File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
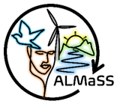 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
36 #ifndef DK_OPotatoIndustry_H
37 #define DK_OPotatoIndustry_H
39 #define DK_OPOI_BASE 69500
40 #define DK_OPOI_FORCESPRING a_field->m_user[1]
41 #define DK_OPOI_SANDY a_field->m_user[2]
129 #endif // DK_OPotatoIndustry_H
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
Definition: DK_OPotatoIndustry.h:64
Definition: DK_OPotatoIndustry.h:69
Definition: DK_OPotatoIndustry.h:79
virtual bool SpringPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the spring on a_field.
Definition: FarmFuncs.cpp:421
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: DK_OPotatoIndustry.h:60
bool m_lock
Definition: Farm.h:384
virtual bool StubbleCultivatorHeavy(LE *a_field, double a_user, int a_days)
Carry out a stubble cultivation event on a_field. This is non-inversion type of cultivation which can...
Definition: FarmFuncs.cpp:245
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
virtual bool StrawRemoval(LE *a_field, double a_user, int a_days)
Straw covering applied on a_field.
Definition: FarmFuncs.cpp:1752
Definition: DK_OPotatoIndustry.h:59
Definition: LandscapeFarmingEnums.h:722
Definition: DK_OPotatoIndustry.h:54
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: DK_OPotatoIndustry.h:61
Definition: LandscapeFarmingEnums.h:721
Definition: DK_OPotatoIndustry.h:71
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
int m_base_elements_no
Definition: Farm.h:505
Definition: DK_OPotatoIndustry.h:68
void SetUpFarmCategoryInformation()
Definition: DK_OPotatoIndustry.h:88
DK_OPotatoIndustry(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DK_OPotatoIndustry.h:83
Definition: LandscapeFarmingEnums.h:390
int m_first_date
Definition: Farm.h:501
Definition: DK_OPotatoIndustry.h:74
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
virtual bool HillingUp(LE *a_field, double a_user, int a_days)
Do hilling up on a_field, probably of potatoes.
Definition: FarmFuncs.cpp:1302
Definition: DK_OPotatoIndustry.h:73
The base class for all crops.
Definition: Farm.h:495
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: DK_OPotatoIndustry.h:55
Definition: DK_OPotatoIndustry.h:63
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
Definition: DK_OPotatoIndustry.h:58
Definition: LandscapeFarmingEnums.h:1008
int GetSoilType()
Definition: Elements.h:302
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
Definition: LandscapeFarmingEnums.h:1011
#define DK_OPOI_BASE
Definition: DK_OPotatoIndustry.h:39
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
Definition: DK_OPotatoIndustry.h:48
Definition: DK_OPotatoIndustry.h:53
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DK_OPotatoIndustry.h:52
Definition: DK_OPotatoIndustry.h:50
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
Definition: DK_OPotatoIndustry.h:72
int m_todo
Definition: Farm.h:388
Definition: LandscapeFarmingEnums.h:719
Definition: DK_OPotatoIndustry.h:47
Definition: DK_OPotatoIndustry.h:51
Definition: DK_OPotatoIndustry.h:46
virtual bool HarvestLong(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field (only differs in the DoIt chance cf harvest)
Definition: FarmFuncs.cpp:1421
Definition: DK_OPotatoIndustry.h:70
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
virtual bool RowCultivation(LE *a_field, double a_user, int a_days)
Carry out a harrowing between crop rows on a_field.
Definition: FarmFuncs.cpp:1183
Definition: DK_OPotatoIndustry.h:56
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: DK_OPotatoIndustry.h:57
Definition: DK_OPotatoIndustry.h:44
The base class for all farm types.
Definition: Farm.h:755
bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
Definition: DK_OPotatoIndustry.cpp:32
#define DK_OPOI_FORCESPRING
Definition: DK_OPotatoIndustry.h:40
Definition: LandscapeFarmingEnums.h:1012
int DayInYear(void)
Definition: Calendar.h:58
Definition: DK_OPotatoIndustry.h:62
Definition: DK_OPotatoIndustry.h:45
#define DK_OPOI_SANDY
Definition: DK_OPotatoIndustry.h:41
DK_OPotatoIndustryToDo
Definition: DK_OPotatoIndustry.h:43
Definition: DK_OPotatoIndustry.h:65
Definition: DK_OPotatoIndustry.h:67
FarmEvent * m_ev
Definition: Farm.h:500
Definition: LandscapeFarmingEnums.h:720
virtual bool BurnTop(LE *a_field, double a_user, int a_days)
Burn tops of e.g. potatoes on a_field.
Definition: FarmFuncs.cpp:1587
Definition: DK_OPotatoIndustry.h:49
Definition: MapErrorMsg.h:34
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
Definition: DK_OPotatoIndustry.h:66