File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
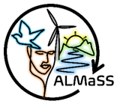 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
33 #ifndef SubPopulation_Population_ManagerH
34 #define SubPopulation_Population_ManagerH
212 SubPopulation_Population_Manager(
Landscape* L,
string DevReproFile =
"",
int a_sub_w=10,
int a_sub_h=10,
int a_num_life_stage=5,
int a_max_long_dist = 1000,
int a_peak_long_dist = 100,
float a_scale_wind_speed = 1.1,
float a_max_wind_speed = 16,
int a_wind_direc_num = 8,
int a_wind_speed_step_size = 2,
int a_max_alive_day = 300);
265 virtual int calNextStage(
int current_stage,
double density = 1);
267 virtual int calOffspringStage(
int current_stage,
double *offspring_num=NULL,
double a_age=1,
double a_density = -1,
double a_growth_stage=0,
double* a_propotion=NULL,
bool winter_host_flag=
false);
280 void doFlying(
int ind,
int index_x,
int index_y);
302 double calLandingCurve(
double a_dis,
double a_peak,
double a_furthest);
309 virtual void SupplyLocXY(
unsigned index_x,
unsigned index_y,
int & x,
int & y)
344 virtual void readHosts(
string a_file_name);
355 double m_num_killed_by_parasitoid;
356 double m_num_parasitoid;
357 double m_num_parasitoid_egg;
358 double m_num_new_parasitoid_egg_daily;
362 virtual void Run(
int NoTSteps);
Base class for all population managers.
Definition: PopulationManager.h:554
std::vector< int > supplyWinterTolePeriod(TTypesOfLandscapeElement a_tole)
The function to supply the available period vector for the given winter tole host.
Definition: SubPopulation_Population_Manager.h:331
double getSubpopulationInCellLifeStage(int x_indx, int y_indx, int a_life_stage)
Supply the subpopulation size at the given life stage in the given cell.
Definition: SubPopulation_Population_Manager.cpp:331
int m_long_move_mask_x_num_half
Variable to record the dimension of the short distance movement mask. - x.
Definition: SubPopulation_Population_Manager.h:143
CfgFloat cfg_Subpopu_Density_Threshold("SUBPOPU_DENSITY_THRESHOLD", CFG_CUSTOM, 1000)
The class to handle all subpopulation-based ainimal population related matters in the whole landscape...
Definition: SubPopulation_Population_Manager.h:77
double supplyAllPopulationGivenStage(int index)
Supply the population size at the given life stage for the who landscape.
Definition: SubPopulation_Population_Manager.cpp:342
#define M_PI
Definition: sunset.h:33
double m_max_short_distance
The longest distance that the specieces can move for short distance dispersal.
Definition: SubPopulation_Population_Manager.h:137
blitz::Array< SubPopulation *, 2 > m_the_subpopulation_array
Vector to store the all the pointers for the subpopulation object.
Definition: SubPopulation_Population_Manager.h:115
unsigned int SupplyNumberOfPolygons(void)
Returns the number of polygons in the landscape.
Definition: Landscape.h:2127
Definition: MapErrorMsg.h:37
blitz::Array< double, 1 > m_total_num_each_stage
Array to hold the total number of alive animals under different life stages in the whole landscape.
Definition: SubPopulation_Population_Manager.h:81
double SupplyGreenBiomass(int a_polyref)
Returns the green biomass of the vegetation using the polygon reference number a_polyref.
Definition: Landscape.h:1612
int m_max_alive_days
The longest alive day among all the life stages.
Definition: SubPopulation_Population_Manager.h:156
bool supplyWinterHostOn(void)
Definition: SubPopulation_Population_Manager.h:350
void updateWholePopulationArray(int a_listindex, double number)
The function to update the whole population array in the whole landscape.
Definition: SubPopulation_Population_Manager.cpp:227
float m_max_flying_wind_speed
The larget wind speed for winged adults to fly.
Definition: SubPopulation_Population_Manager.h:127
double SupplyTemp(void)
Passes a request on to the associated Weather class function, the temperature for the current day.
Definition: Landscape.h:1993
bool supplySummerHostOn(void)
Definition: SubPopulation_Population_Manager.h:349
TTypesOfVegetation SupplyVegType(int a_x, int a_y)
Returns the vegetation type of the polygon using the polygon reference number a_polyref or coordinate...
Definition: Landscape.h:1925
int supplyNextLifeStage(int life_circle_index, int life_stage_index)
Supply the next life stage index for a given life stage and a given life path.
Definition: SubPopulation_Population_Manager.h:246
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
Landscape * g_landscape_p
blitz::Array< double, 2 > m_wind_direction_array
The array to hold the wind directions.
Definition: SubPopulation_Population_Manager.h:188
virtual void SetNoProbesAndSpeciesSpecificFunctions(int a_pn)
Sets up probe and species specifics.
Definition: SubPopulation_Population_Manager.cpp:1000
virtual unsigned supplyDevelopmentSeason()
The function to supply the development season.
Definition: SubPopulation_Population_Manager.h:292
int x
x-coord
Definition: SubPopulation_Population_Manager.h:50
std::vector< int > m_flying_life_stage_array
The vector to store the life stages that can fly.
Definition: SubPopulation_Population_Manager.h:194
void addNewDay()
Add new day to the newest and oldest array.
Definition: SubPopulation_Population_Manager.cpp:628
char * value() const
Definition: Configurator.h:182
double SupplyWind(void)
Passes a request on to the associated Weather class function, the wind speed for the current day.
Definition: Landscape.h:2061
virtual void writeCalibrationFiles(void)
Write probe files for calibration.
Definition: SubPopulation_Population_Manager.h:347
int SupplyWindDirection(void)
Passes a request on to the associated Weather class function, the wind direction in 4 directions for ...
Definition: Landscape.h:2067
double supplyMortality(int a_life_stage, int a_age)
The function to supply the mortality rate for the given life stage and the age.
Definition: SubPopulation_Population_Manager.h:296
blitz::Array< int, 1 > m_long_move_mask_x_num_half_array
Array to store the number of cells for longest flying at different windspeed.
Definition: SubPopulation_Population_Manager.h:146
double * starting_popu_density
Starting weighted population density for the subpopulation.
Definition: SubPopulation_Population_Manager.h:68
std::vector< int > supplyVecLocMoveLifeStages()
Supply the vector of life stages that can do local movement.
Definition: SubPopulation_Population_Manager.h:321
blitz::Array< double, 1 > m_current_landing_array
Temporal array for the landing subpopulation.
Definition: SubPopulation_Population_Manager.h:168
String configurator entry class.
Definition: Configurator.h:173
double calLandingCurve(double a_dis, double a_peak, double a_furthest)
The function to calculate the landing curve along the wind direction.
Definition: SubPopulation_Population_Manager.cpp:896
const char * m_ListNames[32]
A list of life-stage names.
Definition: PopulationManager.h:628
blitz::Array< int, 1 > m_wind_speed_lookup_table
The look up table for wind speed index in the flying mask.
Definition: SubPopulation_Population_Manager.h:135
blitz::Array< double, 2 > m_cell_suitability
Array for suitable value in each subpopulation cell.
Definition: SubPopulation_Population_Manager.h:113
int m_num_life_stage
The number of life stages for the animal.
Definition: SubPopulation_Population_Manager.h:103
bool empty_flag
Indicator show whether it is an empty subpopulation.
Definition: SubPopulation_Population_Manager.h:64
void readDevReproFile(string inputfile)
Function to read the development time and lifestage.
Definition: SubPopulation_Population_Manager.cpp:414
blitz::Array< double, 1 > m_lowest_temperature_die
The lowest temperature that will cause the species die.
Definition: SubPopulation_Population_Manager.h:88
double getTotalSubpopulationInCell(int x_indx, int y_indx)
Supply the total subpopulation size in the given cell.
Definition: SubPopulation_Population_Manager.cpp:324
bool m_mul_hosts_flag
Flag to show whether it has both winter and summer host.
Definition: SubPopulation_Population_Manager.h:105
SubPopulation_Population_Manager * NPM
SubPopulation_Population_Manager pointer.
Definition: SubPopulation_Population_Manager.h:62
int m_long_move_mask_x_num
Variable to record the dimension of the long distance movement mask. - x.
Definition: SubPopulation_Population_Manager.h:139
double value() const
Definition: Configurator.h:142
Used for creation of a new struct_SubPopulation object.
Definition: SubPopulation_Population_Manager.h:46
bool m_summer_host_on
The flag to enable summer host.
Definition: SubPopulation_Population_Manager.h:204
TTypesOfVegetation TranslateVegCodes(std::string &str)
Converts strings to tov_
Definition: Farm.cpp:2180
int SupplyMonth(void)
Passes a request on to the associated Calendar class function, returns m_month + 1 (the calendar mont...
Definition: Landscape.h:2272
virtual void DoLast()
Definition: SubPopulation_Population_Manager.cpp:478
double getSuitabilityInCell(int x_indx, int y_indx)
Supply the suitability in the given cell.
Definition: SubPopulation_Population_Manager.h:238
blitz::Array< double, 2 > m_cell_popu_density
Array for weighted population density in each subpopulation cell.
Definition: SubPopulation_Population_Manager.h:111
int m_peak_long_distance
The distance for the peak amount of landing.
Definition: SubPopulation_Population_Manager.h:123
virtual void DoFirst()
Definition: SubPopulation_Population_Manager.cpp:691
virtual bool isEnoughNextLifeStage(int a_life_stage)
The funcition to check whether it is read for the next life stage.
Definition: SubPopulation_Population_Manager.cpp:624
int m_wind_direction_num
The number of wind directions.
Definition: SubPopulation_Population_Manager.h:131
double supplyCellHeight()
Supply the height of a subpopulation cell.
Definition: SubPopulation_Population_Manager.h:226
Landscape * L
Landscape pointer.
Definition: SubPopulation_Population_Manager.h:60
int w
area width
Definition: SubPopulation_Population_Manager.h:54
std::map< int, int > m_summer_hosts_tole_period_map
The map to store the index for the summer host period.
Definition: SubPopulation_Population_Manager.h:182
virtual void doSpecicesLastThing()
The special last thing for the drived species.
Definition: SubPopulation_Population_Manager.h:338
int m_ListNameLength
the number of life-stages simulated in the population manager
Definition: PopulationManager.h:626
bool supplyFirstFlagLifeStage(int life_stage)
The function to supply the flag of first for the given life stage.
Definition: SubPopulation_Population_Manager.h:276
SubPopulation * CreateObjects(TAnimal *pvo, struct_SubPopulation *data, int number)
Definition: SubPopulation_Population_Manager.cpp:976
int m_long_move_mask_y_num
Variable to record the dimension of the long distance movement mask. - y.
Definition: SubPopulation_Population_Manager.h:141
Definition: SubPopulation.h:43
void doLocalMovement(int index_x, int index_y, double proportion)
The function for local movement.
Definition: SubPopulation_Population_Manager.cpp:844
int supplyNumFlyingLifeStages()
Supply the number of life stages that can fly.
Definition: SubPopulation_Population_Manager.h:319
virtual void readHosts(string a_file_name)
The fuction to read the host lists for aphid.
Definition: SubPopulation_Population_Manager.cpp:1006
int supplyNumLocMovLifeStages()
Supply the number of life stages thah can do local movement.
Definition: SubPopulation_Population_Manager.h:323
bool isSummerHostTole(TTypesOfLandscapeElement a_ele)
Test whether it is a summer host tole.
Definition: SubPopulation_Population_Manager.cpp:810
blitz::Array< int, 2 > m_index_new_old
Array for index of the newest and oldest life stages.
Definition: SubPopulation_Population_Manager.h:154
SubPopulation * supplySubPopulationPointer(int indx, int indy)
Supply the pointer of the subpopulation object in the given cell.
Definition: SubPopulation_Population_Manager.cpp:338
bool value() const
Definition: Configurator.h:164
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
virtual void calLongMovementMask(void)
Function to calculate the movement mask.
Definition: SubPopulation_Population_Manager.cpp:488
double supplySizeSubpopulationCell()
Supply the size of aphid subpopulation cell.
Definition: SubPopulation_Population_Manager.h:228
int m_max_long_distance
The longest distance that the specieces can fly for long distance dispersal.
Definition: SubPopulation_Population_Manager.h:121
std::vector< std::vector< int > > m_winter_hosts_tole_period
The vector to store the exsiting period for winter host tole.
Definition: SubPopulation_Population_Manager.h:172
void doFlying(int ind, int index_x, int index_y)
The function to fly the winged adults.
Definition: SubPopulation_Population_Manager.cpp:708
std::vector< TTypesOfLandscapeElement > m_winter_hosts_tole
The vector to hold winter host landscape type.
Definition: SubPopulation_Population_Manager.h:170
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:358
bool isWinterHostTole(TTypesOfLandscapeElement a_ele)
Test whether it is a winter host tole.
Definition: SubPopulation_Population_Manager.cpp:800
int SimH
stores the simulation height
Definition: PopulationManager.h:614
int m_wind_speed_step_size
The sampling step size for wind speed.
Definition: SubPopulation_Population_Manager.h:129
bool isWinterHostTov(TTypesOfVegetation a_ele)
Test whether it is a winter host tov.
Definition: SubPopulation_Population_Manager.cpp:820
virtual void SupplyLocXY(unsigned index_x, unsigned index_y, int &x, int &y)
A stub for identifying an individual at a location.
Definition: SubPopulation_Population_Manager.h:309
void SetSpeciesFunctions(TTypesOfPopulation a_species)
This is the jumping off point for any landscape related species setup, it creates function pointers t...
Definition: Landscape.cpp:7821
friend class Subpopulation
Definition: SubPopulation_Population_Manager.h:93
virtual int calNextStage(int current_stage, double density=1)
Return the next life stage for the given life stage.
Definition: SubPopulation_Population_Manager.cpp:659
std::vector< TTypesOfVegetation > m_winter_hosts_tov
The vector to hold winter host vegetation type.
Definition: SubPopulation_Population_Manager.h:176
Bool configurator entry class.
Definition: Configurator.h:155
virtual void updateDevelopmentSeason()
The function to update the development season.
Definition: SubPopulation_Population_Manager.h:269
int species
species ID
Definition: SubPopulation_Population_Manager.h:58
std::vector< int > supplySummerTolePeriod(TTypesOfLandscapeElement a_tole)
The function to supply the available period vector for the given summer tole host.
Definition: SubPopulation_Population_Manager.h:333
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
int index_y
Definition: SubPopulation_Population_Manager.h:70
int m_sub_h
Variable to record the height of the subpopulation cell.
Definition: SubPopulation_Population_Manager.h:109
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
double SupplyVegBiomass(int a_polyref)
Returns the biomass of the vegetation using the polygon reference number a_polyref or based on the x,...
Definition: Landscape.h:1542
blitz::Array< double, 1 > supplyMortalityStageArray(int a_life_stage)
The function to supply the 1D base morality rate array for the given life stage.
Definition: SubPopulation_Population_Manager.h:300
blitz::Array< int, 1 > m_long_move_mask_y_num_half_array
Definition: SubPopulation_Population_Manager.h:147
Definition: LandscapeFarmingEnums.h:67
std::vector< TTypesOfVegetation > m_summer_hosts_tov
The vector to hold sumber host vegetation type.
Definition: SubPopulation_Population_Manager.h:184
int supplyAgeInDay(int lifestage_index, int column_index)
The function to return the age in days given an element in the subpopulation table.
Definition: SubPopulation_Population_Manager.cpp:990
std::vector< int > m_local_moving_life_stage_array
The vector to store the life stags that can move locally.
Definition: SubPopulation_Population_Manager.h:198
int supplyLifeStageNum()
Supply the number of life stage.
Definition: SubPopulation_Population_Manager.h:242
TTypesOfPopulation
An enum to hold all the possible types of population handled by a Population_Manager class.
Definition: PopulationManager.h:57
std::vector< TTypesOfLandscapeElement > m_summer_hosts_tole
The vector to hold summer host landscape type.
Definition: SubPopulation_Population_Manager.h:178
std::vector< std::vector< SubPopulation * > > m_vec_subpopulation_pointers_polygon
The vector used to store the pointers of all the subpopulation objects in each polygon.
Definition: SubPopulation_Population_Manager.h:202
std::vector< std::vector< int > > m_summer_hosts_tole_period
The vector to store the exsiting period for summer host tole.
Definition: SubPopulation_Population_Manager.h:180
std::vector< std::vector< int > > m_index_next_life_stage
The array to store the index for the ones go to the next life stage or die. -1 means nothing.
Definition: SubPopulation_Population_Manager.h:86
virtual ~SubPopulation_Population_Manager(void)
SubPopulation_Manager Destructor.
Definition: SubPopulation_Population_Manager.cpp:212
virtual unsigned GetPopulationSize(int a_listindex)
Must be re-implemented in descendent classes.
Definition: SubPopulation_Population_Manager.h:324
blitz::Array< double, 2 > m_life_circle_path
The life circle array. It could have more than one life circle paths.
Definition: SubPopulation_Population_Manager.h:160
int SimW
stores the simulation width
Definition: PopulationManager.h:616
blitz::Array< double, 4 > m_long_move_mask
Long distance movement mask.
Definition: SubPopulation_Population_Manager.h:119
int m_short_move_mask_y_num
Variable to record the dimension of the short distance movement mask. - y.
Definition: SubPopulation_Population_Manager.h:150
int supplyMaxColNum()
Supply the maximum number of column in the development array.
Definition: SubPopulation_Population_Manager.h:244
blitz::Array< double, 1 > m_highest_temperature_die
The highest temperature that will cause the species die.
Definition: SubPopulation_Population_Manager.h:90
virtual unsigned GetLiveArraySize(int a_listindex)
Must be re-implemented in descendent classes. Gets the number of 'live' objects.
Definition: SubPopulation_Population_Manager.cpp:410
blitz::Array< double, 1 > m_lowest_temp_dev
Lowest development temperatures for each life stage. This is the last step in each day.
Definition: SubPopulation_Population_Manager.h:158
std::vector< int > m_landing_life_stage_array
The vector to store the life stage when a flying one lands, it could be different from the flying one...
Definition: SubPopulation_Population_Manager.h:196
int h
area height
Definition: SubPopulation_Population_Manager.h:56
void updateWholePopulation()
Update whole population info.
Definition: SubPopulation_Population_Manager.cpp:240
virtual void subpopuBaseOutputProbe()
Below are the functions for saving result.
Definition: SubPopulation_Population_Manager.cpp:349
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
std::vector< int > supplyOldEnoughIndex(int life_stage_index)
Definition: SubPopulation_Population_Manager.h:247
double m_size_cell
The size of each subpopulation cell.
Definition: SubPopulation_Population_Manager.h:83
blitz::Array< double, 2 > m_development_degree_day
The array to store the average max and std development time for each life stage, max and std time for...
Definition: SubPopulation_Population_Manager.h:97
blitz::Array< double, 2 > supplyMortalityWholeArray(void)
The function to supply the whole base mortality rate array.
Definition: SubPopulation_Population_Manager.h:298
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
blitz::Array< double, 2 > m_current_mortality_array
This a lookup table for the age and temperature dependent mortality rate. This is the same for the wh...
Definition: SubPopulation_Population_Manager.h:186
SubPopulation_Population_Manager(Landscape *L, string DevReproFile="", int a_sub_w=10, int a_sub_h=10, int a_num_life_stage=5, int a_max_long_dist=1000, int a_peak_long_dist=100, float a_scale_wind_speed=1.1, float a_max_wind_speed=16, int a_wind_direc_num=8, int a_wind_speed_step_size=2, int a_max_alive_day=300)
Definition: SubPopulation_Population_Manager.cpp:61
FarmManager * g_farmmanager
Definition: Farm.cpp:638
void doDevelopment()
Function to make the development for all the subpopulation object.
Definition: SubPopulation_Population_Manager.cpp:566
bool openSubpopulationBaseProbeFile()
Open the storing file.
Definition: SubPopulation_Population_Manager.cpp:394
int index_x
Definition: SubPopulation_Population_Manager.h:69
Double configurator entry class.
Definition: Configurator.h:126
string m_SimulationName
stores the simulation name
Definition: PopulationManager.h:622
virtual void initialisePopulation()
The function to initialise the population when starting the simulation which requires rewritten in th...
Definition: SubPopulation_Population_Manager.cpp:906
int m_num_x_range
The number of subpopulation in x range.
Definition: SubPopulation_Population_Manager.h:99
float m_scale_wind_speed
The scale for wind.
Definition: SubPopulation_Population_Manager.h:125
std::ofstream m_subpopulation_base_prb_file
The file for the storing the data.
Definition: SubPopulation_Population_Manager.h:200
double supplyCellWidth()
Supply the width of a subpopulation cell.
Definition: SubPopulation_Population_Manager.h:224
blitz::Array< bool, 1 > m_first_flag_life_stage
Array to track whether it is the first existence for a life stage.
Definition: SubPopulation_Population_Manager.h:164
int supplyCellNumY()
Supply number of subpopulation in y coordinate.
Definition: SubPopulation_Population_Manager.h:222
void relocatePopulation(void)
Relocate the population in each cell based on the suitability and movement ability.
Definition: SubPopulation_Population_Manager.cpp:452
blitz::Array< blitz::Array< double, 2 >, 2 > m_landing_masks
The array to store the landing masks. The vector is indexed by the wind speed index first and the sec...
Definition: SubPopulation_Population_Manager.h:191
Definition: Configurator.h:70
bool m_hibernated_hatch_flag
Flag variable to indicate hibernated eggs are ready to hatch.
Definition: SubPopulation_Population_Manager.h:162
virtual void updateMortalityArray(void)
This function is used to update the daily mortality rate for all the ages. In this base class,...
Definition: SubPopulation_Population_Manager.cpp:879
double supplyAgeInDayDegree(int lifestage_index, int column_index)
Definition: SubPopulation_Population_Manager.h:308
virtual void Run(int NoTSteps)
Definition: SubPopulation_Population_Manager.cpp:256
void setFirstFlagLifeStage(int life_stage, bool pvalue)
The function to set the flag indicating whether a given lifestage is the first time of existing.
Definition: SubPopulation_Population_Manager.h:274
The class for base animal using subpopulation method.
Definition: SubPopulation.h:56
blitz::Array< double, 1 > m_current_flying_array
Temporal array for the flying subpopulation.
Definition: SubPopulation_Population_Manager.h:166
virtual void doParasitoidDevelopment()
The function for parasitoid calculation, it does nothing in this base class.
Definition: SubPopulation_Population_Manager.h:336
std::map< int, int > m_winter_hosts_tole_period_map
The map to store the index for the winter host period.
Definition: SubPopulation_Population_Manager.h:174
double supplyTotalPopulationInCell(int x_indx, int y_indx)
Supply whole population size at the given cell.
int m_wind_speed_num
The number of wind speed samples.
Definition: SubPopulation_Population_Manager.h:133
int m_num_y_range
The number of subpopulation in y range.
Definition: SubPopulation_Population_Manager.h:101
blitz::Array< double, 4 > m_short_move_mask
Short distance movement mask.
Definition: SubPopulation_Population_Manager.h:117
int SupplyPolyRefIndex(int a_x, int a_y)
Get the index to the m_elems array for a polygon at location x,y.
Definition: Landscape.h:2172
int m_long_move_mask_y_num_half
Definition: SubPopulation_Population_Manager.h:144
The Farm Manager class.
Definition: Farm.h:1461
int supplyCellNumX()
Supply number of subpopulation in x coordinate.
Definition: SubPopulation_Population_Manager.h:220
Definition: SubPopulation.h:48
virtual int calOffspringStage(int current_stage, double *offspring_num=NULL, double a_age=1, double a_density=-1, double a_growth_stage=0, double *a_propotion=NULL, bool winter_host_flag=false)
Calculate the offspring life stage.
Definition: SubPopulation_Population_Manager.cpp:1117
double * starting_suitability
Starting suitability for the subpopulation.
Definition: SubPopulation_Population_Manager.h:66
int SupplyDaylength(void)
Passes a request on to the associated Weather class function, the day length for the current day.
Definition: Landscape.h:2201
blitz::Array< double, 2 > m_accumu_degree_days
Array for accumulated degree days for each life circle.
Definition: SubPopulation_Population_Manager.h:152
int y
y-coord
Definition: SubPopulation_Population_Manager.h:52
void setOldIndex(int life_stage, int p_value)
The function to set the oldest index for the given life stage.
Definition: SubPopulation_Population_Manager.cpp:701
blitz::Array< double, 1 > m_optimal_temperature
The optimal development temperature for the species. (Mortality rate = 0).
Definition: SubPopulation_Population_Manager.h:92
int m_short_move_mask_x_num
Definition: SubPopulation_Population_Manager.h:148
TTypesOfPopulation g_Species
Definition: PopulationManager.cpp:101
CfgBool cfg_Subpopu_Base_Output_Used("SUBPOPU_BASE_OUTPUT_USED", CFG_CUSTOM, true)
CfgStr cfg_Subpopu_Base_Output_Filename("SUBPOPU_BASE_OUTPUT_FILENAME", CFG_CUSTOM, "SubpopuBaseOutput.txt")
bool isSummerHostTov(TTypesOfVegetation a_ele)
Test whether it is a summer host tov.
Definition: SubPopulation_Population_Manager.cpp:830
int m_sub_w
Variable to record the width of the subpopulation cell.
Definition: SubPopulation_Population_Manager.h:107
int supplyNewestIndex(int life_stage_index)
Definition: SubPopulation_Population_Manager.h:248
std::vector< int > supplyVecFlyingLifeStages()
Supply the vector of life stages for flying.
Definition: SubPopulation_Population_Manager.h:317
bool m_winter_host_on
The flag to enalble winter host.
Definition: SubPopulation_Population_Manager.h:206