Loading [MathJax]/extensions/ams.js
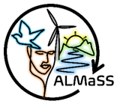 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
33 #ifndef SubPopulationAnimalH
34 #define SubPopulationAnimalH
114 SubPopulation(
int p_x,
int p_y,
Landscape* p_L,
SubPopulation_Population_Manager* p_NPM,
bool a_empty_flag,
int a_index_x,
int a_index_y,
double* p_suitability=NULL,
double* p_weight_density=NULL,
int number=-1,
int a_SpeciesID=999,
TTypesOfLandscapeElement p_winter_landscape_host=
tole_Foobar,
TTypesOfLandscapeElement p_summer_landscape_host=
tole_Foobar,
bool p_farm_flag=
false);
void removeAnimalNumGivenStage(int source_type)
Function to remove one life stage completely.
Definition: SubPopulation.cpp:142
unsigned m_SpeciesID
A unique ID number for this species.
Definition: SubPopulation.h:62
The class to handle all subpopulation-based ainimal population related matters in the whole landscape...
Definition: SubPopulation_Population_Manager.h:77
double * m_popu_density
Variable to store the weighted population density pointer.
Definition: SubPopulation.h:75
double SupplyGreenBiomass(int a_polyref)
Returns the green biomass of the vegetation using the polygon reference number a_polyref.
Definition: Landscape.h:1612
TTypesOfLandscapeElement m_winter_landscape_host
The flag to show whether it is a winter landscape type host.
Definition: SubPopulation.h:96
bool m_landing_local_move_flag
landing_enable_local_movement_flag
Definition: SubPopulation.h:110
SubPopulation(int p_x, int p_y, Landscape *p_L, SubPopulation_Population_Manager *p_NPM, bool a_empty_flag, int a_index_x, int a_index_y, double *p_suitability=NULL, double *p_weight_density=NULL, int number=-1, int a_SpeciesID=999, TTypesOfLandscapeElement p_winter_landscape_host=tole_Foobar, TTypesOfLandscapeElement p_summer_landscape_host=tole_Foobar, bool p_farm_flag=false)
Subpopulation constructor.
Definition: SubPopulation.cpp:49
TTypesOfVegetation SupplyVegType(int a_x, int a_y)
Returns the vegetation type of the polygon using the polygon reference number a_polyref or coordinate...
Definition: Landscape.h:1925
TTypesOfLandscapeElement SupplyElementType(int a_polyref)
Returns the landscape type of the polygon using the polygon reference number a_polyref or coordinates...
Definition: Landscape.h:1732
void addAnimalNumGivenStageColumn(int source_type, int a_column, double a_num)
Add animal number for the given life stage at specific column (age) in the animal number array.
Definition: SubPopulation.cpp:100
virtual void Step()
Step behaviour - must be implemented in descendent classes.
Definition: SubPopulation.cpp:153
Definition: LandscapeFarmingEnums.h:183
virtual double calBioticMortalityRate(int a_life_stage)
The function to calculate the biotic mortalit rate.
Definition: SubPopulation.h:158
SubPopulation_Object
Definition: SubPopulation.h:42
virtual void doReproduction()
Definition: SubPopulation.cpp:294
unsigned getSpeciesID()
A typical interface function - this one returns the SpeciesID number as an unsigned integer.
Definition: SubPopulation.h:117
virtual void doDevelopment()
Definition: SubPopulation.cpp:172
blitz::Array< double, 1 > m_died_number_each_life_stage
Array used for mortality.
Definition: SubPopulation.h:90
double m_parasitoid_egg_num
The variable to recourd the number of parasitoid egg.
Definition: SubPopulation.h:108
void setSpeciesID(unsigned a_spID)
A typical interface function - this one returns the SpeciesID number as an unsigned integer.
Definition: SubPopulation.h:119
virtual void killByParasitoid()
The function to kill subpopulation by parasitoid.
Definition: SubPopulation.h:166
virtual void setParasitoidEggNum(double a_num)
The function to set the parasitoid egg number.
Definition: SubPopulation.h:162
bool m_empty_cell
Flag to show whether it is an empty subpopulation cell.
Definition: SubPopulation.h:60
blitz::Array< double, 1 > getArrayForOneLifeStage(int life_stage)
Get the numbers of the given life stage at different ages.
Definition: SubPopulation.cpp:209
SubPopulation_Population_Manager * m_OurPopulationManager
This is a time saving pointer to the correct population manager object.
Definition: SubPopulation.h:73
bool supplyFirstFlagLifeStage(int life_stage)
The function to supply the flag of first for the given life stage.
Definition: SubPopulation_Population_Manager.h:276
virtual ~SubPopulation()=default
Definition: SubPopulation.h:47
Definition: SubPopulation.h:43
Definition: SubPopulation.h:45
int supplyNumFlyingLifeStages()
Supply the number of life stages that can fly.
Definition: SubPopulation_Population_Manager.h:319
int supplyNumLocMovLifeStages()
Supply the number of life stages thah can do local movement.
Definition: SubPopulation_Population_Manager.h:323
bool isSummerHostTole(TTypesOfLandscapeElement a_ele)
Test whether it is a summer host tole.
Definition: SubPopulation_Population_Manager.cpp:810
double getFlyingNum(int ind)
Supply the number of winged ones that want to fly away,.
Definition: SubPopulation.h:152
virtual void doDropingWings()
For some specices, they drop the wings at some situation.
Definition: SubPopulation.h:145
blitz::Array< double, 1 > getFlyingArray(int ind)
Supply the flying array for flying.
Definition: SubPopulation.h:150
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
double supplySizeSubpopulationCell()
Supply the size of aphid subpopulation cell.
Definition: SubPopulation_Population_Manager.h:228
double getPrasitoidEggNum()
Definition: SubPopulation.h:171
TTypesOfLandscapeElement
Values that represent the types of landscape polygon that are represented in ALMaSS.
Definition: LandscapeFarmingEnums.h:57
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
blitz::Array< double, 2 > temp_mortality_array
The array used for mortality calculation.
Definition: SubPopulation.h:64
virtual double calCellRelatedMortalityWeight()
This function is used to calculate the cell realted weight to multiply with the temperature and age b...
Definition: SubPopulation.h:148
blitz::Array< double, 2 > m_local_moving_array
Array used to store the number of adults willing for local movement.
Definition: SubPopulation.h:94
std::vector< int > m_summer_landscape_host_period
The starting and ending month for the summer landscape host.
Definition: SubPopulation.h:102
virtual void setParasitoidNum(double a_num)
The function to set the parasitoid number.
Definition: SubPopulation.h:160
int m_index_y
Variable to store the index of y in the population manager.
Definition: SubPopulation.h:71
Definition: MapErrorMsg.h:43
int m_Location_y
The objects ALMaSS y coordinate.
Definition: PopulationManager.h:366
virtual int calNextStage(int current_stage, double density=1)
Return the next life stage for the given life stage.
Definition: SubPopulation_Population_Manager.cpp:659
static Landscape * m_OurLandscape
A pointer to the landscape object shared with all TAnimal objects.
Definition: PopulationManager.h:342
double getTotalSubpopulation(void)
Return totalpopulation in the cell.
Definition: SubPopulation.h:123
The base class for all ALMaSS animal classes. Includes all the functionality required to be handled b...
Definition: PopulationManager.h:200
void addAnimalNumGivenStage(int source_type, blitz::Array< double, 1 > *a_animal_num_array)
Add animal number for the given life stage.
Definition: SubPopulation.cpp:121
Definition: SubPopulation.h:46
void setFlyLocalMovFlag(bool a_flag)
The function to set the flag to enable local movement triged by landing activity.
Definition: SubPopulation.h:174
blitz::Array< double, 1 > m_temp_droping_wings_array
Array used for droping wings.
Definition: SubPopulation.h:88
bool isFarm()
The function to return whether it is part of farm.
Definition: SubPopulation.h:178
TTypesOfLandscapeElement m_summer_landscape_host
The flag to show whether it is a summer landscape type host.
Definition: SubPopulation.h:98
double * m_suitability
Variable to store the suitability pointer.
Definition: SubPopulation.h:77
double getNumforOneLifeStage(int source_type)
Return the number of animals in a specific life stage in the cell.
Definition: SubPopulation.cpp:95
int supplyLifeStageNum()
Supply the number of life stage.
Definition: SubPopulation_Population_Manager.h:242
void setSummerHostPeriod(int start_month, int end_month)
Set the available period for summer landscape host.
Definition: SubPopulation.h:156
std::vector< int > m_winter_landscape_host_period
The staring and ending month for the winter landscape host.
Definition: SubPopulation.h:100
int supplyMaxColNum()
Supply the maximum number of column in the development array.
Definition: SubPopulation_Population_Manager.h:244
blitz::Array< double, 2 > m_animal_num_array
Variables to record numbers of the animals at different life stages and age in canlander days.
Definition: SubPopulation.h:83
virtual void BeginStep()
BeingStep behaviour - must be implemented in descendent classes.
Definition: SubPopulation.h:179
double getPrasitoidNum()
The function to supply the parasitoid number.
Definition: SubPopulation.h:170
std::vector< int > supplyOldEnoughIndex(int life_stage_index)
Definition: SubPopulation_Population_Manager.h:247
Definition: SubPopulation.h:44
double m_parasitoid_num
The variable to record the number of parasitoid.
Definition: SubPopulation.h:106
void addAnimalFromAnotherCell(int source_type, int a_column, double a_num)
Add the number of animal at the given source and column that moved from another grid.
Definition: SubPopulation.cpp:337
blitz::Array< double, 2 > supplyMortalityWholeArray(void)
The function to supply the whole base mortality rate array.
Definition: SubPopulation_Population_Manager.h:298
int supplySuitability()
Definition: SubPopulation.h:172
virtual void giveBirthParasitoidEgg(double a_egg_num)
The function to give birth of parasitiod eggs.
Definition: SubPopulation.h:164
virtual void calPopuDensity(void)
Function to calculate the weighted population density for the cell.
Definition: SubPopulation.cpp:350
double removeAnimalPortionGivenStageColumn(int source_type, int a_column, double a_prop)
Remove propotion of animals for the given species and age.
Definition: SubPopulation.cpp:326
virtual void hatchParasitoidEggs()
The function to hatch parasitoid eggs.
Definition: SubPopulation.h:168
bool m_farm_flag
The flag to show whether it is part of farm.
Definition: SubPopulation.h:104
bool isNewlyLanded()
Supply whether it is newly landed grid.
Definition: SubPopulation.h:176
void setFirstFlagLifeStage(int life_stage, bool pvalue)
The function to set the flag indicating whether a given lifestage is the first time of existing.
Definition: SubPopulation_Population_Manager.h:274
The class for base animal using subpopulation method.
Definition: SubPopulation.h:56
double m_whole_population_in_cell
Variable to track the whole population in the current cell.
Definition: SubPopulation.h:79
void setWinterHostPeriod(int start_month, int end_month)
Set the available period for winter landscape host.
Definition: SubPopulation.h:154
int m_index_x
Variable to store the index of x in the population manager.
Definition: SubPopulation.h:69
int m_Location_x
The objects ALMaSS x coordinate.
Definition: PopulationManager.h:362
Definition: SubPopulation.h:48
virtual int calOffspringStage(int current_stage, double *offspring_num=NULL, double a_age=1, double a_density=-1, double a_growth_stage=0, double *a_propotion=NULL, bool winter_host_flag=false)
Calculate the offspring life stage.
Definition: SubPopulation_Population_Manager.cpp:1117
virtual void doMovement()
Definition: SubPopulation.cpp:312
void setOldIndex(int life_stage, int p_value)
The function to set the oldest index for the given life stage.
Definition: SubPopulation_Population_Manager.cpp:701
blitz::Array< double, 2 > m_flying_array
Array used to store the number of winged adults willing to fly away.
Definition: SubPopulation.h:92
virtual void calSuitability(void)
Function to calculate the suitability for the cell.
Definition: SubPopulation.cpp:343
blitz::Array< double, 2 > m_temp_animal_num_array
Tempraral variables for manipulting the animal number array.
Definition: SubPopulation.h:85
bool isSummerHostTov(TTypesOfVegetation a_ele)
Test whether it is a summer host tov.
Definition: SubPopulation_Population_Manager.cpp:830
blitz::Array< double, 1 > m_population_each_life_stage
Array to hold the population number for each life stage.
Definition: SubPopulation.h:81
int supplyNewestIndex(int life_stage_index)
Definition: SubPopulation_Population_Manager.h:248
std::vector< int > supplyVecFlyingLifeStages()
Supply the vector of life stages for flying.
Definition: SubPopulation_Population_Manager.h:317
virtual void doMortality()
Definition: SubPopulation.cpp:213