Loading [MathJax]/extensions/ams.js
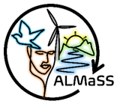 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
50 #ifndef DK_OCLOVERGRASSGRAZED1_H
51 #define DK_OCLOVERGRASSGRAZED1_H
53 #define DK_OC1_BASE 61700
54 #define GRASS_READY_OC1 m_field->m_user[0]
139 #endif // DK_OCloverGrassGrazed1_H
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: DK_OCloverGrassGrazed1.h:79
Definition: DK_OCloverGrassGrazed1.h:74
Definition: DK_OCloverGrassGrazed1.h:67
bool m_forcespring
Definition: Farm.h:392
DK_OCloverGrassGrazed1 class .
Definition: DK_OCloverGrassGrazed1.h:95
bool IsStockFarmer(void)
Definition: Farm.h:961
bool m_lock
Definition: Farm.h:384
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
virtual bool CattleIsOut(LE *a_field, double a_user, int a_days, int a_max)
Generate a 'cattle_out' event for every day the cattle are on a_field.
Definition: FarmFuncs.cpp:2470
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool CutToSilage(LE *a_field, double a_user, int a_days)
Cut vegetation for silage on a_field.
Definition: FarmFuncs.cpp:1644
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
CfgInt cfg_farm_cattle_grass_high
Definition: LandscapeFarmingEnums.h:374
virtual void ForceGrowthTest(void)
Definition: Elements.h:188
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
int m_base_elements_no
Definition: Farm.h:505
Definition: DK_OCloverGrassGrazed1.h:73
Definition: DK_OCloverGrassGrazed1.h:82
int m_first_date
Definition: Farm.h:501
The base class for all crops.
Definition: Farm.h:495
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: DK_OCloverGrassGrazed1.h:80
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: LandscapeFarmingEnums.h:1009
void SetUpFarmCategoryInformation()
Definition: DK_OCloverGrassGrazed1.h:107
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
DK_OCloverGrassGrazed1(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DK_OCloverGrassGrazed1.h:99
Bool configurator entry class.
Definition: Configurator.h:155
Definition: LandscapeFarmingEnums.h:1003
#define GRASS_READY_OC1
Definition: DK_OCloverGrassGrazed1.h:54
virtual double GetVegHeight(void)
Definition: Elements.h:148
Definition: LandscapeFarmingEnums.h:1011
Definition: DK_OCloverGrassGrazed1.h:70
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
CfgInt cfg_farm_cattle_grass_low
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
int value() const
Definition: Configurator.h:116
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: DK_OCloverGrassGrazed1.h:77
Definition: Elements.h:86
virtual bool Water(LE *a_field, double a_user, int a_days)
Carry out a watering on a_field.
Definition: FarmFuncs.cpp:1330
int m_last_date
Definition: Farm.h:503
Definition: DK_OCloverGrassGrazed1.h:66
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DK_OCloverGrassGrazed1.h:81
Definition: LandscapeFarmingEnums.h:1010
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Integer configurator entry class.
Definition: Configurator.h:102
Definition: DK_OCloverGrassGrazed1.h:78
The base class for all farm types.
Definition: Farm.h:755
Definition: DK_OCloverGrassGrazed1.h:68
Definition: DK_OCloverGrassGrazed1.h:83
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: DK_OCloverGrassGrazed1.cpp:88
Definition: Configurator.h:70
int DayInYear(void)
Definition: Calendar.h:58
Definition: DK_OCloverGrassGrazed1.h:69
CfgBool cfg_DK_OCloverGrassGrazed1_SkScrapes("DK_CROP_oc1_SK_SCRAPES", CFG_CUSTOM, false)
Definition: DK_OCloverGrassGrazed1.h:71
Definition: DK_OCloverGrassGrazed1.h:75
Definition: DK_OCloverGrassGrazed1.h:76
Definition: DK_OCloverGrassGrazed1.h:72
FarmEvent * m_ev
Definition: Farm.h:500
virtual bool CattleOut(LE *a_field, double a_user, int a_days)
Start a grazing event on a_field today.
Definition: FarmFuncs.cpp:2368
Definition: MapErrorMsg.h:34
DK_OCloverGrassGrazed1ToDo
Definition: DK_OCloverGrassGrazed1.h:65
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
#define DK_OC1_BASE
Definition: DK_OCloverGrassGrazed1.h:53