Loading [MathJax]/extensions/ams.js
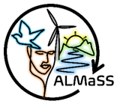 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
45 #define PTCABBAGE_BASE 31200
125 #endif // PTCABBAGE_H
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
Definition: LandscapeFarmingEnums.h:1005
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
#define PTCABBAGE_BASE
Definition: PTCabbage.h:45
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
virtual bool Irrigation(LE *a_field, double a_user, int a_days, int a_max)
Generate an 'irrigation' event with a frequency defined by a_freq in the irrigation period on a_field...
Definition: FarmFuncs.cpp:1856
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: PTCabbage.cpp:55
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
Definition: PTCabbage.h:60
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
Definition: PTCabbage.h:65
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
int m_base_elements_no
Definition: Farm.h:505
Definition: PTCabbage.h:64
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
CfgBool g_farm_fixed_crop_enable
Definition: PTCabbage.h:56
Definition: PTCabbage.h:55
The base class for all crops.
Definition: Farm.h:495
PTCabbage class .
Definition: PTCabbage.h:79
Definition: PTCabbage.h:54
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: LandscapeFarmingEnums.h:330
virtual bool IrrigationStart(LE *a_field, double a_user, int a_days)
Start a irrigation event on a_field today.
Definition: FarmFuncs.cpp:1834
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: PTCabbage.h:59
Definition: PTCabbage.h:66
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Bool configurator entry class.
Definition: Configurator.h:155
Definition: LandscapeFarmingEnums.h:1003
Definition: LandscapeFarmingEnums.h:1011
long OldDays(void)
Definition: Calendar.h:60
Definition: LandscapeFarmingEnums.h:627
Definition: PTCabbage.h:67
Definition: PTCabbage.h:57
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: PTCabbage.h:53
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
PTCabbage(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: PTCabbage.h:83
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
Definition: PTCabbage.h:61
Definition: LandscapeFarmingEnums.h:1012
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
Definition: PTCabbage.h:62
int DayInYear(void)
Definition: Calendar.h:58
void SetCropClassification(int a_classification)
Definition: Farm.h:549
virtual bool WinterHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the winter on a_field.
Definition: FarmFuncs.cpp:1539
Definition: PTCabbage.h:58
Definition: PTCabbage.h:63
PTCabbageToDo
Definition: PTCabbage.h:52
void SetUpFarmCategoryInformation()
Definition: PTCabbage.h:94
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751