Loading [MathJax]/extensions/ams.js
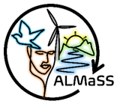 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
50 #ifndef DE_HERBSPERENNIAL_1YEAR_H
51 #define DE_HERBSPERENNIAL_1YEAR_H
53 #define DE_HP1Y_BASE 39000
131 #endif // DE_HERBSPERENNIAL_1YEAR_H
int GetMDates(int a, int b)
Definition: Elements.h:405
bool m_forcespring
Definition: Farm.h:392
Definition: DE_HerbsPerennial_1year.h:71
virtual bool PreseedingCultivator(LE *a_field, double a_user, int a_days)
Carry out preseeding cultivation on a_field (tilling set including cultivator and string roller to co...
Definition: FarmFuncs.cpp:312
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
bool IsStockFarmer(void)
Definition: Farm.h:961
bool m_lock
Definition: Farm.h:384
Definition: DE_HerbsPerennial_1year.h:69
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
Definition: DE_HerbsPerennial_1year.h:76
Definition: DE_HerbsPerennial_1year.h:66
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: DE_HerbsPerennial_1year.h:61
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
#define DE_HP1Y_BASE
Definition: DE_HerbsPerennial_1year.h:53
virtual bool SpringSow(LE *a_field, double a_user, int a_days, double a_seed_coating_amount=-1, PlantProtectionProducts a_ppp=ppp_foobar)
Carry out a sowing event in the spring on a_field.
Definition: FarmFuncs.cpp:501
Definition: DE_HerbsPerennial_1year.h:72
int m_base_elements_no
Definition: Farm.h:505
DE_HerbsPerennial_1yearToDo
Definition: DE_HerbsPerennial_1year.h:60
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: DE_HerbsPerennial_1year.cpp:82
int m_first_date
Definition: Farm.h:501
The base class for all crops.
Definition: Farm.h:495
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
Definition: DE_HerbsPerennial_1year.h:70
virtual bool GreenHarvest(LE *a_field, double a_user, int a_days)
GreenHarvest (remove of excess production that may affect the desired quality of the fruits,...
Definition: FarmFuncs.cpp:1942
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
DE_HerbsPerennial_1year(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DE_HerbsPerennial_1year.h:88
Definition: LandscapeFarmingEnums.h:1004
Definition: DE_HerbsPerennial_1year.h:63
long Date(void)
Definition: Calendar.h:57
Definition: DE_HerbsPerennial_1year.h:79
Definition: LandscapeFarmingEnums.h:1008
virtual bool FP_N(LE *a_field, double a_user, int a_days)
Apply N fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:700
Definition: LandscapeFarmingEnums.h:1003
Definition: DE_HerbsPerennial_1year.h:65
Definition: LandscapeFarmingEnums.h:513
long OldDays(void)
Definition: Calendar.h:60
Definition: DE_HerbsPerennial_1year.h:64
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
virtual bool FA_N(LE *a_field, double a_user, int a_days)
Apply N fertilizer, on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:713
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
Definition: DE_HerbsPerennial_1year.h:62
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
Definition: DE_HerbsPerennial_1year.h:73
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
int m_todo
Definition: Farm.h:388
Definition: DE_HerbsPerennial_1year.h:77
Definition: DE_HerbsPerennial_1year.h:68
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: DE_HerbsPerennial_1year.h:67
Definition: Elements.h:86
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DE_HerbsPerennial_1year.h:75
virtual bool AutumnHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the autumn on a_field.
Definition: FarmFuncs.cpp:285
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
Definition: LandscapeFarmingEnums.h:1012
virtual bool WinterPlough(LE *a_field, double a_user, int a_days)
Carry out a ploughing event in the winter on a_field.
Definition: FarmFuncs.cpp:395
Definition: DE_HerbsPerennial_1year.h:74
int DayInYear(void)
Definition: Calendar.h:58
void SetUpFarmCategoryInformation()
Definition: DE_HerbsPerennial_1year.h:96
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: DE_HerbsPerennial_1year.h:78
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: DE_HerbsPerennial_1year.h:84
virtual bool SpringHarrow(LE *a_field, double a_user, int a_days)
Carry out a harrow event in the spring on a_field.
Definition: FarmFuncs.cpp:459
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751
virtual bool CutWeeds(LE *a_field, double a_user, int a_days)
Carry out weed topping on a_field.
Definition: FarmFuncs.cpp:1629