Loading [MathJax]/extensions/ams.js
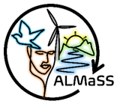 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
24 #define NLCABBAGE_BASE 20400
28 #define NL_CAB_WINTER_PLOUGH a_field->m_user[1]
130 #endif // NLCABBAGE_H
Definition: NLCabbage.h:42
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: NLCabbage.h:56
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool FP_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply Manganse Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:840
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
CfgInt cfg_CAB_InsecticideDay
Provided to allow configuration control of the first insecticide spray in cabbage crops - this change...
Definition: NLCabbage.h:37
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
NLCabbageToDo
Definition: NLCabbage.h:35
Definition: LandscapeFarmingEnums.h:1006
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
Definition: NLCabbage.h:45
Definition: NLCabbage.h:63
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
The base class for all crops.
Definition: Farm.h:495
Definition: NLCabbage.h:44
bool value() const
Definition: Configurator.h:164
Definition: LandscapeFarmingEnums.h:1009
Definition: NLCabbage.h:46
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
NLCabbage(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: NLCabbage.h:79
Definition: NLCabbage.h:59
long Date(void)
Definition: Calendar.h:57
Definition: NLCabbage.h:51
#define NLCABBAGE_BASE
Definition: NLCabbage.h:24
NLCabbage class .
Definition: NLCabbage.h:75
void SetUpFarmCategoryInformation()
Definition: NLCabbage.h:88
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: NLCabbage.h:61
Definition: LandscapeFarmingEnums.h:1008
Definition: NLCabbage.h:58
int GetSoilType()
Definition: Elements.h:302
Bool configurator entry class.
Definition: Configurator.h:155
Definition: NLCabbage.h:52
Definition: NLCabbage.h:38
Definition: LandscapeFarmingEnums.h:1003
Definition: LandscapeFarmingEnums.h:1011
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: NLCabbage.cpp:64
Definition: NLCabbage.h:41
virtual bool FA_ManganeseSulphate(LE *a_field, double a_user, int a_days)
Apply manganese sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1095
long OldDays(void)
Definition: Calendar.h:60
Definition: NLCabbage.h:60
CfgBool cfg_pest_cabbage_on
Turn on pesticides for cabbage.
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
Definition: NLCabbage.h:53
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: NLCabbage.h:49
Definition: NLCabbage.h:50
Definition: NLCabbage.h:40
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: NLCabbage.h:39
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
CfgInt cfg_CAB_InsecticideMonth
Provided to allow configuration control of the first insecticide spray in cabbage crops - this change...
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: NLCabbage.h:62
virtual double GetGreenBiomass(void)
Definition: Elements.h:160
virtual bool ShallowHarrow(LE *a_field, double a_user, int a_days)
Carry out a shallow harrow event on a_field, e.g., after grass cutting event.
Definition: FarmFuncs.cpp:473
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
Definition: NLCabbage.h:36
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: NLCabbage.h:43
CfgFloat l_pest_insecticide_amount
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
#define NL_CAB_WINTER_PLOUGH
A flag used to indicate autumn ploughing status.
Definition: NLCabbage.h:28
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
The base class for all farm types.
Definition: Farm.h:755
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Double configurator entry class.
Definition: Configurator.h:126
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: LandscapeFarmingEnums.h:1012
Definition: LandscapeFarmingEnums.h:257
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
Definition: NLCabbage.h:47
Definition: NLCabbage.h:57
int DayInYear(void)
Definition: Calendar.h:58
bool m_forcespringpossible
Used to signal that the crop can be forced to start in spring.
Definition: Farm.h:508
Definition: NLCabbage.h:54
FarmEvent * m_ev
Definition: Farm.h:500
Definition: NLCabbage.h:55
Definition: NLCabbage.h:48
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751