Loading [MathJax]/extensions/ams.js
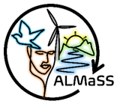 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
45 #define PTRYEGRASS_BASE 32100
147 #endif // PTRYEGRASS_H
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
PTRyegrass class .
Definition: PTRyegrass.h:91
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
Definition: PTRyegrass.h:58
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
Definition: PTRyegrass.h:73
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: PTRyegrass.h:53
int m_base_elements_no
Definition: Farm.h:505
virtual bool CutToHay(LE *a_field, double a_user, int a_days)
Carry out hay cutting on a_field.
Definition: FarmFuncs.cpp:1607
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
PTRyegrassToDo
Definition: PTRyegrass.h:52
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
The base class for all crops.
Definition: Farm.h:495
Definition: PTRyegrass.h:76
Definition: PTRyegrass.h:57
Definition: PTRyegrass.h:67
Definition: PTRyegrass.h:59
PTRyegrass(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: PTRyegrass.h:95
Definition: PTRyegrass.h:63
Definition: LandscapeFarmingEnums.h:1009
Definition: PTRyegrass.h:77
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
#define PTRYEGRASS_BASE
Definition: PTRyegrass.h:45
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Bool configurator entry class.
Definition: Configurator.h:155
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: PTRyegrass.h:71
Definition: PTRyegrass.h:65
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: PTRyegrass.h:56
Definition: PTRyegrass.h:69
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: PTRyegrass.h:61
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: PTRyegrass.h:66
int m_todo
Definition: Farm.h:388
CfgFloat l_pest_insecticipt_amount
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
CfgInt cfg_WW_InsecticideDay
Provided to allow configuration control of the first insecticide spray in winter wheat - this changes...
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: PTRyegrass.h:54
Definition: PTRyegrass.h:64
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: LandscapeFarmingEnums.h:324
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
Definition: PTRyegrass.h:62
Definition: PTRyegrass.h:68
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
Definition: PTRyegrass.h:70
The base class for all farm types.
Definition: Farm.h:755
Double configurator entry class.
Definition: Configurator.h:126
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
Definition: LandscapeFarmingEnums.h:1012
void SetUpFarmCategoryInformation()
Definition: PTRyegrass.h:105
int DayInYear(void)
Definition: Calendar.h:58
Definition: PTRyegrass.h:78
Definition: PTRyegrass.h:55
Definition: PTRyegrass.h:60
CfgBool cfg_pest_springwheat_on
Turn on pesticides for spring wheat.
CfgInt cfg_WW_InsecticideMonth
Provided to allow configuration control of the first insecticide spray in winter wheat - this changes...
virtual bool HayTurning(LE *a_field, double a_user, int a_days)
Carry out hay turning on a_field.
Definition: FarmFuncs.cpp:1491
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: PTRyegrass.h:75
Definition: PTRyegrass.h:72
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: PTRyegrass.cpp:62
Definition: PTRyegrass.h:74
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751