Loading [MathJax]/extensions/ams.js
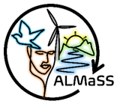 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
28 #ifndef SpringBarleyStriglingSingle_H
29 #define SpringBarleyStriglingSingle_H
31 #define SBSTS_BASE 7100
32 #define SBSTS_SLURRY_DONE m_field->m_user[0]
33 #define SBSTS_MANURE_DONE m_field->m_user[1]
34 #define SBSTS_SLURRY_EXEC m_field->m_user[2]
35 #define SBSTS_MANURE_EXEC m_field->m_user[3]
36 #define SBSTS_DID_AUTUMN_PLOUGH m_field->m_user[4]
38 #define SBSTS_HERBI_DATE m_field->m_user[0]
39 #define SBSTS_GR_DATE m_field->m_user[1]
40 #define SBSTS_FUNGI_DATE m_field->m_user[2]
41 #define SBSTS_WATER_DATE m_field->m_user[3]
42 #define SBSTS_INSECT_DATE m_field->m_user[4]
132 #endif // SpringBarleyStriglingSingle_H
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: SpringBarleyStriglingSingle.h:49
virtual bool SpringRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the spring on a_field.
Definition: FarmFuncs.cpp:487
Definition: SpringBarleyStriglingSingle.h:64
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
void SetUpFarmCategoryInformation()
Definition: SpringBarleyStriglingSingle.h:86
Definition: LandscapeFarmingEnums.h:1005
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Strigling(LE *a_field, double a_user, int a_days)
Carry out a mechanical weeding on a_field.
Definition: FarmFuncs.cpp:1206
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
#define SBSTS_WATER_DATE
Definition: SpringBarleyStriglingSingle.h:41
CfgInt cfg_SingleStriglingTime("STR_SINGLESTRIGLINGTIME", CFG_CUSTOM, 1)
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
#define SBSTS_SLURRY_DONE
Definition: SpringBarleyStriglingSingle.h:32
#define SBSTS_GR_DATE
Definition: SpringBarleyStriglingSingle.h:39
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: SpringBarleyStriglingSingle.h:48
bool m_first_year
Definition: Farm.h:386
Definition: SpringBarleyStriglingSingle.h:77
Definition: SpringBarleyStriglingSingle.h:62
CfgFloat cfg_strigling_prop
Definition: SpringBarleyStriglingSingle.h:70
class Calendar * g_date
Definition: Calendar.cpp:37
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
#define SBSTS_MANURE_EXEC
Definition: SpringBarleyStriglingSingle.h:35
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
double value() const
Definition: Configurator.h:142
Definition: SpringBarleyStriglingSingle.h:53
int m_base_elements_no
Definition: Farm.h:505
Definition: SpringBarleyStriglingSingle.h:45
SBSTSToDo
Definition: SpringBarleyStriglingSingle.h:44
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: SpringBarleyStriglingSingle.h:52
Definition: SpringBarleyStriglingSingle.h:65
The base class for all crops.
Definition: Farm.h:495
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
CfgFloat cfg_ins_app_prop1
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
CfgFloat cfg_greg_app_prop
Definition: SpringBarleyStriglingSingle.h:68
Definition: SpringBarleyStriglingSingle.h:60
Definition: SpringBarleyStriglingSingle.h:61
#define SBSTS_DID_AUTUMN_PLOUGH
Definition: SpringBarleyStriglingSingle.h:36
Definition: SpringBarleyStriglingSingle.h:57
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: SpringBarleyStriglingSingle.h:54
#define SBSTS_SLURRY_EXEC
Definition: SpringBarleyStriglingSingle.h:34
#define SBSTS_MANURE_DONE
Definition: SpringBarleyStriglingSingle.h:33
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
Definition: SpringBarleyStriglingSingle.h:66
Definition: LandscapeFarmingEnums.h:1011
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: SpringBarleyStriglingSingle.h:67
Farm * m_farm
Definition: Farm.h:498
#define SBSTS_HERBI_DATE
Definition: SpringBarleyStriglingSingle.h:38
LE * m_field
Definition: Farm.h:499
int value() const
Definition: Configurator.h:116
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
Definition: SpringBarleyStriglingSingle.h:51
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: SpringBarleyStriglingSingle.h:63
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
virtual bool FP_LiquidNH3(LE *a_field, double a_user, int a_days)
Apply liquid ammonia fertilizer to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:808
The base class for all farm types.
Definition: Farm.h:755
Definition: SpringBarleyStriglingSingle.h:50
Definition: SpringBarleyStriglingSingle.h:55
Double configurator entry class.
Definition: Configurator.h:126
Definition: SpringBarleyStriglingSingle.h:59
Definition: LandscapeFarmingEnums.h:1012
virtual bool GrowthRegulator(LE *a_field, double a_user, int a_days)
Apply growth regulator to a_field.
Definition: FarmFuncs.cpp:2070
Definition: Configurator.h:70
int DayInYear(void)
Definition: Calendar.h:58
Definition: SpringBarleyStriglingSingle.h:58
#define SBSTS_FUNGI_DATE
Definition: SpringBarleyStriglingSingle.h:40
CfgFloat cfg_herbi_app_prop
#define SBSTS_INSECT_DATE
Definition: SpringBarleyStriglingSingle.h:42
#define SBSTS_BASE
Definition: SpringBarleyStriglingSingle.h:31
Definition: SpringBarleyStriglingSingle.h:71
SpringBarleyStriglingSingle(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: SpringBarleyStriglingSingle.h:81
CfgFloat cfg_fungi_app_prop1
bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
Definition: SpringBarleyStriglingSingle.cpp:43
FarmEvent * m_ev
Definition: Farm.h:500
Definition: SpringBarleyStriglingSingle.h:56
Definition: SpringBarleyStriglingSingle.h:72
Definition: MapErrorMsg.h:34
Definition: SpringBarleyStriglingSingle.h:69
Definition: LandscapeFarmingEnums.h:1007
Definition: SpringBarleyStriglingSingle.h:46
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
Definition: SpringBarleyStriglingSingle.h:47