File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
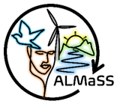 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
50 #ifndef DE_ASPARAGUSESTABLISHEDPLANTATION_H
51 #define DE_ASPARAGUSESTABLISHEDPLANTATION_H
53 #define DE_AEP_BASE 38900
58 #define CROP_HARVEST_DO m_field->m_user[1]
146 #endif // DE_ASPARAGUSESTABLISHEDPLANTATION_H
void SetUpFarmCategoryInformation()
Definition: DE_AsparagusEstablishedPlantation.h:106
int GetMDates(int a, int b)
Definition: Elements.h:405
Definition: DE_AsparagusEstablishedPlantation.h:74
Definition: DE_AsparagusEstablishedPlantation.h:66
Definition: LandscapeFarmingEnums.h:1005
virtual bool Do(Farm *a_farm, LE *a_field, FarmEvent *a_ev)
The one and only method for a crop management plan. All farm actions go through here.
Definition: DE_AsparagusEstablishedPlantation.cpp:89
Definition: DE_AsparagusEstablishedPlantation.h:73
Definition: DE_AsparagusEstablishedPlantation.h:75
virtual bool HerbicideTreat(LE *a_field, double a_user, int a_days)
Apply herbicide to a_field.
Definition: FarmFuncs.cpp:2025
Definition: DE_AsparagusEstablishedPlantation.h:83
bool IsStockFarmer(void)
Definition: Farm.h:961
Definition: DE_AsparagusEstablishedPlantation.h:79
bool m_lock
Definition: Farm.h:384
virtual bool ProductApplication(LE *a_field, double a_user, int a_days, double a_applicationrate, PlantProtectionProducts a_ppp, bool a_isgranularpesticide=false, int a_orcharddrifttype=0)
Apply test pesticide to a_field.
Definition: FarmFuncs.cpp:2267
A struct to hold the information required to trigger a farm event.
Definition: Farm.h:372
virtual bool Irrigation(LE *a_field, double a_user, int a_days, int a_max)
Generate an 'irrigation' event with a frequency defined by a_freq in the irrigation period on a_field...
Definition: FarmFuncs.cpp:1856
void ClearManagementActionSum()
clears the management action counters
Definition: Elements.h:247
Definition: DE_AsparagusEstablishedPlantation.h:80
DE_AsparagusEstablishedPlantationToDo
Definition: DE_AsparagusEstablishedPlantation.h:65
bool DoIt_prob(double a_probability)
Return chance out of 0 to 1.
Definition: Farm.cpp:864
class Calendar * g_date
Definition: Calendar.cpp:37
Definition: LandscapeFarmingEnums.h:1006
virtual bool FA_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1110
Definition: DE_AsparagusEstablishedPlantation.h:94
#define CROP_HARVEST_DO
A flag used to indicate autumn ploughing status.
Definition: DE_AsparagusEstablishedPlantation.h:58
int m_base_elements_no
Definition: Farm.h:505
Definition: DE_AsparagusEstablishedPlantation.h:68
Definition: DE_AsparagusEstablishedPlantation.h:67
int m_first_date
Definition: Farm.h:501
virtual bool FungicideTreat(LE *a_field, double a_user, int a_days)
Apply fungicide to a_field.
Definition: FarmFuncs.cpp:2101
virtual bool HillingUp(LE *a_field, double a_user, int a_days)
Do hilling up on a_field, probably of potatoes.
Definition: FarmFuncs.cpp:1302
The base class for all crops.
Definition: Farm.h:495
virtual bool FP_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:673
CfgArray_Double cfg_pest_product_amounts
Amount of pesticide applied in grams of active substance per hectare for each of the 10 pesticides.
Definition: DE_AsparagusEstablishedPlantation.h:89
bool value() const
Definition: Configurator.h:164
CfgBool cfg_pest_asparagus_on
Turn on pesticides for asparagus.
virtual bool FP_Manure(LE *a_field, double a_user, int a_days)
Spread manure on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:896
virtual bool IrrigationStart(LE *a_field, double a_user, int a_days)
Start a irrigation event on a_field today.
Definition: FarmFuncs.cpp:1834
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: LandscapeFarmingEnums.h:1004
Definition: DE_AsparagusEstablishedPlantation.h:88
Definition: DE_AsparagusEstablishedPlantation.h:84
long Date(void)
Definition: Calendar.h:57
Definition: LandscapeFarmingEnums.h:1008
virtual bool FP_N(LE *a_field, double a_user, int a_days)
Apply N fertilizer, on a_field owned by an arable farmer.
Definition: FarmFuncs.cpp:700
virtual bool HarvestShoots(LE *a_field, double a_user, int a_days)
HarvestShoots applied on a_field (e.g. asparagus) - details needs to be added (e.g....
Definition: FarmFuncs.cpp:1883
Bool configurator entry class.
Definition: Configurator.h:155
Definition: LandscapeFarmingEnums.h:1003
Definition: LandscapeFarmingEnums.h:1011
Definition: DE_AsparagusEstablishedPlantation.h:70
long OldDays(void)
Definition: Calendar.h:60
Definition: DE_AsparagusEstablishedPlantation.h:81
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
Definition: Configurator.h:208
virtual bool FA_N(LE *a_field, double a_user, int a_days)
Apply N fertilizer, on a_field owned by a stock farmer.
Definition: FarmFuncs.cpp:713
bool StartUpCrop(int a_spring, std::vector< std::vector< int >> a_flexdates, int a_todo)
Holds the translation between the farm operation enum for each cropand the farm management category a...
Definition: Farm.cpp:652
double SupplyPestIncidenceFactor()
Returns Landscape::m_pestincidencefactor.
Definition: Landscape.h:325
Definition: DE_AsparagusEstablishedPlantation.h:76
Farm * m_farm
Definition: Farm.h:498
LE * m_field
Definition: Farm.h:499
vector< FarmManagementCategory > m_ManagementCategories
Holds the translation between the farm operation enum for each crop and the farm management category ...
Definition: Farm.h:530
std::vector< double > value() const
Definition: Configurator.h:219
Landscape * g_landscape_ptr
Definition: Landscape.cpp:352
int m_todo
Definition: Farm.h:388
virtual bool InsecticideTreat(LE *a_field, double a_user, int a_days)
Apply insecticide to a_field.
Definition: FarmFuncs.cpp:2135
virtual bool ShallowHarrow(LE *a_field, double a_user, int a_days)
Carry out a shallow harrow event on a_field, e.g., after grass cutting event.
Definition: FarmFuncs.cpp:473
TTypesOfCrops
Definition: LandscapeFarmingEnums.h:781
Definition: DE_AsparagusEstablishedPlantation.h:69
virtual bool RowCultivation(LE *a_field, double a_user, int a_days)
Carry out a harrowing between crop rows on a_field.
Definition: FarmFuncs.cpp:1183
Definition: Elements.h:86
Definition: DE_AsparagusEstablishedPlantation.h:82
int m_last_date
Definition: Farm.h:503
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
Definition: DE_AsparagusEstablishedPlantation.h:87
Definition: DE_AsparagusEstablishedPlantation.h:77
#define DE_AEP_BASE
Definition: DE_AsparagusEstablishedPlantation.h:53
TTypesOfVegetation
Values that represent the types of vegetation that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:192
The base class for all farm types.
Definition: Farm.h:755
static int m_date_modifier
Holds a value that shifts test pesticide use by this many days in crops modified to use it.
Definition: Farm.h:514
Definition: DE_AsparagusEstablishedPlantation.h:72
Definition: LandscapeFarmingEnums.h:1012
DE_AsparagusEstablishedPlantation(TTypesOfVegetation a_tov, TTypesOfCrops a_toc, Landscape *a_L)
Definition: DE_AsparagusEstablishedPlantation.h:98
Definition: DE_AsparagusEstablishedPlantation.h:78
int DayInYear(void)
Definition: Calendar.h:58
virtual bool FA_PK(LE *a_field, double a_user, int a_days)
Apply PK fertilizer to a_field owned by an stock farmer.
Definition: FarmFuncs.cpp:1010
Definition: DE_AsparagusEstablishedPlantation.h:86
Definition: DE_AsparagusEstablishedPlantation.h:71
FarmEvent * m_ev
Definition: Farm.h:500
Definition: MapErrorMsg.h:34
Definition: LandscapeFarmingEnums.h:1079
Definition: DE_AsparagusEstablishedPlantation.h:85
Definition: LandscapeFarmingEnums.h:1007
FarmManagementCategory
Definition: LandscapeFarmingEnums.h:1001
Definition: LandscapeFarmingEnums.h:511
void SimpleEvent_(long a_date, int a_todo, bool a_lock, Farm *a_farm, LE *a_field)
Adds an event to this crop management without relying on member variables.
Definition: Farm.cpp:751