File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
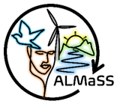 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
50 #ifndef IRWINTERBARLEY_H
51 #define IRWINTERBARLEY_H
53 #define IR_WB_BASE 90500
57 #define IR_WB_MT a_field->m_user[1]
58 #define IR_WB_CP a_field->m_user[2]
165 #endif // IRWINTERBARLEY_H
int GetMDates(int a, int b)
Definition: Elements.h:405
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
Definition: LandscapeFarmingEnums.h:1005
void SetMDates(int a, int b, int c)
Definition: Elements.h:406
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
Definition: IRWinterBarley.h:82
bool IsStockFarmer(void)
Definition: Farm.h:961
CfgFloat cfg_fungi_app_prop1
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: IRWinterBarley.h:70
int GetYearNumber(void)
Definition: Calendar.h:72
Definition: IRWinterBarley.h:91
Definition: IRWinterBarley.h:80
Definition: IRWinterBarley.h:85
bool m_first_year
Definition: Farm.h:386
virtual bool Molluscicide(LE *a_field, double a_user, int a_days)
Apply molluscicide to a_field.
Definition: FarmFuncs.cpp:2310
Definition: IRWinterBarley.h:81
int GetPoly(void)
Returns the polyref number for this polygon.
Definition: Elements.h:238
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
TTypesOfVegetation GetPreviousTov(int a_index)
Definition: Farm.h:966
#define IR_WB_MT
A flag used to indicate autumn ploughing status.
Definition: IRWinterBarley.h:57
TTypesOfFarm GetType(void)
Definition: Farm.h:956
CfgFloat cfg_herbi_app_prop
int m_base_elements_no
Definition: Farm.h:505
Definition: IRWinterBarley.h:71
CfgFloat cfg_ins_app_prop2
Definition: IRWinterBarley.h:69
Definition: IRWinterBarley.h:73
CfgFloat cfg_ins_app_prop1
int m_first_date
Definition: Farm.h:501
int m_startday
Definition: Farm.h:385
CfgInt cfg_SB_InsecticideMonth
Provided to allow configuration control of the first insecticide spray in spring barley crops - this ...
Definition: SpringBarleySilage.cpp:43
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: IRWinterBarley.h:68
CfgBool cfg_IR_WinterBarley_SkScrapes("IR_CROP_WB_SK_SCRAPES", CFG_CUSTOM, false)
The base class for all crops.
Definition: Farm.h:495
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
#define IR_WB_BASE
Definition: IRWinterBarley.h:53
bool value() const
Definition: Configurator.h:164
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: IRWinterBarley.h:74
Definition: IRWinterBarley.h:78
IRWinterBarley class .
Definition: IRWinterBarley.h:107
bool m_skylarkscrapes
For management testing of skylark scrapes.
Definition: Elements.h:95
long Date(void)
Definition: Calendar.h:57
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Definition: LandscapeFarmingEnums.h:1008
Definition: IRWinterBarley.h:87
Definition: IRWinterBarley.h:90
Bool configurator entry class.
Definition: Configurator.h:155
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: IRWinterBarley.h:95
Definition: IRWinterBarley.h:93
Definition: LandscapeFarmingEnums.h:1003
Definition: IRWinterBarley.h:88
CfgFloat cfg_ins_app_prop3
long OldDays(void)
Definition: Calendar.h:60
Definition: IRWinterBarley.h:75
CfgInt cfg_SB_InsecticideDay
Provided to allow configuration control of the first insecticide spray in spring barley crops - this ...
Definition: SpringBarleySilage.cpp:42
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
int GetRotIndex(void)
Definition: Elements.h:373
IRWinterBarley(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: IRWinterBarley.h:111
TTypesOfVegetation m_next_tov
Definition: Farm.h:390
void SetUpFarmCategoryInformation()
Definition: IRWinterBarley.h:119
Definition: IRWinterBarley.h:77
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
IRWinterBarleyToDo
Definition: IRWinterBarley.h:66
CfgFloat cfg_greg_app_prop
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: IRWinterBarley.h:92
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
CfgBool cfg_pest_winterbarley_on
Turn on pesticides for winter barley.
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
virtual bool AutumnRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the autumn on a_field.
Definition: FarmFuncs.cpp:299
virtual bool FP_Slurry(LE *a_field, double a_user, int a_days)
Apply slurry to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:823
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: LandscapeFarmingEnums.h:696
Definition: Elements.h:86
CfgFloat cfg_fungi_app_prop2
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
Definition: IRWinterBarley.h:86
Definition: IRWinterBarley.h:72
Definition: IRWinterBarley.h:89
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
The base class for all farm types.
Definition: Farm.h:755
Double configurator entry class.
Definition: Configurator.h:126
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
Definition: LandscapeFarmingEnums.h:1012
Definition: IRWinterBarley.h:76
Definition: IRWinterBarley.h:83
Definition: Configurator.h:70
Farm * GetOwner(void)
Definition: Elements.h:256
int DayInYear(void)
Definition: Calendar.h:58
Definition: IRWinterBarley.h:94
Definition: IRWinterBarley.h:84
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: IRWinterBarley.cpp:98
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
void SetMConstants(int a, int c)
Definition: Elements.h:408
Definition: IRWinterBarley.h:67
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
Definition: IRWinterBarley.h:79
CfgFloat l_pest_insecticide_amount
#define IR_WB_CP
Definition: IRWinterBarley.h:58