File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
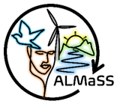 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
28 #ifndef DE_OWINTERWHEAT_H
29 #define DE_OWINTERWHEAT_H
31 #define DE_OWINTERWHEAT_BASE 38200
107 #endif // DE_OWINTERWHEAT_H
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: DE_OWinterWheat.h:36
Definition: DE_OWinterWheat.h:46
Definition: DE_OWinterWheat.h:51
virtual bool SpringRoll(LE *a_field, double a_user, int a_days)
Carry out a roll event in the spring on a_field.
Definition: FarmFuncs.cpp:487
Definition: LandscapeFarmingEnums.h:497
virtual bool FA_Slurry(LE *a_field, double a_user, int a_days)
Spready slurry on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1067
CfgFloat cfg_strigling_prop
bool IsStockFarmer(void)
Definition: Farm.h:961
virtual bool Strigling(LE *a_field, double a_user, int a_days)
Carry out a mechanical weeding on a_field.
Definition: FarmFuncs.cpp:1206
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
Definition: DE_OWinterWheat.h:44
Definition: DE_OWinterWheat.h:50
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
bool DoIt(double a_probability)
Return chance out of 0 to 100.
Definition: Farm.cpp:856
Definition: DE_OWinterWheat.h:42
Definition: DE_OWinterWheat.h:53
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
Definition: DE_OWinterWheat.h:59
int m_base_elements_no
Definition: Farm.h:505
Definition: DE_OWinterWheat.h:47
int m_first_date
Definition: Farm.h:501
DE_OWinterWheat(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DE_OWinterWheat.h:63
DE_OWinterWheatToDo
Definition: DE_OWinterWheat.h:33
The base class for all crops.
Definition: Farm.h:495
Definition: DE_OWinterWheat.h:54
#define DE_OWINTERWHEAT_BASE
Definition: DE_OWinterWheat.h:31
Definition: DE_OWinterWheat.h:49
Definition: DE_OWinterWheat.h:34
Definition: DE_OWinterWheat.h:35
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: DE_OWinterWheat.h:48
Definition: LandscapeFarmingEnums.h:1009
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: DE_OWinterWheat.h:37
Definition: LandscapeFarmingEnums.h:1004
Definition: DE_OWinterWheat.h:41
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
virtual bool HayBailing(LE *a_field, double a_user, int a_days)
Carry out hay bailing on a_field.
Definition: FarmFuncs.cpp:1507
Definition: LandscapeFarmingEnums.h:1003
virtual bool StrawChopping(LE *a_field, double a_user, int a_days)
Carry out straw chopping on a_field.
Definition: FarmFuncs.cpp:1475
void SetUpFarmCategoryInformation()
Definition: DE_OWinterWheat.h:68
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
Definition: DE_OWinterWheat.h:52
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: DE_OWinterWheat.h:39
Definition: Elements.h:86
Definition: DE_OWinterWheat.h:40
int m_last_date
Definition: Farm.h:503
virtual bool AutumnPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the autumn on a_field.
Definition: FarmFuncs.cpp:212
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
virtual bool DeepPlough(LE *a_field, double a_user, int a_days)
Carry out a deep ploughing event on a_field.
Definition: FarmFuncs.cpp:408
Double configurator entry class.
Definition: Configurator.h:126
virtual bool AutumnSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the autumn on a_field.
Definition: FarmFuncs.cpp:360
Definition: LandscapeFarmingEnums.h:1012
Definition: DE_OWinterWheat.h:38
int DayInYear(void)
Definition: Calendar.h:58
Definition: DE_OWinterWheat.h:43
Definition: DE_OWinterWheat.h:55
virtual bool HayTurning(LE *a_field, double a_user, int a_days)
Carry out hay turning on a_field.
Definition: FarmFuncs.cpp:1491
FarmEvent * m_ev
Definition: Farm.h:500
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
Definition: DE_OWinterWheat.cpp:33
Definition: MapErrorMsg.h:34
Definition: DE_OWinterWheat.h:45
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool StubbleHarrowing(LE *a_field, double a_user, int a_days)
Carry out stubble harrowing on a_field.
Definition: FarmFuncs.cpp:1523
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751