Loading [MathJax]/extensions/ams.js
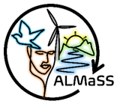 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
24 #define PLCarrots_BASE 25300
28 #define PL_CA_STUBBLE_PLOUGH a_field->m_user[1]
29 #define PL_CA_WEEDING a_field->m_user[2]
30 #define PL_CA_HERBI a_field->m_user[3]
128 #endif // PLCarrots_H
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: PLCarrots.h:52
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
Definition: PLCarrots.h:45
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Strigling(LE *a_field, double a_user, int a_days)
Carry out a mechanical weeding on a_field.
Definition: FarmFuncs.cpp:1206
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
int GetMConstants(int a)
Definition: Elements.h:407
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
CfgFloat l_pest_insecticide_amount
int GetYearNumber(void)
Definition: Calendar.h:72
PLCarrotsToDo
Definition: PLCarrots.h:37
Definition: PLCarrots.h:44
bool m_first_year
Definition: Farm.h:386
virtual bool StubblePlough(LE *a_field, double a_user, int a_days)
Carry out a stubble ploughing event on a_field. This is similar to normal plough but shallow (normall...
Definition: FarmFuncs.cpp:232
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
Definition: PLCarrots.h:47
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: PLCarrots.h:48
Definition: LandscapeFarmingEnums.h:245
virtual bool BedForming(LE *a_field, double a_user, int a_days)
Do bed forming up on a_field, probably of carrots.
Definition: FarmFuncs.cpp:1316
Definition: LandscapeFarmingEnums.h:1006
#define PL_CA_STUBBLE_PLOUGH
A flag used to indicate autumn ploughing status.
Definition: PLCarrots.h:28
Definition: PLCarrots.h:46
#define PLCarrots_BASE
Definition: PLCarrots.h:24
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
Definition: PLCarrots.h:41
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
TTypesOfFarm GetType(void)
Definition: Farm.h:956
int m_base_elements_no
Definition: Farm.h:505
Definition: PLCarrots.h:38
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: PLCarrots.h:64
CfgInt cfg_CA_InsecticideDay
The base class for all crops.
Definition: Farm.h:495
Definition: PLCarrots.h:53
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
Definition: PLCarrots.h:49
Definition: PLCarrots.h:60
bool value() const
Definition: Configurator.h:164
Definition: PLCarrots.h:43
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: PLCarrots.h:56
long Date(void)
Definition: Calendar.h:57
Definition: PLCarrots.h:63
PLCarrots(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: PLCarrots.h:80
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: PLCarrots.h:62
Definition: LandscapeFarmingEnums.h:1008
Definition: PLCarrots.h:58
Bool configurator entry class.
Definition: Configurator.h:155
Definition: PLCarrots.h:50
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
void SetUpFarmCategoryInformation()
Definition: PLCarrots.h:88
Definition: PLCarrots.h:51
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
int GetRotIndex(void)
Definition: Elements.h:373
Definition: PLCarrots.h:54
#define PL_CA_HERBI
Definition: PLCarrots.h:30
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
CfgInt cfg_CA_InsecticideMonth
std::vector< double > value() const
Definition: Configurator.h:219
int m_todo
Definition: Farm.h:388
Definition: PLCarrots.h:57
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: LandscapeFarmingEnums.h:696
Definition: Elements.h:86
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: PLCarrots.cpp:65
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: PLCarrots.h:61
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
The base class for all farm types.
Definition: Farm.h:755
Double configurator entry class.
Definition: Configurator.h:126
Definition: LandscapeFarmingEnums.h:1012
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Definition: PLCarrots.h:42
#define PL_CA_WEEDING
Definition: PLCarrots.h:29
CfgArray_Double cfg_seedcoating_product_amounts
Amount of seed coating pesticide applied in grams of active substance per hectare for each of the 10 ...
Definition: PLCarrots.h:59
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
void SetMConstants(int a, int c)
Definition: Elements.h:408
FarmEvent * m_ev
Definition: Farm.h:500
CfgBool cfg_pest_carrots_on
Turn on pesticides for carrots.
Definition: MapErrorMsg.h:34
CfgBool cfg_seedcoating_carrots_on
Turn on seed coating for carrots.
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
PLCarrots class .
Definition: PLCarrots.h:76
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
Definition: PLCarrots.h:40
Definition: PLCarrots.h:39
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751
Definition: PLCarrots.h:55