Loading [MathJax]/extensions/ams.js
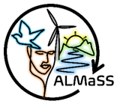 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
24 #define NLCARROTS_BASE 20600
28 #define NL_CA_WINTER_PLOUGH a_field->m_user[1]
29 #define NL_CA_HERBI1 a_field->m_user[2]
30 #define NL_CA_HERBI2 a_field->m_user[3]
31 #define NL_CA_FUNGI1 a_field->m_user[4]
32 #define NL_CA_FUNGI2 a_field->m_user[5]
117 #endif // NLCARROTS_H
int GetMDates(int a, int b)
Definition: Elements.h:405
#define NLCARROTS_BASE
Definition: NLCarrots.h:24
bool m_forcespring
Definition: Farm.h:392
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: NLCarrots.h:45
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
#define NL_CA_WINTER_PLOUGH
A flag used to indicate autumn ploughing status.
Definition: NLCarrots.h:28
Definition: NLCarrots.h:57
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: NLCarrots.h:49
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
virtual bool BedForming(LE *a_field, double a_user, int a_days)
Do bed forming up on a_field, probably of carrots.
Definition: FarmFuncs.cpp:1316
Definition: LandscapeFarmingEnums.h:1006
CfgFloat l_pest_insecticide_amount
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
Definition: NLCarrots.h:46
Definition: NLCarrots.h:53
int m_base_elements_no
Definition: Farm.h:505
Definition: NLCarrots.h:58
Definition: NLCarrots.h:41
Definition: NLCarrots.h:40
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
The base class for all crops.
Definition: Farm.h:495
Definition: NLCarrots.h:47
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
void SetUpFarmCategoryInformation()
Definition: NLCarrots.h:84
CfgInt cfg_CA_InsecticideDay
Definition: NLCarrots.h:44
long Date(void)
Definition: Calendar.h:57
Definition: NLCarrots.h:56
Definition: NLCarrots.h:52
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
int GetSoilType()
Definition: Elements.h:302
Bool configurator entry class.
Definition: Configurator.h:155
NLCarrots(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: NLCarrots.h:75
Definition: LandscapeFarmingEnums.h:1003
#define NL_CA_HERBI2
Definition: NLCarrots.h:30
Definition: NLCarrots.h:51
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
#define NL_CA_FUNGI1
Definition: NLCarrots.h:31
Definition: Configurator.h:208
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: NLCarrots.h:42
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
NLCarrotsToDo
Definition: NLCarrots.h:39
int m_todo
Definition: Farm.h:388
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
#define NL_CA_HERBI1
Definition: NLCarrots.h:29
Definition: NLCarrots.h:55
Definition: Elements.h:86
Definition: NLCarrots.h:43
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: NLCarrots.h:59
CfgInt cfg_CA_InsecticideMonth
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
The base class for all farm types.
Definition: Farm.h:755
Definition: NLCarrots.h:48
Double configurator entry class.
Definition: Configurator.h:126
Definition: LandscapeFarmingEnums.h:1012
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
int DayInYear(void)
Definition: Calendar.h:58
CfgBool cfg_pest_carrots_on
Turn on pesticides for carrots.
Definition: NLCarrots.h:54
NLCarrots class .
Definition: NLCarrots.h:71
#define NL_CA_FUNGI2
Definition: NLCarrots.h:32
bool m_forcespringpossible
Used to signal that the crop can be forced to start in spring.
Definition: Farm.h:508
FarmEvent * m_ev
Definition: Farm.h:500
Definition: NLCarrots.h:50
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1007
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: NLCarrots.cpp:64
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751
Definition: LandscapeFarmingEnums.h:252