Loading [MathJax]/extensions/ams.js
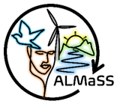 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
42 #ifndef PTSHRUBPASTURES_H
43 #define PTSHRUBPASTURES_H
45 #define PTSHRUBPASTURES_BASE 31000
49 #define PT_SP1_CATTLEOUT_DATE a_field->m_user[1]
50 #define PT_SP2_CATTLEOUT_DATE a_field->m_user[2]
51 #define PT_SP3_CATTLEOUT_DATE a_field->m_user[3]
52 #define PT_SP_YEARS_AFTER_PLOUGH a_field->m_user[4]
122 #endif // PTSHRUBPASTURES_H
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
Definition: PTShrubPastures.h:66
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
int GetYearNumber(void)
Definition: Calendar.h:72
#define PT_SP3_CATTLEOUT_DATE
Definition: PTShrubPastures.h:51
#define PT_SP1_CATTLEOUT_DATE
A flag used to indicate cattle out dates.
Definition: PTShrubPastures.h:49
class Calendar * g_date
Definition: Calendar.cpp:37
PTShrubPasturesToDo
Definition: PTShrubPastures.h:59
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
The base class for all crops.
Definition: Farm.h:495
virtual bool CattleIsOutLow2(LE *a_field, double a_user, int a_days, int a_max, int a_max_days)
Generate a 'cattle_out_low2' event for every day the cattle are on a_field, cattle is low grazing and...
Definition: FarmFuncs.cpp:2607
virtual bool CattleOutLowGrazing(LE *a_field, double a_user, int a_days)
Start a extensive grazing event on a_field today.
Definition: FarmFuncs.cpp:2439
Definition: LandscapeFarmingEnums.h:318
PTShrubPastures class .
Definition: PTShrubPastures.h:83
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: PTShrubPastures.h:60
Definition: PTShrubPastures.h:67
long Date(void)
Definition: Calendar.h:57
Definition: PTShrubPastures.h:61
Definition: PTShrubPastures.h:62
CfgBool g_farm_fixed_crop_enable
Bool configurator entry class.
Definition: Configurator.h:155
Definition: LandscapeFarmingEnums.h:1003
Definition: PTShrubPastures.h:63
Definition: PTShrubPastures.h:64
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
#define PT_SP2_CATTLEOUT_DATE
Definition: PTShrubPastures.h:50
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
Definition: PTShrubPastures.h:65
Definition: PTShrubPastures.h:69
PTShrubPastures(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: PTShrubPastures.h:87
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
#define PT_SP_YEARS_AFTER_PLOUGH
Definition: PTShrubPastures.h:52
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: PTShrubPastures.cpp:55
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
#define PTSHRUBPASTURES_BASE
Definition: PTShrubPastures.h:45
Definition: LandscapeFarmingEnums.h:1010
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
void SetUpFarmCategoryInformation()
Definition: PTShrubPastures.h:95
int DayInYear(void)
Definition: Calendar.h:58
Definition: PTShrubPastures.h:68
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: PTShrubPastures.h:70
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751