File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
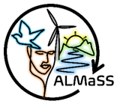 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
26 #ifndef OedothoraxPopulationManagerH
27 #define OedothoraxPopulationManagerH
43 virtual void Init (
void);
54 #ifdef __RECORD_RECOVERY_POLYGONS
56 void RecordRecoveryPolygons();
57 int m_RecoveryPolygons[101];
58 int m_RecoveryPolygonsC[101];
static CfgFloat cfg_Oedothorax_EggProducHA("ERIGONE_EGGPRODUCHA", CFG_CUSTOM, 14000)
bool m_EggProdThresholdPassed
A flag for passing minimum temperature for producing eggs.
Definition: Spider_BaseClasses.h:141
static CfgFloat cfg_Oedothorax_EggProducConst("OEDOTHORAX_EGGPRODUCCONST", CFG_CUSTOM, 1000)
static CfgFloat cfg_Oedothorax_SpiderMinWalkTemp("OEDOTHORAX_MIN_WALK_TEMP", CFG_CUSTOM, 3)
static CfgFloat cfg_Oedothorax_JuvDevelThreshold("OEDOTHORAX_JUVDEVELTHRESHOLD", CFG_CUSTOM, 0.0)
static int m_DenDependenceConst0
This is the number of local spiders needed before density dependent mortality will kill
Definition: Spider_BaseClasses.h:290
int Supply_m_Location_x() const
Returns the ALMaSS x-coordinate.
Definition: PopulationManager.h:239
static CfgFloat cfg_Oedothorax_JuvDevelRHO25("OEDOTHORAX_EGGVDEVELRH", CFG_CUSTOM, 0.094332631)
SimplePositionMap * m_EggPosMap
Pointer to the egg position map
Definition: Spider_BaseClasses.h:112
virtual void KillThis()
Sets all parameters ready for object destruction.
Definition: PopulationManager.h:263
The generic base class for spider eggsacs
Definition: Spider_BaseClasses.h:344
MovementMapUnsigned * m_MoveMap
A representation of the landscape in terms of quality - NB MUST be assigned by the descendent populat...
Definition: Spider_BaseClasses.h:110
virtual TAnimal * SupplyAnimalPtr(unsigned int a_index, unsigned int a_animal)
Returns the pointer indexed by a_index and a_animal. Note NO RANGE CHECK.
Definition: PopulationManager.h:678
double SupplyTemp(void)
Passes a request on to the associated Weather class function, the temperature for the current day.
Definition: Landscape.h:1993
static std::array< int, 31 > m_DispDistances
An array to hold the dispersal distances possible as a probability 0-30 (x out of 10000)
Definition: Spider_BaseClasses.h:118
std::vector< std::vector< omp_nest_lock_t * > > m_MapGuard
This is used to make sure animals will not behaviour at the same area - multi threads.
Definition: PopulationManager.h:790
int SupplyPolygonRef() const
Returns the polygon reference where the object is located.
Definition: PopulationManager.h:229
int m_BallooningStop
A limiter for the day in year to stop ballooning - to be assigned in a descendent classes population ...
Definition: Spider_BaseClasses.h:149
static CfgFloat cfg_Oedothorax_MaxDistBalloonable("OEDOTHORAX_MAXDISTBALLOONABLE", CFG_CUSTOM, 2200)
Definition: Oedothorax_Population_Manager.h:39
CfgBool cfg_ReallyBigOutputMonthly_used
static CfgFloat cfg_Oedothorax_DroughtScaler("OEDOTHORAX_DROUGHTSCALER", CFG_CUSTOM, 0.0000125)
virtual void DoFirst(void)
DoFirst method, to be overridden in descendent classes
Definition: Oedothorax_Population_Manager.cpp:307
static CfgFloat cfg_Oedothorax_JuvDevelHA("OEDOTHORAX_JUVVDEVELHA", CFG_CUSTOM, 23457.2191)
int m_WindDirection
Todays wind direction
Definition: Spider_BaseClasses.h:143
TTypesOfPopulation m_population_type
Definition: PopulationManager.h:858
double SupplyWind(void)
Passes a request on to the associated Weather class function, the wind speed for the current day.
Definition: Landscape.h:2061
double m_EggProdDDegsInt
Links reproduction to food levels and day degrees - intermediate food
Definition: Spider_BaseClasses.h:165
vector< unsigned > BeforeStepActions
Holds the season list of possible before step actions.
Definition: PopulationManager.h:819
double GetRHPeriod(long a_date, unsigned int a_period)
Definition: Weather.cpp:723
double m_JuvDegreesGood
Contribution to day degrees under good food.
Definition: Spider_BaseClasses.h:157
double m_JuvDegreesIntermediate
Contribution to day degrees under intermediate food
Definition: Spider_BaseClasses.h:159
static double m_JuvDevelConst
Day degrees maturation threshold
Definition: Spider_BaseClasses.h:398
static double m_EggProducConst
Daydegrees threshold needed to produce an eggsac
Definition: Spider_BaseClasses.h:464
int m_BallooningStart
A limiter for the day in year to start ballooning - to be assigned in a descendent classes population...
Definition: Spider_BaseClasses.h:147
class Calendar * g_date
Definition: Calendar.cpp:37
const char * m_ListNames[32]
A list of life-stage names.
Definition: PopulationManager.h:628
static std::array< int, 40 > m_DispersalChance
Disperals probability as a function of bad habitat days
Definition: Spider_BaseClasses.h:396
Landscape * L
Definition: Spider_BaseClasses.h:99
virtual void Init(void)
An initiation method to initialise all the necessary values - must be overridden for species specific...
Definition: Oedothorax_Population_Manager.cpp:147
Used to map locations of individuals for density estimates - space inefficient but good for testing.
Definition: PositionMap.h:45
static CfgInt cfg_Oedothorax_HatDensityDepMortConst("OEDOTHORAX_HATDENSITYDEPMORTCONST", CFG_CUSTOM, 3)
static CfgFloat cfg_Oedothorax_EggProducRHO25("ERIGONE_EGGPRODUCRH", CFG_CUSTOM, 0.46)
SimplePositionMap * m_AdultPosMap
Pointer to the egg position map
Definition: Spider_BaseClasses.h:116
static CfgFloat cfg_Oedothorax_JuvDevelIntermediate("OEDOTHORAX_JUVDEVELINTERMEDIATE", CFG_CUSTOM, 0.66666666)
static CfgInt cfg_Oedothorax_BallooningStart("OEDOTHORAX_BALLOONINGSTART", CFG_CUSTOM, 91)
static int m_DailyFemaleMort
Daily mortality probability
Definition: Spider_BaseClasses.h:468
double value() const
Definition: Configurator.h:142
int noEggs
Definition: Spider_BaseClasses.h:101
int SupplyMonth(void)
Passes a request on to the associated Calendar class function, returns m_month + 1 (the calendar mont...
Definition: Landscape.h:2272
int m_EggSacSpread
Used to spread spiderlings on day 1 of hatch - to be assigned in a descendent classes population mana...
Definition: Spider_BaseClasses.h:135
static CfgFloat cfg_Oedothorax_EggProducPoor("OEDOTHORAX_EGGPRODUCPOOR", CFG_CUSTOM, 0.33)
virtual void ClearMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:60
virtual void SetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:55
bool m_WalkingOnly
A flag to denote the spider does not balloon
Definition: Spider_BaseClasses.h:169
int m_DoubleEggSacSpread
Twice the m_EggSacSpread, used to save multiplications
Definition: Spider_BaseClasses.h:137
int m_ListNameLength
the number of life-stages simulated in the population manager
Definition: PopulationManager.h:626
Definition: Oedothorax.h:51
static CfgFloat cfg_Oedothorax_MaxBalloonPercent("OEDOTHORAX_MAXBALLOONPERCENT", CFG_CUSTOM, 5)
static CfgFloat cfg_Oedothorax_EggDevelThreshold2("OEDOTHORAX_EGGDEVELTHRESHOLD2", CFG_CUSTOM, 25.0)
void ZeroEggSacDegrees()
Reset eggsac day degrees counter to zero
Definition: Spider_BaseClasses.h:490
double m_EggDegrees[365]
Cumulative day degrees from 1st Jan
Definition: Spider_BaseClasses.h:155
bool value() const
Definition: Configurator.h:164
double m_EggProdDDegsPoor
Links reproduction to food levels and day degrees - poor food
Definition: Spider_BaseClasses.h:167
static CfgInt cfg_Oedothorax_SmallestBallooningDistance("OEDOTHORAX_SMALLESTBALLOONDIST", CFG_CUSTOM, 70)
The minimum ballooning distnace.
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
const std::array< int, 40 > Oedothorax_DispersalChance
Definition: Oedothorax_Population_Manager.cpp:59
static CfgFloat cfg_Oedothorax_JuvDevelPoor("OEDOTHORAX_JUVDEVELPOOR", CFG_CUSTOM, 0.33333333)
AOR_Probe * m_AOR_Probe
A pointer to the AOR probe.
Definition: PopulationManager.h:875
Spider_Population_Manager * SpPM
Definition: Spider_BaseClasses.h:100
const double MinBallooningTemp
Definition: Oedothorax_Population_Manager.cpp:69
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
static CfgInt cfg_OedothoraxDensityDependentConstantZero("OEDOTHORAX_DENDEPCONSTZERO", CFG_CUSTOM, 0)
Oedothorax_Population_Manager(Landscape *p_L)
Definition: Oedothorax_Population_Manager.cpp:126
virtual bool GetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:50
virtual void DoProbe(int a_lifestage)
Definition: AOR_Probe.cpp:104
double m_TodaysDroughtScore[3]
Holds the current drought score for three vegetation classes
Definition: Spider_BaseClasses.h:131
static int m_JuvDensityDepMortConst
Juvenile density dependent mortality threshold
Definition: Spider_BaseClasses.h:394
static CfgInt cfg_OedothoraxStartNos("OEDOTHORAX_STARTNO", CFG_CUSTOM, 50000)
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
long Date(void)
Definition: Calendar.h:57
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
The generic base class for juvenile spiders
Definition: Spider_BaseClasses.h:389
Bool configurator entry class.
Definition: Configurator.h:155
static CfgInt cfg_Oedothorax_DailyEggMort("OEDOTHORAX_DAILYEGGMORT", CFG_CUSTOM, 40)
The generic base class for female spiders
Definition: Spider_BaseClasses.h:459
int SupplyWindDirection8(void)
Passes a request on to the associated Weather class function, the wind direction in 8 directions for ...
Definition: Landscape.h:2072
This is a data class that is used to create new instances of spider objects by CreateObjects in the r...
Definition: Spider_BaseClasses.h:94
int Supply_m_Location_y() const
Returns the ALMaSS y-coordinate.
Definition: PopulationManager.h:243
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
int x
Definition: Spider_BaseClasses.h:97
Movement maps are used for rapid computing of animal movement. This version uses values of 0-3 only.
Definition: MovementMap.h:88
virtual void TheRipleysOutputProbe(FILE *a_prb)
A method to generate the Ripley probe output
Definition: Oedothorax_Population_Manager.cpp:539
int y
Definition: Spider_BaseClasses.h:98
static CfgInt cfg_Oedothorax_JuvDensityDepMortConst("OEDOTHORAX_JUVDENSITYDEPMORTCONST", CFG_CUSTOM, 4)
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
static CfgInt cfg_Oedothorax_Max_Egg_Production("OEDOTHORAX_MAX_EGG_PRODUCTION", CFG_CUSTOM, 15)
bool m_MinWalkTemp
A flag to show whether minimum walking temperature is reached
Definition: Spider_BaseClasses.h:171
int SupplyYearNumber(void)
Passes a request on to the associated Calendar class function, returns m_simulationyear
Definition: Landscape.h:2287
static CfgFloat cfg_Oedothorax_DroughtRHThreshold("OEDOTHORAX_DROUGHTRHTHRESHOLD", CFG_CUSTOM, 90.0)
int value() const
Definition: Configurator.h:116
int m_TodaysMonth
Holds the current month
Definition: Spider_BaseClasses.h:129
double BallooningHrs[52 *7]
The daily number of ballooning hours - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:125
double m_EggDevelopmentThreshold
The lower threshold for egg development - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:151
static int m_DailyEggMortConst
This is a space for holding cfg species specific parameters - this is the daily egg mortality probabi...
Definition: Spider_BaseClasses.h:351
static CfgFloat cfg_Oedothorax_TempDailyJuvMortParameterB("OEDOTHORAX_DAILYTEMPJUVMORTPARAMB", CFG_CUSTOM, 0.011)
bool OpenTheRipleysOutputProbe()
Definition: PopulationManager.cpp:897
static CfgInt cfg_Oedothorax_BallooningStop("OEDOTHORAX_BALLOONINGSTOP", CFG_CUSTOM, 180)
CfgBool cfg_CfgRipleysOutputUsed
static CfgFloat cfg_Oedothorax_EggDevelConst2("OEDOTHORAX_EGGDEVELCONSTTWO", CFG_CUSTOM, 1000)
class Weather * g_weather
Definition: Weather.cpp:49
bool OpenTheReallyBigProbe()
Definition: PopulationManager.cpp:1005
unsigned GetLiveArraySize(int a_listindex) override
Gets the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:657
static CfgFloat cfg_Oedothorax_EggDevelHA("OEDOTHORAX_EGGVDEVELHA", CFG_CUSTOM, 16894.22)
Definition: Oedothorax.h:41
CfgBool cfg_ReallyBigOutputUsed
Definition: Spider_BaseClasses.h:105
double GetTempPeriod(long a_date, unsigned int a_period)
Definition: Weather.cpp:707
CfgBool cfg_AorOutput_used
Integer configurator entry class.
Definition: Configurator.h:102
virtual void TheAOROutputProbe()
A method to generate the AOR probe output
Definition: Oedothorax_Population_Manager.cpp:533
double m_DailyJuvMort
The daily probability of a juvenile dying - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:121
static int m_Max_Egg_Production
The maximum number of eggs possible
Definition: Spider_BaseClasses.h:466
Double configurator entry class.
Definition: Configurator.h:126
string m_SimulationName
stores the simulation name
Definition: PopulationManager.h:622
static CfgFloat cfg_Oedothorax_EggDevelRHO25("OEDOTHORAX_EGGVDEVELRH", CFG_CUSTOM, 0.1132253)
CfgInt cfg_PmEventfrequency
static CfgFloat cfg_Oedothorax_TempDailyJuvMortParameterA("OEDOTHORAX_DAILYTEMPJUVMORTPARAMA", CFG_CUSTOM, -0.0006)
void CreateObjects(int ob_type, TAnimal *pvo, struct_Spider *data, int number)
Creates new spider objects - this must be overridden in descendent classes
Definition: Oedothorax_Population_Manager.cpp:458
static CfgFloat cfg_Oedothorax_DroughtTThreshold("OEDOTHORAX_DROUGHTTTHRESHOLD", CFG_CUSTOM, 10.0)
static int m_SimH
The height of the landscape
Definition: Spider_BaseClasses.h:294
static CfgInt cfg_Oedothorax_DailyFemaleMort("OEDOTHORAX_DAILYFEMALEMORT", CFG_CUSTOM, 3)
int m_guard_cell_size
Definition: PopulationManager.h:793
static CfgFloat cfg_Oedothorax_EggProducIntemediate("OEDOTHORAX_EGGPRODUCINTERMEDIATE", CFG_CUSTOM, 0.66)
Definition: Configurator.h:70
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
static CfgFloat cfg_Oedothorax_EggProducThreshold("OEDOTHORAX_EGGPRODUCTHRESHOLD", CFG_CUSTOM, 7.0)
static double m_EggDevelConst
This is a space for holding cfg species specific parameters - this is a developmental threshold for h...
Definition: Spider_BaseClasses.h:353
static int m_SimW
The width of the landscape
Definition: Spider_BaseClasses.h:292
static CfgInt cfg_Oedothorax_EggSacSpread("OEDOTHORAX_EGG_SAC_SPREAD", CFG_CUSTOM, 10)
Definition: PopulationManager.h:71
static CfgFloat cfg_Oedothorax_EggDevelThreshold("OEDOTHORAX_EGGDEVELTHRESHOLD", CFG_CUSTOM, 0.0)
Definition: Oedothorax.h:61
static CfgInt cfg_Oedothorax_BallooningDistanceInterval("OEDOTHORAX_BALLOONINGSTEP", CFG_CUSTOM, 10)
The size of the frequency category for ballooning distances.
double m_BallooningMortalityPerMeter
The mortality of ballooned distance - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:139
void PushIndividual(const unsigned a_listindex, TAnimal *a_individual_ptr)
Definition: PopulationManager.cpp:1682
ofstream * ReallyBigOutputPrb
Definition: PopulationManager.h:873
Definition: Spider_BaseClasses.h:49
virtual void KillThis()
Destroys the spider
Definition: Spider_BaseClasses.h:321
static int m_HatDensityDepMortConst
This is a space for holding cfg species specific parameters - this is the mortality constant associat...
Definition: Spider_BaseClasses.h:349
Definition: SubPopulation.h:48
void Catastrophe()
Allows for the possibility to create population level mortality
Definition: Oedothorax_Population_Manager.cpp:490
static CfgFloat cfg_Oedothorax_DailyJuvMort("OEDOTHORAX_DAILYJUVMORT", CFG_CUSTOM, 0.003)
double m_EggDevelopmentThreshold2
The upper threshold for egg development - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:153
double m_MinWalkTempThreshold
A minimum dispersal by walking temperature
Definition: Spider_BaseClasses.h:173
double m_JuvDegreesPoor
Contribution to day degrees under poor food
Definition: Spider_BaseClasses.h:161
long SupplyGlobalDate(void)
Passes a request on to the associated Calendar class function, returns the simulation global date for...
Definition: Landscape.h:2292
int m_DispersalDistances[10000]
The distribution of dispersal distances - to be assigned in a descendent classes population manager
Definition: Spider_BaseClasses.h:123
virtual ~Oedothorax_Population_Manager()
Definition: Oedothorax_Population_Manager.cpp:140
static CfgFloat cfg_Oedothorax_JuvDevelConst2("OEDOTHORAX_JUVDEVELCONSTTWO", CFG_CUSTOM, 1000.0)
double m_EggProdDDegsGood
Links reproduction to food levels and day degrees - good food.
Definition: Spider_BaseClasses.h:163
double m_TodaysBallooningTime
The current day's ballooning time
Definition: Spider_BaseClasses.h:133
Movement maps are used for rapid computing of animal movement. This version uses values of 0 to max i...
Definition: MovementMap.h:52
void IncLiveArraySize(int a_listindex)
Increments the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:665