Loading [MathJax]/extensions/ams.js
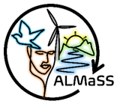 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
50 #ifndef FI_SPRINGWHEAT_H
51 #define FI_SPRINGWHEAT_H
53 #define FI_SW_BASE 70700
57 #define FI_SW_AUTUMN_PLOUGH a_field->m_user[1]
58 #define FI_SW_DECIDE_TO_HERB a_field->m_user[2]
59 #define FI_SW_DECIDE_TO_FI a_field->m_user[3]
151 #endif // FI_SPRINGWHEAT_H
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
CfgFloat cfg_herbi_app_prop
Definition: LandscapeFarmingEnums.h:1005
Definition: FI_SpringOat.h:76
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
Definition: FI_SpringWheat.h:69
Definition: FI_SpringOat.h:81
Definition: FI_SpringOat.h:70
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
Definition: FI_SpringWheat.h:87
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
Definition: FI_SpringWheat.h:71
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: FI_SpringWheat.h:88
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: FI_SpringOat.h:84
Definition: FI_SpringOat.h:71
#define FI_SW_BASE
Definition: FI_SpringWheat.h:53
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
Definition: FI_SpringWheat.h:74
int m_base_elements_no
Definition: Farm.h:505
Definition: FI_SpringOat.h:68
Definition: FI_SpringOat.h:72
int m_first_date
Definition: Farm.h:501
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: FI_SpringWheat.cpp:98
CfgFloat cfg_ins_app_prop3
The base class for all crops.
Definition: Farm.h:495
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: FI_SpringWheat.h:82
CfgBool cfg_pest_springwheat_on
Turn on pesticides for spring wheat.
bool value() const
Definition: Configurator.h:164
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: FI_SpringOat.h:75
Definition: FI_SpringWheat.h:84
Definition: FI_SpringOat.h:82
Definition: FI_SpringOat.h:86
FI_SpringWheatToDo
Definition: FI_SpringWheat.h:67
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Bool configurator entry class.
Definition: Configurator.h:155
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
Definition: FI_SpringWheat.h:76
long OldDays(void)
Definition: Calendar.h:60
CfgInt cfg_SW_InsecticideMonth
Definition: FI_SpringOat.h:85
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
Definition: FI_SpringWheat.h:72
Definition: LandscapeFarmingEnums.h:531
CfgFloat cfg_greg_app_prop
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
void SetUpFarmCategoryInformation()
Definition: FI_SpringWheat.h:113
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
CfgFloat l_pest_insecticide_amount
Definition: FI_SpringWheat.h:85
Definition: FI_SpringWheat.h:68
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: FI_SpringWheat.h:80
std::vector< double > value() const
Definition: Configurator.h:219
Definition: FI_SpringOat.h:74
virtual bool PreseedingCultivatorSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out preseeding cultivation together with sow on a_field (tilling and sowing set including culti...
Definition: FarmFuncs.cpp:325
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
CfgInt cfg_SW_InsecticideDay
virtual bool BiocideTreat(LE *a_field, double a_user, int a_days)
Apply Biocide to a_field.
Definition: FarmFuncs.cpp:2175
Definition: FI_SpringOat.h:87
Definition: FI_SpringWheat.h:77
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: FI_SpringOat.h:79
virtual bool SpringSowWithFerti(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event with start fertilizer in the spring on a_field.
Definition: FarmFuncs.cpp:537
Definition: FI_SpringWheat.h:86
Definition: FI_SpringOat.h:80
Definition: FI_SpringWheat.h:78
CfgFloat cfg_ins_app_prop1
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
The base class for all farm types.
Definition: Farm.h:755
Definition: FI_SpringWheat.h:81
Definition: FI_SpringWheat.h:73
Definition: FI_SpringWheat.h:83
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Double configurator entry class.
Definition: Configurator.h:126
FI_SpringWheat(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: FI_SpringWheat.h:104
Definition: FI_SpringWheat.h:70
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: LandscapeFarmingEnums.h:1012
CfgFloat cfg_fungi_app_prop2
virtual bool GrowthRegulator(LE *a_field, double a_user, int a_days)
Apply growth regulator to a_field.
Definition: FarmFuncs.cpp:2070
CfgBool cfg_FI_SpringWheat_SkScrapes("DK_CROP_SW_SK_SCRAPES", CFG_CUSTOM, false)
Definition: Configurator.h:70
int DayInYear(void)
Definition: Calendar.h:58
CfgFloat cfg_ins_app_prop2
bool m_forcespringpossible
Used to signal that the crop can be forced to start in spring.
Definition: Farm.h:508
Definition: FI_SpringWheat.h:75
Definition: FI_SpringWheat.h:79
Definition: FI_SpringOat.h:78
FarmEvent * m_ev
Definition: Farm.h:500
Definition: FI_SpringOat.h:73
CfgFloat cfg_fungi_app_prop1
Definition: MapErrorMsg.h:34
Definition: FI_SpringOat.h:77
Definition: LandscapeFarmingEnums.h:1079
Definition: FI_SpringOat.h:83
FI_SpringWheat class .
Definition: FI_SpringOat.h:99
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
virtual bool FP_NPKS(LE *a_field, double a_user, int a_days)
Apply NPKS fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:630
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751