasterMap::~RasterMap |
( |
void |
| ) |
|
◆ CellReplacementNeighbour()
int RasterMap::CellReplacementNeighbour |
( |
int |
a_x, |
|
|
int |
a_y, |
|
|
int |
a_polyref |
|
) |
| |
A method for helping remove tiny polygons.
Replaces a cell value with the most common value from the surrounding 8 cells. If near the edge nothing is done. Return value is the new value of the cell or -1 if nothing is done. First tests to make sure that the polygon we are removing is at these coords.
161 if ((a_x < 1) || (a_x >
m_width - 2) || (a_y < 1) || (a_y >
m_height - 2))
return -1;
162 int toreplace =
Get(a_x, a_y);
163 if (toreplace != a_polyref)
165 g_msg->
Warn(
"RasterMap::CellReplacementNeighbour: x,y pair does not match polyref ", a_polyref);
168 int surroundingcells[8];
169 surroundingcells[0] =
Get(a_x - 1, a_y - 1);
170 surroundingcells[1] =
Get(a_x , a_y - 1);
171 surroundingcells[2] =
Get(a_x + 1, a_y - 1);
172 surroundingcells[3] =
Get(a_x - 1, a_y);
173 surroundingcells[4] =
Get(a_x + 1, a_y);
174 surroundingcells[5] =
Get(a_x - 1, a_y + 1);
175 surroundingcells[6] =
Get(a_x , a_y + 1);
176 surroundingcells[7] =
Get(a_x + 1, a_y + 1);
179 for (
int i = 0; i < 8; i++)
182 for (
int j = 0; j < 8; j++)
184 if (surroundingcells[j] == surroundingcells[i])
count[i]++;
189 for (
int i = 0; i < 8; i++)
191 if (
count[i]>found) index = i;
197 Put(a_x, a_y, surroundingcells[index]);
References count, g_msg, and MapErrorMsg::Warn().
◆ Get()
int RasterMap::Get |
( |
int |
a_x, |
|
|
int |
a_y |
|
) |
| |
|
inline |
References g_msg, MapErrorMsg::Warn(), WARN_BUG, and MapErrorMsg::WarnAddInfo().
Referenced by Landscape::GetActualGooseGrazingForage(), Landscape::GetOwner_tole(), PesticideMap::Spray(), Landscape::SupplyAttIsForest(), Landscape::SupplyAttIsHigh(), Landscape::SupplyAttIsUrbanNoVeg(), Landscape::SupplyAttIsVegGrass(), Landscape::SupplyAttIsVegPatchy(), Landscape::SupplyAttIsWater(), Landscape::SupplyAttIsWoody(), Landscape::SupplyAttUserDefinedBool(), Landscape::SupplyBirdMaizeForage(), Landscape::SupplyBirdSeedForage(), Landscape::SupplyCentroidX(), Landscape::SupplyCentroidY(), Landscape::SupplyCountryDesig(), Landscape::SupplyCropType(), Landscape::SupplyDeadBiomass(), Landscape::SupplyElementSubType(), Landscape::SupplyElementType(), Landscape::SupplyElementTypeCC(), Landscape::SupplyFarmOwner(), Landscape::SupplyFarmOwnerIndex(), Landscape::SupplyFarmRotFilename(), Landscape::SupplyFarmType(), Landscape::SupplyGrazingPressure(), Landscape::SupplyGreenBiomass(), Landscape::SupplyGreenBiomassProp(), Landscape::SupplyGreenBiomassTotal(), Landscape::SupplyHasTramlines(), Landscape::SupplyInsects(), Landscape::SupplyInterestedGreenBiomass(), Landscape::SupplyInterestedGreenBiomassTotal(), Landscape::SupplyIsVeg(), Landscape::SupplyJustSprayed(), Landscape::SupplyLAGreen(), Landscape::SupplyLastSownVeg(), Landscape::SupplyLastTreatment(), Landscape::SupplyLATotal(), Landscape::SupplyNectar(), Landscape::SupplyOpenness(), Landscape::SupplyOwner_tole(), Landscape::SupplyPollen(), Landscape::SupplyPolyLEptr(), Landscape::SupplyPolyRef(), Landscape::SupplyPolyRefCC(), Landscape::SupplyPolyRefIndex(), Landscape::SupplyRoadWidth(), Landscape::SupplySoilType(), Landscape::SupplySoilTypeR(), Landscape::SupplySugar(), Landscape::SupplyTotalNectar(), Landscape::SupplyTotalPollen(), Landscape::SupplyTrafficLoad(), Landscape::SupplyUMRef(), Landscape::SupplyVegAge(), Landscape::SupplyVegBiomass(), Landscape::SupplyVegCover(), Landscape::SupplyVegDensity(), Landscape::SupplyVegDigestibility(), Landscape::SupplyVegGrowthStage(), Landscape::SupplyVegHeight(), Landscape::SupplyVegType(), Landscape::SupplyWeedBiomass(), and Pesticide::TwinMapSpray().
◆ GetID()
char* RasterMap::GetID |
( |
void |
| ) |
|
|
inline |
◆ GetMagicP()
int * RasterMap::GetMagicP |
( |
int |
a_x, |
|
|
int |
a_y |
|
) |
| |
|
inline |
◆ Init()
void RasterMap::Init |
( |
const char * |
a_mapfile, |
|
|
Landscape * |
m_landscape |
|
) |
| |
|
protected |
65 ifstream IFile(a_mapfile, ios::binary);
66 if (!IFile.is_open()) {
74 IFile.read((
char*)&
m_width,
sizeof(
int));
75 IFile.read((
char*)&
m_height,
sizeof(
int));
80 "RasterMap::RasterMap(): Out of memory.",
"");
92 for (
int x = 0; x < mx; x++)
94 for (
int y = 0; y < my; y++)
101 sprintf(error_num,
"%d", pref);
102 g_msg->
Warn(
WARN_FILE,
"RasterMap::RasterMap(): Unknown/Negative polygon map number (could be a hole):", error_num);
108 sprintf(error_num,
"%d", lastref);
109 g_msg->
Warn(
WARN_FILE,
"Last polygon map number was good though, using it:", error_num);
125 sprintf(error_num,
"%d",
Get(x, y));
126 g_msg->
Warn(
WARN_FILE,
"RasterMap::RasterMap():" " Unknown polygon ref number:", error_num);
134 sprintf(error_num,
"%d",
Get(x, y));
135 g_msg->
Warn(
WARN_FILE,
"RasterMap::RasterMap():" " Unknown polygon ref number:", error_num);
References g_msg, MapErrorMsg::Warn(), WARN_FATAL, WARN_FILE, and MapErrorMsg::WarnAddInfo().
◆ MapHeight()
int RasterMap::MapHeight |
( |
void |
| ) |
|
|
inline |
◆ MapWidth()
int RasterMap::MapWidth |
( |
void |
| ) |
|
|
inline |
◆ MissingCellReplace()
bool RasterMap::MissingCellReplace |
( |
int |
a_x, |
|
|
int |
a_y, |
|
|
bool |
a_fieldsonly |
|
) |
| |
A method for removing missing polygons.
203 int surroundingcells[8];
204 surroundingcells[0] =
Get(a_x - 1, a_y - 1);
205 surroundingcells[1] =
Get(a_x, a_y - 1);
206 surroundingcells[2] =
Get(a_x + 1, a_y - 1);
207 surroundingcells[3] =
Get(a_x - 1, a_y);
208 surroundingcells[4] =
Get(a_x + 1, a_y);
209 surroundingcells[5] =
Get(a_x - 1, a_y + 1);
210 surroundingcells[6] =
Get(a_x, a_y + 1);
211 surroundingcells[7] =
Get(a_x + 1, a_y + 1);
216 for (
unsigned i = 0; i < 8; i++)
221 Put(a_x, a_y, surroundingcells[(i + offset) % 8]);
References g_random_fnc().
◆ MissingCellReplaceWrap()
bool RasterMap::MissingCellReplaceWrap |
( |
int |
a_x, |
|
|
int |
a_y, |
|
|
bool |
a_fieldsonly |
|
) |
| |
A method for removing missing polygons - tests for edge conditions.
232 int surroundingcells[8];
233 if (a_x >= 1 && a_y >= 1) surroundingcells[0] =
Get(a_x - 1, a_y - 1);
else surroundingcells[0] =
Get(a_x, a_y);
234 if (a_y >= 1) surroundingcells[1] =
Get(a_x, a_y - 1);
else surroundingcells[1] =
Get(a_x, a_y);
235 if (a_x < m_width-1 && a_y >= 1) surroundingcells[2] =
Get(a_x + 1, a_y - 1);
else surroundingcells[2] =
Get(a_x, a_y);
236 if (a_x >= 1) surroundingcells[3] =
Get(a_x - 1, a_y);
else surroundingcells[3] =
Get(a_x, a_y);
237 if (a_x <
m_width-1) surroundingcells[4] =
Get(a_x + 1, a_y);
else surroundingcells[4] =
Get(a_x, a_y);
238 if (a_x >= 1 && a_y <
m_height-1) surroundingcells[5] =
Get(a_x - 1, a_y + 1);
else surroundingcells[5] =
Get(a_x, a_y);
239 if (a_y <
m_height-1) surroundingcells[6] =
Get(a_x, a_y + 1);
else surroundingcells[6] =
Get(a_x, a_y);
240 if (a_x <
m_width-1 && a_y <
m_height-1) surroundingcells[7] =
Get(a_x + 1, a_y + 1);
else surroundingcells[7] =
Get(a_x, a_y);
245 for (
unsigned i = 0; i < 8; i++)
252 Put(a_x, a_y, surroundingcells[(i + offset) % 8]);
References g_random_fnc(), tole_Field, tole_Orchard, tole_PermanentSetaside, tole_PermPasture, tole_PermPastureLowYield, tole_PermPastureTussocky, tole_PermPastureTussockyWet, and tole_Vildtager.
◆ Put()
void RasterMap::Put |
( |
int |
a_x, |
|
|
int |
a_y, |
|
|
int |
a_elem |
|
) |
| |
|
inline |
◆ m_height
The height of the landscape.
◆ m_id
A 12 character ID for the map.
◆ m_landscape
A pointer to the parent landscape class.
◆ m_map
The raster of the landscape.
◆ m_width
The width of the landscape.
The documentation for this class was generated from the following files:
Definition: MapErrorMsg.h:37
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
Landscape * m_landscape
A pointer to the parent landscape class.
Definition: Rastermap.h:51
void WarnAddInfo(MapErrorState a_level, std::string a_add1, std::string a_add2)
Definition: MapErrorMsg.cpp:160
int Get(int a_x, int a_y)
Definition: Rastermap.h:82
Definition: LandscapeFarmingEnums.h:122
Definition: MapErrorMsg.h:35
void Put(int a_x, int a_y, int a_elem)
Definition: Rastermap.h:60
Definition: LandscapeFarmingEnums.h:68
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
char m_id[12]
A 12 character ID for the map.
Definition: Rastermap.h:43
int m_height
The height of the landscape.
Definition: Rastermap.h:49
bool IsFieldType(TTypesOfLandscapeElement a_tole)
Function for backwards code compatibility when TOV attributes would now be used.
Definition: Landscape.h:998
Definition: LandscapeFarmingEnums.h:66
int * m_map
The raster of the landscape.
Definition: Rastermap.h:45
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
double SupplyPolygonArea(int a_polyref)
Returns the polygon area of a particular polygon referenced by polygon.
Definition: Landscape.h:1058
Definition: LandscapeFarmingEnums.h:67
int m_width
The width of the landscape.
Definition: Rastermap.h:47
void Init(const char *a_mapfile, Landscape *m_landscape)
Definition: Rastermap.cpp:58
int SupplyPolymapping(int a_index)
Returns the value of the m_polymapping array indexed by a_index.
Definition: Landscape.h:329
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: LandscapeFarmingEnums.h:93
Definition: LandscapeFarmingEnums.h:65
Definition: LandscapeFarmingEnums.h:121
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
Definition: SubPopulation.h:48
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:69