File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
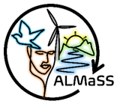 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
10 #define TESTCROP_BASE 1
14 #define TC_WW_FERTI_P1 a_field->m_user[1]
15 #define TC_WW_FERTI_S1 a_field->m_user[2]
16 #define TC_WW_STUBBLE_PLOUGH a_field->m_user[3]
17 #define TC_WW_DECIDE_TO_GR a_field->m_user[4]
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: TestCrop.h:59
Definition: LandscapeFarmingEnums.h:1005
#define TC_WW_DECIDE_TO_GR
Definition: TestCrop.h:17
CfgFloat cfg_WW_NINV_tillage_prop2
Provided to allow configuration control of the proportion of farmers doing second non-inversion tilla...
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
bool IsStockFarmer(void)
Definition: Farm.h:961
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
bool m_lock
Definition: Farm.h:384
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
int GetMConstants(int a)
Definition: Elements.h:407
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: TestCrop.h:41
int GetYearNumber(void)
Definition: Calendar.h:72
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: TestCrop.cpp:54
Definition: TestCrop.h:56
bool m_first_year
Definition: Farm.h:386
CfgBool cfg_pest_winterwheat_on
Turn on pesticides for winter wheat.
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
TestCropToDo
Definition: TestCrop.h:24
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
CfgFloat cfg_WW_NINV_tillage_prop1
Provided to allow configuration control of the proportion of farmers doing first non-inversion tillag...
Farmer * GetFarmer()
Returns the pointer to this farm's farmer.
Definition: Farm.h:817
Definition: LandscapeFarmingEnums.h:1006
Definition: TestCrop.h:60
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
Definition: TestCrop.h:52
Definition: TestCrop.h:29
double value() const
Definition: Configurator.h:142
Definition: TestCrop.h:54
Definition: TestCrop.h:42
Definition: TestCrop.h:67
Definition: TestCrop.h:69
TTypesOfFarm GetType(void)
Definition: Farm.h:956
Definition: TestCrop.h:55
int m_base_elements_no
Definition: Farm.h:505
Definition: TestCrop.h:25
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
Definition: TestCrop.h:66
Definition: TestCrop.h:53
Definition: TestCrop.h:77
The base class for all crops.
Definition: Farm.h:495
Definition: TestCrop.h:48
bool value() const
Definition: Configurator.h:164
Definition: TestCrop.h:32
#define TC_WW_FERTI_P1
A flag used to indicate autumn ploughing status.
Definition: TestCrop.h:14
Definition: TestCrop.h:37
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
TestCrop(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: TestCrop.h:81
Definition: TestCrop.h:43
Definition: TestCrop.h:40
Definition: TestCrop.h:64
long Date(void)
Definition: Calendar.h:57
Definition: TestCrop.h:28
Definition: TestCrop.h:70
Definition: TestCrop.h:31
Definition: LandscapeFarmingEnums.h:1008
Bool configurator entry class.
Definition: Configurator.h:155
Definition: TestCrop.h:47
CfgInt cfg_WW_InsecticideMonth
Provided to allow configuration control of the first insecticide spray in winter wheat - this changes...
Definition: LandscapeFarmingEnums.h:1003
Definition: TestCrop.h:46
CfgFloat cfg_WW_isecticide_prop1
Provided to allow configuration control of the proportion of farmers doing first insecticide spray in...
Definition: TestCrop.h:36
long OldDays(void)
Definition: Calendar.h:60
Definition: TestCrop.h:58
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
int GetRotIndex(void)
Definition: Elements.h:373
Definition: TestCrop.h:30
CfgInt cfg_WW_InsecticideDay
Provided to allow configuration control of the first insecticide spray in winter wheat - this changes...
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
CfgFloat cfg_WW_isecticide_prop2
Provided to allow configuration control of the proportion of farmers doing second insecticide spray i...
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
Definition: TestCrop.h:45
int m_todo
Definition: Farm.h:388
Definition: TestCrop.h:72
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
Definition: TestCrop.h:39
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: LandscapeFarmingEnums.h:696
Definition: Elements.h:86
Definition: TestCrop.h:34
void SetUpFarmCategoryInformation()
Definition: TestCrop.h:89
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
CfgFloat cfg_WW_isecticide_prop3
Provided to allow configuration control of the proportion of farmers doing third insecticide spray in...
CfgFloat cfg_WW_conv_tillage_prop1
Provided to allow configuration control of the proportion of farmers doing first conventional tillage...
Definition: TestCrop.h:35
Definition: TestCrop.h:65
Definition: TestCrop.h:61
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
#define TESTCROP_BASE
Definition: TestCrop.h:10
The base class for all farm types.
Definition: Farm.h:755
Definition: TestCrop.h:71
CfgFloat cfg_WW_conv_tillage_prop2
Provided to allow configuration control of the proportion of farmers doing second conventional tillag...
Double configurator entry class.
Definition: Configurator.h:126
Definition: TestCrop.h:63
Definition: LandscapeFarmingEnums.h:1012
Definition: TestCrop.h:26
Definition: TestCrop.h:68
Definition: TestCrop.h:27
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Definition: TestCrop.h:49
#define TC_WW_FERTI_S1
Definition: TestCrop.h:15
Definition: TestCrop.h:33
Definition: TestCrop.h:62
Definition: TestCrop.h:50
Definition: TestCrop.h:73
Definition: TestCrop.h:57
Definition: TestCrop.h:38
void SetMConstants(int a, int c)
Definition: Elements.h:408
#define TC_WW_STUBBLE_PLOUGH
Definition: TestCrop.h:16
CfgFloat l_pest_insecticide_amount
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: TestCrop.h:44
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751
Definition: TestCrop.h:51