Loading [MathJax]/extensions/ams.js
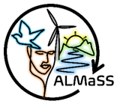 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
31 #define OFBeet_BASE 3100
33 #define OFB_DID_HARROW m_field->m_user[0]
34 #define OFB_DID_NPKS_ONE m_field->m_user[1]
35 #define OFB_DID_SLURRY m_field->m_user[2]
36 #define OFB_SOW_DATE m_field->m_user[3]
38 #define OFB_DID_ROW_TWO m_field->m_user[0]
39 #define OFB_DID_NPKS_TWO m_field->m_user[2]
40 #define OFB_DID_WATER_ONE m_field->m_user[3]
41 #define OFB_TRULY_DID_WATER_ONE m_field->m_user[4]
108 #endif // OFodderBeet_h
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: OFodderBeet.h:54
virtual bool SpringRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the spring on a_field.
Definition: FarmFuncs.cpp:487
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
#define OFB_DID_NPKS_ONE
Definition: OFodderBeet.h:34
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
#define OFB_DID_ROW_TWO
Definition: OFodderBeet.h:38
Definition: OFodderBeet.h:46
Definition: OFodderBeet.h:65
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
int GetMConstants(int a)
Definition: Elements.h:407
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: OFodderBeet.h:48
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: OFodderBeet.h:60
int GetYearNumber(void)
Definition: Calendar.h:72
bool m_first_year
Definition: Farm.h:386
Definition: OFodderBeet.h:58
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: OFodderBeet.h:56
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
TTypesOfFarm GetType(void)
Definition: Farm.h:956
int m_base_elements_no
Definition: Farm.h:505
Definition: OFodderBeet.h:50
Definition: OFodderBeet.h:49
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
The base class for all crops.
Definition: Farm.h:495
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: OFodderBeet.h:51
Definition: OFodderBeet.h:57
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Definition: LandscapeFarmingEnums.h:1003
void SetVegPatchy(bool p)
Definition: Elements.h:229
Definition: LandscapeFarmingEnums.h:1011
Definition: OFodderBeet.h:47
long OldDays(void)
Definition: Calendar.h:60
OFodderBeet(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: OFodderBeet.h:69
Definition: OFodderBeet.h:44
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
#define OFB_TRULY_DID_WATER_ONE
Definition: OFodderBeet.h:41
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
#define OFB_DID_WATER_ONE
Definition: OFodderBeet.h:40
#define OFB_DID_HARROW
Definition: OFodderBeet.h:33
Definition: OFodderBeet.h:52
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
virtual bool RowCultivation(LE *a_field, double a_user, int a_days)
Carry out a harrowing between crop rows on a_field.
Definition: FarmFuncs.cpp:1183
Definition: LandscapeFarmingEnums.h:696
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
Definition: LandscapeFarmingEnums.h:1012
#define OFB_DID_SLURRY
Definition: OFodderBeet.h:35
OFBToDo
Definition: OFodderBeet.h:43
int DayInYear(void)
Definition: Calendar.h:58
Definition: OFodderBeet.h:53
Definition: OFodderBeet.h:59
Definition: OFodderBeet.h:45
#define OFBeet_BASE
Definition: OFodderBeet.h:31
void SetMConstants(int a, int c)
Definition: Elements.h:408
Definition: OFodderBeet.h:55
void SetUpFarmCategoryInformation()
Definition: OFodderBeet.h:74
void ChooseNextCrop(int a_no_dates)
Chooses the next crop to grow in a field.
Definition: Farm.cpp:756
FarmEvent * m_ev
Definition: Farm.h:500
#define OFB_DID_NPKS_TWO
Definition: OFodderBeet.h:39
Definition: MapErrorMsg.h:34
#define OFB_SOW_DATE
Definition: OFodderBeet.h:36
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
Definition: OFodderBeet.cpp:31
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459