File failed to load: https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/extensions/ams.js
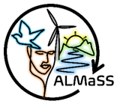 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
28 #ifndef Bembidion_allH
29 #define Bembidion_allH
CfgInt cfg_Beetlestartnos
The number of beetles to start in the simulation.
Definition: Treatment.h:129
Definition: Treatment.h:64
Definition: Treatment.h:84
Bembidion_Larvae1(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr)
constructor for Larvae1
Definition: Bembidion_All.cpp:63
bool IsStartHabitat(int a_x, int a_y) const
Used to specify legal starting habitats for simulation start-up.
Definition: Beetle_BaseClasses.cpp:1557
Class for beetle larval stage 1, most functionality is in Beetle_Larvae.
Definition: Bembidion_All.h:89
Definition: Treatment.h:71
Definition: Treatment.h:88
Definition: Treatment.h:68
Definition: Beetle_BaseClasses.h:69
Definition: Treatment.h:52
Definition: Treatment.h:89
CfgInt cfg_BeetleTotalNoEggs
The maximum number of eggs a beetle can produce.
double g_rand_uni_fnc()
Definition: ALMaSS_Random.cpp:56
Definition: Treatment.h:94
Definition: Treatment.h:142
int m_InFieldNo
In-field counter.
Definition: Beetle_BaseClasses.h:1117
TTypesOfVegetation TranslateVegTypes(int VegReference)
Returns vegetation type translated from the ALMaSS reference number.
Definition: Landscape.h:2326
virtual TAnimal * SupplyAnimalPtr(unsigned int a_index, unsigned int a_animal)
Returns the pointer indexed by a_index and a_animal. Note NO RANGE CHECK.
Definition: PopulationManager.h:678
void DoFirst() override
Does day degree development calculations here.
Definition: Bembidion_All.cpp:304
Definition: Treatment.h:83
Definition: Treatment.h:99
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: Bembidion_All.cpp:99
Definition: Treatment.h:76
Definition: Beetle_BaseClasses.h:86
CfgInt cfg_pm_EventFrequency
Definition: Treatment.h:35
Definition: Treatment.h:81
Definition: Bembidion_All.h:166
Definition: Treatment.h:43
Definition: Treatment.h:91
Definition: Treatment.h:127
Definition: Treatment.h:103
Bembidion_Pupae(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr)
constructor for pupae
Definition: Bembidion_All.cpp:107
TTypesOfPopulation m_population_type
Definition: PopulationManager.h:858
static int m_StartAggregatingDay
Definition: Beetle_BaseClasses.h:887
Definition: Treatment.h:121
void CreateObjects(int a_ob_type, TAnimal *a_pvo_ptr, void *null, Struct_Beetle *a_data, int a_number) override
Method to add beetles to the population.
Definition: Bembidion_All.cpp:253
Definition: Treatment.h:44
Definition: Treatment.h:56
Definition: Treatment.h:125
CfgBool cfg_ReallyBigOutput_used
double m_AdultEggLayingThreshold
Temperature threshold for egg laying.
Definition: Beetle_BaseClasses.h:1064
Definition: Treatment.h:61
Definition: Treatment.h:80
Definition: Treatment.h:60
Definition: Treatment.h:90
Definition: Treatment.h:123
Definition: Treatment.h:131
virtual double GetInsecticideApplication() const
the method overrides the method that returns the mortality due to insecticide application
Definition: Beetle_BaseClasses.h:367
Used to map locations of individuals for density estimates - space inefficient but good for testing.
Definition: PositionMap.h:45
int m_EggCounter
The number of eggs produced.
Definition: Beetle_BaseClasses.h:876
TTypesOfBeetleState CheckDormancy() override
Override for standard beetle CheckDormancy due to death test.
Definition: Bembidion_All.cpp:150
Definition: Treatment.h:138
Definition: Treatment.h:122
Definition: Treatment.h:39
Landscape * m_l
Definition: Beetle_BaseClasses.h:129
Bembidion_Larvae3(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr)
constructor for Larvae3
Definition: Bembidion_All.cpp:93
Definition: Treatment.h:110
Definition: Treatment.h:51
The class describing the Bembidion Egg_List objects. In general case this class should have only a co...
Definition: Bembidion_All.h:72
double value() const
Definition: Configurator.h:142
Definition: Treatment.h:143
Class for beetle larval stage 2, most functionality is in Beetle_Larvae.
Definition: Bembidion_All.h:103
FarmToDo
Definition: Treatment.h:31
static CfgArray_Double cfg_BembidionDevelopmentInflectionPoints("BEMBIDION_DEVELOPMENTINFLECTIONPOINTS", CFG_CUSTOM, 10, vector< double >{12.0, 12.0, 12.0, 12.0, 12.0, 9999, 9999, 9999, 9999, 9999})
Inflection point in day degree calculations - default bembidion.
Definition: Treatment.h:133
static int m_DayInYear
A holder for the day in year shared with all TAnimal objects.
Definition: PopulationManager.h:358
Definition: Treatment.h:104
Definition: Treatment.h:85
bool CheckForDispersal()
the method that checks for the dispersal
Definition: Beetle_BaseClasses.cpp:974
static CfgInt cfg_BembidionLarvalMortCategories("BEMBIDION_LARVALMORTCATEGORIES", CFG_CUSTOM, 7)
Definition: ALMaSS_Random.h:70
void CalculateDailyEggProduction(double a_temptoday) override
Figure out the maximum number of eggs that can be laid today - must be overridden in descendent class...
Definition: Bembidion_All.cpp:292
Definition: Treatment.h:140
TTypesOfBeetleState
The enumeration lists all beetle behavioural states used by all the beetle species.
Definition: Beetle_BaseClasses.h:58
Definition: Treatment.h:38
Definition: Treatment.h:117
unsigned m_Lifestage
This is a useful parameter holding the beetle type (this includes larval stages)
Definition: Beetle_BaseClasses.h:166
Definition: Treatment.h:120
double m_HibernateDegrees
The number of day degrees experienced in the spring which may trigger dispersal.
Definition: Beetle_BaseClasses.h:871
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: Bembidion_All.cpp:85
int m_y
Definition: Beetle_BaseClasses.h:128
Definition: Treatment.h:59
Definition: PopulationManager.h:62
Definition: Treatment.h:100
Class for beetle larval stage 3, most functionality is in Beetle_Larvae.
Definition: Bembidion_All.h:117
Definition: Treatment.h:124
Definition: Treatment.h:139
Definition: Treatment.h:126
CfgInt cfg_BeetleLarvalStagesNum
The number of larval stages for this species.
static void SetTodaysEggProduction(const int a_value)
Daily temperature determined egg production.
Definition: Beetle_BaseClasses.h:848
Definition: Treatment.h:149
Definition: Treatment.h:132
Definition: Treatment.h:148
Definition: Treatment.h:67
double calcDailyMortChance(int a_temp2, double a_lengthOfStageAtTemp) override
calculates the length of the pupal stage depending on the temperature
Definition: Bembidion_All.cpp:111
Landscape * m_TheLandscape
holds an internal pointer to the landscape
Definition: PopulationManager.h:624
Definition: Treatment.h:42
Definition: Treatment.h:65
Definition: Treatment.h:45
Definition: Treatment.h:98
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
Definition: Treatment.h:77
Definition: Treatment.h:96
double m_negDegrees
The number of negative day degrees experienced.
Definition: Beetle_BaseClasses.h:869
void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Beetle_Population_Manager *a_bpm_ptr) override
ReInit for object pool.
Definition: Beetle_BaseClasses.cpp:655
CfgInt cfg_BeetleHibernationEndDay
The latest day in a year for beetle to wake up from habination.
Definition: Treatment.h:54
Definition: Treatment.h:97
Definition: Treatment.h:141
static void SetLarvalDailyMort(const vector< double > &a_value, const int a_numLarvalStages, const int a_catagories)
Set the daily fixed mortality probability based on the larval stages and temperature.
Definition: Beetle_BaseClasses.h:582
The class describing the beetle Egg_List objects.
Definition: Beetle_BaseClasses.h:382
CfgInt cfg_BeetleInCropRef
A reference to a crop type if we are looking for mortality locations within a special crop.
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
int m_LarvalStage
Current larval growth stage (1-3), used as a small speed hack.
Definition: Beetle_BaseClasses.h:619
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
CfgBool cfg_RipleysOutput_used
Definition: Treatment.h:70
Definition: Treatment.h:46
The population manager class for beetles.
Definition: Beetle_BaseClasses.h:1006
const char * g_simulationName
Bool configurator entry class.
Definition: Configurator.h:155
Bembidion_Egg_List(int a_today, Bembidion_Population_Manager *a_bpm_ptr, Landscape *a_l_ptr)
Egg_List class constructor.
Definition: Bembidion_All.cpp:57
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
virtual void ReInit(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr)
ReInit for object pool.
Definition: Bembidion_All.cpp:70
Definition: Treatment.h:135
Definition: Treatment.h:82
Definition: Treatment.h:63
Movement maps are used for rapid computing of animal movement. This version uses values of 0-3 only.
Definition: MovementMap.h:88
Definition: Treatment.h:62
Definition: Treatment.h:119
Definition: Treatment.h:72
Definition: Treatment.h:112
Definition: Treatment.h:137
Definition: Treatment.h:40
Definition: Treatment.h:53
virtual double GetHarvestMortality() const
the method overrides the method that returns the mortality due to harvest
Definition: Beetle_BaseClasses.h:369
Definition: Treatment.h:145
CfgFloat cfg_biocide_reduction_val
Definition: Configurator.h:208
TTypesOfVegetation m_InCropRef
In crop tole reference.
Definition: Beetle_BaseClasses.h:1123
Definition: Treatment.h:86
int m_BeetleLarvalStagesNum
Definition: Beetle_BaseClasses.h:1058
int SupplyDayInYear(void)
Passes a request on to the associated Calendar class function, the day in the year.
Definition: Landscape.h:2267
bool m_CanReproduce_bool
Signal reproductive readiness.
Definition: Beetle_BaseClasses.h:969
Definition: Treatment.h:95
Definition: Treatment.h:147
Definition: Treatment.h:36
void DoFirst() override
Does day degree development calculations here.
Definition: Beetle_BaseClasses.cpp:1563
Definition: Treatment.h:116
Definition: Treatment.h:93
static double m_AdultDispersalThreshold
Adult dispersal temp threshold for DD calcuation.
Definition: Beetle_BaseClasses.h:921
double g_speedy_Divides[2001]
A generally useful array of fast divide calculators by multiplication.
Definition: Landscape.cpp:344
Definition: Treatment.h:114
int value() const
Definition: Configurator.h:116
Definition: Treatment.h:75
std::vector< double > value() const
Definition: Configurator.h:219
Definition: Beetle_BaseClasses.h:85
Definition: Treatment.h:47
Definition: Bembidion_All.h:131
Definition: Treatment.h:106
TTypesOfBeetleState m_CurrentBState
Current behavioural state.
Definition: Beetle_BaseClasses.h:158
double m_MortalityTempMax
Threshold temperatures for mortalities: Max.
Definition: Beetle_BaseClasses.h:1046
TTypesOfBeetleState St_Hibernate() override
Override for state hibernate for Bembidion.
Definition: Bembidion_All.cpp:173
The class describing the beetle larvae objects.
Definition: Beetle_BaseClasses.h:535
A data class for Beetle data.
Definition: Beetle_BaseClasses.h:125
The class describing the adult (female) beetle objects.
Definition: Beetle_BaseClasses.h:793
Definition: Treatment.h:146
Definition: Treatment.h:134
Definition: Bembidion_All.h:145
Definition: Treatment.h:118
Definition: Beetle_BaseClasses.h:88
Definition: Treatment.h:113
double m_EggProductionSlope
the slope of the linear function that defines todays egg production
Definition: Beetle_BaseClasses.h:1066
Definition: Treatment.h:41
int m_InCropNo
In-crop counter.
Definition: Beetle_BaseClasses.h:1119
Integer configurator entry class.
Definition: Configurator.h:102
static double m_TemperatureToday
A holder for the temperature today shared with all TAnimal objects.
Definition: PopulationManager.h:354
Definition: Treatment.h:37
Definition: Treatment.h:79
The class describing the beetle pupae objects.
Definition: Beetle_BaseClasses.h:688
void CheckReproduction() override
Starts reproduction when ready.
Definition: Bembidion_All.h:157
vector< double > m_DevelopmentInflectionPoints
Inflection point in day degree calculations for non-adult stages.
Definition: Beetle_BaseClasses.h:1070
Definition: Treatment.h:74
Definition: Beetle_BaseClasses.h:83
Double configurator entry class.
Definition: Configurator.h:126
string m_SimulationName
stores the simulation name
Definition: PopulationManager.h:622
bool OnFarmEvent(FarmToDo a_event, Beetle_Base *a_caller) override
Returns the consequence of farme events at a the current location.
Definition: Bembidion_All.cpp:323
Definition: Beetle_BaseClasses.h:71
Definition: Treatment.h:136
Definition: Treatment.h:69
Definition: Treatment.h:105
bool WinterMort() const override
The method for calculating overwintering mortality.
Definition: Bembidion_All.cpp:138
Definition: Treatment.h:78
Bembidion_Larvae2(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr)
constructor for Larvae2
Definition: Bembidion_All.cpp:78
Definition: Configurator.h:70
int g_random_fnc(const int a_range)
Definition: ALMaSS_Random.cpp:74
int GetStartAggregationDayProb() const
return the aggregation start day probability
Definition: Beetle_BaseClasses.cpp:903
Definition: Treatment.h:66
Definition: Treatment.h:92
Definition: Treatment.h:102
static CfgArray_Double cfg_BembidionLarvalDailyTemperatureMort("BEMBIDION_LARVALDAILYTEMPERATUREMORT", CFG_CUSTOM, 3 *7, vector< double >{ 0.0855, 0.0855, 0.1036, 0.0563, 0.0515, 0.0657, 0.0957, 0.0626, 0.0626, 0.0940, 0.0529, 0.0492, 0.0633, 0.0933, 0.0631, 0.0631, 0.0545, 0.0268, 0.0236, 0.0295, 0.0595 })
The daily fixed temperature related mmortality larval probability.
The base class for all beetles.
Definition: Beetle_BaseClasses.h:150
int m_OffFieldNo
Off-field counter.
Definition: Beetle_BaseClasses.h:1121
Definition: Treatment.h:57
Definition: Treatment.h:87
void PushIndividual(const unsigned a_listindex, TAnimal *a_individual_ptr)
Definition: PopulationManager.cpp:1682
Definition: Treatment.h:101
Definition: Beetle_BaseClasses.h:73
int m_x
Definition: Beetle_BaseClasses.h:127
Definition: Treatment.h:73
vector< vector< forward_list< TAnimal * > * > > TheSubArrays
Hold all the animal pointers.
Definition: PopulationManager.h:804
Definition: Treatment.h:55
void Warn(std::string a_msg1, std::string a_msg2)
Wrapper for the g_msg Warn function.
Definition: Landscape.h:2250
Definition: Beetle_BaseClasses.h:82
virtual double GetStriglingMortality() const
Mortality by Strigling: similar for all forms– should use base class method.
Definition: Beetle_BaseClasses.h:363
Definition: Treatment.h:34
Definition: Beetle_BaseClasses.h:87
virtual double GetSoilCultivationMortality() const
the method overrides the method that returns the mortality due to soil cultivation
Definition: Beetle_BaseClasses.h:365
Definition: Treatment.h:58
Definition: Treatment.h:128
Bembidion_Population_Manager(Landscape *a_l_ptr)
Constructor.
Definition: Bembidion_All.cpp:207
Definition: Beetle_BaseClasses.h:84
Definition: Beetle_BaseClasses.h:70
Bembidion_Adult(int a_x, int a_y, Landscape *a_l_ptr, Bembidion_Population_Manager *a_bpm_ptr)
constructor for Bembidion adult
Definition: Bembidion_All.cpp:133
Definition: Treatment.h:115
Definition: Treatment.h:33
Definition: Treatment.h:107
vector< std::array< double, 365 > > m_DayDegs
Storage for daily day degrees for non-adult stages.
Definition: Beetle_BaseClasses.h:1052
void IncLiveArraySize(int a_listindex)
Increments the number of 'live' objects for a list index in the TheArray.
Definition: PopulationManager.h:665