File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
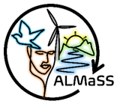 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
259 virtual void Init(
void);
269 bool InSquare(
int p_x,
int p_y,
int p_sqx,
int p_sqy,
int p_range);
276 void RecordDeath(
int a_type) { m_Deaths[a_type]++; }
278 void RecordJDeath() { m_JDeaths++; }
280 void RecordMature() { m_Mature++; }
326 virtual bool EggPosValid(
unsigned a_x,
unsigned a_y);
340 virtual void SetPosMap(
unsigned x,
unsigned y);
384 virtual void Hatch(
int a_eggsackspread,
unsigned a_doubleeggsacspread,
unsigned a_noeggs,
unsigned a_range);
438 virtual int BalloonTo(
int direction,
int distance);
440 virtual int WalkTo(
int direction);
double GetEggDegreesGood()
Returns todays egg sac production day degrees for good food
Definition: Spider_BaseClasses.h:233
bool m_EggProdThresholdPassed
A flag for passing minimum temperature for producing eggs.
Definition: Spider_BaseClasses.h:141
double m_droughtFactor
The running score of drought conditions
Definition: Spider_BaseClasses.h:407
void Init(Spider_Population_Manager *p_spMan)
Used it initialise objects (also used by ReInit)
Definition: Spider_BaseClasses.cpp:141
double GetBTime(int day)
Returns ballooning hours for a given day
Definition: Spider_BaseClasses.h:214
bool HatchDensityMort(int a_x, int a_y, int a_range)
Checks for density-dependent mortality at this location
Definition: Spider_BaseClasses.cpp:107
static int m_DenDependenceConst0
This is the number of local spiders needed before density dependent mortality will kill
Definition: Spider_BaseClasses.h:290
Spider_Population_Manager(Landscape *p_L, int N)
The constructor
Definition: Spider_BaseClasses.cpp:1075
int st_Reproduce()
The behavioural state reproduce
Definition: Spider_BaseClasses.cpp:1004
virtual bool BallooningMortality(int dist)
Determines the ballooning mortality associated with distance dist
Definition: Spider_BaseClasses.cpp:612
SimplePositionMap * m_EggPosMap
Pointer to the egg position map
Definition: Spider_BaseClasses.h:112
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
virtual TTypesOfSpiderState st_AssessHabitat()
The behavioural state assess habitat
Definition: Spider_BaseClasses.h:426
SimplePositionMap * m_OurPosMap
Contains a pointer to the relevant position map - allocation of this must be controlled by the descen...
Definition: Spider_BaseClasses.h:328
The generic base class for spider eggsacs
Definition: Spider_BaseClasses.h:344
MovementMapUnsigned * m_MoveMap
A representation of the landscape in terms of quality - NB MUST be assigned by the descendent populat...
Definition: Spider_BaseClasses.h:110
Definition: Spider_BaseClasses.h:85
static CfgFloat cfg_SpiderEggPPPThreshold("SPID_EGGPPPTHRESHOLD", CFG_CUSTOM, 99999.9, 0.0, 100000)
PPP effects threshold.
int m_DaysSinceRain
The number of days since last rain
Definition: Spider_BaseClasses.h:127
virtual void Hatch(int a_eggsackspread, unsigned a_doubleeggsacspread, unsigned a_noeggs, unsigned a_range)
Determines the number and location of spiderlings to survive hatching and triggers creation of juveni...
Definition: Spider_BaseClasses.cpp:303
static std::array< int, 31 > m_DispDistances
An array to hold the dispersal distances possible as a probability 0-30 (x out of 10000)
Definition: Spider_BaseClasses.h:118
virtual TTypesOfSpiderState st_Balloon()
The behavioural state balloon
Definition: Spider_BaseClasses.cpp:545
Spider_Base(int x, int y, Landscape *L, Spider_Population_Manager *SpMan)
Constructor
Definition: Spider_BaseClasses.cpp:132
double m_pesticide_accum
Body-burden of pesticde
Definition: Spider_BaseClasses.h:296
int m_BallooningStop
A limiter for the day in year to stop ballooning - to be assigned in a descendent classes population ...
Definition: Spider_BaseClasses.h:149
double m_AgeDegrees
A local pointer the population manager
Definition: Spider_BaseClasses.h:300
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
static CfgFloat cfg_SpiderAdultPPPEffectProb("SPID_ADPPPEFFECTPROB", CFG_CUSTOM, 0, 0.0, 1.0)
Effect probability on threshold excedence.
void EndStep()
EndStep behaviour - must be implemented in descendent classes.
Definition: Spider_BaseClasses.cpp:189
bool m_MustBalloon
Flag to force ballooning if possible
Definition: Spider_BaseClasses.h:403
int m_WindDirection
Todays wind direction
Definition: Spider_BaseClasses.h:143
int m_BadHabitatDays
The number of days in bad conditions
Definition: Spider_BaseClasses.h:405
virtual bool OnFarmEvent(FarmToDo event)
Determines the impact of any farm management events at the juvenile's location
Definition: Spider_BaseClasses.h:419
virtual int GetPosMapDensity(unsigned x, unsigned y, unsigned range)
Returns total number of non-zero locations within range of this coordinate (TL corner)
Definition: Spider_BaseClasses.cpp:160
static CfgFloat cfg_SpiderEggPPPEffectProb("SPID_EGGPPPEFFECTPROB", CFG_CUSTOM, 0, 0.0, 1.0)
Effect probability on threshold excedence.
int GetTodaysMonth()
Return current month
Definition: Spider_BaseClasses.h:201
double m_EggProdDDegsInt
Links reproduction to food levels and day degrees - intermediate food
Definition: Spider_BaseClasses.h:165
vector< unsigned > BeforeStepActions
Holds the season list of possible before step actions.
Definition: PopulationManager.h:819
double m_JuvDegreesGood
Contribution to day degrees under good food.
Definition: Spider_BaseClasses.h:157
double m_JuvDegreesIntermediate
Contribution to day degrees under intermediate food
Definition: Spider_BaseClasses.h:159
Definition: Spider_BaseClasses.h:60
virtual void ReInit(int x, int y, Landscape *L, Spider_Population_Manager *EPM, int Eggs)
Used to reinitialise and object
Definition: Spider_BaseClasses.cpp:229
void ReInit(int x, int y, Landscape *L, Spider_Population_Manager *EPM)
Reinitialise reused objects
Definition: Spider_BaseClasses.cpp:881
int DroughtMort[8]
Definition: Spider_BaseClasses.cpp:81
virtual void st_Hatch()
The behavioural state hatch
Definition: Spider_BaseClasses.cpp:281
static double m_JuvDevelConst
Day degrees maturation threshold
Definition: Spider_BaseClasses.h:398
virtual SpiderFoodQuality AssessFood()
Evaluates food status at current location
Definition: Spider_BaseClasses.cpp:599
static double m_EggProducConst
Daydegrees threshold needed to produce an eggsac
Definition: Spider_BaseClasses.h:464
static CfgFloat cfg_LowFoodLevel("SPID_LOWFOODLEVEL", CFG_CUSTOM, 10.58)
virtual void CreateObjects(int ob_type, TAnimal *pvo, struct_Spider *data, int number)
Creates new spider objects - this must be overridden in descendent classes
Definition: Spider_BaseClasses.h:273
char m_MyDirection
The currrent movement direction
Definition: Spider_BaseClasses.h:401
int m_guard_cell_y
The index y to the guard cell.
Definition: PopulationManager.h:374
Spider_Population_Manager * m_OurPopulationManager
Definition: Spider_BaseClasses.h:309
int m_BallooningStart
A limiter for the day in year to start ballooning - to be assigned in a descendent classes population...
Definition: Spider_BaseClasses.h:147
int m_DateLaid
The day in year the eggsac was produced
Definition: Spider_BaseClasses.h:356
const char * m_ListNames[32]
A list of life-stage names.
Definition: PopulationManager.h:628
virtual bool OnFarmEvent(FarmToDo event)
Determines the impact of any farm management events at the female's location
Definition: Spider_BaseClasses.h:488
Definition: Spider_BaseClasses.h:73
static CfgInt cfg_BalDensityDepMortConst("SPID_BALDENSITYDEPMORTCONST", CFG_CUSTOM, 1)
Definition: Spider_BaseClasses.h:84
int GetDoubleEggSacSpread()
Return 2 x EggSacSpread value
Definition: Spider_BaseClasses.h:189
static std::array< int, 40 > m_DispersalChance
Disperals probability as a function of bad habitat days
Definition: Spider_BaseClasses.h:396
double GetTodaysDroughtSc(int index)
Returns the drought score for high, medium or low plant biomass
Definition: Spider_BaseClasses.h:204
Landscape * L
Definition: Spider_BaseClasses.h:99
Used to map locations of individuals for density estimates - space inefficient but good for testing.
Definition: PositionMap.h:45
virtual TTypesOfSpiderState AssessHabitat()
Evaluates the habitat at current location
Definition: Spider_BaseClasses.cpp:666
int GetEggSacSpread()
Return the EggSacSpread value
Definition: Spider_BaseClasses.h:185
Definition: Spider_BaseClasses.h:74
virtual void ReInit(int x, int y, Landscape *L, Spider_Population_Manager *SpPM)
Used to reinitialise reused objects
Definition: Spider_BaseClasses.cpp:363
SimplePositionMap * m_AdultPosMap
Pointer to the egg position map
Definition: Spider_BaseClasses.h:116
static int m_DailyFemaleMort
Daily mortality probability
Definition: Spider_BaseClasses.h:468
double value() const
Definition: Configurator.h:142
int noEggs
Definition: Spider_BaseClasses.h:101
FarmToDo
Definition: Treatment.h:31
int m_EggSacSpread
Used to spread spiderlings on day 1 of hatch - to be assigned in a descendent classes population mana...
Definition: Spider_BaseClasses.h:135
int GetNoEggs()
Returns the number of eggs in the eggsac
Definition: Spider_BaseClasses.h:373
int GetDispDist(int chance)
Returns the dispsersal distance associated with a particular frequency value
Definition: Spider_BaseClasses.h:210
static CfgFloat cfg_SpiderAdultPPPElimiationRate("SPID_ADPPPELIMIATIONRATE", CFG_CUSTOM, 0.0, 0.0, 1.0)
the daily adult elimination rate for pesticides
virtual void ClearMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:60
virtual void SetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:55
bool InSquare(int p_x, int p_y, int p_sqx, int p_sqy, int p_range)
Returns true if p_x,p_y is inside the square defined by p_sqz,p_sqy as TL corner and p_range size len...
Definition: Spider_BaseClasses.cpp:1168
TTypesOfSpiderState
The enumeration lists all spider behavioural states used by all spider species.
Definition: Spider_BaseClasses.h:68
static CfgInt cfg_WalDensityDepMortConst("SPID_WALDENSITYDEPMORTCONST", CFG_CUSTOM, 1)
bool m_WalkingOnly
A flag to denote the spider does not balloon
Definition: Spider_BaseClasses.h:169
int m_DoubleEggSacSpread
Twice the m_EggSacSpread, used to save multiplications
Definition: Spider_BaseClasses.h:137
int m_ListNameLength
the number of life-stages simulated in the population manager
Definition: PopulationManager.h:626
virtual int CheckPosMap(unsigned x, unsigned y)
Returns the value in m_OurPosMap for this location
Definition: Spider_BaseClasses.cpp:150
static CfgFloat cfg_SpiderAdultPPPThreshold("SPID_ADPPPTHRESHOLD", CFG_CUSTOM, 99999.9, 0.0, 100000)
PPP effects threshold.
virtual bool OnFarmEvent(FarmToDo event)
Determines the impact of any farm management events at the eggsac's location
Definition: Spider_BaseClasses.h:375
bool spider_tole_egss_position_valid(Landscape *m_OurLandscape, int x, int y)
Definition: Spider_toletoc.cpp:3
void ZeroEggSacDegrees()
Reset eggsac day degrees counter to zero
Definition: Spider_BaseClasses.h:490
TTypesOfSpiderState m_CurrentSpState
Stores the current behavioural state
Definition: Spider_BaseClasses.h:304
unsigned m_Lifestage
This is a useful parameter holding the spider type.
Definition: Spider_BaseClasses.h:306
double m_EggDegrees[365]
Cumulative day degrees from 1st Jan
Definition: Spider_BaseClasses.h:155
virtual void Step()
The Step code
Definition: Spider_BaseClasses.cpp:949
double GetJuvDegrees_good()
Returns todays juvenile development day degrees for good food
Definition: Spider_BaseClasses.h:236
Definition: Spider_BaseClasses.h:77
unsigned GetMapValue(unsigned a_x, unsigned a_y) const
Definition: MovementMap.h:56
double m_EggProdDDegsPoor
Links reproduction to food levels and day degrees - poor food
Definition: Spider_BaseClasses.h:167
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
void EndStep()
EndStep behaviour - must be implemented in descendent classes.
Definition: Spider_BaseClasses.cpp:175
virtual int BalloonTo(int direction, int distance)
Carry out ballooning in a given direction and distance
Definition: Spider_BaseClasses.cpp:713
virtual void SetPosMap(unsigned x, unsigned y)
Sets a PosMap location to non-zero
Definition: Spider_BaseClasses.cpp:170
Definition: Spider_BaseClasses.h:80
Spider_Population_Manager * SpPM
Definition: Spider_BaseClasses.h:100
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
Definition: Spider_BaseClasses.h:57
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
double GetBallooningMortalityPerMeter()
Return ballooning mortality per meter
Definition: Spider_BaseClasses.h:193
int SimH
stores the simulation height
Definition: PopulationManager.h:614
TTypesOfSpiderState st_Develop()
The behavioural state develop
Definition: Spider_BaseClasses.cpp:289
virtual void DoFirst(void)
DoFirst method, to be overridden in descendent classes
Definition: Spider_BaseClasses.h:261
Definition: Spider_BaseClasses.h:83
virtual bool GetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:50
double m_TodaysDroughtScore[3]
Holds the current drought score for three vegetation classes
Definition: Spider_BaseClasses.h:131
static int m_JuvDensityDepMortConst
Juvenile density dependent mortality threshold
Definition: Spider_BaseClasses.h:394
virtual SpiderFoodQuality CheckToleTovIndex()
Returns the movement map value at current location
Definition: Spider_BaseClasses.cpp:653
double SupplyPesticide(int a_x, int a_y, PlantProtectionProducts a_ppp)
Gets total pesticide for a location.
Definition: Landscape.cpp:1386
Definition: Spider_BaseClasses.h:72
virtual TTypesOfSpiderState st_Walk()
The bheavioural state walk
Definition: Spider_BaseClasses.cpp:569
int m_Age
Stores the spiders age in days
Definition: Spider_BaseClasses.h:302
virtual void Maturation()
The behavioural state maturation
Definition: Spider_BaseClasses.cpp:507
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
SpiderFoodQuality spider_toc_index(Landscape *m_OurLandscape, int x, int y)
Definition: Spider_toletoc.cpp:70
void CreateEggSac(int a_NoEggs)
Creates the egg sac in the system.
Definition: Spider_BaseClasses.cpp:1054
The generic base class for juvenile spiders
Definition: Spider_BaseClasses.h:389
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
void CheckManagement()
Used to start a check for any management related effects at the objects current location.
Definition: PopulationManager.cpp:1591
The generic base class for female spiders
Definition: Spider_BaseClasses.h:459
This is a data class that is used to create new instances of spider objects by CreateObjects in the r...
Definition: Spider_BaseClasses.h:94
Definition: Spider_BaseClasses.h:59
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
Definition: Spider_BaseClasses.h:62
double GetEggDevelDegrees(int day)
Returns todays egg development day degrees
Definition: Spider_BaseClasses.h:245
bool ProduceEggSac()
Produce an eggsac at current location
Definition: Spider_BaseClasses.cpp:1035
bool GetWalking()
Returns walking only flag
Definition: Spider_BaseClasses.h:251
int x
Definition: Spider_BaseClasses.h:97
Definition: Spider_BaseClasses.h:61
int m_NoEggs
The number of eggs contained in the eggsac
Definition: Spider_BaseClasses.h:358
void SetNoEggs(int Eggs)
Sets the number of eggs attribute
Definition: Spider_BaseClasses.h:371
int y
Definition: Spider_BaseClasses.h:98
double GetEggDegreesInt()
Returns todays egg sac production day degrees for intermediate food
Definition: Spider_BaseClasses.h:230
double GetJuvDegrees_intermediate()
Returns todays juvenile development day degrees for intermediate food
Definition: Spider_BaseClasses.h:239
Base class for all population managers for agent based models.
Definition: PopulationManager.h:645
Spider_Egg(int x, int y, Landscape *L, Spider_Population_Manager *EPM, int Eggs)
The Spider_Egg constructor
Definition: Spider_BaseClasses.cpp:222
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
SpiderFoodQuality
The enumeration lists five catagories of habitat for spiders. Chameleon is used when the vegetation t...
Definition: Spider_BaseClasses.h:55
virtual void Step()
The Step code
Definition: Spider_BaseClasses.cpp:407
Definition: Spider_BaseClasses.h:75
bool m_StepDone
Indicates whether the iterative step code is done for this timestep.
Definition: PopulationManager.h:133
bool m_is_paralleled
This is used to indicate whether the species is paralleled.
Definition: PopulationManager.h:796
virtual int WalkTo(int direction)
Walk in a given direction
Definition: Spider_BaseClasses.cpp:785
bool m_MinWalkTemp
A flag to show whether minimum walking temperature is reached
Definition: Spider_BaseClasses.h:171
TTypesOfSpiders
The enumeration lists all spider life stages used by all spider species.
Definition: Spider_BaseClasses.h:45
virtual ~Spider_Population_Manager()
Destructor
Definition: Spider_BaseClasses.cpp:1156
virtual void TheAOROutputProbe()
A method to generate the AOR probe output
Definition: Spider_BaseClasses.h:265
virtual TTypesOfSpiderState st_Walk()
the behavioural state walk
Definition: Spider_BaseClasses.cpp:890
virtual void Step()
The Step code
Definition: Spider_BaseClasses.cpp:243
int value() const
Definition: Configurator.h:116
int GetWindDirection()
Returns the wind direction
Definition: Spider_BaseClasses.h:221
int m_TodaysMonth
Holds the current month
Definition: Spider_BaseClasses.h:129
virtual void AddToBadHabitatDays()
Increments bad habitat days up to max of 39
Definition: Spider_BaseClasses.h:452
double BallooningHrs[52 *7]
The daily number of ballooning hours - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:125
int GetDaysSinceRain()
Return the number of days since rain
Definition: Spider_BaseClasses.h:197
double m_EggDevelopmentThreshold
The lower threshold for egg development - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:151
bool IsBallooningWeather()
Returns the flag for ballooning weather
Definition: Spider_BaseClasses.h:224
static int m_DailyEggMortConst
This is a space for holding cfg species specific parameters - this is the daily egg mortality probabi...
Definition: Spider_BaseClasses.h:351
int SimW
stores the simulation width
Definition: PopulationManager.h:616
virtual void TheRipleysOutputProbe(FILE *a_prb)
A method to generate the Ripley probe output
Definition: Spider_BaseClasses.h:267
static CfgFloat cfg_HighFoodLevel("SPID_HIGHFOODLEVEL", CFG_CUSTOM, 18.515)
void UpdateGuardMap(int a_x, int a_y, int &a_index_x, int &a_index_y)
Get the index of the guard map for the given location.
Definition: PopulationManager.h:768
Definition: Spider_BaseClasses.h:48
const char * StateNames[100]
Definition: PopulationManager.h:801
virtual void Init(void)
An initiation method to initialise all the necessary values - must be overridden for species specific...
Definition: Spider_BaseClasses.cpp:1163
int m_lifespan
Limits the age to which the female spider can reach
Definition: Spider_BaseClasses.h:475
double SupplyInsects(int a_polyref)
Returns the insect biomass on a polygon using the polygon reference number a_polyref or based on the ...
Definition: Landscape.h:1712
Spider_Female(int x, int y, Landscape *L, Spider_Population_Manager *EPM)
The construtor
Definition: Spider_BaseClasses.cpp:873
double GetBTimeToday()
Returns todays ballooning time
Definition: Spider_BaseClasses.h:218
virtual void Catastrophe()
Allows for the possibility to create population level mortality
Definition: Spider_BaseClasses.h:271
virtual int CalculateEggsPerEggSac()
Determines the number of eggs per egg sac - This must be overidden in descendent classes.
Definition: Spider_BaseClasses.h:497
virtual void ReinitialiseObject(int a_x, int a_y, Landscape *a_l_ptr)
Definition: PopulationManager.h:282
virtual int WhatState()
Returns the current spider behavioural state
Definition: Spider_BaseClasses.h:317
Definition: Spider_BaseClasses.h:105
Movementmap.h This file contains the headers for the MovementMap class
virtual int Walk()
Carries out walking
Definition: Spider_BaseClasses.cpp:639
Integer configurator entry class.
Definition: Configurator.h:102
virtual void CalcDrought()
For extension in descendent classes to calculate drought days for mortality
Definition: Spider_BaseClasses.h:454
double m_DailyJuvMort
The daily probability of a juvenile dying - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:121
double GetJuvDegrees_poor()
Returns todays juvenile development day degrees for poor food
Definition: Spider_BaseClasses.h:242
static CfgFloat cfg_NoFoodLevel("SPID_NOFOODLEVEL", CFG_CUSTOM, 1.25)
int m_guard_cell_x
The index x to the guard cell.
Definition: PopulationManager.h:370
virtual void BeginStep()
The begin step code
Definition: Spider_BaseClasses.cpp:238
Definition: Spider_BaseClasses.h:87
static int m_Max_Egg_Production
The maximum number of eggs possible
Definition: Spider_BaseClasses.h:466
bool CheckHumidity(int, int)
Returns humid or not
Definition: Spider_BaseClasses.h:248
Double configurator entry class.
Definition: Configurator.h:126
static int m_SimH
The height of the landscape
Definition: Spider_BaseClasses.h:294
virtual void BeginStep()
The BeingStep code
Definition: Spider_BaseClasses.cpp:926
Definition: Spider_BaseClasses.h:285
virtual int Balloon()
Carries out ballooning
Definition: Spider_BaseClasses.cpp:622
Definition: Configurator.h:70
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
virtual void BeginStep()
The BeginStep code
Definition: Spider_BaseClasses.cpp:374
static double m_EggDevelConst
This is a space for holding cfg species specific parameters - this is a developmental threshold for h...
Definition: Spider_BaseClasses.h:353
static int m_SimW
The width of the landscape
Definition: Spider_BaseClasses.h:292
bool GetMinWalkTemp()
Returns the minimum walking temperature
Definition: Spider_BaseClasses.h:254
virtual TTypesOfSpiderState st_Balloon()
The behavioural state balloon
Definition: Spider_BaseClasses.cpp:907
double GetJuvMort()
Return current juvenile mortality rate
Definition: Spider_BaseClasses.h:207
Definition: Spider_BaseClasses.h:47
double m_BallooningMortalityPerMeter
The mortality of ballooned distance - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:139
int m_CurrentStateNo
The basic state number for all objects - '-1' indicates death.
Definition: PopulationManager.h:131
const double BallooningHours[52]
Definition: Spider_BaseClasses.cpp:73
Definition: Spider_BaseClasses.h:71
Definition: Spider_BaseClasses.h:58
bool spider_tole_lethal(Landscape *m_OurLandscape, int x, int y)
Definition: Spider_toletoc.cpp:59
virtual TTypesOfSpiderState st_Develop()
The behavioural state development
Definition: Spider_BaseClasses.cpp:468
PositionMap.h This file contains the headers for the PositionMap class
SimplePositionMap * m_JuvPosMap
Pointer to the egg position map
Definition: Spider_BaseClasses.h:114
virtual int GetMapDensity(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:65
virtual void st_Die()
The behavioural state die
Definition: Spider_BaseClasses.cpp:275
Definition: Spider_BaseClasses.h:49
bool spider_tole_juvenile_maturation_valid(Landscape *m_OurLandscape, int x, int y)
Definition: Spider_toletoc.cpp:40
virtual void KillThis()
Destroys the spider
Definition: Spider_BaseClasses.h:321
static CfgFloat cfg_SpiderJuvPPPElimiationRate("SPID_JUVPPPELIMIATIONRATE", CFG_CUSTOM, 0.0, 0.0, 1.0)
the daily juvenile elimination rate for pesticides
Definition: Spider_BaseClasses.h:81
static CfgFloat cfg_SpiderJuvPPPEffectProb("SPID_JUVPPPEFFECTPROB", CFG_CUSTOM, 0, 0.0, 1.0)
Effect probability on threshold excedence.
void Warn(std::string a_msg1, std::string a_msg2)
Wrapper for the g_msg Warn function.
Definition: Landscape.h:2250
static CfgFloat cfg_SpiderEggPPPElimiationRate("SPID_EGGPPPELIMIATIONRATE", CFG_CUSTOM, 0.0, 0.0, 1.0)
the daily egg elimination rate for pesticides
Spider_Juvenile(int x, int y, Landscape *L, Spider_Population_Manager *SpPM)
the constructor
Definition: Spider_BaseClasses.cpp:351
void ReInit(int x, int y, Landscape *L, Spider_Population_Manager *SpMan)
Reinitialise object code
Definition: Spider_BaseClasses.cpp:136
double m_EggSacDegrees
Day degrees sum for eggsac production
Definition: Spider_BaseClasses.h:471
virtual bool OnFarmEvent(FarmToDo)
Determines the impact of any farm management events at the spiders location - must be overridden.
Definition: Spider_BaseClasses.h:319
virtual bool GetMapPositive(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:75
virtual bool GetPosMapPositive(unsigned x, unsigned y, unsigned range)
Returns whether there are any non-zero values within range of this coordinate (TL corner)
Definition: Spider_BaseClasses.cpp:155
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
virtual bool EggPosValid(unsigned a_x, unsigned a_y)
Checks if its possible to create an eggsac here
Definition: Spider_BaseClasses.cpp:100
virtual void ClearPosMap(unsigned x, unsigned y)
Clears a PosMap location
Definition: Spider_BaseClasses.cpp:165
static int m_HatDensityDepMortConst
This is a space for holding cfg species specific parameters - this is the mortality constant associat...
Definition: Spider_BaseClasses.h:349
Definition: SubPopulation.h:48
bool m_BallooningWeather
A flag for whether the weather is correct for ballooning
Definition: Spider_BaseClasses.h:145
Definition: Spider_BaseClasses.h:79
virtual void SpecialWinterMort()
Used to impose extra mortaltiy to those spiderlings that don't reach a minimum developmental stage by...
Definition: Spider_BaseClasses.cpp:589
double GetEggDegreesPoor()
Returns todays egg sac production day degrees for poor food
Definition: Spider_BaseClasses.h:227
double m_EggDevelopmentThreshold2
The upper threshold for egg development - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:153
double m_MinWalkTempThreshold
A minimum dispersal by walking temperature
Definition: Spider_BaseClasses.h:173
double m_JuvDegreesPoor
Contribution to day degrees under poor food
Definition: Spider_BaseClasses.h:161
Definition: LandscapeFarmingEnums.h:1079
int m_DispersalDistances[10000]
The distribution of dispersal distances - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:123
int m_EggsProduced
Record of the number of eggs produced
Definition: Spider_BaseClasses.h:473
static CfgInt cfg_ExtraCompetionMortality("SPID_EXTRACOMPETITIONMORTALITY", CFG_CUSTOM, 10)
void EndStep()
EndStep behaviour - must be implemented in descendent classes.
Definition: Spider_BaseClasses.cpp:203
double m_EggProdDDegsGood
Links reproduction to food levels and day degrees - good food.
Definition: Spider_BaseClasses.h:163
static CfgFloat cfg_SpiderJuvPPPThreshold("SPID_JUVPPPTHRESHOLD", CFG_CUSTOM, 99999.9, 0.0, 100000)
PPP effects threshold.
double m_TodaysBallooningTime
The current day's ballooning time
Definition: Spider_BaseClasses.h:133
Movement maps are used for rapid computing of animal movement. This version uses values of 0 to max i...
Definition: MovementMap.h:52