File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
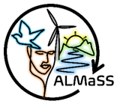 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
28 #ifndef OSBarleySilage_H
29 #define OSBarleySilage_H
31 #define OSBS_BASE 3800
90 #endif // OSBarleySilage_H
int GetMDates(int a, int b)
Definition: Elements.h:405
virtual bool SpringRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the spring on a_field.
Definition: FarmFuncs.cpp:487
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: OSBarleySilage.h:35
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
bool m_lock
Definition: Farm.h:384
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool CutToSilage(LE *a_field, double a_user, int a_days)
Cut vegetation for silage on a_field.
Definition: FarmFuncs.cpp:1644
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
bool m_first_year
Definition: Farm.h:386
class Calendar * g_date
Definition: Calendar.cpp:37
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
Definition: OSBarleySilage.h:36
The base class for all crops.
Definition: Farm.h:495
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: LandscapeFarmingEnums.h:1009
Definition: OSBarleySilage.h:41
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Definition: LandscapeFarmingEnums.h:1003
Definition: OSBarleySilage.h:39
Definition: LandscapeFarmingEnums.h:1011
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: OSBarleySilage.h:38
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: OSBarleySilage.h:42
Definition: OSBarleySilage.h:43
int m_todo
Definition: Farm.h:388
Definition: OSBarleySilage.h:45
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: OSBarleySilage.h:51
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
OSBarleySilage(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: OSBarleySilage.h:55
bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
Definition: OSBarleySilage.cpp:31
virtual void SetGrowthPhase(int)
Definition: Elements.h:185
void SetUpFarmCategoryInformation()
Definition: OSBarleySilage.h:60
Definition: OSBarleySilage.h:37
int DayInYear(void)
Definition: Calendar.h:58
Definition: OSBarleySilage.h:40
Definition: OSBarleySilage.h:44
FarmEvent * m_ev
Definition: Farm.h:500
OBSBSToDo
Definition: OSBarleySilage.h:33
Definition: MapErrorMsg.h:34
Definition: OSBarleySilage.h:46
Definition: OSBarleySilage.h:34
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
#define OSBS_BASE
Definition: OSBarleySilage.h:31
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459