Loading [MathJax]/extensions/ams.js
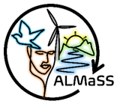 |
ALMaSS
1.2 (after EcoStack, March 2024)
The Animal, Landscape and Man Simulation System
|
Go to the documentation of this file.
68 for (
unsigned x = a_x; x<a_x+a_range; x++)
70 for (
unsigned y = a_y; y<a_y+a_range; y++)
77 for (
unsigned x = a_x; x<a_x+a_range; x++)
79 for (
unsigned y = a_y; y<a_y+a_range; y++)
120 #ifdef Poecilus_Debug
140 for (
unsigned x = a_x; x <a_x+a_range; x++)
144 for (
unsigned y = a_y; y < a_y+a_range; y++){
157 for (
unsigned x = a_x; x < a_x + a_range; x++)
159 for (
unsigned y = a_y; y < a_y + a_range; y++)
166 for (
unsigned x = a_x; x < a_x + a_range; x++)
168 for (
unsigned y = a_y; y < a_y + a_range; y++)
180 for (
unsigned i = 0; i < sz; i++) nos +=
m_TheMapSpmi[i];
188 template<
typename AnimalTypes>
200 virtual void AddMapValue(
unsigned a_x,
unsigned a_y, AnimalTypes* a_value)
237 virtual bool IsMapValue(
unsigned a_x,
unsigned a_y, AnimalTypes* a_value)
250 for (
unsigned x = a_x; x < a_x + a_range; x++)
252 for (
unsigned y = a_y; y < a_y + a_range; y++)
262 for (
unsigned x = a_x; x < a_x + a_range; x++)
264 for (
unsigned y = a_y; y < a_y + a_range; y++)
271 for (
unsigned x = a_x; x < a_x + a_range; x++)
273 for (
unsigned y = a_y; y < a_y + a_range; y++)
285 for (
unsigned i = 0; i < sz; i++) nos +=
m_TheMapSpmi[i]->size();
293 template <
typename AnimalTypes>
296 template <
typename AnimalTypes>
300 m_TheMapSpmi.resize(m_maxx * m_maxy);
301 for (
unsigned y = 0; y < m_maxy; y++)
303 for (
unsigned x = 0; x < m_maxx; x++)
305 m_TheMapSpmi[x + y * m_maxx] =
new vector<AnimalTypes*>();
309 template <
typename AnimalTypes>
311 for (
unsigned y = 0; y < m_maxy; y++)
313 for (
unsigned x = 0; x < m_maxx; x++)
315 delete m_TheMapSpmi[x + y * m_maxx];
365 for (
unsigned x = a_x; x<a_x+a_range; x++)
367 for (
unsigned y = a_y; y<a_y+a_range; y++)
377 for (
unsigned x = a_x; x<a_x+a_range; x++)
379 for (
unsigned y = a_y; y<a_y+a_range; y++)
419 unsigned int nCount=0 ;
~ScalablePositionMap()
Definition: PositionMap.cpp:568
int m_ScaleFactor
A binary scaling factor ( 1 = 2x2m 2=4x4m 3 =8x8m etc.. )
Definition: PositionMap.h:328
unsigned int m_maxx
Definition: PositionMap.h:94
int GetMapDensity5x5(unsigned x, unsigned y)
Definition: PositionMap.cpp:416
void SetMapValue(unsigned x, unsigned y)
Definition: PositionMap.cpp:184
void ClearMapValue(unsigned x, unsigned y)
Definition: PositionMap.cpp:197
virtual int GetMapDensity(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:129
int GetTotalSpace(int x, int y)
Definition: PositionMap.cpp:221
virtual int GetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:95
PointerInt * m_TheMap
Definition: PositionMap.h:394
#define __3264minus1
Definition: PositionMap.cpp:45
PositionMap(unsigned a_size)
Definition: PositionMap.cpp:137
Used to map locations of individuals for density estimates - space inefficient but good for testing.
Definition: PositionMap.h:45
virtual ~SimplePositionMap()
Definition: PositionMap.cpp:91
virtual void DecMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:115
ScalablePositionMap(Landscape *L, int a_ScaleFactor)
Definition: PositionMap.cpp:549
unsigned int m_maxy
Definition: PositionMap.h:94
virtual ~SimplePositionMapPointers()
Definition: PositionMap.h:310
virtual int GetMapDensityEdge(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:152
bool GetMapPositive(unsigned x, unsigned y, unsigned range)
Definition: PositionMap.cpp:348
virtual void ClearMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:60
bool GetMapPositive(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:372
virtual void SetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:55
Definition: PositionMap.h:189
virtual int GetMapDensity(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:247
virtual int GetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:195
void Init()
Definition: PositionMap.cpp:156
bool GetMapDensity32(unsigned x, unsigned y)
Definition: PositionMap.cpp:484
#define __3264minus0
Definition: PositionMap.cpp:46
virtual bool IsMapValue(unsigned a_x, unsigned a_y, AnimalTypes *a_value)
Definition: PositionMap.h:237
Used to map locations of individuals for density estimates.
Definition: PositionMap.h:391
void ClearMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:353
vector< vector< AnimalTypes * > * > m_TheMapSpmi
Definition: PositionMap.h:192
virtual void SetMapValue(unsigned a_x, unsigned a_y, int a_value)
Definition: PositionMap.h:100
#define __3264divide
Definition: PositionMap.cpp:47
uint64 PointerInt
Definition: ALMaSS_Setup.h:43
virtual int SumMap()
Definition: PositionMap.h:280
The landscape class containing all environmental and topographical data.
Definition: Landscape.h:142
int GetMapDensity(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:359
virtual bool GetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:50
PointerInt m_TheBitMaskArray[65]
Definition: PositionMap.h:396
int GetMapValue(unsigned x, unsigned y)
Definition: PositionMap.cpp:209
int SupplySimAreaHeight(void)
Gets the simulation landscape height.
Definition: Landscape.h:2302
int SupplySimAreaWidth(void)
Gets the simulation landscape width.
Definition: Landscape.h:2297
virtual bool GetMapPositive(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:164
int GetTotalN(int x, int y)
Definition: PositionMap.cpp:237
PointerInt m_maxy
Definition: PositionMap.h:395
PointerInt m_TheBitMaskArray2[65]
Definition: PositionMap.h:397
vector< int > m_TheMapSpmi
Definition: PositionMap.h:93
virtual void ClearMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:206
void Warn(MapErrorState a_level, std::string a_msg1, std::string a_msg2)
Definition: MapErrorMsg.cpp:69
PointerInt m_xmaxx
Definition: PositionMap.h:395
Definition: PositionMap.h:90
virtual ~SimplePositionMapInt()
Definition: PositionMap.cpp:119
PointerInt BitCount(PointerInt n)
Definition: PositionMap.h:418
void SetMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:347
virtual void AddMapValue(unsigned a_x, unsigned a_y, AnimalTypes *a_value)
Definition: PositionMap.h:200
Used to map locations of individuals for density estimates. Each cell can only contain one value (it ...
Definition: PositionMap.h:324
SimplePositionMapPointers()
Definition: PositionMap.h:294
SimplePositionMap()
Definition: PositionMap.cpp:55
virtual void ClearMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:105
virtual bool RemoveMapValue(unsigned a_x, unsigned a_y, AnimalTypes *a_value)
Definition: PositionMap.h:225
int GetMapDensity(unsigned x, unsigned y)
Definition: PositionMap.cpp:253
bool GetMapValue(unsigned a_x, unsigned a_y)
Get the value a x,y.
Definition: PositionMap.h:334
bool GetMapPositiveB(unsigned x, unsigned y, unsigned range)
Definition: PositionMap.cpp:520
~PositionMap()
Definition: PositionMap.cpp:149
MapErrorMsg * g_msg
Definition: MapErrorMsg.cpp:41
unsigned int m_maxx
Definition: PositionMap.h:49
SimplePositionMapInt()
Definition: PositionMap.cpp:98
Landscape * m_ALandscape
Definition: PositionMap.h:413
virtual void IncMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:110
PointerInt m_maxx
Definition: PositionMap.h:395
bool * m_TheMap
Definition: PositionMap.h:48
unsigned int m_maxy
Definition: PositionMap.h:49
virtual bool GetMapPositive(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:269
virtual int GetMapDensity(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:65
unsigned int m_maxx
Definition: PositionMap.h:193
virtual bool GetMapPositive(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:75
virtual int SumMap()
Definition: PositionMap.h:175
virtual AnimalTypes * DecMapValue(unsigned a_x, unsigned a_y)
Definition: PositionMap.h:213
Definition: MapErrorMsg.h:34
virtual int GetMapDensityEdge(unsigned a_x, unsigned a_y, unsigned a_range)
Definition: PositionMap.h:257
unsigned int m_maxy
Definition: PositionMap.h:193