File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
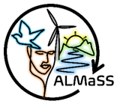 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Definition: DE_Orchard.h:83
#define DE_ORCH_BASE
Definition: DE_Orchard.h:51
Definition: DE_Orchard.h:88
Definition: DE_Orchard.h:92
Definition: LandscapeFarmingEnums.h:1005
Definition: DE_Orchard.h:86
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
bool m_lock
Definition: Farm.h:384
Definition: DE_Orchard.h:99
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
Definition: DE_Orchard.h:70
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
Definition: DE_Orchard.h:69
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
Definition: DE_Orchard.h:84
Definition: DE_Orchard.h:91
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
Definition: DE_Orchard.h:98
Definition: DE_Orchard.h:76
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
Definition: DE_Orchard.h:100
Definition: DE_Orchard.h:65
int m_base_elements_no
Definition: Farm.h:505
DE_Orchard class .
Definition: DE_Orchard.h:113
Definition: DE_Orchard.h:90
Definition: DE_Orchard.h:78
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
Definition: DE_Orchard.h:77
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: DE_Orchard.h:68
Definition: DE_Orchard.h:85
The base class for all crops.
Definition: Farm.h:495
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: DE_Orchard.h:95
bool value() const
Definition: Configurator.h:164
Definition: DE_Orchard.h:87
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: DE_Orchard.cpp:89
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
void SetUpFarmCategoryInformation()
Definition: DE_Orchard.h:127
Definition: DE_Orchard.h:82
CfgBool cfg_pest_orchard_on
Turn on pesticides for orchard.
long Date(void)
Definition: Calendar.h:57
Definition: DE_Orchard.h:71
Definition: DE_Orchard.h:66
virtual bool FP_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply Ammonium Sulphate to a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:882
Bool configurator entry class.
Definition: Configurator.h:155
Definition: LandscapeFarmingEnums.h:1003
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DE_Orchard.h:81
double SupplyPestIncidenceFactor()
Returns Landscape::m_pestincidencefactor.
Definition: Landscape.h:325
Definition: DE_Orchard.h:72
Definition: DE_Orchard.h:73
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Landscape * g_landscape_ptr
Definition: Landscape.cpp:352
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: DE_Orchard.h:79
Definition: DE_Orchard.h:94
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
DE_Orchard(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DE_Orchard.h:117
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DE_Orchard.h:93
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
virtual bool FA_AmmoniumSulphate(LE *a_field, double a_user, int a_days)
Apply ammonium sulphate to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1081
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
Definition: LandscapeFarmingEnums.h:518
Definition: DE_Orchard.h:101
Definition: DE_Orchard.h:64
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: DE_Orchard.h:96
Definition: LandscapeFarmingEnums.h:1012
virtual bool FruitHarvest(LE *a_field, double a_user, int a_days)
FruitHarvest (harvest of the mature fruits, e.g., grapes) applied on a_field.
Definition: FarmFuncs.cpp:1959
Definition: DE_Orchard.h:80
DE_ORCHToDo
A flag used to indicate autumn ploughing status.
Definition: DE_Orchard.h:63
Definition: DE_Orchard.h:67
Definition: DE_Orchard.h:89
int DayInYear(void)
Definition: Calendar.h:58
Definition: DE_Orchard.h:97
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
Definition: DE_Orchard.h:75
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: DE_Orchard.h:74
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751