Loading [MathJax]/extensions/ams.js
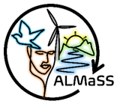 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
55 #define DE_LEG_BASE 36100
145 #endif // DE_LEGUMES_H
Definition: DE_Legumes.h:66
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: DE_Legumes.h:83
Definition: LandscapeFarmingEnums.h:1005
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: DE_Legumes.h:78
virtual bool Harvest(LE *a_field, double a_user, int a_days)
Carry out a harvest on a_field.
Definition: FarmFuncs.cpp:1364
bool m_lock
Definition: Farm.h:384
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: DE_Legumes.cpp:91
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
DE_Legumes class .
Definition: DE_Legumes.h:97
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
Definition: DE_Legumes.h:79
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
int m_base_elements_no
Definition: Farm.h:505
Definition: DE_Legumes.h:70
virtual bool FP_SK(LE *a_field, double a_user, int a_days)
Apply SK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:794
int m_first_date
Definition: Farm.h:501
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
Definition: DE_Legumes.h:75
The base class for all crops.
Definition: Farm.h:495
Definition: DE_Legumes.h:69
Definition: LandscapeFarmingEnums.h:476
bool value() const
Definition: Configurator.h:164
Definition: DE_Legumes.h:84
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
long Date(void)
Definition: Calendar.h:57
DE_LegumesToDo
Flags.
Definition: DE_Legumes.h:65
Landscape * g_landscape_ptr
Definition: Landscape.cpp:352
Definition: LandscapeFarmingEnums.h:1008
Bool configurator entry class.
Definition: Configurator.h:155
Definition: LandscapeFarmingEnums.h:1003
Definition: DE_Legumes.h:74
long OldDays(void)
Definition: Calendar.h:60
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
double SupplyPestIncidenceFactor()
Returns Landscape::m_pestincidencefactor.
Definition: Landscape.h:325
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
virtual bool FA_SK(LE *a_field, double a_user, int a_days)
Apply SK fertilizer, on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1052
Definition: DE_Legumes.h:82
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
Definition: DE_Legumes.h:80
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: DE_Legumes.h:77
Definition: DE_Legumes.h:81
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DE_Legumes.h:72
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
Definition: DE_Legumes.h:76
The base class for all farm types.
Definition: Farm.h:755
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: LandscapeFarmingEnums.h:1012
void SetUpFarmCategoryInformation()
Definition: DE_Legumes.h:109
Definition: DE_Legumes.h:85
int DayInYear(void)
Definition: Calendar.h:58
#define DE_LEG_BASE
Definition: DE_Legumes.h:55
CfgBool cfg_pest_peas_on
Turn on pesticides for peas.
Definition: DE_Legumes.h:68
FarmEvent * m_ev
Definition: Farm.h:500
Definition: DE_Legumes.h:71
Definition: MapErrorMsg.h:34
DE_Legumes(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DE_Legumes.h:101
Definition: LandscapeFarmingEnums.h:1079
Definition: DE_Legumes.h:73
Definition: LandscapeFarmingEnums.h:1007
Definition: DE_Legumes.h:67
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751