Loading [MathJax]/extensions/ams.js
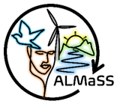 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
53 #define DE_OORCH_RC_DATE m_field->m_user[0]
55 #define DE_OORCH_BASE 39600
Definition: DE_OOrchard.h:79
Definition: DE_OOrchard.h:110
Definition: DE_OOrchard.h:119
virtual bool OrganicInsecticide(LE *a_field, double a_user, int a_days)
Biocide applied on a_field.
Definition: FarmFuncs.cpp:2195
Definition: DE_OOrchard.h:92
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: DE_OOrchard.h:98
bool m_lock
Definition: Farm.h:384
Definition: DE_OOrchard.h:99
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
Definition: DE_OOrchard.h:72
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
virtual bool FP_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:645
Definition: DE_OOrchard.h:124
Definition: DE_OOrchard.h:82
Definition: DE_OOrchard.h:105
Definition: DE_OOrchard.h:107
Definition: DE_OOrchard.h:95
Definition: DE_OOrchard.h:75
Definition: DE_OOrchard.h:122
Definition: DE_OOrchard.h:85
Definition: DE_OOrchard.h:108
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: DE_OOrchard.h:118
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
Definition: DE_OOrchard.h:83
Definition: DE_OOrchard.h:88
Definition: DE_OOrchard.h:109
int m_base_elements_no
Definition: Farm.h:505
int m_first_date
Definition: Farm.h:501
virtual bool FA_NPK(LE *a_field, double a_user, int a_days)
Apply NPK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:982
Definition: DE_OOrchard.h:121
Definition: DE_OOrchard.h:71
The base class for all crops.
Definition: Farm.h:495
void SimpleEvent(long a_date, int a_todo, bool a_lock)
Adds an event to this crop management.
Definition: Farm.cpp:747
Definition: DE_OOrchard.h:106
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: DE_OOrchard.cpp:87
Definition: DE_OOrchard.h:87
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
Definition: DE_OOrchard.h:117
Definition: DE_OOrchard.h:80
Definition: DE_OOrchard.h:128
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
Definition: DE_OOrchard.h:116
Definition: DE_OOrchard.h:113
Definition: LandscapeFarmingEnums.h:1003
virtual bool FA_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:1168
Definition: DE_OOrchard.h:102
Definition: DE_OOrchard.h:81
Definition: DE_OOrchard.h:129
Definition: DE_OOrchard.h:69
Definition: DE_OOrchard.h:89
long OldDays(void)
Definition: Calendar.h:60
Definition: DE_OOrchard.h:100
Definition: LandscapeFarmingEnums.h:519
Definition: DE_OOrchard.h:130
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
virtual bool FP_Calcium(LE *a_field, double a_user, int a_days)
Calcium applied on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:954
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DE_OOrchard.h:91
Definition: DE_OOrchard.h:103
Definition: DE_OOrchard.h:84
Definition: DE_OOrchard.h:78
virtual bool ManualWeeding(LE *a_field, double a_user, int a_days)
Manual weeding on a_field - no tramlines since weeding by hand, the bush stays on field with same veg...
Definition: FarmFuncs.cpp:2002
Definition: DE_OOrchard.h:90
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
virtual bool Pheromone(LE *a_field, double a_user, int a_days)
Pheromone applied on a_field - same as with org. pesticides for now.
Definition: FarmFuncs.cpp:2249
Definition: DE_OOrchard.h:73
int m_todo
Definition: Farm.h:388
#define DE_OORCH_BASE
Definition: DE_OOrchard.h:55
Definition: DE_OOrchard.h:70
Definition: DE_OOrchard.h:94
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
virtual bool RowCultivation(LE *a_field, double a_user, int a_days)
Carry out a harrowing between crop rows on a_field.
Definition: FarmFuncs.cpp:1183
Definition: DE_OOrchard.h:112
Definition: DE_OOrchard.h:127
Definition: DE_OOrchard.h:104
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
Definition: DE_OOrchard.h:74
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DE_OOrchard.h:111
Definition: DE_OOrchard.h:68
Definition: DE_OOrchard.h:93
#define DE_OORCH_RC_DATE
Definition: DE_OOrchard.h:53
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
DE_OOrchard class .
Definition: DE_OOrchard.h:142
Definition: DE_OOrchard.h:125
The base class for all farm types.
Definition: Farm.h:755
Definition: DE_OOrchard.h:114
Definition: DE_OOrchard.h:76
Definition: LandscapeFarmingEnums.h:1012
virtual bool FruitHarvest(LE *a_field, double a_user, int a_days)
FruitHarvest (harvest of the mature fruits, e.g., grapes) applied on a_field.
Definition: FarmFuncs.cpp:1959
DE_OORCHToDo
A flag used to indicate autumn ploughing status.
Definition: DE_OOrchard.h:67
Definition: DE_OOrchard.h:96
Definition: DE_OOrchard.h:97
Landscape * g_landscape_ptr
Definition: Landscape.cpp:352
Definition: DE_OOrchard.h:77
int DayInYear(void)
Definition: Calendar.h:58
DE_OOrchard(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DE_OOrchard.h:146
virtual bool OrganicFungicide(LE *a_field, double a_user, int a_days)
Biocide applied on a_field.
Definition: FarmFuncs.cpp:2231
Definition: DE_OOrchard.h:123
Definition: DE_OOrchard.h:126
Definition: DE_OOrchard.h:101
void SetUpFarmCategoryInformation()
Definition: DE_OOrchard.h:156
FarmEvent * m_ev
Definition: Farm.h:500
Definition: DE_OOrchard.h:86
Definition: MapErrorMsg.h:34
Definition: DE_OOrchard.h:115
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: DE_OOrchard.h:120
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751