Loading [MathJax]/extensions/ams.js
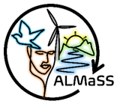 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
21 #ifndef NLCARROTSSPRING_H
22 #define NLCARROTSSPRING_H
24 #define NLCARROTSSPRING_BASE 20700
28 #define NL_CAS_WINTER_PLOUGH a_field->m_user[1]
29 #define NL_CAS_HERBI1 a_field->m_user[2]
30 #define NL_CAS_HERBI2 a_field->m_user[3]
31 #define NL_CAS_FUNGI1 a_field->m_user[4]
32 #define NL_CAS_FUNGI2 a_field->m_user[5]
112 #endif // NLCARROTSSPRING_H
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
Definition: NLCarrotsSpring.h:50
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: NLCarrotsSpring.h:56
CfgFloat l_pest_insecticide_amount
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
Definition: NLCarrotsSpring.h:49
Definition: NLCarrotsSpring.h:47
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
#define NL_CAS_FUNGI2
Definition: NLCarrotsSpring.h:32
#define NL_CAS_HERBI2
Definition: NLCarrotsSpring.h:30
virtual bool BedForming(LE *a_field, double a_user, int a_days)
Do bed forming up on a_field, probably of carrots.
Definition: FarmFuncs.cpp:1316
Definition: LandscapeFarmingEnums.h:1006
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
int m_base_elements_no
Definition: Farm.h:505
NLCarrotsSpring class .
Definition: NLCarrotsSpring.h:69
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
The base class for all crops.
Definition: Farm.h:495
Definition: NLCarrotsSpring.h:43
Definition: NLCarrotsSpring.h:52
Definition: NLCarrotsSpring.h:57
CfgBool cfg_pest_carrots_on
Turn on pesticides for carrots.
#define NLCARROTSSPRING_BASE
Definition: NLCarrotsSpring.h:24
Definition: NLCarrotsSpring.h:44
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: NLCarrotsSpring.h:45
void SetUpFarmCategoryInformation()
Definition: NLCarrotsSpring.h:81
CfgInt cfg_CAS_InsecticideDay
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
int GetSoilType()
Definition: Elements.h:302
Bool configurator entry class.
Definition: Configurator.h:155
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: NLCarrotsSpring.cpp:64
Definition: LandscapeFarmingEnums.h:1003
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: NLCarrotsSpring.h:48
Definition: NLCarrotsSpring.h:53
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
int m_todo
Definition: Farm.h:388
NLCarrotsSpringToDo
Definition: NLCarrotsSpring.h:39
Definition: NLCarrotsSpring.h:41
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: NLCarrotsSpring.h:54
Definition: NLCarrotsSpring.h:40
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
#define NL_CAS_FUNGI1
Definition: NLCarrotsSpring.h:31
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: NLCarrotsSpring.h:42
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
The base class for all farm types.
Definition: Farm.h:755
Double configurator entry class.
Definition: Configurator.h:126
Definition: LandscapeFarmingEnums.h:1012
Definition: NLCarrotsSpring.h:46
Definition: NLCarrotsSpring.h:55
Definition: NLCarrotsSpring.h:51
int DayInYear(void)
Definition: Calendar.h:58
#define NL_CAS_HERBI1
Definition: NLCarrotsSpring.h:29
CfgInt cfg_CAS_InsecticideMonth
#define NL_CAS_WINTER_PLOUGH
A flag used to indicate autumn ploughing status.
Definition: NLCarrotsSpring.h:28
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1007
Definition: LandscapeFarmingEnums.h:263
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
NLCarrotsSpring(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: NLCarrotsSpring.h:73
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751